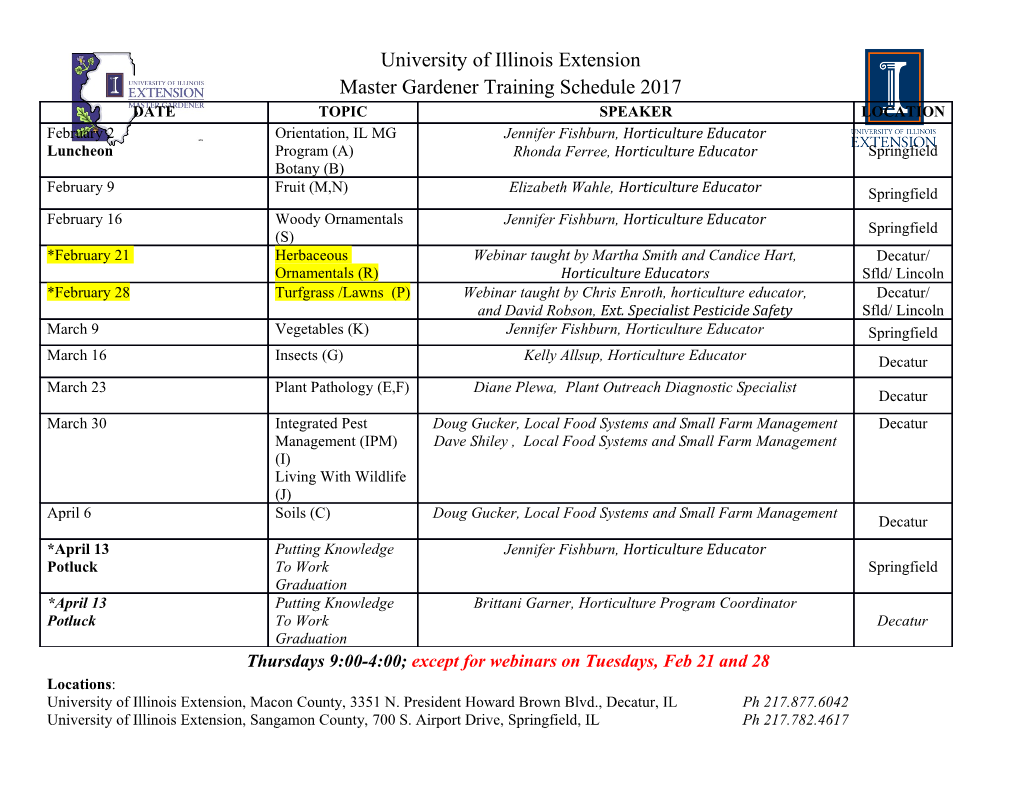
A Performance comparison Between ASP.NET Core and Express.js for creating Web APIs MAIN FIELD: Computer Science AUTHOR: Oliver Karlsson SUPERVISOR: Peter Larsson-Green JONK¨ OPING:¨ July 2021 This final thesis has been carried out at the School of Engineering at J¨onk¨oping University within computer science. The author is responsible for the presented opinions, conclusions, and results. Examinator: Anders Adlemo Handledare: Peter Larsson-Green Scope: 15 hp (bachelor's degree) Datum: 2021-07-25 Mail Address: Visiting Address: Phone: Box 1026 Gjuterigatan 5 036-10 10 00 (vx) 551 11 J¨onk¨oping 1 Abstract Modern web applications are growing in complexity and becoming more widely used. Using frameworks to build APIs is a popular way for both hobby developers and businesses to speed up development time and save costs. With this dependence on frameworks to be the foundation for potentially large applications comes the need to understand their performance qualities and which areas they are best suited for. This study compares the performance of the two similarly popular frameworks ASP.NET Core and Express.js, when used together with a MySQL Database to build Web APIs. This was done by building two different API implementations in each framework, one employing a RESTful approach and the other using the new querying language GraphQL. Experiments were run where the peak CPU usage, peak memory usage and response times were measured. The results of the experiments were that in a RESTful API, ASP.NET Core is faster at serving requests during lower loads whereas Express.js outperforms ASP.NET Core when faced with a higher amount of concurrent requests that fetch a lot of data. In a GraphQL API Express.js was able to perform similarly or better in all cases in terms of response times and resource usage compared to ASP.NET Core. Keywords API, Framework, REST, GraphQL, HTTP, SQL, ASP.NET Core, Express.js, Operating System, CPU, Memory, Response times. 2 Contents 1 Introduction 5 1.1 Background . .5 1.2 Problem description . .6 1.3 Limitations . .6 1.4 Aim & Research Objectives . .7 2 Theoretical Framework 7 2.1 Link between research questions and theory . .7 2.2 Representational State Transfer . .7 2.2.1 The Six Principles of REST . .8 2.2.2 Request & Response . .8 2.3 GraphQL . .9 2.3.1 Schema . .9 2.3.2 Query . 10 2.3.3 Execution flow . 10 2.4 ASP.NET Core . 10 2.4.1 Threads . 11 2.4.2 Tasks & Async/Await . 11 2.4.3 C# Compiler . 12 2.4.4 Memory Management . 12 2.5 Express.js . 13 2.5.1 Event Loop . 13 2.5.2 V8 JavaScript engine . 15 2.5.3 Memory Management . 15 3 Method and Implementation 16 3.1 Work Process . 16 3.2 Data Collection . 17 3.3 Test Design . 18 3.4 Database . 18 3.4.1 Schema . 18 3.4.2 Data . 19 3.5 Implementation . 19 3.5.1 API Specification . 20 3.6 ASP.NET Core . 21 3.6.1 GraphQL . 21 3.7 Express.js . 21 3.7.1 GraphQL . 22 3.8 Environments . 22 3.8.1 Client . 22 3.8.2 Server . 22 3.9 Validity & Reliability . 23 4 Empirical Data 24 4.1 REST . 24 4.2 GraphQL . 27 3 5 Analysis 30 5.1 Research Question 1 . 30 5.1.1 CPU Usage . 30 5.1.2 Memory Usage . 31 5.1.3 Response times . 32 5.2 Research Question 2 . 33 5.2.1 CPU Usage . 33 5.2.2 Memory Usage . 34 5.2.3 Response times . 35 6 Conclusions & Discussion 36 6.1 Findings . 36 6.2 Implications . 37 6.3 Limitations . 37 6.4 Conclusion . 37 6.5 Future Work . 38 7 References 39 A Appendices 42 A SQL .................................... 42 B Top & Grep Commands . 43 C Faker.js App . 43 D Application Repositories . 44 4 1 Introduction 1.1 Background The use of Application Programming Interfaces(APIs) when creating mobile and web applications is an effective way for an application to have remote access to data or other devices, but because of this there is a greater need for performance than before. APIs are commonly found in IoT(Internet Of Things) devices where things such as common household items or vehicles are able to exchange information or be controlled by a user through the internet. They are also used to create Single Page Applications which are applications that loads a single web document with the necessary HTML, CSS and JavaScript. These static pages then fetch the content that should be displayed through a Web API. Frameworks can be viewed as "semi-complete" applications that provide a specific set of structures and functionalities[1] that developers can extend and use to build complete software. Frameworks encourage reusing code, allowing for quick implementation of functionality thereby cutting development times and costs[2]. This makes them a very attractive solution to developers and companies that