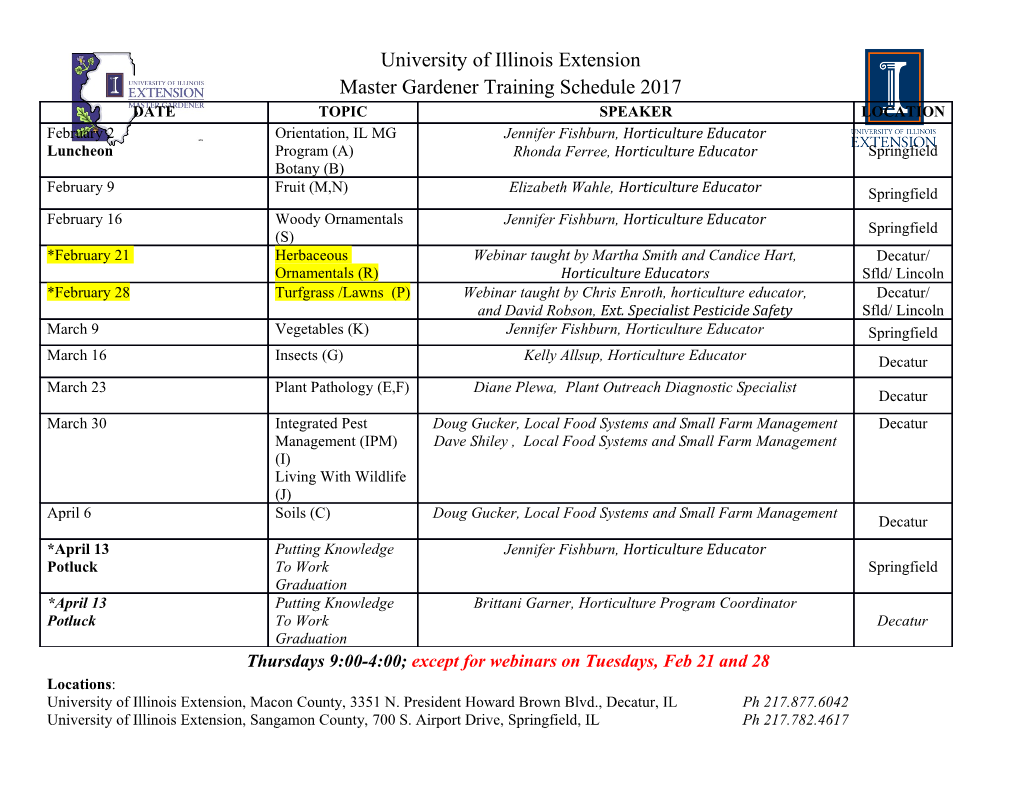
5Lesson 5: The JavaScript Document Object Model (DOM) Objectives By the end of the lesson, you will be able to: 2.2.1: Use JavaScript to manipulate the Document Object Model (DOM). 2.2.2: Use the window object of the DOM. 2.2.3: Manipulate properties and methods of the document object within the DOM. 2.2.4: Use the with statement. 2.2.5: Use the image object of the DOM, including image rollover creation. 2.2.6: Use the history object of the DOM. 2.2.7: Evaluate and change URL information with the location object of the DOM. 2.2.8: Use the navigator object of the DOM. 5-2 JavaScript Specialist Pre-Assessment Questions 1. The opener property of the window object refers to: a. a parent frame in a frameset. b. a parent window. c. the location of a window. d. a child window of another window. 2. The close() method of the document object: a. closes a document in a frame. b. closes all cookies associated with a document. c. closes a document in a window. d. closes the data stream to a document. 3. Discuss the difference between the name property and the src property of the image object. © 2011 Certification Partners, LLC. — All Rights Reserved. Version 1.01 Lesson 5: The JavaScript Document Object Model (DOM) 5-3 The JavaScript Document Object Model (DOM) OBJECTIVE JavaScript was designed explicitly for Web page use. To take advantage of the different 2.2.1: Manipulating features and capabilities provided by a browser, special browser objects have been built the DOM with JavaScript into the JavaScript language. These browser objects provide properties and methods to create sophisticated JavaScript programs. Collectively, these objects are known as the JavaScript Document Object Model (DOM). In addition, the JavaScript language itself provides objects that help you manipulate string, date and math information in useful ways. JavaScript also provides the Array object, which you will use later in this course. The JavaScript object model divides objects into three general groups: browser objects, language objects and form field objects. Figure 5-1 shows a diagram of commonly used objects recognized by JavaScript. Figure 5-1: JavaScript object hierarchy Document Object As mentioned, the objects in the preceding figure are a combination of different types of Model (DOM) A W3C specification objects. Some objects, such as the window, document and form objects, represent intended to render elements of the Document Object Model (DOM). The DOM is a World Wide Web an X/HTML page or Consortium (W3C) programming specification intended to give developers access to all XML document as a programmable elements within an X/HTML page or XML document. Other objects, such as Array and object. String, represent built-in JavaScript objects. These built-in objects will be discussed later in this course. The major browsers (Microsoft Internet Explorer and Mozilla Firefox) have introduced other objects not shown in the figure. Also, neither major browser supports all the objects shown in the figure, nor do they always support the same objects in the same manner. These compatibility issues pose a serious concern to JavaScript developers. One of the challenges in creating effective JavaScript-enabled Web applications is overcoming compatibility issues between the major browsers. Due to the complexity of these compatibility issues and the time constraints of this course, we will focus on the objects commonly used by both major browsers in this lesson. As new browsers are introduced and new browser versions are released, the chasm between the browsers narrows. Eventually, JavaScript should be standardized across all browsers. Until then, it is of utmost importance to test your JavaScript in several © 2011 Certification Partners, LLC. — All Rights Reserved. Version 1.01 5-4 JavaScript Specialist browsers, as well as in mobile devices, to ensure your code is compatible on as many platforms as possible. Containership containership The hierarchy illustrated in the preceding figure demonstrates the principle of In programming, the principle that containership. For example, the button and checkbox objects are contained within the objects are form object, which is itself contained within the document object, which is contained contained within within the top-level window object. larger objects, and some objects cannot be used or Containership relates to the principle that some objects cannot be used or referenced referenced without without a reference to the parent (container) object. For example, you cannot reference a also referencing the parent (container) form element in JavaScript without referring both to the form object and the document object. object that contains the form object. To refer to the check box named chkTennis on a form named myForm, you would write document.myForm.chkTennis. The window object is the default object that contains many objects under it, thus