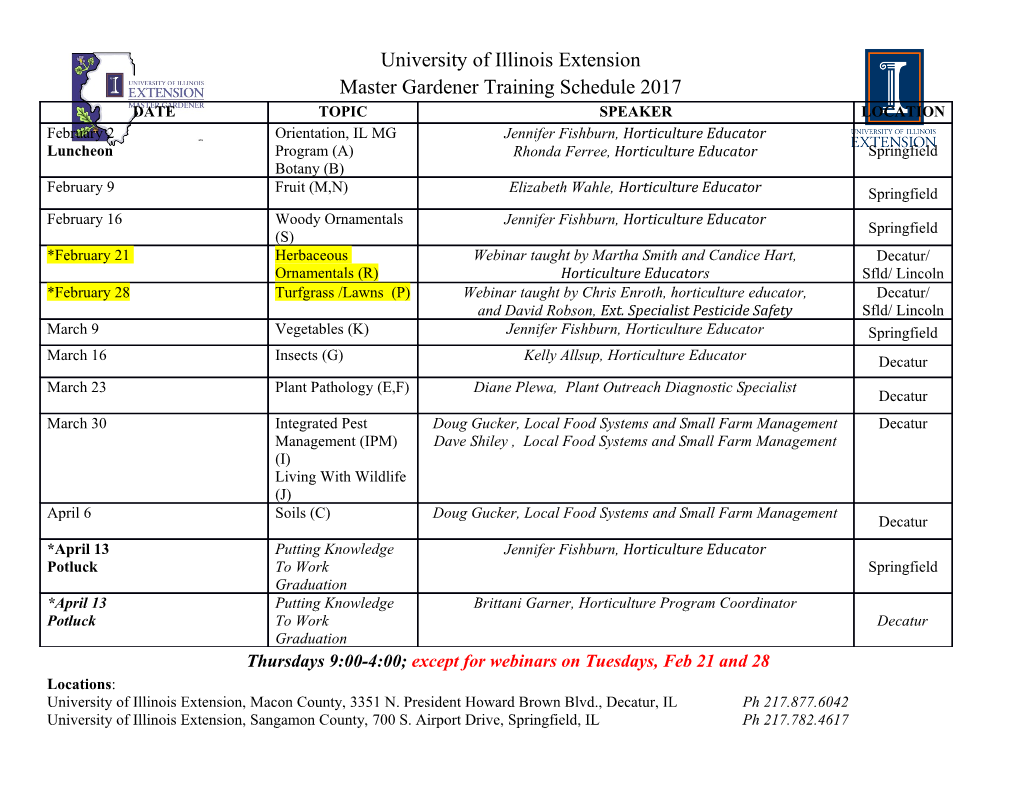
CS51: Abstraction and Design in Computation Stuart M. Shieber Now playing: “Living With Substitutions” Stephan Panev Living With Substitutions John A. Paulson School of Engineering and Applied Sciences 1 Harvard University 7: The substitution model 2 Big ideas The informal semantics of OCaml can be made more rigorous through the substitution model of evaluation. The substitution model can be made explicit in a metacircular interpreter. Rigorous semantics is easier to provide for smaller languages The lambda calculus, the core of OCaml, is very small indeed yet computationally universal 3 The substitution model: Arithmetic and let...in binding 4 From OCaml expressions lecture 2... expr ::= value numbers, strings, bools, etc. | id variables (x, foo, etc.) | expr1 binop expr2 binary operators (3 + 4) | expr1 expr2 function application (foo 42) | let id = expr1 in expr2 local naming | if expr1 then expr2 else expr3 conditional | fun id -> expr anonymous functions 5 OCaml expressions expr ::= value numbers, strings, bools, etc. | id variables (x, foo, etc.) | expr1 binop expr2 binary operators (3 + 4) | expr1 expr2 function application (foo 42) | let id = expr1 in expr2 local naming | if expr1 then expr2 else expr3 conditional | fun id -> expr anonymous functions 6 Evaluation: values 3 ⟹ 3 To evaluate m: Result is m 4 ⟹ 4 5 ⟹ 5 7 Evaluation: binary operators 3 + (4 * 5) To evaluate P + Q: 3 ⟹ 3 Evaluate P to m Evaluate Q to n Result is m+n 8 Evaluation: binary operators 3 + (4 * 5) To evaluate P + Q: 3 ⟹ 3 Evaluate P to m 4 * 5 4 ⟹ 4 Evaluate Q to n 5 ⟹ 5 Result is m+n 9 Evaluation: binary operators 3 + (4 * 5) To evaluate P + Q: 3 ⟹ 3 Evaluate P to m 4 * 5 4 ⟹ 4 Evaluate Q to n 5 ⟹ 5 Result is m+n ⟹ 20 10 Evaluation: binary operators 3 + (4 * 5) To evaluate P + Q: 3 ⟹ 3 Evaluate P to m 4 * 5 4 ⟹ 4 Evaluate Q to n 5 ⟹ 5 Result is m+n ⟹ 20 ⟹ 23 11 Evaluation: let...in expressions let x = 3 in x*x To evaluate let x = Q in P: Evaluate Q to Q' Evaluate P[x ↦ Q'] to R Result is R 12 Evaluation: let...in expressions let x = 3 in x*x To evaluate let x = Q in P: Evaluate Q to Q' Evaluate P[x ↦ Q'] to R Result is R P[x ↦ Q] means substitute Q for (appropriate) x in P 12 Evaluation: let...in expressions let x = 3 in x*x To evaluate 3 ⟹ 3 let x = Q in P: Evaluate Q to Q' Evaluate P[x ↦ Q'] to R Result is R P[x ↦ Q] means substitute Q for (appropriate) x in P 13 Evaluation: let...in expressions let x = 3 in x*x To evaluate 3 ⟹ 3 let x = Q in P: 3 * 3 3 ⟹ 3 Evaluate Q to Q' 3 ⟹ 3 Evaluate P[x ↦ Q'] to R ⟹ 9 Result is R P[x ↦ Q] means substitute Q for (appropriate) x in P 14 Evaluation: let...in expressions let x = 3 in x*x To evaluate 3 ⟹ 3 let x = Q in P: 3 * 3 3 ⟹ 3 Evaluate Q to Q' 3 ⟹ 3 Evaluate P[x ↦ Q'] to R ⟹ 9 Result is R ⟹ 9 P[x ↦ Q] means substitute Q for (appropriate) x in P 15 The indiscernibility of identicals That A is the same as B signifies that the one can be substituted for the other, salva veritate, in any proposition whatever. – Gottfried Wilhelm