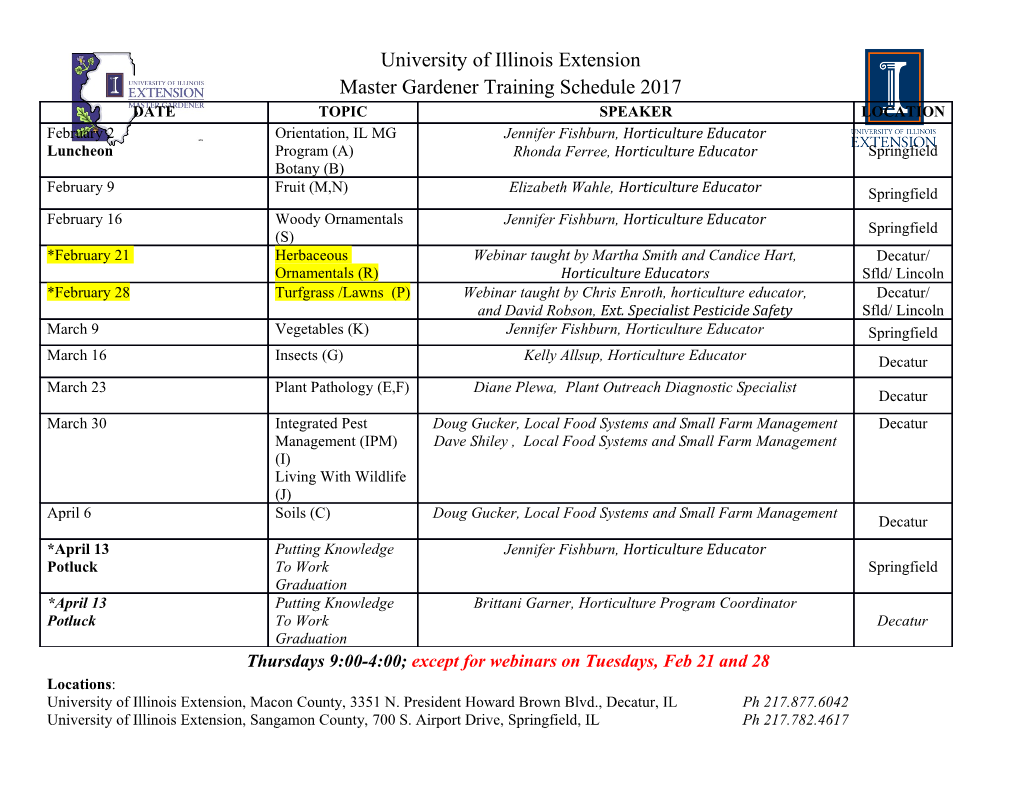
#15 CONTENTS INCLUDE: n Groovy/Java Integration n Language Elements n Operators n Groovy Collective Datatypes n Meta Programming n Hot Tips and more... By Dierk König Visit refcardz.com GroovyShell ABOUT GROOVY Use groovy.util.GroovyShell for more flexibility in the Binding and optional pre-parsing: Groovy is a dynamic language for the Java™ Virtual Machine (JVM). It shines with full object-orientation, scriptability, optional GroovyShell shell= new GroovyShell(); typing, operator customization, lexical declarations for the Script scpt = shell.parse("y = x*x"); most common data types, advanced concepts like closures and Binding binding = new Binding(); ranges, compact property syntax and seamless Java™ integra- scpt.setBinding(binding); tion. This reference card provides exactly the kind of information binding.setVariable("x", 2); you are likely to look up when programming Groovy. scpt.run(); (int) binding.getVariable("y"); STARTING GROOVY Chapter 11 of Groovy in Action has more details about Install Groovy from http://groovy.codehaus.org and you will integration options. Here is an overview: have the following commands available: Integration option Features/properties Command Purpose Eval/GroovyShell for small expressions + reloading, security groovy Execute Groovy code GroovyScriptEngine for dependent scripts + reloading groovyc Compile Groovy code - classes, security groovysh Open Groovy shell GroovyClassLoader the catch-all solution + reloading, security groovyConsole Open Groovy UI console Spring Beans integrates with Spring + reloading java2groovy Migration helper JSR-223 easy language switch but limited in API - reloading, security requires Java 6 The groovy command comes with -h and --help options to show all options and required arguments. Typical usages are: www.dzone.com Get More Refcardz! Execute file MyScript.groovy LANGUAGE ELEMENTS groovy MyScript Evaluate (e) on the command line Classes & Scripts groovy -e "print 12.5*Math.PI" A Groovy class declaration looks like in Java. Default visibility modifier is public Print (p) for each line of input echo 12.5 | groovy -pe class MyClass { "line.toDouble() * Math.PI" void myMethod(String argument) { Inline edit (i) filedata.txt by reversing each line and save a backup } groovy -i.bak –pe } → "line.reverse()" data.txt GROOVY/JAVA INTEGRATION Get More Refcardz (They’re free!) From Groovy, you can call any Java code like you would do from Java. It’s identical. n Authoritative content n Designed for developers From Java, you can call Groovy code in the following ways. n Written by top experts Note that you need to have the groovy-all.jar in your classpath. n Latest tools & technologies Cross-compilation n Hot tips & examples Use groovyc, the <groovyc/> ant task or your IDE integration to n compile your groovy code together with your Java code. This Bonus content online enables you to use your Groovy code as if it was written in Java. n New issue every 1-2 weeks Eval Subscribe Now for FREE! Use class groovy.util.Eval for evaluating simple code that is Refcardz.com captured in a Java String: (int) Eval.xyz(1,2,3,"x+y+z"); Groovy DZone, Inc. | www.dzone.com 2 Groovy tech facts at your fingertips Language Elements (Classes and Scripts), continued Customizable Operators, continued When a .groovy file or any other source of Groovy code Operator Method contains code that is not enclosed in a class declaration, a-- a.previous() --a