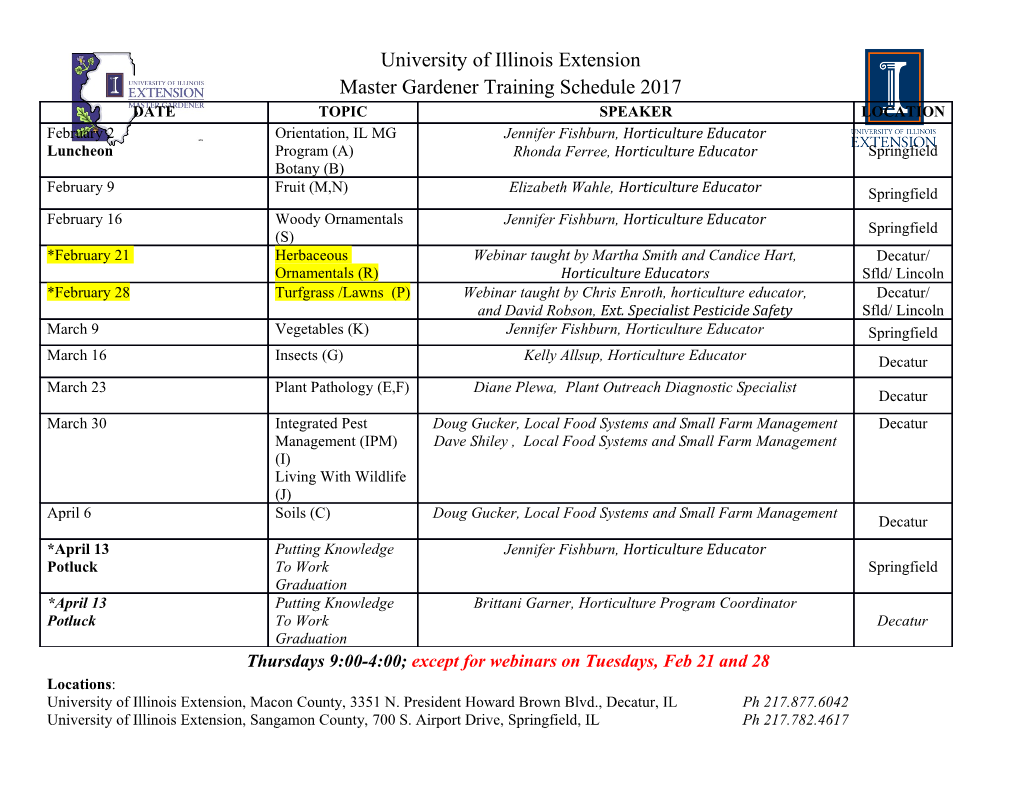
Local Search Methods for Constraint Problems Muhammad Rafiq Bin Muhammad This thesis is submitted in total fulfilment of the requirements of the degree of Master in Computer Science NICTA Victoria Research Lab Department of Computer Science and Software Engineering University of Melbourne Australia November, 2006 Revised On October, 2007 Final Revised On February, 2008 (Acid-Free Paper) Abstract This thesis investigates the use of local search methods in solving constraint prob- lems. Such problems are very hard in general and local search offers a useful and successful alternative to existing techniques. The focus of the thesis is to analyze the techniques of invariants used in local search. The use of invariants have recently become the cornerstone of local search technology as they provide a declarative way to specify incremental algorithms. We have produced a series of program libraries in C++ known as the One-Way-Solver. The One-Way-Solver includes the implementation of incremental data structures and is a useful tool for the implementation of local search. The One-Way-Solver is applied to two challenging constraint problems, the Boolean Satisfiability testing (SAT) and university course timetabling problems. In the implementation of Boolean Satisfiability testing (SAT), we introduced a pure non-clausal solver based on well-known stochastic local search techniques. It works directly on arbitrary non-clausal formula including psuedo-Boolean constructs and other extended Boolean operators. We introduced a novel scoring function using positive and negative values and empirically show that on certain benchmarks our non-CNF local search solver can outperform highly optimized CNF local search solvers as well as existing CNF and non-CNF complete solvers. In the implementation of university course timetabling problem, we developed a weighted penalty scheme (WPS) local search technique. WPS is a local search method guided by a multiplier that provides a force to determine the concentration of the search as well as mechanism to escape local minima hence it can be considered as a meta-heuristic technique. When used with the One-Way-Solver, WPS achieved very promising results in solving university course timetabling problem. Declaration The work in this thesis is based on research carried out at the NICTA Victoria Re- search Lab, the Department of Computer Science and Software Engineering, Uni- versity of Melbourne, Australia. This is to certify that (i) no part of this thesis has been submitted elsewhere for any other degree or qual- ification. (ii) the thesis comprises only my original work towards the Master. (iii) due acknowledgement has been made in the text to all other material used. (iv) the thesis is less than 100,000 words in length, exclusive of tables, maps, bibli- ographies and appendices. ................................ (MUHAMMAD RAFIQ BIN MUHAMMAD) Copyright c 2006 by Muhammad Rafiq Bin Muhammad. “The copyright of this thesis rests with the author. No quotations from it should be published without the author’s prior written consent and information derived from it should be acknowledged”. iii Acknowledgements Mostly I would like to thank my supervisor Professor Peter Stuckey for his boundless help, criticism and patience. Without his supervision and expert knowledge in Artificial Intelligence especially in constraint optimization techniques, this thesis would not have been possible. I would also like to thank all the members of G12 research group. In no particular order, thanks to: Mark Wallace, Ralph Becket, Mark Brown, Zoltan, Kim Marriott, Peter Schachte, Neng Fa and Reza who have provided help and advice during my attachment with the group. Special thanks to Noraini for her thorough proof reading and constructive criticism. Finally, I thank my wife Nurul and my kids Nurin and Amin for their patience, encouragement and companionship. Thanks to all who gave me the support during these last few years. Thanks to God Almighty for making my effort a success. iv Contents Abstract ii Declaration iii Acknowledgements iv 1 Introduction 1 1.1 Constraint Satisfaction Problem (CSP) . ... 2 1.2 Techniques in solving Constraint Problems . .... 3 1.2.1 Mathematical Programming Techniques . 3 1.2.2 Searching Techniques . 4 1.3 ConstraintProgramming . 7 1.4 ThesisContributions ........................... 8 1.5 ThesisOrganization............................ 9 2 Local Search and Invariants 11 2.1 StochasticLocalSearch. 12 2.1.1 ProblemDefinition . 13 2.1.2 ObjectiveFunction . 14 2.1.3 Local Move and Neighborhood Function . 15 2.1.4 LocalMinima/Maxima . 16 2.1.5 Meta-Heuristics . 17 2.1.6 BeyondMeta-Heuristics . 25 2.2 Invariants: One-Way-Solver . 25 2.2.1 Invariants ............................. 27 v Contents vi 2.2.2 ArithmeticInvariants. 27 2.2.3 ListInvariants........................... 35 2.2.4 Implementation . .. .. 42 2.3 ChapterSummary ............................ 44 3 Stochastic Non-CNF SAT Solver 46 3.1 SatisfiabilityProblem. 46 3.1.1 SATSolvers............................ 47 3.1.2 StochasticLocalSearchandSAT . 48 3.2 Non-CNFSAT .............................. 49 3.2.1 TheStateofnon-CNFSATSolvers . 51 3.3 StochasticLocalSearchNon-CNFSAT . 52 3.3.1 Score................................ 52 3.3.2 SearchingforaSolution . 58 3.3.3 Implementation . .. .. 60 3.4 Non-CNFSATBenchmarks . 61 3.4.1 HardRandomNon-CNFFormula . 61 3.4.2 Social Golfers as Non-CNF SAT Problem . 61 3.4.3 Minimum Disagreement Parity Problem . 64 3.5 ExperimentalResults. 67 3.5.1 HardRandomNon-CNFFormula . 68 3.5.2 SocialGolferProblem . 69 3.5.3 Minimal Disagreement Parity Problem . 70 3.6 ChapterSummary ............................ 70 4 University Course Timetabling Problem 72 4.1 University Course Timetabling . 72 4.2 Methods in Course Timetabling . 74 4.2.1 SequentialApproaches . 74 4.2.2 ClusterApproaches. 75 4.2.3 Constraint Programming Approaches . 75 4.2.4 Meta-Heuristic Local Search Approaches . 76 Contents vii 4.2.5 HybridApproaches . 77 4.3 Course Timetabling Benchmarks . 77 4.3.1 A Simple Timetabling Example . 78 4.4 Timetabling Problem Modeling . 79 4.5 SolutionRepresentation . 81 4.6 SearchingforSolution . .. .. 82 4.6.1 Searching for Feasible Solution . 82 4.6.2 SearchingforQualitySolution . 86 4.7 Implementation .............................. 86 4.8 ExperimentResults............................ 87 4.9 ChapterSummary ............................ 