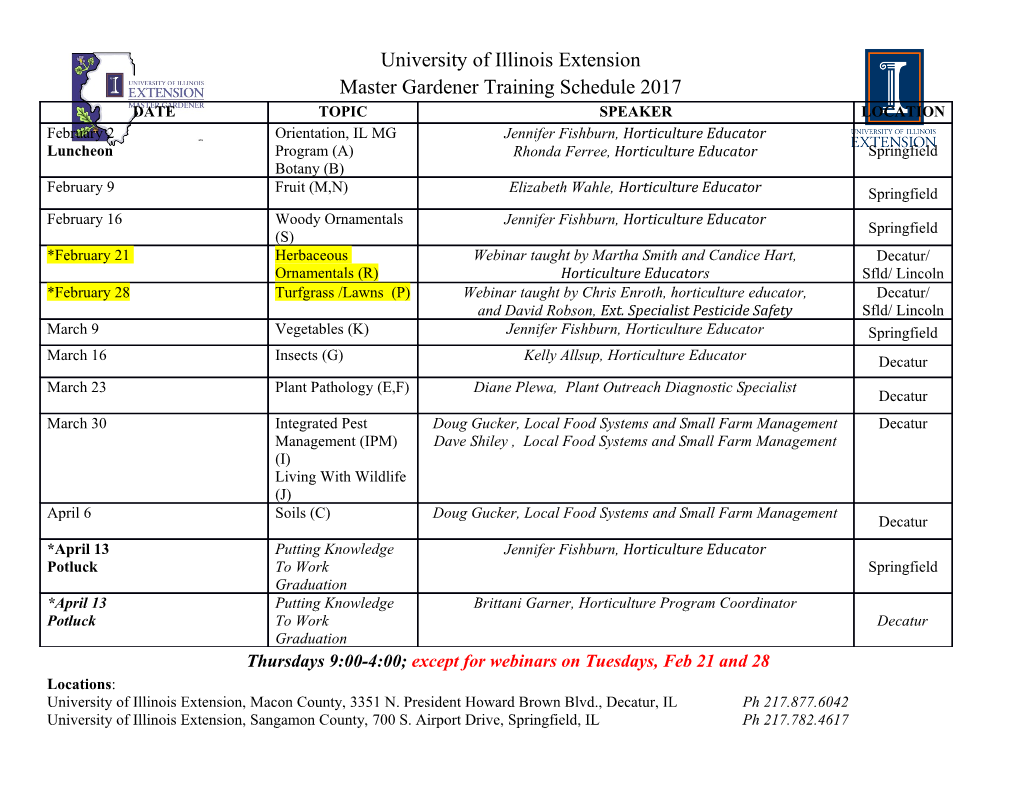
8 Priority Queues 8 Priority Queues A Priority Queue S is a dynamic set data structure that supports the following operations: ñ S: build(x1; : : : ; xn): Creates a data-structure that contains An addressable Priority Queue also supports: just the elements x1; : : : ; xn. ñ handle S: insert(x): Adds element x to the data-structure, ñ S: insert(x): Adds element x to the data-structure. and returns a handle to the object for future reference. ñ element S: minimum(): Returns an element x S with 2 ñ S: delete(h): Deletes element specified through handle h. minimum key-value key[x]. ñ S: decrease-key(h; k): Decreases the key of the element ñ element delete-min : Deletes the element with S: () specified by handle h to k. Assumes that the key is at least minimum key-value from and returns it. S k before the operation. ñ boolean S: is-empty(): Returns true if the data-structure is empty and false otherwise. Sometimes we also have ñ S: merge(S ): S : S S ; S : . 0 = [ 0 0 = 8 Priority Queues © Harald Räcke 303 © Harald Räcke 304 Dijkstra’s Shortest Path Algorithm Prim’s Minimum Spanning Tree Algorithm Algorithm 19 Prim-MST(G (V , E, d), s V) Algorithm 18 Shortest-Path(G (V , E, d), s V) = ∈ = ∈ 1: Input: weighted graph G (V , E, d); start vertex s; 1: Input: weighted graph G (V , E, d); start vertex s; = = 2: Output: pred-fields encode MST; 2: Output: key-field of every node contains distance from s; 3: S.build(); // build empty priority queue 3: S.build(); // build empty priority queue 4: for all v V s do 4: for all v V s do ∈ \{ } ∈ \{ } 5: v. key ; 5: v. key ; ← ∞ ← ∞ 6: hv S.insert(v); 6: hv S.insert(v); ← ← 7: s. key 0; S.insert(s); 7: s. key 0; S.insert(s); ← ← 8: while S.is-empty() false do 8: while S.is-empty() false do = = 9: v S.delete-min(); 9: v S.delete-min(); ← ← 10: for all x V s.t. v, x E do 10: for all x V s.t. (v, x) E do ∈ { } ∈ ∈ ∈ 11: if x. key > d(v, x) then 11: if x. key > v. key d(v, x) then + 12: S.decrease-key(hx,d(v, x)); 12: S.decrease-key(hx,v. key d(v, x)); + 13: x. key d(v, x); 13: x. key v. key d(v, x); ← ← + 14: x. pred v; ← 8 Priority Queues 8 Priority Queues © Harald Räcke 305 © Harald Räcke 306 Analysis of Dijkstra and Prim 8 Priority Queues Both algorithms require: Binary Binomial Fibonacci Operation BST * ñ 1 build() operation Heap Heap Heap build n log log n ñ V insert() operations n n n n j j minimum 1 log n log n 1 ñ V delete-min() operations j j is-empty 1 1 1 1 ñ V is-empty() operations insert log n log n log n 1 j j delete log n** log n log n log n ñ E decrease-key() operations j j delete-min log n log n log n log n decrease-key log n log n log n 1 How good a running time can we obtain? merge n n log n log n 1 Note that most applications use build() only to create an empty * Fibonacciheap which heapsthen only give costs an time** 1The. standard version of binary heaps is not address- amortized guarantee. able. Hence, it does not support a delete. 