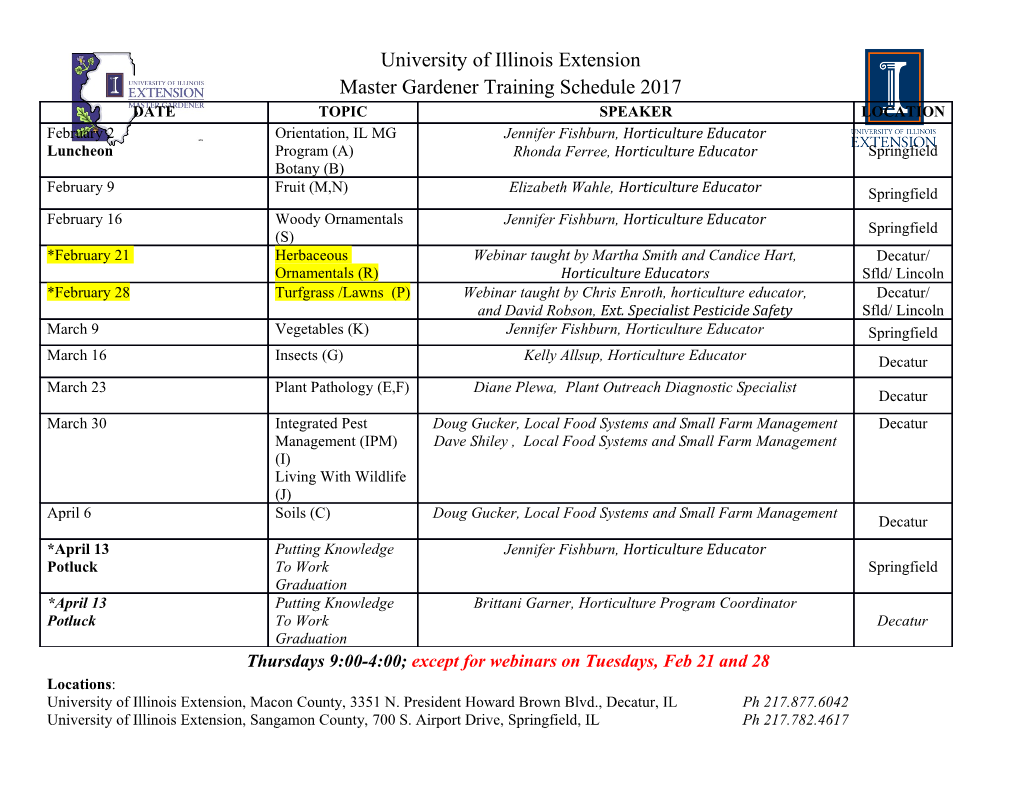
Python Programming for the Mathematically Literate A Brief Introduction S. R. Doty Department of Mathematics and Statistics Loyola University Chicago Chicago, IL 60660 USA c 2013 All rights reserved September 9, 2013 Contents 1 Preliminaries 1 1.1 WhatisPython? ........................ 1 1.1.1 Freeandopen-sourcesoftware . 2 1.1.2 Libraries......................... 2 1.1.3 Sage ........................... 2 1.2 Installationanddocumentation . 3 1.3 Python2versusPython3 ................... 3 2 Getting Started 4 2.1 RunningPythoninaterminal . 4 2.1.1 Integerdivision ..................... 6 2.1.2 Variablesandassignment. 6 2.1.3 Themagicunderscorevariable. 7 2.1.4 Compoundcommands . 7 2.2 RunningPythoninIDLE ................... 8 2.3 Quittingtheinterpreter . 9 2.4 Loadingcommandsfromthelibrary. 9 2.4.1 Importingfunctions. 9 2.4.2 Importingmodules . 10 2.5 Definingfunctions........................ 10 2.5.1 Reservedwords ..................... 12 2.5.2 Lambdafunctions. 12 2.6 Files ............................... 12 ii CONTENTS iii 2.6.1 RunninginaTerminal . 13 2.6.2 Importingfromthecommandline . 13 2.6.3 UsingIDLE ....................... 14 2.7 Thedevelopmentcycle . 14 2.7.1 Syntaxerrors ...................... 15 2.7.2 Logicalerrors ...................... 15 2.7.3 Exceptions........................ 15 2.7.4 Testing.......................... 16 2.8 Scripts.............................. 16 3 Python Commands 18 3.1 Commentsanddocstrings . 18 3.2 Numbersandotherdatatypes. 19 3.2.1 The type function ................... 19 3.2.2 Strings.......................... 20 3.2.3 Listsandtuples..................... 21 3.2.4 The range function................... 21 3.2.5 Booleanvalues ..................... 23 3.3 Expressions ........................... 23 3.3.1 Impliedcontinuation . 24 3.4 Operators ............................ 24 3.4.1 Arithmeticoperators . 25 3.4.2 Comparisonoperators . 26 3.4.3 Membershiptesting. 26 3.5 Variablesandassignment. 27 3.5.1 Variablenamingconventions . 27 3.5.2 Assignmentstatements . 27 3.6 Decisions............................. 29 3.7 Loops .............................. 30 3.7.1 for loop......................... 30 3.7.2 while loop........................ 31 iv CONTENTS 3.7.3 break, continue, and pass .............. 31 3.7.4 else inloops ...................... 33 3.8 Lists ............................... 33 3.8.1 Lengthofalist;theemptylist. 34 3.8.2 Sublists(slicing) . 35 3.8.3 Joiningtwolists. 36 3.8.4 Multiplyingalistbyanumber. 36 3.8.5 Listmethods ...................... 37 3.8.6 Listcomprehensions . 38 3.9 Strings.............................. 40 4 Developing Functions 42 4.1 Whyfunctions? ......................... 43 4.2 Listproblems .......................... 45 4.2.1 Maximumofalist ................... 45 4.2.2 Searchingalist ..................... 46 4.2.3 Findingthefirstandlastmaximum . 46 4.2.4 Reversingalist ..................... 47 4.2.5 Meanandstandarddeviation . 48 4.2.6 Sortingalist ...................... 49 4.2.7 Binarysearch ...................... 50 4.3 Arithmetic............................ 51 4.3.1 Computingfactorials . 51 4.3.2 Binaryrepresentation. 52 4.3.3 Decimalexpansionsoffractions . 53 4.4 Calculus ............................. 