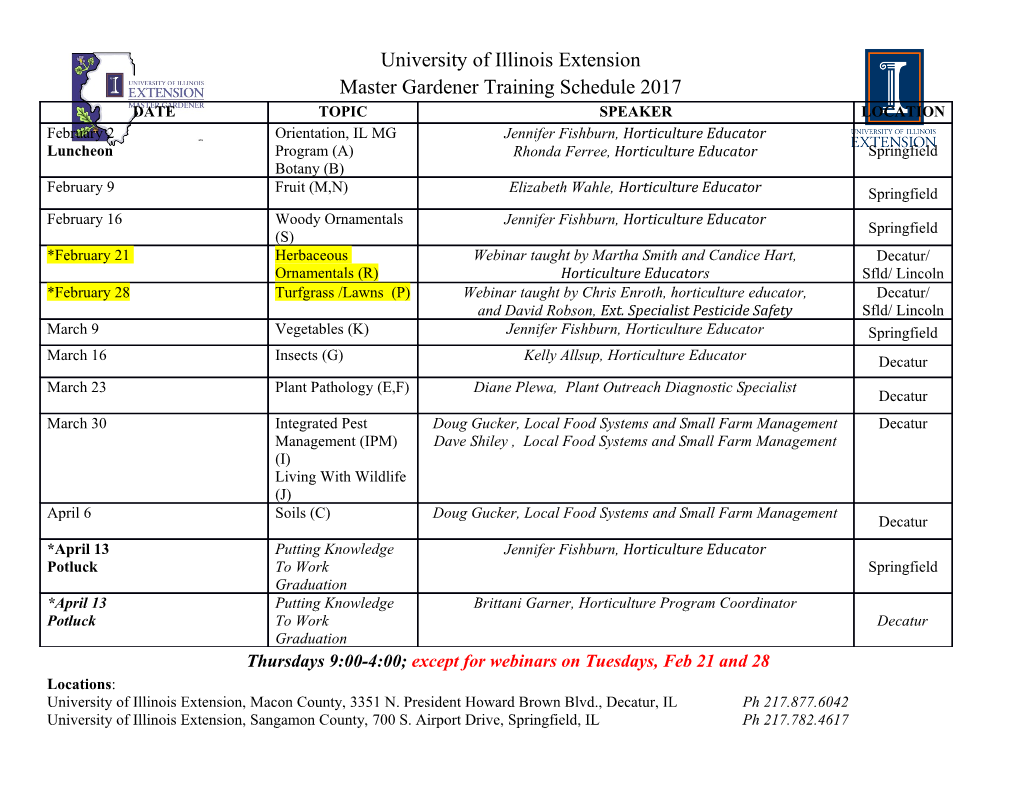
Prime Numbers COMPSCI 230 — Discrete Math January 26, 2016 COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 1 / 17 Announcement • Homework 2 is out, due on February 2nd • Click on Homework 2 ! COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 2 / 17 Outline 1 Prime Numbers The Sieve of Eratosthenes Python Implementations GCD and Co-Primes COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 3 / 17 Prime Numbers Primes • Natural numbers greater than 1 with no proper divisors • 1, 2, 3, 6 | 6 but 1, 7 | 7 • Numbers in red are proper divisors • Fundamental theorem of arithmetic: 1 < 푛 ∈ 퐍 ⇒ 푛 = ∏ 푝푖 푖 where the 푝푖s are prime • Example: 26040 = 23 × 3 × 5 × 7 × 31 • Fundamental in number theory • Applied in cryptography, electrical communications, computer chips,... COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 4 / 17 Prime Numbers Problems About Primes • Generate all primes up to 푛 (sieve) • Test whether 푛 is prime • Is 802117 prime? • Factor 푛 into primes • 802117 = 821 × 977 • You did factor, which subsumes test, so you know both • There are much more efficient methods COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 5 / 17 Prime Numbers The Sieve of Eratosthenes The Sieve of Eratosthenes To generate all primes up to 푛: • List all integers from 2 to 푛 • Let 푝 = 2 (smallest prime) • Cross 2푝, 3푝, … from the list • First remaining number must be prime • Set 푝 to that, and repeat until no more numbers are left COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 6 / 17 Prime Numbers The Sieve of Eratosthenes Example: 푛 = 36 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 7 / 17 Prime Numbers The Sieve of Eratosthenes The Sieve of Eratosthenes • Speedups exist • Takes large amounts of storage for nontrivial 푛 • Each number is touched at least once • Takes at least 푛 steps COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 8 / 17 Prime Numbers Python Implementations Primes in Python: Caveat • There is a pyprimes module in Python that implements several algorithms to generate primes, test for primality, and factor into primes • Please do explore pyprimes if interested • However, you are required to study how to accomplish the simpler versions of these tasks on your own, without pyprimes COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 9 / 17 Prime Numbers Python Implementations The Sieve in Python def sieve(n): primes = [] integers = list(range(2, n+1)) while integers: p = integers[0] primes += [p] integers = [k for k in integers if k % p > 0] return primes >>> sieve(40) [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37] COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 10 / 17 Prime Numbers Python Implementations Alternative Implementation • Current: • Generate all integers up to 푛 • For each new prime, delete its multiples • Alternative: for k in range(2, n+1): • Keep k if it is not a multiple of one of the