then this code is considered a Script, e.g. a**b a.power(b) println "Hello World" a|b a.or(b) Scripts differ from classes in that they have a Binding that a&b a.and(b) serves as a container for undeclared references (that are not a^b a.xor(b) allowed in classes). ~a ~a a.bitwiseNegate() // sometimes referred to as negate | +a a.positive() // sometimes referred to as unaryMinus | -a a.negative() // sometimes referred to as unaryPlus println text // expected in Binding a[b] a.getAt(b) result = 1 // is put into Binding a[b] = c a.putAt(b, c) Optional Typing a << b a.leftShift(b) Static types can be used like in Java and will be obeyed a >> b a.rightShift(b) at runtime. Dynamic typing is used by replacing the type a >>> b a.rightShiftUnsigned(b) declaration with the def keyword. Formal parameters to switch(a){ b.isCase(a) case b: // b is a classifier method and closure declarations can even omit the def. } [a].grep(b) Properties if(a in b) Properties are declared as fields with the default visibility a == b a.equals(b) modifier, no matter what type is used. a != b ! a.equals(b) a <=> b a.compareTo(b) class MyClass { a > b a.compareTo(b) > 0 String stringProp def dynamicProp a >= b a.compareTo(b) >= 0 } a < b a.compareTo(b) < 0 a <= b a.compareTo(b) <= 0 Java-style getters and setters are compiled into the bytecode a as B a.asType(B) automatically. Properties are referred to like Actively look for opportunities to implement println obj.stringProp // getter Hot operator methods in your own Groovy class. obj.dynamicProp = 1 // setter Tip This often leads to more expressive code. regardless of whether obj was written in Java or Groovy, the Typical candidates are ==, <=>, +, -, <<, respective getters/setters will be called. and isCase(). See also Ranges. Multimethods Methods are dispatched by the runtime type, allowing code like Special Operators class Pers { Operator Meaning Name String name a ? b : c if (a) b else c ternary if boolean equals(Pers other) { a ?: b a ? a : b Elvis name == other.name a?.b a==null ? a : a.b null safe } a(*list) a(list[0], list[1], ...) spread } assert new Pers(name:'x') == new Pers(name:'x') list*.a() [list[0].a(), list[1].a() ...] spread-dot assert new Pers(name:'x') != 1 a.&b reference to method b in method closure object a as closure a.@field direct field access dot-at OPERATORS SIMPLE DATATYPES Customizable Operators Operators can be customized by implementing/ overriding Numbers the respective method. All Groovy numbers are objects, not primitive types. Literal declarations are: Operator Method a + b a.plus(b) Type Example literals a – b a.minus(b) java.lang.Integer 15, 0x1234ffff a * b a.multiply(b) java.lang.Long 100L, 100l java.lang.Float 1.23f, 4.56F a / b a.div(b) java.lang.Double 1.23d, 4.56D a % b a.mod(b) java.math.BigInteger 123g, 456G a++ a.next() ++a java.math.BigDecimal 1.23, 4.56, 1.4E4, 2.8e4, 1.23g, 1.23G DZone, Inc. | www.dzone.com 3 Groovy tech facts at your fingertips Simple Datatypes (Numbers), continued Regular Expressions, continued Coercion rules for math operations are explained in Groovy Symbol Meaning in Action, chapter 3. Some examples to remember are: . any character ^ start of line (or start of document, when in single-line mode) Expression Result type $ end of line (or end of document, when in single-line mode) 1f * 2f Double \d digit character 1f / 2f Double \D any character except digits (Byte)1 + (Byte)2 Integer \s whitespace character 1 * 2L Long \S any character except whitespace 1 / 2 BigDecimal (0.5) \w word character (int)(1/2) Integer (0) \W any character except word characters 1.intdiv(2) Integer (0) \b word boundary Integer.MAX_VALUE+1 Integer () grouping 2**31 Integer (x|y) x or y as in (Groovy|Java|Ruby) 2**33 Long \1 backmatch to group one, e.g. find doubled characters with (.)