window does not need to be referenced by name. The document object contains the form object, which contains the form elements including the checkbox object. Note that the built-in JavaScript language objects (which are objects that do not refer to browser-related objects) are named with a capital letter. Always remember that JavaScript is case-specific. Referring to an object with improper capitalization will result in an error. Commonly used objects In this lesson, we will discuss some of the most commonly used objects and their associated properties and methods. The following sections discuss these objects. We will also introduce the navigator object, which we will examine further elsewhere in the course. The window Object OBJECTIVE The window object is the highest-level object in the JavaScript object hierarchy, and is 2.2.2: The window also the default object. The window object represents the frame of the browser and the object mechanisms associated with it, such as scroll bars, navigation bars, menu bars and so forth. Table 5-1 lists commonly used properties associated with the window object. Table 5-1: Properties of window object Property of window Description closed Returns a Boolean value indicating whether a window is open or closed. defaultStatus Specifies or returns a string value containing the default status bar text. frames Returns an array that tracks the number of frames in a window. You can target a frame in JavaScript by referencing its frame number in the frames array. Note: Frames are no longer commonly used on the Web. length Returns an integer value representing the number of frames in the parent window. Also returns the number of elements in the window.frames array. location Specifies or returns the location object for the current window. name Specifies or returns a string value containing the name of the window or frame. opener Returns a reference to the window that called (or opened) the current window. © 2011 Certification Partners, LLC. — All Rights Reserved. Version 1.01 Lesson 5: The JavaScript Document Object Model (DOM) 5-5 Table 5-1: Properties of window object (cont'd) Property of window Description parent Returns a string value containing the name of the parent window, if it has one. self Returns a string value containing the name of the current window. Alternative for using the name property as previously described. status Specifies or returns a string value representing the status bar text. top Returns a string value containing the name of the topmost window. window An alternative for the self or name property. Table 5-2 lists commonly used methods associated with the window object Table 5-2: Methods of window object Method of window Object Description alert("message text") Creates a dialog box with message text as the message. close() Closes the current window. You must write window.close() to ensure that this command is associated with a window object, and not some other JavaScript object. confirm("message text") Creates a dialog box with message text displayed, along with OK and Cancel buttons. moveBy(x, y) The moveBy() method moves a window by x pixels on the x-axis and by y pixels on the y-axis. moveTo(x, y) The moveTo() method moves the top-left corner of a window to the specified position on the x-axis and y-axis. Both methods are available in Microsoft Internet Explorer 4.x (and later) and Mozilla Firefox 1.x (and later); older browsers do not recognize them. open("URL","name", Opens a new window, populated by URL, with the target name of "featureList") name, and with the features identified in featureList. print() Provides a script method for printing the contents of the current window and frame. Available in Microsoft Internet Explorer 5.x (and later) and Mozilla Firefox 1.x (and later); older browsers do not recognize it. prompt("message Pops up a message box displaying message text with a text box text","default text") for the user's response, which contains default text if not left empty. In some browsers, the word undefined displays in the text box if left empty. resizeBy(x, y) The resizeBy() method resizes a window by the specified x and y offsets along the x-axis and y-axis. resizeTo(outerHeight, The resizeTo() method resizes a window to the height and outerWidth) width specified by outerHeight and outerWidth. Both methods are available in Microsoft Internet Explorer 4.x (and later) and Mozilla Firefox 1.x (and later); older browsers do not recognize them. scrollBy(x, y) The scrollBy() scrolls the document in a window by the specified x and y offsets along the x-axis and y-axis. scrollTo(x, y) The scrollTo() method scrolls the document in a window to the specified position on the x-axis and y-axis. Both methods are available in Microsoft Internet Explorer 4.x (and later) and Mozilla Firefox 1.x (and later); older browsers do not recognize them.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages44 Page
-
File Size-