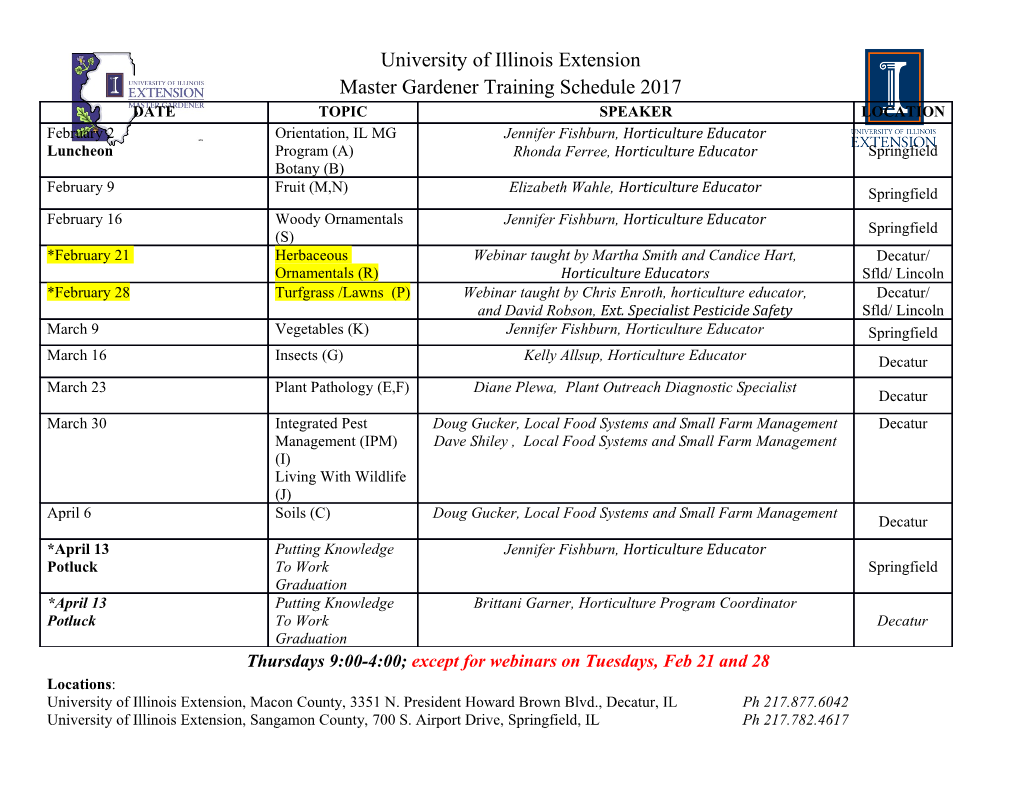
Category Theory for Programmers Bartosz Milewski Category Theory for Programmers Bartosz Milewski Version 0.1, September 2017 This work is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License (cc by-sa 4.0). Converted to LaTeX from a series of blog posts by Bartosz Milewski. PDF compiled by Igal Tabachnik. Contents Preface xiii I Part One 1 1 Category: The Essence of Composition 2 1.1 Arrows as Functions ................... 2 1.2 Properties of Composition ................ 5 1.3 Composition is the Essence of Programming ...... 8 1.4 Challenges ......................... 10 2 Types and Functions 11 2.1 Who Needs Types? .................... 11 2.2 Types Are About Composability ............. 13 2.3 What Are Types? ..................... 14 2.4 Why Do We Need a Mathematical Model? ....... 17 2.5 Pure and Dirty Functions ................. 20 2.6 Examples of Types ..................... 21 2.7 Challenges ......................... 25 iii 3 Categories Great and Small 27 3.1 No Objects ......................... 27 3.2 Simple Graphs ....................... 28 3.3 Orders ........................... 28 3.4 Monoid as Set ....................... 29 3.5 Monoid as Category .................... 34 3.6 Challenges ......................... 37 4 Kleisli Categories 39 4.1 The Writer Category ................... 44 4.2 Writer in Haskell ..................... 48 4.3 Kleisli Categories ..................... 50 4.4 Challenge ......................... 51 5 Products and Coproducts 53 5.1 Initial Object ........................ 54 5.2 Terminal Object ...................... 56 5.3 Duality ........................... 57 5.4 Isomorphisms ....................... 58 5.5 Products .......................... 60 5.6 Coproduct ......................... 66 5.7 Asymmetry ........................ 70 5.8 Challenges ......................... 73 5.9 Bibliography ........................ 74 6 Simple Algebraic Data Types 75 6.1 Product Types ....................... 76 6.2 Records ........................... 80 6.3 Sum Types ......................... 81 6.4 Algebra of Types ..................... 86 iv 6.5 Challenges ......................... 90 7 Functors 92 7.1 Functors in Programming ................. 96 7.1.1 The Maybe Functor ................ 96 7.1.2 Equational Reasoning .............. 98 7.1.3 Optional ...................... 101 7.1.4 Typeclasses .................... 103 7.1.5 Functor in C++ .................. 105 7.1.6 The List Functor ................. 106 7.1.7 The Reader Functor ................ 108 7.2 Functors as Containers .................. 110 7.3 Functor Composition ................... 113 7.4 Challenges ......................... 116 8 Functoriality 117 8.1 Bifunctors ......................... 117 8.2 Product and Coproduct Bifunctors ............ 121 8.3 Functorial Algebraic Data Types ............. 122 8.4 Functors in C++ ...................... 126 8.5 The Writer Functor .................... 129 8.6 Covariant and Contravariant Functors ......... 131 8.7 Profunctors ......................... 134 8.8 Challenges ......................... 136 9 Function Types 138 9.1 Universal Construction .................. 139 9.2 Currying .......................... 