89 5 Conclusions 94 5.1 Invariants ................................. 94 5.2 Non-CNFSAT .............................. 95 5.3 Timetabling ................................ 96 Bibliography 97 List of Figures 2.1 Localminima[59] ............................. 17 2.2 Crossoveroftwochromosomes . 24 2.3 Topological order of arithmetic operations . ..... 28 2.4 Valuation of f(x) when A = 3, B = 4, C = 5 and D =7 ....... 28 2.5 Incrementalupdateofequationtree . .. 29 2.6 Structure representing the invariant variable for addition and sub- tractionoperations . .. .. 29 2.7 Structure representing the invariant variable for multiplication and divisionoperations ............................ 31 2.8 Topological order of arithmetic operations . ..... 32 2.9 Incrementalupdateofequationtree . .. 33 2.10Priorityqueue............................... 34 2.11Don’tcarenodes ............................. 35 2.12 Min heapforlist3,4,5,6,7,8 . 37 3.1 The propositional formula φ = (((¬A ∨ ¬B ∨ ¬C) ∧ ¬D ∧ (¬E ∨ ¬F ))∧¬C ∧((¬D ∨A∨¬E)⊕(C ∧F ))) with scores for the valuation {A, ¬B,C, ¬D, ¬E, F } bracketed. .................... 53 3.2 AND score (a) where the node is false and (b) where it is true .... 54 3.3 OR score (a) where the node is false and (b) where it is true ..... 54 3.4 XOR score (a) where the node is false and (b) where it is true .... 55 3.5 ATMOST 2 score (a) where the node is false and (b) where it is true 56 3.6 ATLEAST 2 score (a) where the node is false and (b) where it is true 57 viii List of Figures ix 3.7 COUNT 2 score (a) where the node is true and (b)(c) where they are false .................................... 58 3.8 The formula φ with scores for the valuation {A, ¬B,C, ¬D,E,F }... 60 3.9 The formula φ with scores for the valuation {A, ¬B,C, ¬D,E, ¬F }. 60 4.1 Example of empty timetable slots . 80 4.2 WPS: Escaping local minima . 83 4.3 Result of soft constraint violation for small instances ......... 89 4.4 Result of soft constraint violation for medium instances........ 90 4.5 Result of soft constraint violation for large01 instances ........ 90 4.6 Result of soft constraint violation for large02 instances ........ 91 4.7 Percentage of invalid solution for large01 . ..... 91 4.8 Percentage of invalid solution for large02 . ..... 92 4.9 Comparison for Feasible Time between Naive and Heuristic Move for MediumInstances............................. 92 4.10 Comparison for Feasible Time between Naive and Heuristic Move for LargeInstances .............................. 93 List of Tables 2.1 Gene encoding of variable x ....................... 23 3.1 Comparison of number of variables and clauses between CNF and Non-CNFencoding ............................ 64 3.2 Comparison of CNF and non-CNF encoding of MDP problem . 67 3.3 Comparative results for CNF solvers on hard random formulas . 68 3.4 Comparative results for non-CNF solvers on hard random formulas . 69 3.5 Comparative results for solvers on social golfer problems ....... 69 3.6 Comparative results for solvers on MDP problems . .... 70 4.1 RoomFeatures .............................. 78 4.2 Features required by each event . 78 4.3 Students subscription to events . 79 4.4
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages121 Page
-
File Size-