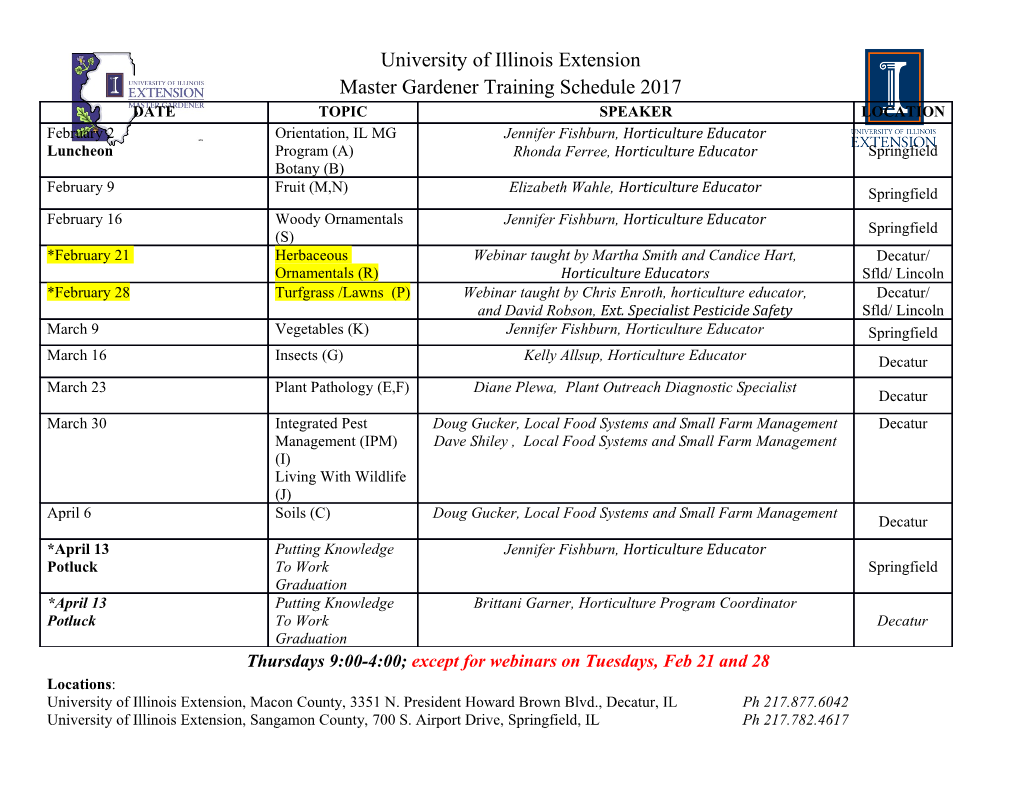
Module 5 – JavaScript, AJAX, and jQuery • Module 5 Contains an Individual and Group component – Both are due on Wednesday Oct 23rd – Start early on this module – One of the most time consuming modules in the course • Read the WIKI before starting along with a few JavaScript and jQuery tutorials 1Extensible- CSE 330 – Creative Networking Programming Platform and Rapid Prototyping 1 Module 5 • JavaScript – Scripting language to add interactivity to HTML pages – Java and JavaScript are completely different languages • AJAX – Asynchronous JavaScript and XML (AJAX) – Allows for retrieval of data from weB servers in the Background • jQuery – JavaScript liBrary 2Extensible- CSE 330 – Creative Networking Programming Platform and Rapid Prototyping 2 JavaScript • Popular scripting language widely supported in browsers to add interaction – Pop-Up Windows – User Input Forms – Calculations – Special Effects • Implementation of the ECMAScript language 3Extensible- CSE 330 – Creative Networking Programming Platform and Rapid Prototyping 3 JavaScript • JavaScript is a prototyped-based scripting language – Dynamic, weakly typed • Interpreted language – You do not compile it • Uses prototypes instead of classes for inheritance 4Extensible- CSE 330 – Creative Networking Programming Platform and Rapid Prototyping 4 Is JavaScript like Java? • Java and JavaScript are similar like Car and Carpet are similar • Two completely different languages with different concepts – Java is compiled, JavaScript is interpreted – Java is object-oriented, JavaScript is prototyped based – Java requires strict typing, JavaScript allows for dynamic typing 5Extensible- CSE 330 – Creative Networking Programming Platform and Rapid Prototyping 5 JavaScript Basics • Declare a variable with let let foo = “JS is fun”; • Declare a constant with const const bar = ”This is will not change”; • Older versions of JavaScript do not support let and const and use var instead – In this course we will use let and const and avoid using var • Define a function with function function foo() { //Some JS code here } • Functions are also objects 6Extensible- CSE 330 – Creative Networking Programming Platform and Rapid Prototyping 6 Writing a JavaScript Program • JavaScript programs can either be placed – directly into the HTML file or – can be saved in external files • You can place JavaScript anywhere within the HTML file: within – the <HEAD> tags or – the <BODY> tags 7Extensible- CSE 330 – Creative Networking Programming Platform and Rapid Prototyping 7 Using the <SCRIPT> Tag <script src=url></script> – SRC property is required only if you place your program in a separate file <script> script commands and comments </script> • Older versions of HTML (before 5) use a slightly different format – <script type=“text/javascript”> – This is no longer necessary, simply use <script> 8Extensible- CSE 330 – Creative Networking Programming Platform and Rapid Prototyping 8 JavaScript Example (part 1) <!DOCTYPE html> <html> <head><title>My JavaScript Example</title> <script> function MsgBox(textstring) { alert(textstring); } </script> 9Extensible- CSE 330 – Creative Networking Programming Platform and Rapid Prototyping 9 JavaScript Example (part 2) <body> <form> <input type = "text" name ="text1" > <input type = "submit" value ="Show Me" onClick="MsgBox(form.text1.value)"> </form> </body> </html> 10Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 10 JavaScript Example <!DOCTYPE html> <html lang="en"> <head><title>My JavaScript Example</title> <script> function MsgBox(textstring) { alert(textstring); } </script> </head> <body> <form> <input type = "text" name ="text1" > <input type = "submit" value ="Show Me" onClick="MsgBox(form.text1.value)"> </form> </body> </html> 11Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 11 JavaScript Event Listeners • We can control events in a more granular way using Event Listeners • Event Listeners allow for custom behavior for every element document.getElementById("hello").addEventListener("click",MsgBox, false); 12Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 12 JavaScript Additional Information • The CSE 330 Wiki provides a great intro into JavaScript along with some excellent links to more comprehensive tutorials • Additional JS tutorials – http://www.quirksmode.org/js/contents.html – https://docs.webplatform.org/wiki/Meta:javascript/t utorials 13Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 13 JavaScript Debugging • JSHint is a great resources to help debug