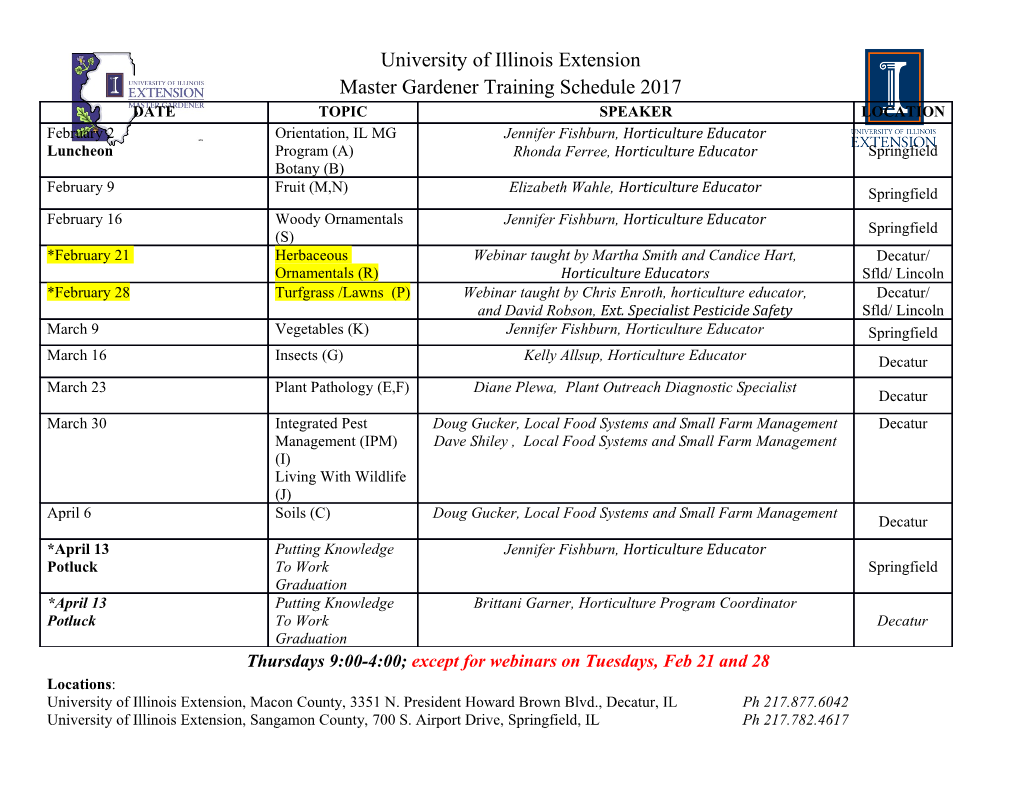
Concepts of Object-Oriented Programming Peter Müller Chair of Programming Methodology Autumn Semester 2016 5. Information Hiding and Encapsulation 2 5. Information Hiding and Encapsulation 5.1 Information Hiding 5.2 Encapsulation Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 3 Information Hiding ▪ Definition Information hiding is a technique for reducing the dependencies between modules: - The intended client is provided with all the information needed to use the module correctly, and with nothing more - The client uses only the (publicly) available information ▪ Information hiding deals with programs, that is, with static aspects ▪ Contracts are part of the exported interfaces Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 4 Objectives ▪ Establish strict interfaces class Set { … ▪ Hide implementation // contract or documentation details public void insert( Object o ) { … } ▪ Reduce dependencies } between modules class BoundedSet { - Classes can be studied and Set rep; understood in isolation int maxSize; - Classes interact only in public void insert( Object o ) { simple, well-defined ways if ( rep.size( ) < maxSize ) rep.insert( o ); } } Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 5 The Client Interface of a Class ▪ Class name class SymbolTable extends Dictionary<String,String> ▪ Type parameters implements Map<String,String> { and their bounds public int size; ▪ Super-interfaces public void add( String key,key, String value ) ▪ Signatures of { put( key, value ); } exported methods public String lookup( String key ) and fields throws IllegalArgumentException { return atKey( key ); ▪ Client interface of } direct superclass } Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 6 What about Inheritance? ▪ Is the name of the class SymbolTable { superclass part of the Dictionary<String,String> rep; client interface or an public SymbolTable( ) implementation detail? { … } public void add( String key, String value ) { … } ▪ In Java, inheritance is public String lookup( String key ) done by subclassing { … } ▪ Subtype information } must be part of the client Dictionary<String,String> d; interface d = new SymbolTable(); d.put( “var”, “5” ); Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 7 The Client Interface of a Class ▪ Class name class SymbolTable extends Dictionary<String,String> ▪ Type parameters implements Map<String,String> { and their bounds public int size; ▪ Super-class public void add( String key, String value ) ▪ Super-interfaces { put( key, value ); } ▪ Signatures of public String lookup( String key ) exported methods throws IllegalArgumentException { return atKey( key ); and fields } ▪ Client interface of } direct superclass Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 8 Other Interfaces ▪ Subclass interface package coop.util; public class DList { - Efficient access to protected Node first, last; superclass fields private int modCount; - Access to auxiliary protected void modified( ) superclass methods { modCount++; } … ▪ Friend interface } - Mutual access to implementations of package coop.util; cooperating classes /* default */ class Node { /* default */ Object elem; - Hiding auxiliary classes /* default */ Node next, prev; ▪ And others … } Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 9 Expressing Information Hiding ▪ Java: Access modifiers - public client interface - protected subclass + friend interface - Default access friend interface - private implementation ▪ Eiffel: Clients clause in feature declarations - feature { ANY } client interface - feature { T } friend interface for class T - feature { NONE } implementation (only “this”-object) - All exports include subclasses Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 10 Safe Changes ▪ Consistent renaming of package coop.util; hidden elements public class DList { ▪ Modification of hidden implementation as long protected Node first, last; as exported functionality is preserved private int modCountversion; ; protected void incrModCount(modified( ) ) ▪ Access modifiers and { modCountversion++;++; } } clients clauses specify … what classes might be } affected by a change Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 11 Exchanging Implementations ▪ Observable behavior class Coordinate { must be preserved private