primes found so far • Does not require to make all integers first • range(2, n+1) is an iterable, not a list • Think of it as a function that returns the next item if and when you ask for it • Advantages: • No long list of integers to store • Can be converted to a generator COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 11 / 17 Prime Numbers Python Implementations Python Generators We would like to say def primesUntilTired(): print('Hit return for the next prime,', 'anything else and return to stop') for p in allPrimes(): if input() == '': print(p, end = '') else: break • allPrimes() is a generator • Can also say prime = allPrimes() • next(prime) gives 2 • next(prime) gives 3 • ... COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 12 / 17 Prime Numbers Python Implementations Generators • A generator is a reentrant function that returns a value every time it is suspended • Execution can be suspended ... • ... and a value returned. • If the function is called again, ... • ... the function is resumed where it had been suspended • If there is no more code to execute in the generator, a StopIteration exception is raised COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 13 / 17 Prime Numbers Python Implementations A Python Prime Generator def allPrimes(): • Designed as an (infinite) loop primes = [] k = 2 • At yield: while True: isPrime = True • Suspend allPrimes for p in primes: • Remember state if k % p == 0: isPrime = False • Return argument of break yield if isPrime: primes += [k] • If allPrimes is called again, yield k k += 1 resume execution right after yield, with the old state • No StopIteration is ever raised in this case COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 14 / 17 Prime Numbers GCD and Co-Primes Greatest Common Divisor • Let 푚, 푛 be positive integers (푚, 푛 > 0) • GCD(푚, 푛) is the largest positive integer 푔 such that 푔|푚 and 푔|푛 • GCD(120, 700) = 20 • GCD(24, 72) = 24 • GCD(푎, 푏) = GCD(푏, 푎) • GCD(1, 푛) = 1 • GCD(1, 1) = 1 COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 15 / 17 Prime Numbers GCD and Co-Primes Euclid’s Algorithm • Computes GCD(푥, 푦) for 푥, 푦 > 0 • Based on the following recursion (FDM pages 18-19, without proof for now): 푦 if 푦|푥 GCD(푥, 푦) = { GCD(푦, 푥 MOD 푦) otherwise • Examples: GCD(15, 4) = GCD(4, 3) = GCD(3, 1) = 1 GCD(4, 15) = GCD(15, 4) = GCD(4, 3) = … = 1 COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 16 / 17 Prime Numbers GCD and Co-Primes Co-Primes • Two positive integers 푚 and 푛 are co-primes iff GCD(푚, 푛) = 1 • Not co-primes: 푚 = 24 = 23 × 3 and 푛 = 15 = 3 × 5 • Co-primes: 푚 = 24 = 23 × 3 and 푛 = 35 = 5 × 7 • 1 is co-prime with every positive integer: GCD(1, 푛) = GCD(푛, 1) = 1 • ... including itself: GCD(1, 1) = 1 • If 푚, 푛 > 1, then 푚 and 푛 are co-prime iff they share no prime factors COMPSCI 230 — Discrete Math Prime Numbers January 26, 2016 17 / 17.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages17 Page
-
File Size-