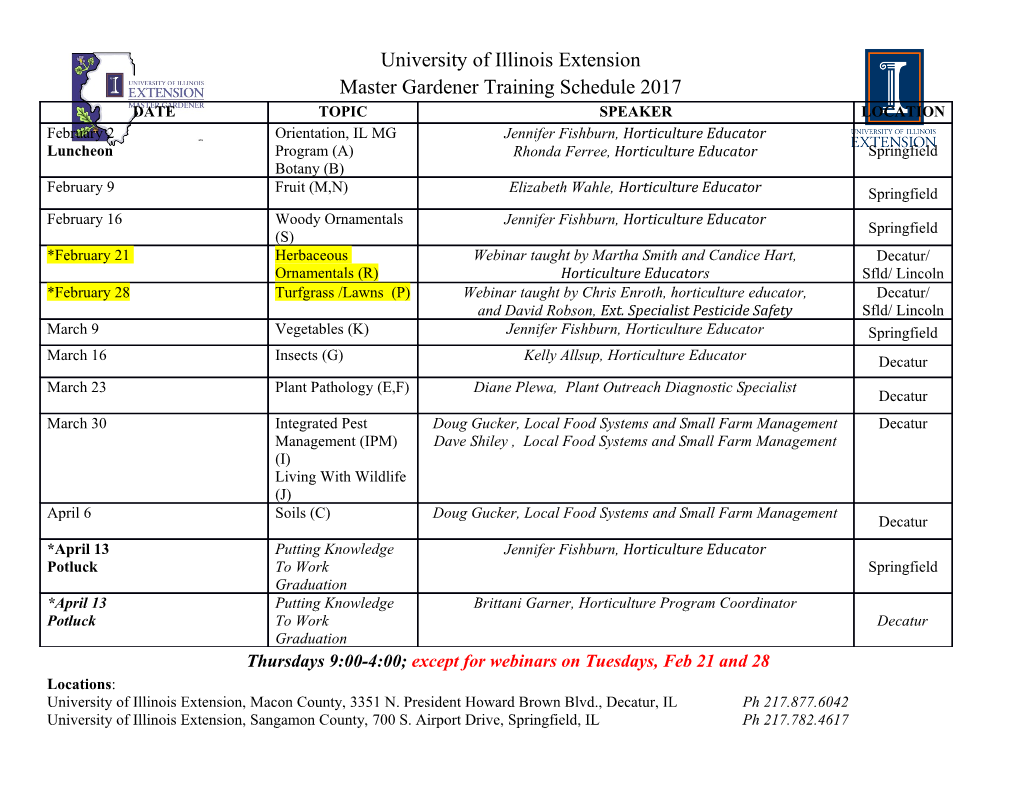
Anonymous Functions © 2008 Haim Michael Introduction An anonymous function is a function without a name. We can create an anonymous function by creating a function expression and assigning it to a variable. The function expression is the anonymous function. ... var ob = function(numA,numB) { return numA+numB; }; ... © 2008 Haim Michael Introduction <html> <head></head> <body> <script type="text/javascript"> var gogo = function(numA,numB) { return numA+numB; }; alert(gogo(4,3)); </script> </body> </html> © 2008 Haim Michael Recursive Functions When developing an anonymous function we can use the callee function in order to implement recursion. © 2008 Haim Michael Recursive Functions <html> <head></head> <body> <script type="text/javascript"> var factorial = function(num) { if(num==0) { return 1; } else { return num*arguments.callee(num-1); } } var factor = factorial; alert("factorial of 4 is "+factor(4)); </script> </body> </html> © 2008 Haim Michael Closures Closure is the combination of a function bundled together (enclosed) with reference to its surrounding state (the lexical environment). In JavaScript, we create a closure whenever we define a function. When we define a function within another function then the inner function will hold a reference to the local variables of the outer one as well as to all the global variables. © 2008 Haim Michael Closures <html> <head></head> <body> <script type="text/javascript"> var calc = function(numA,numB) { var number_a = function() { return numA * 2; }; var number_b = function() { return numB * 2; }; return number_a()+number_b(); }; alert("calling calc(2,3) returns "+calc(2,3)); </script> </body> </html> © 2008 Haim Michael Closures Using closures might be a bit tricky. The closure always gets the last value of any variable from the enclosed function. © 2008 Haim Michael Closures <html> <head></head> <body> <script type="text/javascript"> var get_functions = function() { var i = 0; var vec = new Array(); while(i<4) { vec[i] = function(){return i*10;}; i++; } return vec; }; var vector = get_functions(); alert((vector[0]()+vector[1]()+vector[2]()+vector[3]())); </script> </body> </html> © 2008 Haim Michael The this Keyword Using this within a method called on a specific object this points at the object on which the method was called. Using this within a global function this points at the Window object. Using this within the scope of a closure points at the Window object as well. © 2008 Haim Michael The this Keyword <html> <head></head> <body> <script type="text/javascript"> var name = "Moshico"; var func = function() { var name = "George"; var f2 = function() { name : "Jane"; var f1 =function() { return this.name; }; return f1(); }; return f2(); }; alert(func()); </script> </body> </html> © 2008 Haim Michael.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages11 Page
-
File Size-