8 Priority Queues © Harald Räcke 307 8 Priority Queues 8.1 Binary Heaps ñ Nearly complete binary tree; only the last level is not full, and this one is filled from left to right. ñ Heap property: A node’s key is not larger than the key of one of its children. Using Binary Heaps, Prim and Dijkstra run in time 7 (( V E ) log V ). O j j + j j j j 9 15 Using Fibonacci Heaps, Prim and Dijkstra run in time ( V log V E ). O j j j j + j j 17 11 31 19 25 43 13 12 80 8 Priority Queues 8.1 Binary Heaps © Harald Räcke 309 © Harald Räcke 310 Binary Heaps 8.1 Binary Heaps Maintain a pointer to the last element x. ñ We can compute the predecessor of x (last element when x is deleted) in time (log n). O go up until the last edge used was a right edge. go left; go right until you reach a leaf Operations: ñ minimum(): return the root-element. Time (1). if you hit the root on the way up, go to the rightmost O element ñ is-empty(): check whether root-pointer is null. Time (1). O 7 9 15 17 11 31 19 25 43 13 12 80 x 8.1 Binary Heaps 8.1 Binary Heaps © Harald Räcke 311 © Harald Räcke 312 8.1 Binary Heaps Insert Maintain a pointer to the last element x. ñ We can compute the successor of x 1. Insert element at successor of x. (last element when an element is inserted) in time (log n). 2. Exchange with parent until heap property is fulfilled. O go up until the last edge used was a left edge. 7 go right; go left until you reach a null-pointer. if you hit the root on the way up, go to the leftmost 9 14 element; insert a new element as a left child; 7 17 11 15 19 9 15 25 43 13 12 80 311x x 17 11 31 19 Note that an exchange can either be done by moving the data or by changing pointers. The latter method leads to an addressable 25 43 13 12 80 x priority queue. 8.1 Binary Heaps 8.1 Binary Heaps © Harald Räcke 313 © Harald Räcke 314 Delete Binary Heaps 1. Exchange the element to be deleted with the element e pointed to by x. 2. Restore the heap-property for the element e. Operations: 7 ñ minimum(): return the root-element. Time (1). O ñ is-empty(): check whether root-pointer is null. Time (1). O e 13 9 ñ insert(k): insert at x and bubble up. Time (log n). O ñ delete(h): swap with x and bubble up or sift-down. Time 18 1716 12 19 (log n). O 25 43 27 201 x 13 x At its new position e may either travel up or down in the tree (but not both directions). 