144 9.3 Exponentials ........................ 148 9.4 Cartesian Closed Categories ............... 149 v 9.5 Exponentials and Algebraic Data Types ......... 150 9.5.1 Zeroth Power ................... 151 9.5.2 Powers of One .................. 152 9.5.3 First Power .................... 152 9.5.4 Exponentials of Sums .............. 152 9.5.5 Exponentials of Exponentials .......... 153 9.5.6 Exponentials over Products ........... 153 9.6 Curry-Howard Isomorphism ............... 154 9.7 Bibliography ........................ 157 10 Natural Transformations 158 10.1 Polymorphic Functions .................. 162 10.2 Beyond Naturality ..................... 168 10.3 Functor Category ..................... 170 10.4 2-Categories ........................ 175 10.5 Conclusion ......................... 180 10.6 Challenges ......................... 181 II Part Two 183 11 Declarative Programming 184 12 Limits and Colimits 192 12.1 Limit as a Natural Isomorphism ............. 198 12.2 Examples of Limits .................... 203 12.3 Colimits .......................... 211 12.4 Continuity ......................... 213 12.5 Challenges ......................... 215 vi 13 Free Monoids 216 13.1 Free Monoid in Haskell .................. 218 13.2 Free Monoid Universal Construction .......... 219 13.3 Challenges ......................... 224 14 Representable Functors 226 14.1 The Hom Functor ..................... 228 14.2 Representable Functors .................. 231 14.3 Challenges ......................... 236 14.4 Bibliography ........................ 237 15 The Yoneda Lemma 238 15.1 Yoneda in Haskell ..................... 246 15.2 Co-Yoneda ......................... 248 15.3 Challenges ......................... 249 15.4 Bibliography ........................ 249 16 Yoneda Embedding 250 16.1 The Embedding ...................... 253 16.2 Application to Haskell .................. 254 16.3 Preorder Example ..................... 255 16.4 Naturality ......................... 257 16.5 Challenges ......................... 259 III Part Three 260 17 It’s All About Morphisms 261 17.1 Functors .......................... 261 17.2 Commuting Diagrams ................... 262 vii 17.3 Natural Transformations ................. 263 17.4 Natural Isomorphisms ................... 265 17.5 Hom-Sets .......................... 265 17.6 Hom-Set Isomorphisms .................. 266 17.7 Asymmetry of Hom-Sets ................. 267 17.8 Challenges ......................... 268 18 Adjunctions 269 18.1 Adjunction and Unit/Counit Pair ............ 270 18.2 Adjunctions and Hom-Sets ................ 276 18.3 Product from Adjunction ................. 281 18.4 Exponential from Adjunction .............. 286 18.5 Challenges ......................... 287 19 Free/Forgetful Adjunctions 289 19.1 Some Intuitions ...................... 293 19.2 Challenges ......................... 296 20 Monads: Programmer’s Definition 298 20.1 The Kleisli Category .................... 300 20.2 Fish Anatomy ....................... 303 20.3 The do Notation ...................... 305 21 Monads and Effects 310 21.1 The Problem ........................ 310 21.2 The Solution ........................ 311 21.2.1 Partiality ..................... 312 21.2.2 Nondeterminism ................. 