Leibniz Gottfried Wilhelm Leibniz 16 Implementing arithmetic evaluation type expr = | Int of int | Var of varspec | Binop of binop * expr * expr | Let of varspec * expr * expr and binop = Plus | Times and varspec = string ;; 17 find this code in: eval_arith.ml Implementing arithmetic evaluation let x = 3 in Let("x", let y = 5 in Int 3, x * y Let ("y", Int 5, Binop(Times, Var "x", Var "y"))) Concrete syntax Abstract syntax (as represented in OCaml) (as represented in OCaml) 18 Implementing arithmetic evaluation let x = 3 in Let let y = 5 in "x" Int Let x * y 3 "y" Int Binop 5 Times Var Var "x" "y" Concrete syntax Abstract syntax (as represented in OCaml) (as represented in OCaml) 19 Implementing arithmetic evaluation type expr = | Int of int | Var of varspec | Binop of binop * expr * expr | Let of varspec * expr * expr and binop = Plus | Times and varspec = string ;; 20 find this code in: eval_arith.ml Implementing arithmetic evaluation type expr = | Int of int This represents the | Var of varspec occurrence of a variable, a | Binop of binop * expr *free expr occurrence unless | Let of varspec * expr *bound expr by a let binder. and binop = Plus | Times and varspec = string ;; 20 find this code in: eval_arith.ml Implementing arithmetic evaluation type expr = | Int of int | Var of varspec | Binop of binop * expr * expr | Let of varspec * expr * expr and binop = Plus | Times and varspecThe Let = stringbinds all free;; occurrences of its variable in its scope. 20 find this code in: eval_arith.ml Implementing arithmetic evaluation let rec eval (e : expr) : expr = match e with | Int i -> ??? 21 Implementing arithmetic evaluation let rec eval (e : expr) : expr = match e with | Int _ -> e | Binop (op, e1, e2) -> ??? 22 Implementing arithmetic evaluation let rec eval (e : expr) : expr = match e with | Int _ -> e Integers are values; they self-evaluate | Binop (op, e1, e2) -> ??? 22 Implementing arithmetic evaluation let rec eval (e : expr) : expr = match e with | Int _ -> e | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) 23 Implementing arithmetic evaluation let rec eval (e : expr) : expr = match e with | Int _ -> e | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) We'll assume a function that performs the arithmetic dependent on the operation... 23 Implementing arithmetic evaluation let rec eval (e : expr) : expr = match e with | Int _ -> e ...and the evaluated arguments | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) We'll assume a function that performs the arithmetic dependent on the operation... 23 Implementing arithmetic evaluation let binopeval (op : binop) (v1 : expr) (v2 : expr) : expr = match op, v1, v2 with | Plus, Int x1, Int x2 -> Int (x1 + x2) | Plus, _, _ -> raise (IllFormed "can't add non-ints") | Times, Int x1, Int x2 -> Int (x1 * x2) | Times, _, _ -> raise (IllFormed "can't mult non-ints") ;; 24 Implementing arithmetic evaluation let binopeval (op : binop) (v1 : expr) (v2 : expr) : expr = match op, v1, v2 with | Plus, Int x1, Int x2 -> Int (x1 + x2) | Plus, _, _ -> raise (IllFormed "can't add non-ints") | Times, Int x1, Int x2 -> Int (x1 * x2) | Times, _, _ -> raise (IllFormed "can't mult non-ints") ;; 24 Implementing arithmetic evaluation let rec eval (e : expr) : expr = match e with | Int _ -> e | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) | Let (x, def, body) -> ??? 