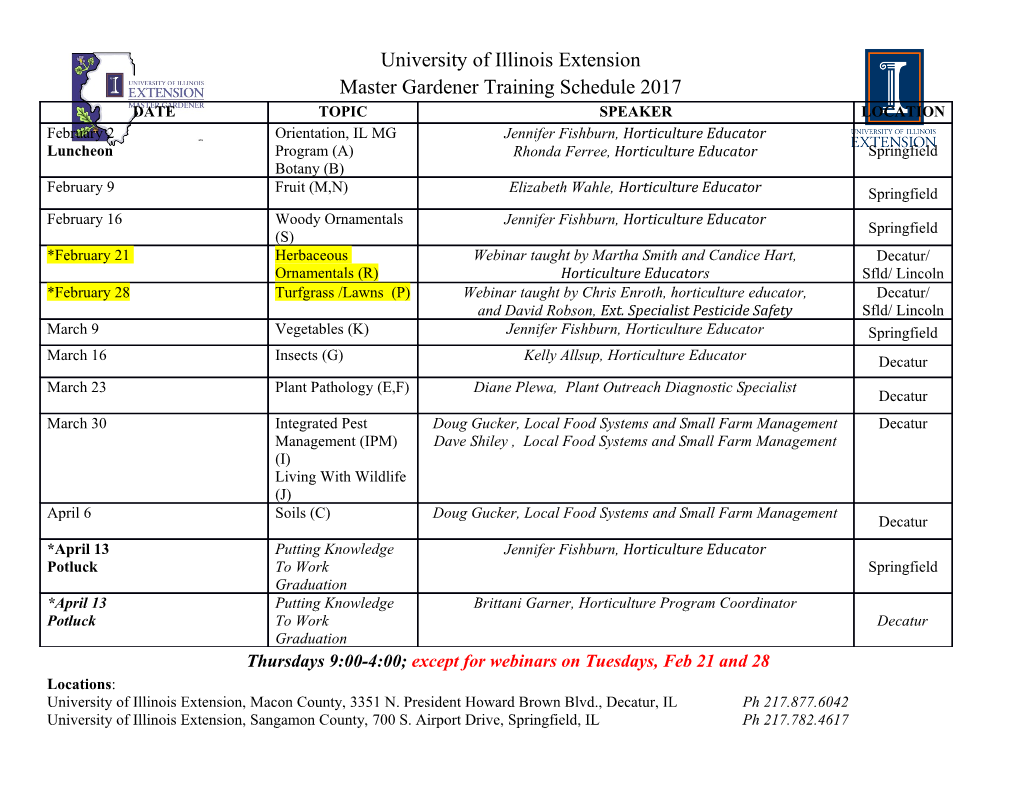
Combinatorial Coding and Lexicographic Ordering Peter Kabal Department of Electrical & Computer Engineering McGill University Montreal, Canada Version 1, February 2018 c 2018 Peter Kabal 2018/02/19 You are free: to Share – to copy, distribute and transmit this work to Remix – to adapt this work Subject to conditions outlined in the license. This work is licensed under the Creative Commons Attribution 3.0 Unported License. To view a copy of this license, visit http://creativecommons. org/licenses/by/3.0/ or send a letter to Creative Commons, 171 Second Street, Suite 300, San Francisco, California, 94105, USA. Revision History: • 2018-02 v1: Initial release Combinatorial Coding 1 1 Introduction This document examines methods to generate a combinatorial index for the selection of items, and the decoding of the index to produce the corresponding selection of items. Marching through the indices produces lexicographically ordered selections. Three cases are considered: selections with no repeated items, selections with repetitions, and selections with prescribed repetition multipli- cities. 2 Combinations – No Repetitions Consider the selection of K items from a list of N items. The order of the items in the selection does not matter, and the selection does not include repeated items. The number of unique ways to select the K items is N N = c K (1) N! = . (N − K)! K! The N items are indexed from 0 to N − 1. The K selected indices of the items can be represented as a vector of N binary values (the selected K indices are non-zero): a = [a0, a1,..., aN−1], an 2 f0, 1g. (2) The constraint on having K non-zero binary digits is N−1 ∑ an = K. (3) n=0 The vector a interpreted as binary number (least significant bit first) lexicographically orders the selections. However, many of the 2N possible values of a do not satisfy the constraint of having exactly K non-zero values. An alternate representation uses a vector of K indices, Ia = [Ia(1), ..., Ia(K)], where Ik 2 f0, 1, . , N − 1g. (4) The vector of indices is in (sorted) ascending order. If Ia is considered to be a N-ary number (least significant digit first), it can also be used to lexicographically order the selections. However, many K of N possible value of Ia are either permutations of allowed (sorted) values or have repeated indices. Combinatorial Coding 2 As an example, consider choosing 3 items from a collection of 20 items. The number of selecti- ons (order does not matter, no repetitions allowed) is 1140. This means that for the vector a, only 1140 of the 1 048 576 possible values represent the selection of exactly 3 values. For the vector Ia, only 1140 values out of 27 000 possible values represent valid sorted-order selections. N The goal is to create a combinatorial index, taking on values from 0 to (K) − 1, from a valid a or Ia vector. Conversely, a valid a or Ia vector will be created from the combinatorial index. Each combinatorial index should correspond to a unique selection of K items – ordered combinatorial indices will correspond to selections in lexicographic order. An algorithm to index the combinatorial cases was presented by Schalkwijk [1].1 Adapted to the convention of increasing lexicographic order, the index is calculated as N−1 n ic(a) = ∑ an , (5) n=0 kn where kn is an item count vector, n kn = ∑ ai. (6) i=0 The value kn is the number of selected items with index less than or equal to n – this count can be n updated on the fly, kn+1 = kn + an. It should be noted that the expression for (k) is defined to be zero for n < k. Using Ia, Eq. (5) can also be written as K Ia(k) ic(Ia) = ∑ . (7) k=1 k Note that this expression assumes that Ia(k) has been sorted (increasing values). Calculating the index involves the summation of K combinatorial values. 2.1 Coding trellis – no repetitions The process of generating the index can be viewed as traversing a trellis. The trellis for K = 3 is shown in Fig. 1. Each diagonally upward transition from node (n, k) has an associated com- n binatorial term (k). Start at the lower left corner with the item index n set to zero, the number 1The present author described the combinatorial coding scheme for use in a multipulse speech coder [2]. A combi- natorial index was used to code the position of a fixed number of pulses chosen from a set of possible positions. After the presentation of that conference paper, J.