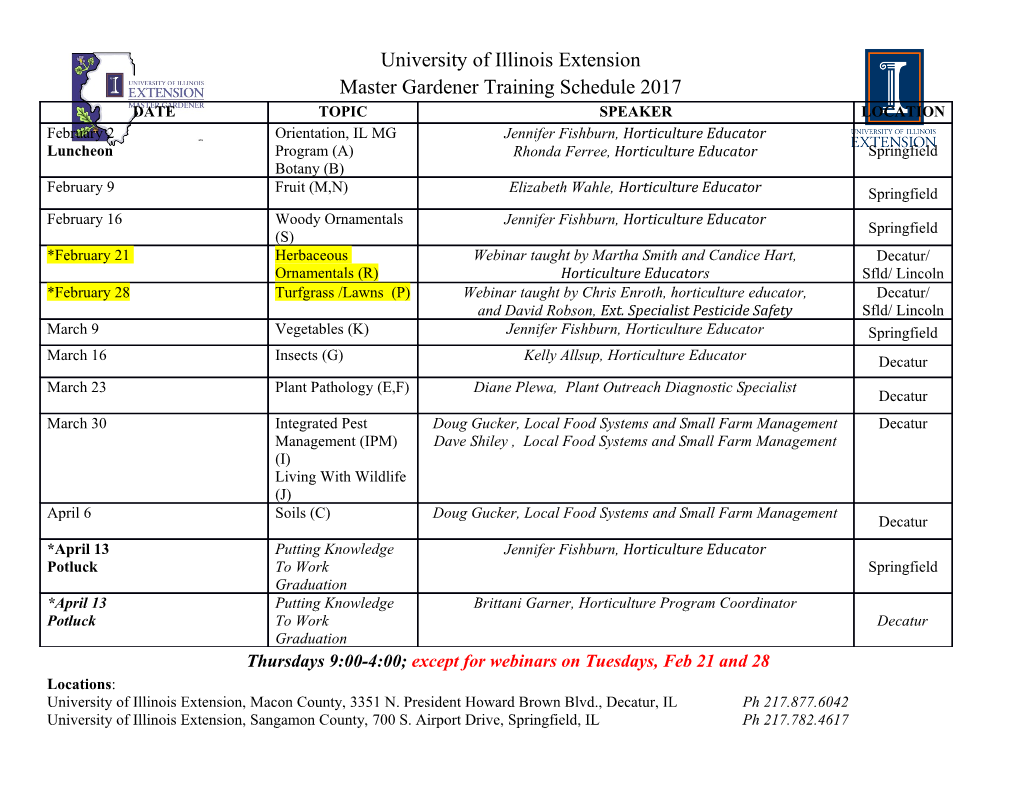
IT 11 076 Examensarbete 15 hp Oktober 2011 Backbone Solver for Water Retaining Magic Squares via Constraint Based Local Search Nicholas Baltzer Institutionen för informationsteknologi Department of Information Technology Abstract Backbone Solver for Water Retaining Magic Squares via Constraint Based Local Search Nicholas Baltzer Teknisk- naturvetenskaplig fakultet UTH-enheten Constraint programming [3] is a powerful tool for solving complex or computationally non-trivial problems. Providing a backbone model for these mathematically Besöksadress: formulated problems, in this case the Magic Square with water retention capabilities, Ångströmlaboratoriet Lägerhyddsvägen 1 makes this tool more available to those who otherwise do not have the prerequisite Hus 4, Plan 0 experience, thus widening the field of use beyond current institutions. A Magic Square is a matrix where each cell has a value ranging from one to the number of cells. These Postadress: values may only appear once in the matrix, and they must be placed such that the Box 536 751 21 Uppsala summation of each row, column, and major diagonal is the same. The water retention capacity [4] of a Magic Square is the volume that can be contained in this matrix if Telefon: each index value is treated as a height. The model has been constructed using the 018 – 471 30 03 Comet 2.1.0 software platform, based on a Local Search algorithm, since the problem Telefax: search space increases too rapidly for a systematic search solution via Gecode [1]. 018 – 471 30 00 Furthermore, two example constraints have been included beyond the scope of the basic solver. Hemsida: http://www.teknat.uu.se/student Handledare: Pierre Flener Ämnesgranskare: Justin Pearson Examinator: Anders Jansson IT 11 076 Tryckt av: Reprocentralen ITC Contents 1 Constraint Programming in Local Search6 1.1 The n-Queens problem............................6 2 The Magic Square Water Retention Problem8 2.1 Symmetry and dependency.......................... 10 3 The problem 12 4 The process 12 4.1 The algorithm................................. 12 4.2 The constraints................................ 12 4.3 The Tabu search............................... 14 4.4 The water retention calculations....................... 16 5 Results 19 6 Discussion 26 7 Acknowledgments 27 List of Figures 1 A solution to the five queens problem....................6 2 A Magic Square with summations displayed................8 3 A 3x3 square randomly generated....................... 10 4 A 6x6 square randomly generated....................... 23 5 The same 6x6 square solved with Tabu length 6, retaining 78 units.... 23 6 A 7x7 square randomly generated....................... 24 7 The same 7x7 square solved with a diamond pattern constraint and Tabu length 7, retaining 165 units.......................... 24 8 A 9x9 square randomly generated....................... 25 9 The same 9x9 square solved with a diamond pattern constraint, a low corner values constraint and Tabu length 9, retaining 849 units...... 25 List of Tables 1 Iteration performance............................. 21 2 Running time performance in milliseconds................. 21 5 Figure 1: A solution to the five queens problem 1 Constraint Programming in Local Search Constraint programming [3] is a tool-set of algorithms that specify relations between vari- ables. The basis of the paradigm is to implement relations between variables and specify the criteria for a solution rather than detail the process to reach a solution. Local Search is an approach for providing a solution non-deterministically, that is, it will compute the best solution from a subset of the solution space. The concept is em- ployed to find the best solutions when the solution space is too large to realistically sort through in a deterministic fashion. For this reason, the constraint solver Comet 2.1.0 [2] was used as it has the requisite tools to perform Local Search operations. 1.1 The n-Queens problem Consider the following problem known as n Queens [5], originally introduced in 1850 by Carl Gauss. The goal is to place n queens on a Chess board of size n × n without having any queen threaten another. Thus, no queen may be placed on the same row, column, or diagonal. Using Constraint Programming deterministically, a solution is found by placing a single queen on the board, then removing from all other queens the possibility of place- ment on a threatening square. As each queen is placed on the board, the threatening squares are removed as possibilities from the remaining queens. The process is a two step sequence, first removing placements from the queens, and then placing a queen on the board. Should no square remain viable while one or