8.1 Binary Heaps 8.1 Binary Heaps © Harald Räcke 315 © Harald Räcke 316 Build Heap Binary Heaps We can build a heap in linear time: 31 Operations: ñ minimum(): Return the root-element. Time (1). 30 2 O ñ is-empty(): Check whether root-pointer is null. Time (1). O ñ insert(k): Insert at x and bubble up. Time (log n). 13 9 5 3 O ñ delete(h): Swap with x and bubble up or sift-down. Time 15 14 11 10 7 6 4 17 (log n). O ñ build(x1; : : : ; xn): Insert elements arbitrarily; then do 16 24 28 23 22 12 27 21 20 26 19 8 25 18 29 35 sift-down operations starting with the lowest layer in the tree. Time (n). O 2` (h `) (2h) (n) · − = O = O levelsX ` 8.1 Binary Heaps 8.1 Binary Heaps © Harald Räcke 317 © Harald Räcke 318 Binary Heaps 8.2 Binomial Heaps The standard implementation of binary heaps is via arrays. Let Binary Binomial Fibonacci A[0; : : : ; n 1] be an array Operation BST − Heap Heap Heap* i 1 ñ The parent of i-th element is at position − . b 2 c build n n log n n log n n ñ The left child of i-th element is at position 2i 1. minimum 1 log n log n 1 + is-empty 1 1 1 1 ñ The right child of i-th element is at position 2i 2. + insert log n log n log n 1 Finding the successor of x is much easier than in the description delete log n** log n log n log n on the previous slide. Simply increase or decrease x. delete-min log n log n log n log n decrease-key log n log n log n 1 The resulting binary heap is not addressable. The elements merge n n log n log n 1 don’t maintain their positions and therefore there are no stable handles. 8.1 Binary Heaps 8.2 Binomial Heaps © Harald Räcke 319 © Harald Räcke 320 Binomial Trees Binomial Trees B0 B1 B2 B3 B4 Properties of Binomial Trees k ñ Bk has 2 nodes. ñ Bk has height k. ñ The root of has degree . Bt Bk k ñ has k nodes on level . Bk ` ` ñ Deleting the root of Bk gives trees B0;B1;:::;Bk 1. − Bt 1 − Bt 1 − 8.2 Binomial Heaps 8.2 Binomial Heaps © Harald Räcke 321 © Harald Räcke 322 Binomial Trees Binomial Trees B4 B3 B0 B2 B1 B1 B2 B0 B3 B4 Deleting the root of B5 leaves sub-trees B4, B3, B2, B1, and B0. Deleting the leaf furthest from the root (in B5) leaves a path that connects the roots of sub-trees B4, B3, B2, B1, and B0. 8.2 Binomial Heaps 8.2 Binomial Heaps © Harald Räcke 323 © Harald Räcke 324 Binomial Trees Binomial Trees 0000 Bk Bk 1 − 1000 0100 0010 0001 Bk 1 − k 1 `−1 − 1100 1010 1001 0110 0101 0011 k 1 −` 1110 1101 1011 0111 1111 The binomial tree Bk is a sub-graph of the hypercube Hk.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages20 Page
-
File Size-