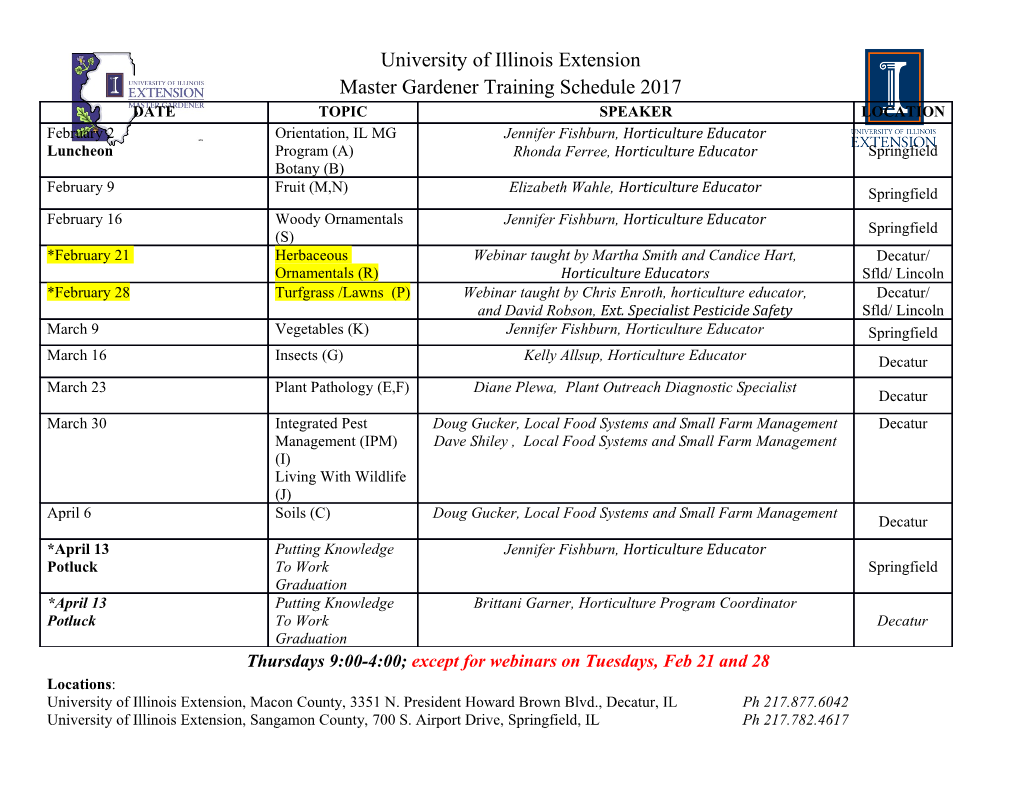
Object-Oriented Programming in Java Buzzwords responsibility-driven design inheritance encapsulation iterators overriding coupling cohesion javadoc interface collection classes mutator methods polymorphic method calls CS2336: Object-Oriented Programming in Java 2 What to Expect • Expect: fundamental concepts, principles, and techniques of object- oriented programming • Not to expect: language details, all Java APIs, JSP/JavaServlet, use of specific tools CS2336: Object-Oriented Programming in Java 3 Fundamental concepts • object • class • method • parameter • data type CS2336: Object-Oriented Programming in Java 4 What is OOP? • OOP: – identifying objects and having them collaborate with each other • procedural programming: – identifying steps that need to be taken to perform a task CS2336: Object-Oriented Programming in Java 5 Objects and classes • objects – represent ‘things’ from the real world, or from some problem domain (example: “the red car down there in the car park”) – An object has a unique identity, state, and behaviors • classes – represent all objects of a kind (example: “car”) CS2336: Object-Oriented Programming in Java 6 Methods and parameters • Objects have operations which can be invoked (Java calls them methods). • The behavior of an object is defined by a set of methods. • Methods may have parameters to pass additional information needed to execute. • Methods may return a result via a return value. • Method signature specifies the types of the parameters and return values CS2336: Object-Oriented Programming in Java 7 Data Types • Parameters have data types, which specify what kind of data should be passed to a parameter. • There are two general kinds of types: primitive types, where values are stored in variables directly, and object types, where references to objects are stored in variables. CS2336: Object-Oriented Programming in Java 8 Other observations • An object has attributes: values stored in data fields. • The state of an object consists of a set of data fields with their current values. CS2336: Object-Oriented Programming in Java 9 Other observations • Many instances can be created from a single class. • The class defines what fields an object has, but each object stores its own set of values (the state of the object). • The class provides a special type of methods, known as constructors, which are invoked to construct objects from the class CS2336: Object-Oriented Programming in Java 10 Classes class Circle { /** The radius of this circle */ double radius = 0.0; Data field /** Construct a circle object */ Circle() { } Constructors /** Construct a circle object */ Circle(double newRadius) { radius = newRadius; } /** Return the area of this circle */ double getArea() { Method return radius * radius * 3.14159; } } CS2336: Object-Oriented Programming in Java 11 Objects Class Name: Circle A class template Data Fields: radius is _______ Methods: getArea Circle Object 1 Circle Object 2 Circle Object 3 Three objects of the Circle class Data Fields: Data Fields: Data Fields: radius is 10 radius is 25 radius is 125 CS2336: Object-Oriented Programming in Java 12 UML Class Diagram UML Class Diagram Circle Class name radius: double Data fields Circle() Constructors and Circle(newRadius: double) methods getArea(): double UML notation circle1: Circle circle2: Circle circle3: Circle for objects radius = 1.0 radius = 25 radius = 125 CS2336: Object-Oriented Programming in Java 13 Constructors Constructors are a special kind of Circle() { methods that are invoked to construct objects. } Circle(double newRadius) { radius = newRadius; } CS2336: Object-Oriented Programming in Java 14 Constructors, cont. • A constructor with no parameters is referred to as a no-arg constructor. • Constructors must have the same name as the class itself. • Constructors do not have a return type— not even void. • Constructors are invoked using the new operator when an object is created. • Constructors play the role of initializing objects. CS2336: Object-Oriented Programming in Java 15 Creating Objects Using Constructors new ClassName(); Example: new Circle(); new Circle(5.0); CS2336: Object-Oriented Programming in Java 16 Default Constructor A class may be declared without constructors. • A no-arg constructor with an empty body is implicitly declared in the class. (a default constructor) • This constructor is provided automatically only if no constructors are explicitly declared in the class. CS2336: Object-Oriented Programming in Java 17 Example: Ticket machines–an external view • Exploring the behavior of a typical ticket machine. – Use the naive-ticket-machine project. – Machines supply tickets of a fixed price. – Methods insertMoney, getBalance, and printTicket are used to enter money, keep track of balance, and print out tickets. CS2336: Object-Oriented Programming in Java 18 Ticket machines – an internal view • Interacting with an object gives us clues about its behavior. • Looking inside allows us to determine how that behavior is provided or implemented. • All Java classes have a similar-looking internal view. CS2336: Object-Oriented Programming in Java 19 Basic class structure The outer wrapper of public class TicketMachine { TicketMachine Inner part of the class omitted. } public class ClassName { Fields The contents of a Constructors Methods class } CS2336: Object-Oriented Programming in Java 20 Fields • Fields store values public class TicketMachine for an object. { private int price; • They are also known private int balance; as instance variables. private int total; • Fields define the Further details omitted. } state of an object. type visibility modifier variable name private int price; CS2336: Object-Oriented Programming in Java 21 Constructors • Constructors public TicketMachine(int ticketCost) initialize an object. { price = ticketCost; • They have the same balance = 0; name as their class. total = 0; } • They store initial values into the fields. • They often receive external parameter values for this. CS2336: Object-Oriented Programming in Java 22 Assignment • Values are stored into fields (and other variables) via assignment statements: – variable = expression; – price = ticketCost; • A variable stores a single value, so any previous value is lost. CS2336: Object-Oriented Programming in Java 23 Accessor methods • Methods implement the behavior of objects. • Accessors provide information about an object. • Methods have a structure consisting of a header and a body. • The header defines the method’s signature. public int getPrice() • The body encloses the method’s statements. CS2336: Object-Oriented Programming in Java 24 Accessor methods return type visibility modifier method name parameter list public int getPrice() (empty) { return price; return statement } start and end of method body (block) CS2336: Object-Oriented Programming in Java 25 Test public class CokeMachine { private price; • What is public CokeMachine() wrong { here? price = 300 } (there are five public int getPrice errors!) { return Price; } CS2336: Object-Oriented Programming in Java 26 Test public class CokeMachine { int private price; • What is public CokeMachine() wrong { here? price = 300; } (there are five public int getPrice() errors!) { return- Price; } } CS2336: Object-Oriented Programming in Java 27 Mutator methods • Have a similar method structure: header and body. • Used to mutate (i.e., change) an object’s state. • Achieved through changing the value of one or more fields. – Typically contain assignment statements. – Typically receive parameters. CS2336: Object-Oriented Programming in Java 28 Mutator methods visibility modifier return type method name parameter public void insertMoney(int amount) { balance = balance + amount; } field being mutated assignment statement CS2336: Object-Oriented Programming in Java 29 Printing from methods public void printTicket() { // Simulate the printing of a ticket. System.out.println("##################"); System.out.println("# Ticket"); System.out.println("# " + price + " cents."); System.out.println("##################"); System.out.println(); // Update the total collected with the balance. total = total + balance; // Clear the balance. balance = 0; } CS2336: Object-Oriented Programming in Java 30 Quiz-String concatenation • System.out.println(5 + 6 + "hello"); 11hello! • System.out.println("hello" + 5 + 6); hello56! CS2336: Object-Oriented Programming in Java 31 Local variables • Fields are one sort of variable. – They store values through the life of an object. – They are accessible throughout the class. • Methods can include shorter-lived variables. – They exist only as long as the method is being executed. – They are only accessible from within the method. CS2336: Object-Oriented Programming in Java 32 Scope and life time • The scope of a local variable is the block it is declared in. • The lifetime of a local variable is the time of execution of the block it is declared in. CS2336: Object-Oriented Programming in Java 33 Local variables A local variable public int refundBalance() { int amountToRefund; amountToRefund = balance; balance = 0; return amountToRefund; } CS2336: Object-Oriented Programming in Java 34 Declaring Object Reference Variables To reference an object, assign the object to a reference variable. To declare a reference variable, use the syntax: ClassName objectRefVar; Example: Circle myCircle; CS2336: Object-Oriented Programming in Java 35 Declaring/Creating Objects in a Single Step ClassName objectRefVar = new ClassName(); Assign object reference Create an object Example:
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages82 Page
-
File Size-