313 21.2.3 Read-Only State ................. 316 21.2.4 Write-Only State ................. 317 viii 21.2.5 State ........................ 318 21.2.6 Exceptions ..................... 319 21.2.7 Continuations ................... 320 21.2.8 Interactive Input ................. 322 21.2.9 Interactive Output ................ 325 21.3 Conclusion ......................... 326 22 Monads Categorically 327 22.1 Monoidal Categories ................... 333 22.2 Monoid in a Monoidal Category ............. 338 22.3 Monads as Monoids .................... 340 22.4 Monads from Adjunctions ................ 343 23 Comonads 347 23.1 Programming with Comonads .............. 348 23.2 The Product Comonad .................. 349 23.3 Dissecting the Composition ............... 350 23.4 The Stream Comonad ................... 353 23.5 Comonad Categorically .................. 355 23.6 The Store Comonad .................... 358 23.7 Challenges ......................... 361 24 F-Algebras 362 24.1 Recursion .......................... 366 24.2 Category of F-Algebras .................. 368 24.3 Natural Numbers ..................... 372 24.4 Catamorphisms ...................... 373 24.5 Folds ............................ 375 24.6 Coalgebras ......................... 377 24.7 Challenges ......................... 380 ix 25 Algebras for Monads 381 25.1 T-algebras ......................... 384 25.2 The Kleisli Category .................... 388 25.3 Coalgebras for Comonads ................ 391 25.4 Lenses ........................... 392 25.5 Challenges ......................... 394 26 Ends and Coends 395 26.1 Dinatural Transformations ................ 397 26.2 Ends ............................ 399 26.3 Ends as Equalizers ..................... 403 26.4 Natural Transformations as Ends ............ 404 26.5 Coends ........................... 406 26.6 Ninja Yoneda Lemma ................... 409 26.7 Profunctor Composition ................. 411 27 Kan Extensions 413 27.1 Right Kan Extension ................... 416 27.2 Kan Extension as Adjunction ............... 418 27.3 Left Kan Extension .................... 420 27.4 Kan Extensions as Ends .................. 424 27.5 Kan Extensions in Haskell ................ 427 27.6 Free Functor ........................ 430 28 Enriched Categories 432 28.1 Why Monoidal Category? ................ 433 28.2 Monoidal Category .................... 434 28.3 Enriched Category .................... 437 28.4 Preorders .......................... 439 28.5 Metric Spaces ....................... 440 x 28.6 Enriched Functors ..................... 441 28.7 Self Enrichment ...................... 443 28.8 Relation to 2-Categories ................. 445 29 Topoi 446 29.1 Subobject Classifier .................... 447 29.2 Topos ............................ 452 29.3 Topoi and Logic ...................... 453 29.4 Challenges ......................... 454 30 Lawvere Theories 455 30.1 Universal Algebra ..................... 455 30.2 Lavwere Theories ..................... 457 30.3 Models of Lawvere Theories ............... 461 30.4 The Theory of Monoids .................. 463 30.5 Lawvere Theories and Monads .............. 464
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages510 Page
-
File Size-