your code – http://www.jshint.com/ • Tools for browsers also exist – Firefox • Firebug extension – Chrome and Safari • Webkit Inspector comes bundled with the browser 14Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 14 JavaScript Examples http://research.engineering.wustl.edu/~todd/cse330/demo/lecture5/ 15Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 15 AJAX • Stands for Asynchronous JavaScript and XML” • Development technique for creating interactive web applications • Not a new Technology but more of a Pattern 16Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 16 Motivation for AJAX • Web pages always RELOAD and never get UPDATED • Users wait for the entire page to load even if a single piece of data is needed • Single request/response restrictions 17Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 17 Components – HTML (or XHTML) and CSS • Presenting information – Document Object Model • Dynamic display and interaction with the information – XMLHttpRequest object • Retrieving data ASYNCHRONOUSLY from the web server. – JavaScript • Binding everything together 18Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 18 The DOM • Document Object Model (DOM): platform- and language-independent way to represent XML – Adopts a tree-based representation – W3C standard, supported by modern browsers • JavaScript uses DOM to manipulate content – To process user events – To process server responses (via XMLHttpRequest) 19Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 19 The DOM • Unlike other programming languages, JavaScript understands HTML and can directly access it • JavaScript uses the HTML Document Object Model to manipulate HTML • The DOM is a hierarchy of HTML things • Use the DOM to build an address to refer to HTML elements in a web page • Levels of the DOM are dot-separated in the syntax 20Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 20 DOM 21Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 21 Accessing the DOM Example https://research.engineering.wustl.edu/~todd/cse330/demo/lecture5/ 22Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 22 Web Application and AJAX 23Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 23 Request Processing 24Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 24 Asynchronous processing - XMLHttpRequest • Allows to execute an HTTP request in background • Callbacks kick back into JavaScript Code • Supported in all standard browsers 25Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 25 Using XMLHttpRequest • First you must create the XMLHttpRequest Object in JavaScript • Example – const xmlHttp = new XMLHttpRequest(); • Older browsers might require different syntax – For example (Internet Explorer versions <9) • const xmlHttp = new ActiveXObject("Microsoft.XMLHTTP"); – We will not worry about JS compatibility with IE < 9 in this course 26Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 26 Using XMLHttpRequest • Transferring data to Server – Open() to initialize connection to Server – Send() to send the actual Data • Example (POST) – xmlHttp.open("POST", "/query.cgi”,true); – xmlHttp.send("name=Bob&[email protected]"); • Example (GET) – xmlHttp.open("GET","hello.txt",true); – xmlHttp.send(null); 27Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 27 What happens after sending data? • XMLHttpRequest contacts the server and retrieves the data – Can take indeterminate amount of time • Create an Event Listener to determine when the object has finished loading with the data we are interested in – Specifically listen for the load event to occur 28Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 28 Events of interest xmlHttp.addEventListener("load”,myCallbackFunction,false); 29Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 29 Using XMLHttpRequest • Parse and display data – responseXML • DOM-structured object – responseText • One complete string • Examples – const nameNode = requester.responseXML.getElementsByTagName("name")[0]; – const nameTextNode = nameNode.childNodes[0]; • From an event function myCallback(event) { alert(“The response “ + event.target.responseText); } 30Extensible- CSE 330 – CreativeNetworking Programming Platform and Rapid Prototyping 30 AJAX Example <script> const xmlHttp = new XMLHttpRequest(); xmlHttp.open("GET","hello.txt",true); xmlHttp.addEventListener("load”,myCallback,false); xmlHttp.send(null); function myCallback(event) { alert(“The response “ + event.target.responseText);
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages27 Page
-
File Size-