54 4.4.1 Derivatives ....................... 54 4.4.2 Trapezoidalrule. 56 4.4.3 Simpson’srule...................... 56 4.4.4 Approximating e .................... 57 4.5 Numbertheory ......................... 60 CONTENTS v 4.5.1 Pythagoreantriples. 60 4.5.2 Dayoftheweekfromthedate. 60 4.5.3 Greatestcommondivisor. 61 4.5.4 ExtendedEuclideanalgorithm . 62 4.5.5 Smallestfactorofanumber . 64 4.5.6 Primefactorizationofanumber . 67 5 Programming with class 68 5.1 Getting started with classes ................. 69 5.1.1 Afirstexample ..................... 69 5.1.2 Secondexample..................... 72 5.1.3 A word about self ................... 74 5.1.4 Built-intypesareclasses,too . 74 5.1.5 Specialmethodsforoperators . 76 5.2 Modulararithmetic . 78 5.3 Rationalnumbers........................ 81 5.4 Polynomialswithintegercoefficients . 85 Preface This book is a short introduction to the Python programming language intended for mathematicians and scientists, teachers and students. The main focus is on using Python to do interesting mathematical calculations, with an emphasis on learning by example. A certain amount of basic mathematical literacy on the part of the reader is assumed throughout. No prior experience with any computer programming language is assumed, although some such previous exposure would be very helpful. Python is a concise, elegant, yet powerful programming language espe- cially adaptable to the purpose of mathematical calculations. Its syntax is especially attractive since it often mimics mathematical notation. Moreover, as an interpreted language, it is especially easy to test Python code using the interpreter. Arbitrary-precision integer arithmetic is supported “out of the box” and it isn’t necessary to declare the type of data. The “batter- ies included” philosophy of Python means that many standard techniques and algorithms are already available in its standard library. Python is used worldwide as a convenient scripting language for web pages. Furthermore, there is now a powerful open-source alternative to Mathematica called Sage which is built on Python, so familiarity with Python enables people to use Sage for many complicated mathematical calculations. I believe that for people who already know something about mathematics and standard mathematical notation, a language such as Python can be mastered faster and better through the study of non-trivial mathematically oriented examples. All the myriad ways of creating strings that say “Hello world” seem to be fairly ubiquitous fare in the current introductory computer programming courses these days, but you won’t find such things a focus of this book. Indeed, there is little discussion of strings herein. Many important topics are, intentionally, left out. I don’t discuss with and try statements, format strings, dictionaries, data files, sets, pickling, or vi CONTENTS vii exception classes. None of the ways in which Python can be used to interact with operating systems and web pages is discussed. There is little discussion of what’s in the standard library. These things can be found in the extensive Python documentation, or one of the many other books on Python that are now available. The main focus here is on