want to get started quickly. Selecting a framework for a specific project often means having to consider multiple factors: which platform can it support and what devices will the userbase use to access it. What will the expected size and complexity of the project be, does it need to be easily scalable to accommodate fluctuations in user traffic? One must also consider the knowledge required to use the framework for its intended goal. Is the developer team already familiar with the language and style it uses, will they need time to learn or should someone with the knowledge be hired? ASP.NET Core and Express.js are two open-source Web frameworks that can be used to develop APIs. Express.js was selected for this study because of its high popularity[3], its simplicity and because it uses JavaScript to develop software which is a popular language for front-end development. ASP.NET Core is just under Express.js in popularity[3] and according to the Stack Overflow developer survey done in February 2020 it is the most preferred web framework by professional developers[4]. 5 1.2 Problem description The amount of web applications that rely on APIs for data is increasing. ProgrammableWeb is an information and news source regarding APIs, they also provide an API directory used to catalogue and document API usage. In 2019 they reported that they added 168 APIs to their directory each month resulting in over 2000 new APIs each year on average[5]. With applications growing in complexity and the number of users on the internet steadily increasing, it can make it harder for a developer to decide which technology is aptly used for a projects desired outcome. Response times and loading times on websites and applications greatly impact user experience and a large portion of this is the server performance. In 2018, BBC reported that for every extra second a page took to load, around 10% of users left[6]. COOK is a company that sells frozen meals through their website. They thought that slow loading times were having a negative effect on their business. A case study was done and showed that reducing the average load time by 850 milliseconds increased the amount of users making a purchase by 7%, and increased customer engagement by 10%[7]. Erlandsson & Remes[8] tested and compared the performances of REST, SOAP and GraphQL on an API built in .NET Core. They found that in most test cases GraphQL performed worse than REST and SOAP regardless of database type used, while REST had the best performance across the board. The cases where GraphQL outperformed REST and SOAP were when a 100 rows were requested in a MySQL database, and when only a single row was requested in a MongoDB database. This shows that there could be a possibility that if a specific framework performs better under certain conditions it would be more effective to make use of a GraphQL architecture. With an increase in applications that depend on APIs and the growing number of people using the internet. The importance of choosing technology that is able to provide users with the performance that they want becomes greater. This study will focus on comparing the performance of ASP.NET Core and Express.js using a REST(REpresentational State Transfer) architecture, and the new querying language GraphQL. The reason for choosing these architectures is because most Web APIs use REST or an architecture close to it, while GraphQL is very new and not widely used[9]. GraphQL has been shown to require less effort compared to REST when implementing the same functionality [10] and knowing it's performance in different frameworks may therefore be a point of interest to both new and experienced developers. 1.3 Limitations This study will only be focusing on the performance of each framework and how they compare to each other. To ensure that the results are not influenced by constantly changing variables such as internet speed, consistency and reliability, both APIs will be hosted offline and accessed through a local area network connected by ethernet with a latency of less than 1ms. The database will also be 6 located on the same machine hosting the APIs to remove any latency that would occur if it were hosted on a separate machine. Both APIs will only be implemented together with a MySQL database. MySQL has consistently been a popular database amongst developers[11{14] for its ease of use, high performance and scalability[15]. To reduce the scope of the study the database will only be used to fetch data from and therefore the performances when writing to a database will not be evaluated in this study.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages45 Page
-
File Size-