25 Implementing arithmeticTo evaluate evaluation let x = Q in P: let rec eval (e : expr) Evaluate: expr = Q to Q' match e with Evaluate P[x ↦ Q'] to R | Int _ -> e Result is R | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) | Let (x, def, body) -> ??? 25 Implementing arithmeticTo evaluate evaluation let x = Q in P: let rec eval (e : expr) Evaluate: expr = Q to Q' match e with Evaluate P[x ↦ Q'] to R | Int _ -> e Result is R | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) | Let (x, def, body) -> eval (subst body x (eval def)) 26 Implementing arithmeticTo evaluate evaluation let x = Q in P: let rec eval (e : expr) Evaluate: expr = Q to Q' match e with Evaluate P[x ↦ Q'] to R | Int _ -> e Result is R | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) | Let (x, def, body) -> eval (subst body x (eval def)) The subst p x q function implements P[x ↦ Q] 26 Implementing arithmetic evaluation let rec eval (e : expr) : expr = match e with | Int _ -> e | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) | Let (x, def, body) -> eval (subst body x (eval def)) | Var x -> ??? 27 find this code in: eval_arith.ml Implementing arithmetic evaluation let rec eval (e : expr) : expr = match e with | Int _ -> e | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) | Let (x, def, body) -> eval (subst body x (eval def)) | Var x -> raise (UnboundVariable x) ;; 28 find this code in: eval_arith.ml Implementing arithmetic evaluation let rec eval (e : expr) : expr = match e with | Int _ -> e | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) | Let (x, def, body) -> eval (subst body x (eval def)) | Var x -> raise (UnboundVariable x) ;; We should never come across an unsubstituted variable. 28 find this code in: eval_arith.ml Implementing arithmetic evaluation let rec eval (e : expr) : expr = match e with | Int _ -> e | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) | ... let binopeval op v1 v2 = match op, v1, v2 with | Plus, Int x1, Int x2 -> Int (x1 + x2) | ... 29 find this code in: eval_arith.ml ImplementingAn interpreter arithmetic for OCaml evaluation (or a subset) that... let rec eval (e : expr) : expr = match e with | Int _ -> e | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) | ... let binopeval op v1 v2 = match op, v1, v2 with | Plus, Int x1, Int x2 -> Int (x1 + x2) | ... 29 find this code in: eval_arith.ml ImplementingAn interpreter arithmetic for OCaml evaluation (or a subset) that... let rec eval (e : expr) : expr = match e with | Int _ -> e | Binop (op, e1, e2) -> binopeval op (eval e1) (eval e2) | ... let binopeval op v1 v2...uses = OCaml constructs in the metalanguage..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages112 Page
-
File Size-