\1 2**3.5 Double x* zero or more occurrences of x. 2G + 1G BigInteger x+ one or more occurrences of x. 2.5G + 1G BigDecimal x? zero or one occurrence of x. 1.5G == 1.5F Boolean (true) x{m,n} at least “m” and at most “n” occurrences of x. 1.1G == 1.1F Boolean (false) x{m} exactly “m” occurrences of x. [a-f] character class containing the characters 'a', 'b', 'c', 'd', 'e', 'f' Strings [^a] character class containing any character except 'a' 'literal String' (?is:x) switches mode when evaluating x; i turns on ignoreCase, s single-line mode '''literal (?=regex) positive lookahead multiline String''' (?<=text) positive lookbehind def lang = 'Groovy' "GString for $lang" "$lang has ${lang.size()} chars" COLLECTIVE DATATYPES """multiline GString with Ranges late eval at ${-> new Date()}""" Ranges appear inclusively like 0..10 or half-exclusively like Placeholders in GStrings are dereferenced at declaration time 0..<10. They are often enclosed in parentheses since the but their text representation is queried at GString String range operator has low precedence. conversion time. assert (0..10).contains(5) /String with unescaped \ included/ assert (0.0..10.0).containsWithinBounds(3.5) Regular Expressions for (item in 0..10) { println item } for (item in 10..0) { println item } The regex find operator =~ (0..<10).each { println it } The regex match operator ==~ Integer ranges are often used for selecting sublists. Range The regex Pattern operator ~String boundaries can be of any type that definesprevious() , next() and Examples: implements Comparable. Notable examples are String and Date. def twister = 'she sells sea shells' // contains word 'she' Lists assert twister =~ 'she' Lists look like arrays but are of type java.util.List plus new methods. // starts with 'she' and ends with 'shells' [1,2,3,4] == (1..4) assert twister ==~ /she.*shells/ [1,2,3] + [1] == [1,2,3,1] // same precompiled [1,2,3] << 1 == [1,2,3,1] def pattern = ~/she.*shells/ [1,2,3,1] - [1] == [2,3] assert pattern.matcher(twister).matches() [1,2,3] * 2 == [1,2,3,1,2,3] [1,[2,3]].flatten() == [1,2,3] // matches are iterable [1,2,3].reverse() == [3,2,1] // words that start with 'sh' [1,2,3].disjoint([4,5,6]) == true def shwords = (twister =~ /\bsh\w*/).collect{it}.join(' ') [1,2,3].intersect([4,3,1]) == [3,1] assert shwords == 'she shells' [1,2,3].collect{ it+3 } == [4,5,6] [1,2,3,1].unique().size() == 3 // replace through logic [1,2,3,1].count(1) == 2 assert twister.replaceAll(/\w+/){ [1,2,3,4].min() == 1 it.size() [1,2,3,4].max() == 4 } == '3 5 3 6' [1,2,3,4].sum() == 10 [4,2,1,3].sort() == [1,2,3,4] // regex groups to closure params [4,2,1,3].findAll{it%2 == 0} == [4,2] // find words with same start and end def anims=['cat','kangaroo','koala'] def matcher = (twister =~ /(\w)(\w+)\1/) anims[2] == 'koala' matcher.each { full, first, rest -> def kanims = anims[1..2] assert full in ['sells','shells'] anims.findAll{it =~ /k.*/} ==kanims assert first == 's' anims.find{ it =~ /k.*/} ==kanims[0] } anims.grep(~/k.*/) ==kanims → DZone, Inc.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages7 Page
-
File Size-