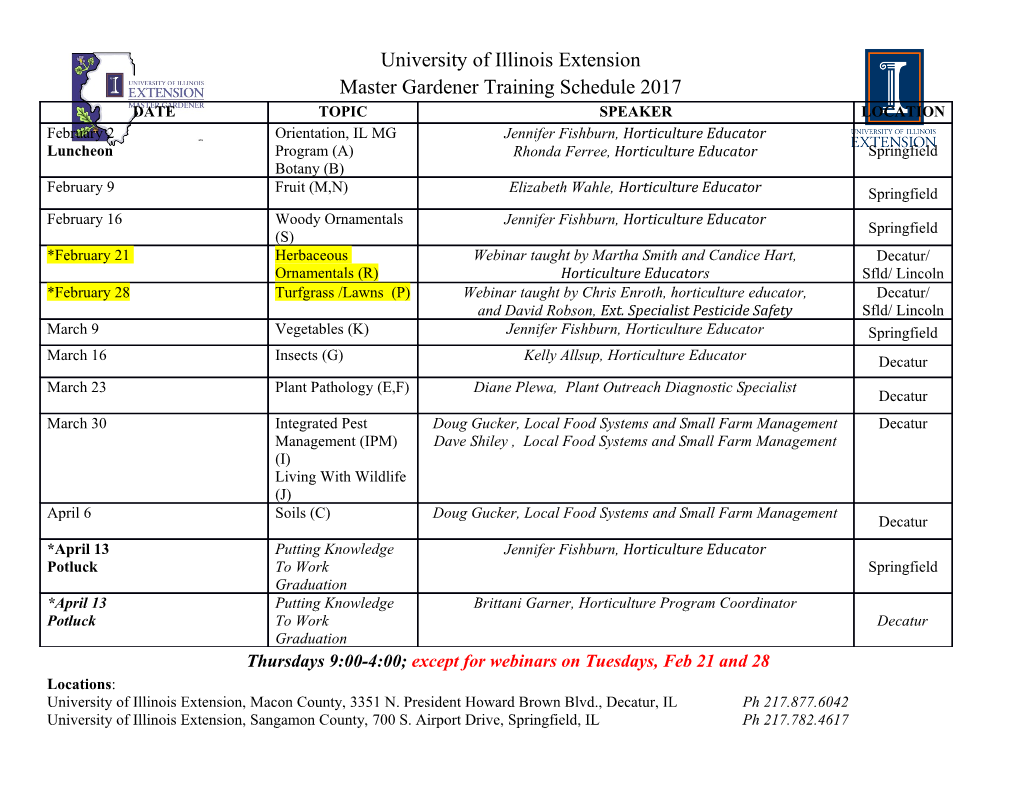
Linux Kernel Module Programming Amir H. Payberah [email protected] Amirkabir University of Technology (Tehran Polytechnic) Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 1 / 46 Introduction Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 2 / 46 I They extend the functionality of the kernel without the need to reboot the system. I One type of module is the device driver. What Is A Kernel Module? I Pieces of code that can be loaded and unloaded into the kernel upon demand. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 3 / 46 I One type of module is the device driver. What Is A Kernel Module? I Pieces of code that can be loaded and unloaded into the kernel upon demand. I They extend the functionality of the kernel without the need to reboot the system. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 3 / 46 What Is A Kernel Module? I Pieces of code that can be loaded and unloaded into the kernel upon demand. I They extend the functionality of the kernel without the need to reboot the system. I One type of module is the device driver. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 3 / 46 Already Loaded Modules I You can see already loaded modules into kernel: lsmod I It gets its information by reading the file /proc/modules. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 4 / 46 I modprobe looks file /lib/modules/<version>/modules.dep. • Contains module dependencies • Created by depmod -a How Do Modules Get Into The Kernel? (1/2) I When the kernel needs a feature that is not resident in the kernel: I The kernel module daemon kmod execs modprobe to load the mod- ule in. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 5 / 46 How Do Modules Get Into The Kernel? (1/2) I When the kernel needs a feature that is not resident in the kernel: I The kernel module daemon kmod execs modprobe to load the mod- ule in. I modprobe looks file /lib/modules/<version>/modules.dep. • Contains module dependencies • Created by depmod -a Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 5 / 46 I insmod is dumb about the location of modules, whereas modprobe is aware of the default location of modules. I modprobe also knows how to figure out the dependencies and load the modules in the right order. How Do Modules Get Into The Kernel? (2/2) I modprobe uses insmod to load any prerequisite modules and the requested module into the kernel. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 6 / 46 I modprobe also knows how to figure out the dependencies and load the modules in the right order. How Do Modules Get Into The Kernel? (2/2) I modprobe uses insmod to load any prerequisite modules and the requested module into the kernel. I insmod is dumb about the location of modules, whereas modprobe is aware of the default location of modules. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 6 / 46 How Do Modules Get Into The Kernel? (2/2) I modprobe uses insmod to load any prerequisite modules and the requested module into the kernel. I insmod is dumb about the location of modules, whereas modprobe is aware of the default location of modules. I modprobe also knows how to figure out the dependencies and load the modules in the right order. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 6 / 46 I modprobe just takes the name, without any extension, and figures out all it needs to know. I For example: insmod /lib/modules/2.6.11/kernel/fs/fat/fat.ko insmod /lib/modules/2.6.11/kernel/fs/msdos/msdos.ko modprobe msdos insmod vs. modprobe I insmod requires you to pass it the full pathname and to insert the modules in the right order. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 7 / 46 I For example: insmod /lib/modules/2.6.11/kernel/fs/fat/fat.ko insmod /lib/modules/2.6.11/kernel/fs/msdos/msdos.ko modprobe msdos insmod vs. modprobe I insmod requires you to pass it the full pathname and to insert the modules in the right order. I modprobe just takes the name, without any extension, and figures out all it needs to know. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 7 / 46 insmod vs. modprobe I insmod requires you to pass it the full pathname and to insert the modules in the right order. I modprobe just takes the name, without any extension, and figures out all it needs to know. I For example: insmod /lib/modules/2.6.11/kernel/fs/fat/fat.ko insmod /lib/modules/2.6.11/kernel/fs/msdos/msdos.ko modprobe msdos Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 7 / 46 Hello World Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 8 / 46 Hello World #include <linux/module.h> /* Needed by all modules */ #include <linux/kernel.h> /* Needed for KERN_INFO */ int init_module(void){ printk(KERN_INFO "Hello world!