-P. Adoul brought the Schalkwijk paper [1] to the this author’s attention. Later, combinatorial coding was used in a standardized speech coder (ITU-T G.723.1 [3]). See [4], for a description of the details of the combinatorial coding and decoding used in G.723.1. Combinatorial Coding 3 of selected items k set to zero, and the combinatorial index set to zero. At each value of n, move horizontally to the right if that item is not selected. If an item is selected, move up diagonally and add the corresponding combinatorial term on the transition to the combinatorial index. The process is terminated after reaching K items. " = # 2 3 4 4 3 2 1 N – N – N – N – •3! •3! •3! • 3 ! • 3 ! • 3 ! • 3 ! " = 2 1 2 3 $ $ N –3 N –2 • ! • ! • ! • ! • ! • ! • ! 2 2 2 " " 2 2 " = 1 0 1 2 3 4 4 3 N – N – •1! •1! •1! •1! •1! • 1 ! • 1 ! " = 0 0 1 2 3 4 5 $ N –4 N –3 N –2 N –1 Fig. 1 Trellis diagram for combinatorial coding for (K = 3) 2.2 Combinatorial index / lexicographic order Consider the partial sum of the combinatorial terms for a sequence of diagonal moves starting from the bottom of the trellis at node (n, 0), L n + k − 1 Sc(L, n) = ∑ , 1 ≤ L ≤ K. (8) k=1 k The goal is to find a closed form for this partial sum. A proof by induction will be used: • Propose a formula for the partial sum of L terms. • Show that this formula applies for L = 1. • Induction hypothesis: Show that the formula applies when the partial sum is extended from L terms to L + 1 terms. The partial sum formula is n + L S (L, n) = − 1. (9) c L A direct direct evaluation for L = 1 shows that the formula applies. Now assume the partial sum Combinatorial Coding 4 formula applies for L terms. For L + 1 terms, n + L S (L + 1, n) = + S c L + 1 L (10) n + L n + L = + − 1. L + 1 L Using the combinatorial identity, p p − 1 p − 1 = + , (11) q q q − 1 then n + L + 1 S (L + 1, n) = − 1. (12) c L + 1 This shows that the partial sum formula applies for all L. From the trellis diagram, the smallest combinatorial index is generated by moving up the left- most diagonals. Application of the sum along the diagonals shows that the leftmost diagonal Sc(K, 0) is zero.) This is the combinatorial index for choosing the first K items. The largest sum is N generated by the rightmost diagonals. This sum is Sc(K, N − K) is (K) − 1. This is the combinato- rial index for choosing the last K items. The goal is to show that the combinatorial index imposes a lexicographic ordering of the se- lected items. Consider an induction argument for a vector a of length n and weight k. All such a vectors result in a path through a sub-trellis ending at node (n, k). Assume that the combinatorial n indices for all such a correspond to a lexicographic order of the vectors. There are (k) vectors of length n and weight k. Combinatorial indices take on all values from zero (path along the leftmost n diagonals in the sub-lattice) to (k) − 1 (path along the rightmost diagonals in the sub-lattice). Now increase the length of a by one. If the added element is zero, there is no change in the lexicographic value of the vector a, k remains unchanged, and there is no change in the combina- torial index. If the added element is a one, the new a is lexicographically larger than the original n vector, and k is increased by one. The corresponding combinatorial index is increased by (k). This value is larger (by one) than the largest possible index produced by the original vector a. The combinatorial index is in the lexicographic order of the new a and the largest possible index at the n+1 new node is (k+1) − 1. It is straightforward to verify that the combinatorial indices for small n and k are in proper order. This then completes a proof of the correct one-to-one ordering of the combinatorial index and the lexicographic order of vectors a for a fixed N and K. Combinatorial Coding 5 2.3 Decoding the combinatorial index – no repetitions The decoding of a combinatorial index to a a vector is accomplished by following the coding trel- lis from its terminal point back to the beginning point. In Fig. 2, the trellis has been redrawn by flipping the coding trellis and reversing the transitions. Start with the combinatorial index at the upper left corner. Compare that combinatorial index with the combinatorial term on the diago- nally downward transition. If the index is larger than the combinatorial index, the corresponding item has been selected. Subtract the combinatorial term from the index and follow the downward diagonal transition.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages32 Page
-
File Size-