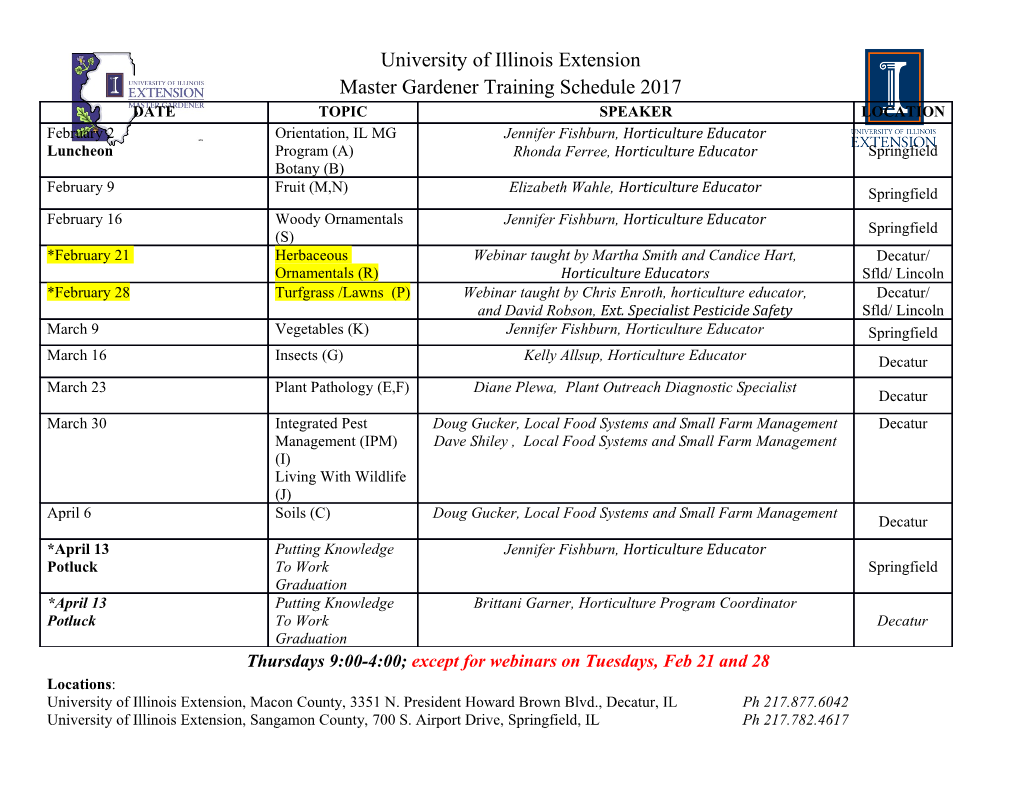
Tractable graph problems and algorithms 1. Graph traversal problems and algorithms . Depth-First Search (DFS) . Breadth First Search (BFS) 2. Minimum spanning tree problems and algorithms . Kruskal’s algorithm . Prim's algorithm 3. Shortest path problems and algorithms . Dijkstra’s Shortest Bellman–Ford algorithm . Floyd–Warshall algorithm 4. Maximum flow problems and algorithms . Ford–Fulkerson algorithm CP412 ADAII, Wilfrid Laurier University Introduction # 1 1. Graph traversal Graph traversal (also known as graph search) refers to the process of visiting (checking and/or updating) each vertex in a graph General traversal strategy 1. Mark all nodes in the graph as NOT REACHED (white). 2. pick a starting node. Mark it as REACHED (grey) and place it on the READY list. 3. pick a node on the READY list. Process it. Remove it from READY, mark it black. Find all its neighbors: those that are NOT REACHED should marked as REACHED and added to READY. 4. repeat 3 until READY is empty. CP412 ADAII, Wilfrid Laurier University Introduction # 2 Depth First Search DFS(Vertex v) { mark v visited set color of v to gray for each successor v' of v { if v' not yet visited { DFS(v') } } set color of v to black } CP412 ADAII, Wilfrid Laurier University Introduction # 3 example Introduction # 4 CP412 ADAII, Wilfrid Laurier University Running Time Analysis of DFS DFS(Vertex v) { // 1 mark v visited // 1 set color of v to gray // 1 for each successor v' of v { if v' not yet visited { DFS(v') // 2 } } set color of v to black // 1 } // T_u <= 6 + 2 deg+(u) Tvu (6 2 d eg ( )) vV vV 62||nE Tvu (6 d eg ( )) vV vV 6||nE Tne(,) (|| V | E |) CP412 ADAII, Wilfrid Laurier University Introduction # 5 DFS with a driver DFS{G} { for each u in V do // Initialize color[u] = white; pred[u] = NULL; time=0; for each u in V do // start a new tree if (color[u] == white) DFS(u); } DFS(u) { color[u] = gray; // u is discovered d[u] = ++time; // u discovery time for each v in Adj(u) do // Visit undiscovered vertex if (color[v] == white) { pred[v] = u; DFS(v); } color[u] = black; // u has finished f[u] = ++time; // u finish time } CP412 ADAII, Wilfrid Laurier University Introduction # 6 Breadth First Search (BFS) - algorithm BFS(G, s) { // G is the graph and s is the starting node for each vertex u ∈ V / {s} { do color[u] ← WHITE // color of vertex u } color[s] ← GRAY Q ← Ø // Q is a FIFO - queue ENQUEUE(Q, s) while (Q ≠ Ø ) { // iterates as long as there are gray vertices. u ← DEQUEUE(Q) for each v ∈ Adj[u] { if (color[v] = WHITE) { // discover the undiscovered adjacent vertices then color[v] ← GRAY // enqueued whenever painted gray ENQUEUE(Q, v) } } color[u] ← BLACK // painted black whenever dequeued } } ref. Introduction to Algorithms by Thomas A more detailed version BFS(G, s) // G is the graph and s is the starting node 1 for each vertex u ∈ V [G] - {s} 2 do color[u] ← WHITE // color of vertex u 3 d[u] ← ∞ // distance from source s to vertex u 4 π[u] ← NIL // predecessor of u 5 color[s] ← GRAY 6 d[s] ← 0 7 π[s] ← NIL 8 Q ← Ø // Q is a FIFO - queue 9 ENQUEUE(Q, s) 10 while Q ≠ Ø // iterates as long as there are gray vertices. Lines 10-18 11 do u ← DEQUEUE(Q) 12 for each v ∈ Adj[u] 13 do if color[v] = WHITE // discover the undiscovered adjacent vertices 14 then color[v] ← GRAY // enqueued whenever painted gray 15 d[v] ← d[u] + 1 16 π[v] ← u 17 ENQUEUE(Q, v) 18 color[u] ← BLACK // painted black whenever dequeued CP412 ADAII, Wilfrid Laurier University Introduction # 8 BFS - example BFS - analysis • Enqueue and Dequeue happen only once for each node. -O(V) • Sum of the lengths of adjacency lists – O(E) (for a directed graph) • Initialization overhead O(V) • Total runtime O(V+E) 2. Minimum Spanning Trees • A Minimum Spanning Tree (MST) in an undirected connected weighted graph is a spanning tree of minimum weight (among all spanning trees). • Example: CP412 ADAII, Wilfrid Laurier University Introduction # 11 The minimum spanning tree may not be unique. However, if