\n"); // A non 0 return means init_module failed; module can't be loaded. return0; } void cleanup_module(void){ printk(KERN_INFO "Goodbye world!.\n"); } Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 9 / 46 I init module(): it is called when the module is insmoded into the kernel. • It registers a handler for something with the kernel. • or it replaces one of the kernel functions with its own code. I cleanup module(): it is called just before it is rmmoded. • It undos whatever init module() did, so the module can be unloaded safely Hello World Structure (1/2) I Two main functions of kernel modules: initialization and cleanup. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 10 / 46 I cleanup module(): it is called just before it is rmmoded. • It undos whatever init module() did, so the module can be unloaded safely Hello World Structure (1/2) I Two main functions of kernel modules: initialization and cleanup. I init module(): it is called when the module is insmoded into the kernel. • It registers a handler for something with the kernel. • or it replaces one of the kernel functions with its own code. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 10 / 46 Hello World Structure (1/2) I Two main functions of kernel modules: initialization and cleanup. I init module(): it is called when the module is insmoded into the kernel. • It registers a handler for something with the kernel. • or it replaces one of the kernel functions with its own code. I cleanup module(): it is called just before it is rmmoded. • It undos whatever init module() did, so the module can be unloaded safely Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 10 / 46 Hello World Structure (2/2) I Every kernel module needs linux/module.h. I It needs linux/kernel.h only for the macro expansion for the printk() log level, e.g., KERN INFO. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 11 / 46 I Each printk() statement comes with a priority. • Eight priority levels I Prints out the message in the kernel ring buffer. • Use dmesg to print the kernel ring buffer. I The message is appended to /var/log/messages, if syslogd is running. Introducing printk() I Logging mechanism for the kernel. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 12 / 46 I Prints out the message in the kernel ring buffer. • Use dmesg to print the kernel ring buffer. I The message is appended to /var/log/messages, if syslogd is running. Introducing printk() I Logging mechanism for the kernel. I Each printk() statement comes with a priority. • Eight priority levels Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 12 / 46 I The message is appended to /var/log/messages, if syslogd is running. Introducing printk() I Logging mechanism for the kernel. I Each printk() statement comes with a priority. • Eight priority levels I Prints out the message in the kernel ring buffer. • Use dmesg to print the kernel ring buffer. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 12 / 46 Introducing printk() I Logging mechanism for the kernel. I Each printk() statement comes with a priority. • Eight priority levels I Prints out the message in the kernel ring buffer. • Use dmesg to print the kernel ring buffer. I The message is appended to /var/log/messages, if syslogd is running. Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 12 / 46 I To compile and insert the module in the kernel: # to compile the module > make # to insert the module in the kernel > sudo insmod hello.ko # to see the module message > dmesg # to remove the module from the kernel > sudo insmod hello.ko Compiling Kernel Modules I A simple Makefile for compiling a module named hello.c. obj-m += hello.o all: make -C /lib/modules/$(shell uname -r)/buildM=$(PWD) modules clean: make -C /lib/modules/$(shell uname -r)/buildM=$(PWD) clean Amir H. Payberah (Tehran Polytechnic) Linux Module Programming 1393/9/17 13 / 46 Compiling Kernel Modules I A simple Makefile for compiling a module named hello.c. obj-m += hello.o all: make -C /lib/modules/$(shell uname -r)/buildM=$(PWD) modules clean: make -C /lib/modules/$(shell uname -r)/buildM=$(PWD) clean I To compile and insert the module in the kernel: # to compile the module > make # to insert the module in the kernel > sudo insmod hello.ko # to see the module message > dmesg # to remove the module from the kernel > sudo insmod hello.ko Amir H.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages66 Page
-
File Size-