the core language and how to use its capabil- ities, especially for mathematical calculations. This document is intended for mathematically literate people just getting started with Python, and I believe that an introduction should not try to be comprehensive. Object- oriented programming is discussed, in Chapter 5, and several examples are given there, but I don’t give any examples of inheritance. In fact, Chapter 5 is rather terse and also quite a bit more abstract than the other chapters. Thanks are due to Andy Harrington who found a number of errors in a previous version, and suggested numerous improvements. —S.R. Doty, Chicago 2013 viii CONTENTS Chapter 1 Preliminaries 1.1 What is Python? Python is an easy to learn, powerful programming language, initially devel- oped by Guido van Rossum. It has efficient high-level data structures and a simple but effective approach to object-oriented programming. Python’s elegant syntax and dynamic typing, together with its interpreted nature, make it an ideal language for scripting and rapid application development in many areas on most platforms. Python is named after the famed British humor group Monty Python’s Flying Circus. This document focuses on learning Python for the purpose of doing math- ematical calculations. We assume the reader has a decent knowledge of basic mathematics, but we try not to assume previous exposure to computer pro- gramming, although some such exposure would certainly be helpful. Python is a good choice for mathematical calculations, since in Python we can write code quickly and test it easily, and the syntax of Python code is similar to the way mathematical ideas tend to be expressed by mathematicians and scientists. Furthermore, Python supports arbitrary precision integer arith- metic out of the box, and as an interpreted language it can be used as a powerful calculator to perform mathematical calculations. Python is also a popular tool used by web developers; especially by a certain company called Google. If you poke around on YouTube you can find videos of Python courses held at Google’s headquarters in Mountain View, California. For readers with prior programming experience, Python allows you to 1 2 CHAPTER 1. PRELIMINARIES use variables without declaring them (i.e., it determines types implicitly), and it relies on indentation as a control structure. You are not forced to define classes of objects in Python (unlike Java) but you are free to do so when convenient. 1.1.1 Free and open-source software Python is free software in the sense of Richard M. Stallman1, meaning that using it guarantees certain freedoms such as the freedom to give away copies and to study or change the source code. Python is also free in the sense that you can get it without spending any money. 1.1.2 Libraries Python itself is a fairly compact language, but it comes with many libraries of standard functions that extend its capabilities in many powerful ways. In addition to the standard libraries that come with any Python installation by default, there are many other libraries that one can install to make Python even more powerful. For the purposes of mathematical
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages103 Page
-
File Size-