the weights of all the edges are pairwise distinct, it is indeed unique CP412 ADAII, Wilfrid Laurier University Introduction # 12 Minimum Spanning Tree Problem Input: a connected weighted undirected graph G, Output: a minimum spanning tree of G. Greedy strategy Sort the edges of G in increasing order by weight. Add an edge as long as it doesn’t create a cycle CP412 ADAII, Wilfrid Laurier University Introduction # 13 Kruskal's algorithm Kruskal's algorithm: sort the edges of G in increasing order by length keep a subgraph S of G, initially empty for each edge e in sorted order if the endpoints of e are disconnected in S add e to S return S The slowest part turns out to be the sorting step, which takes O(m log n) time. CP412 ADAII, Wilfrid Laurier University Introduction # 14 example CP412 ADAII, Wilfrid Laurier University Introduction # 15 Why true Lemma: Let X be any subset of the vertices of G, and let edge e be the smallest edge connecting X to G-X. Then e is part of the minimum spanning tree. Proof: Suppose you have a tree T not containing e; then I want to show that T is not the MST. Let e=(u,v), with u in X and v not in X. Then because T is a spanning tree it contains a unique path from u to v, which together with e forms a cycle in G. This path has to include another edge f connecting X to G-X. T+e-f is another spanning tree (it has the same number of edges, and remains connected since you can replace any path containing f by one going the other way around the cycle). It has smaller weight than t since e has smaller weight than f. So T was not minimum, which is what we wanted to prove. CP412 ADAII, Wilfrid Laurier University Introduction # 16 CP412 ADAII, Wilfrid Laurier University Introduction # 17 The UNION-FIND Data Structure UNION-FIND supports three operations on collections of disjoint sets: Let n be the size of the universe. Create-Set(u) : O(1) Create a set containing the single element Find-Set(u): O(log n) Find the set containing the element Union(u, v): O(log n) Merge the sets respectively containing and into a common set. CP412 ADAII, Wilfrid Laurier University Introduction # 18 Kruskal’s algorithm with FIND-SET CP412 ADAII, Wilfrid Laurier University Introduction # 19 Prim's algorithm Prim's algorithm: let T be a single vertex x while (T has fewer than n vertices) { find the smallest edge connecting T to G-T add it to T } CP412 ADAII, Wilfrid Laurier University Introduction # 20 Why correct? CP412 ADAII, Wilfrid Laurier University Introduction # 21 Prim’s algorithm example CP412 ADAII, Wilfrid Laurier University Introduction # 22 CP412 ADAII, Wilfrid Laurier University Introduction # 23 CP412 ADAII, Wilfrid Laurier University Introduction # 24 CP412 ADAII, Wilfrid Laurier University Introduction # 25 CP412 ADAII, Wilfrid Laurier University Introduction # 26 CP412 ADAII, Wilfrid Laurier University Introduction # 27 CP412 ADAII, Wilfrid Laurier University Introduction # 28 Prim with heaps: make a heap of values (vertex,edge,weight(edge)) initially (v,-,infinity) for each vertex let tree T be empty while (T has fewer than n vertices) { let (v,e,weight(e)) have the smallest weight in the heap remove (v,e,weight(e)) from the heap add v and e to T for each edge f=(u,v) if u is not already in T find value (u,g,weight(g)) in heap if weight(f) < weight(g) replace (u,g,weight(g)) with (u,f,weight(f)) } The result is a total time bound of O((m + n) log n). CP412 ADAII, Wilfrid Laurier University Introduction # 29 Priority Queue is a data structure (can be implemented as a heap) which supports the following operations: insert(u, key): Insert u with the key value key in Q. u = extractMin(): Extract the item with the minimum key value in Q. decreaseKey(u, new-key): Decrease u’s key value to new-key. CP412 ADAII, Wilfrid Laurier University Introduction # 30 CP412 ADAII, Wilfrid Laurier University Introduction # 31 CP412 ADAII, Wilfrid Laurier University Introduction # 32.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages32 Page
-
File Size-