more queens still need to be placed, the algorithm will backtrack to its most recent non-failed branching choice and pick an alternate path. If this algorithm is run multiple times, the same solution will always be presented. Now, consider the problem from a Local Search perspective. Instead of placing the 6 queens one by one on an initially empty board, all queens are placed randomly on the board at the start. Each queen will then receive a "violation" value, indicating how many constraints it is breaking (in this case, how many other queens it threatens). To solve the problem, repeatedly move a queen onto a free square on the board such that the sum of these violations decreases. Once the sum of violations reaches zero, a solution has been found. By randomly placing queens on the board, the algorithm will not necessar- ily find the same solution if run multiple times in a row. Thus, consider the additional optimality constraint that queens should be placed as close to the bottom left corner as possible. With the random starting point some of these solutions will have queens closer to the bottom left corner than others, resulting in a different optimality value. The Local Search approach cannot guarantee that the solution provided is the optimal one, where as the deterministic approach can. However, if the board is of great size, the deterministic algorithm is unlikely to ever finish its calculations within human patience. 7 Figure 2: A Magic Square with summations displayed 2 The Magic Square Water Retention Problem A Magic Square is a square of arbitrary size n × n, though n is always greater than two. Each index of the Magic Square matrix holds a number in the range (1::n2) where n is the length of the matrix side. The "magic" component holds if the summations of each row, each column, and both main diagonals are the same. While calculating a Magic Square is non-deterministic polynomial (NP)-hard; the sum each row, column, and diagonal should have is not. This value is given by the formula n2 · (n2 + 1) ÷ 2n. The water retention of a Magic Square is the volume contained by the non-peripheral indices. Consider the value of each index to be the height of a block on a three dimen- sional Magic Square. Water can only flow in straight directions, so one lower block will retain water if bordered by four blocks of greater height. Water will flow off the matrix should there be a path leading from a given block onto any block on the periphery of the matrix. The water retained on any given index is the minimum of the total height (height of the index + water retained on the index) of the four adjacent indices. If the Magic Square matrix is represented by m and the water retention matrix is represented by w, the formula for water retention is wi;j = mk;l + wk;l − mi;j where mk;l 2 A(mi;j): mk;l + wk;l ≤ mx;y + wx;y 8x 2 (i − 1::i + 1); 8y 2 (j − 1::j + 1) and A(mi;j) is the set of all indices adjacent to mi;j. Implemented via Comet 2.1.0, the constraint code is described below. Water Retention constraint() 1 for each i in rInnerRange 8 2 do for each j in rInnerRange 3 do w[i; j] = max(m[i; j]; min( 4 min(max(w[i + 1; j]; m[i + 1; j]); max(w[i − 1; j]; m[i − 1; j])); 5 min(max(w[i; j + 1]; m[i; j + 1]); max(w[i; j − 1]; m[i; j − 1])))); Likewise, the total water retention is given by the sum of water on all the indices. The problem is thus one of optimisation. The Magic Square constraints greatly reduce the possibilities of value placement on the indices, and introduce symmetries in the ma- trix. Every changed value in the matrix will affect the values in the same row, the same column, and possibly one or more diagonals. Furthermore, each change in a value might affect all non-periphery water retention values. 9 Figure 3: A 3x3 square randomly generated. 2.1 Symmetry and dependency Consider Fig.3, a 3 × 3 Magic Square randomly generated. By using the formula n2 · (n2 + 1) ÷ 2n, we know the sum of each row, column, and main diagonal should be 32 · (32 + 1) ÷ 2 · 3 = 15. Currently, the first row has a sum of 14, which means that any index of that row can be preferentially swapped with another index if its value is one more. Thus, 8 can be swapped with 9, 2 can be swapped with 3, and 4 can be swapped with 5. All of these swaps will reduce the number of violations for the first row. Looking at the first column, the sum is 16 with values 8, 5, and 3. The preferred swaps will thus reduce the sum by 1, and preferred values for the indices will be 1 less than current values, namely 7, 4, and 2. Finally, looking at the right diagonal, the sum is 13, with preferred swaps being at least 1 point greater than the current values.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages28 Page
-
File Size-