double x,y; … ▪ Exported fields limit public double distOrigin( ) modifications severely { return Math.sqrt( x*x + y*y ); } - Use getter and setter } methods instead - Uniform access in Eiffel class Coordinate { ▪ Modifications are critical private double radius, angle; … - Fragile baseclass problem public double distOrigin( ) - Object structures { return radius; } } Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 12 Method Selection in Java (JLS1) class T { ▪ At compile time: public void m( ) { ... } 1. Determine static declaration } 2. Check accessibility 3. Determine invocation mode class S extends T { (virtual / nonvirtual) public void m( ) { ... } } ▪ At run time: class U extends S { } 4. Compute receiver reference 5. Locate method to invoke (based on dynamic type of receiver T v = new U( ); object) v.m( ); Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 13 Rules for Overriding: Access ▪ Access Rule: class Super { … The access modifier of an protected void m( ) { … } overriding method must } provide at least as much access as the overridden class Sub extends Super { method public void m( ) { … } } Default access In class Super or Sub: protected public void test( Super v ) { v.m( ); public } Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 14 Rules for Overriding: Hiding ▪ Override Rule: class Super { … A method Sub.m private void m( ) overrides the superclass { System.out.println(“Super”); } method Super.m only if public void test( Super v ) Super.m is accessible { v.m( ); } from Sub } ▪ If Super.m is not class Sub extends Super { accessible from Sub, it is public void m( ) { System.out.println(“Sub”); } hidden by Sub.m } ▪ Private methods cannot be overridden Super v = new Sub( ); v.test( v ); Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 15 Problems with Default Access Methods ▪ S.m does not override package PT; T.m (T.m is not public class T { void m( ) { ... } accessible in S) } ▪ T.m and S.m are different methods with package PS; same signature public class S extends PT.T { public void m( ) { ... } ▪ Static declaration for } invocation is T.m ▪ At run time, S.m is In package PT: selected and invoked T v = new PS.S( ); v.m( ); Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 16 Corrected Method Selection (JLS2) ▪ Dynamically selected method must override statically determined method ▪ At compile time: ▪ At run time: 1. Determine static 4. Compute receiver declaration reference 2. Check accessibility 5. Locate method to invoke 3. Determine invocation that overrides statically mode (virtual / determined method nonvirtual) Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 17 Problems with Protected Methods ▪ S.m overrides T.m package PT; public class T { ▪ Static declaration is protected void m( ) { ... } T.m, which is } accessible for C package PS; ▪ At run time, S.m is public class S extends PT.T { selected, which is not protected void m( ) { ... } } accessible for C public would package PT; be safe ▪ protected does not public class C { always “provide at public void foo( ) { least as much access” T v = new PS.S( ); v.m( ); } as protected } Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 18 Another Fragile Baseclass Problem class C { Develop int x; Superclass public void inc1( ) { this.inc2( ); } private void inc2( ) { x++; } Implement } Subclass class CS extends C { public void inc2( ) { inc1( ); } } Modify CS cs = new CS( 5 ); Superclass cs.inc2( ); System.out.println( cs.x ); Peter Müller – Concepts of Object-Oriented Programming 5.1 Information Hiding and Encapsulation – Information Hiding 19 Another Fragile Baseclass Problem class C { Develop int x; Superclass public void inc1( ) { this.inc2( ); } protected void inc2( ) { x++; } Implement } Subclass class CS extends C { public void inc2( ) { inc1( ); } } Modify CS cs = new CS( 5 ); Superclass cs.inc2( ); System.out.println( cs.x ); Peter Müller – Concepts of Object-Oriented Programming 5. Information Hiding and Encapsulation 20 5. Information Hiding and Encapsulation 5.1 Information Hiding 5.2 Encapsulation Peter Müller – Concepts of Object-Oriented Programming 5.2 Information Hiding and Encapsulation – Encapsulation 21 Objective ▪ A well-behaved module class Coordinate { operates according to its
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages32 Page
-
File Size-