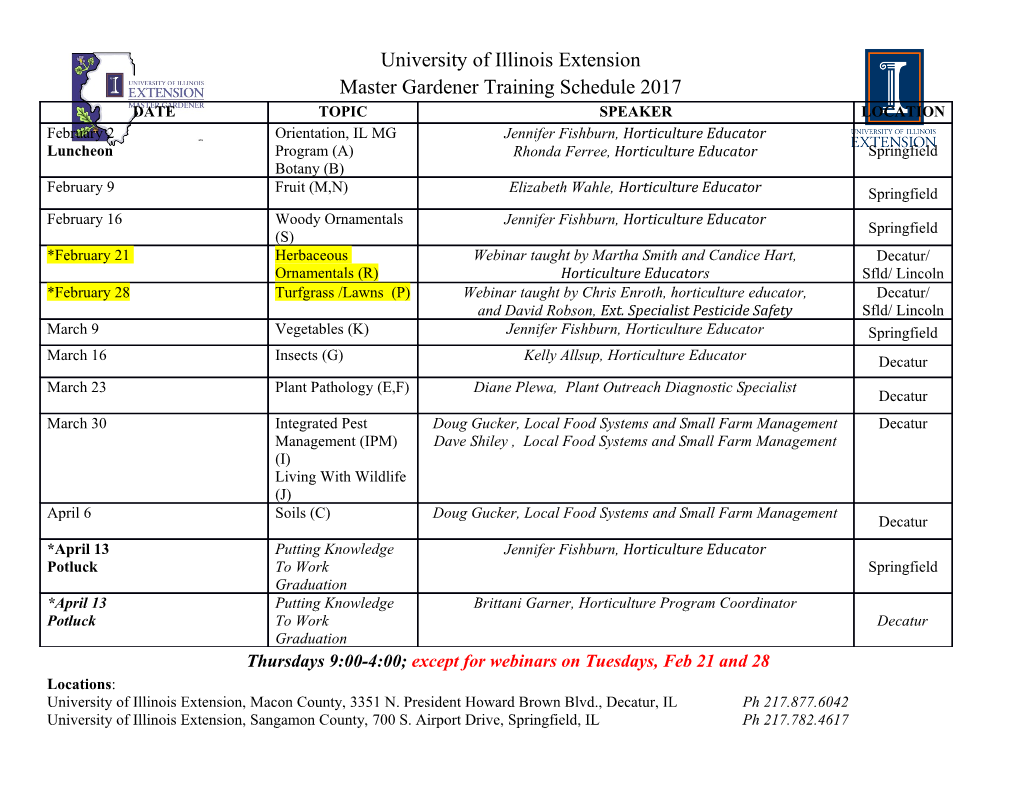
Objects and arrays Loops and Comprehensions If, Else, Unless, and Conditional Assignment · · Objects and arrays are similar to Comprehensions for ... in work · if/else can be written without paren- JavaScript over arrays, objects, and ranges theses and curly braces CoffeeScript · · Comprehensions replace for loops, When each property is listed on its own · Multi-line conditionals are delimited by with optional when guard clauses and line, the commas are optional indentation Quick Reference the value of the current array index: · Objects may be created using indenta- · if and unless can be used in postfix tion instead of explicit braces, similar to for value, index in array form i.e. at the end of the statement coffeescript.org YAML · Array comprehensions are expressions, and can be returned and assigned · · Reserved words, like class, can be used if statements can be used as expres- · as properties of an object without quot- Comprehensions may replace sions. No need for ?: ing them as strings each/forEach, map or select/filter · Use a range when the start and end of a 1 loop is known (integer steps) Chained Comparisons General Lexical Scoping and Variable Safety · Use by to step in fixed-size increments · Whitespace is significant · · · Variables are declared implicitly when When assigning the value of a compre- Use a chained comparison to test if a · Ending a line will terminate expressions used (no var keyword). hension to a variable, CoffeeScript col- value is within a range: - no need to use semicolons lects the result of each iteration into an minimum < value < maximum · The compiler ensures that variables are · array Semicolons can be used to fit multiple declared within lexical scope. An outer · expressions onto a single line variable is not redeclared within an in- Return null, undefined or true if a · Use indentation instead of curly braces ner function when it is in scope loop is only for side-effects Array Slicing and Splicing with Ranges {} to surround blocks of code in · · Using an inner variable can not shadow To iterate over the key and value prop- functions, if statements, switch, and an outer variable, only refer to it. So erties in an object, use of · Ranges can be used to extract slices of try/catch avoid reusing the name of an external · Use: for own key, value of object arrays · Comments starts with # and run to the variable in a deeply nested function to iterate over the keys that are directly · end of the line With two dots [3..6], the range is in- · CoffeeScript output is wrapped in an defined on an object clusive (3, 4, 5, 6) anonymous function, making it diffi- · The only low-level loop is the while · With three dots [3...6], the range ex- Functions cult to accidentally pollute the global loop. It can be used as an expression, cludes the end (3, 4, 5) namespace returning an array containing the result · Functions are defined by an optional list of each iteration through the loop · The same syntax can be used with as- · To create top-level variables for other of parameters in parentheses, an arrow, signment to replace a segment of an ar- scripts, attach them as properties on · until is equivalent to while not and an optional function body. The ray with new values, splicing it window, or to exports in CommonJS. · loop is equivalent to while true empty function looks like: -> · · Use: exports ? this · The do keyword inserts a closure wrap- Strings are immutable and can not be Mostly no need to use parentheses to spliced invoke a function if it is passed argu- per, forwards any arguments and in- vokes a passed function ments. The implicit call wraps forward Splats to the end of the line or block expres- sion. · Splats ... can be used instead of the Try/Catch/Finally Embedded JavaScript · Functions may have default values for variable number of arguments object arguments. Override the default value and are available for both function def- · try/catch statements are as in · Use backquotes `` to embed JavaScript by passing a non-null argument. inition and invocation JavaScript (although expressions) code within CoffeeScript 1 E. Hoigaard © 2554/2011 Rev. α 1 Everything is an Expression Existential Operator Destructuring Assignment · Multiple values, comma separated, can be given for each when clause. If any of · · Functions return their final value Use the existential operator ? to check if · To make extracting values from com- the values match, the clause runs · The return value is fetched from each a variable exists. ? returns true unless plex arrays and objects convenient, branch of execution a variable is null or undefined CoffeeScript implements destructuring · Use ?= for safer conditional assignment assignment String Interpolation, Heredocs, and Block Comments · Return early from a function body by than ||= with numbers or strings · When assigning an array or object lit- using an explicit return · Single-quoted strings are literal. Use · The accessor variant of the existential eral to a value, CoffeeScript breaks up · Variable declarations are at the top of backslash for escape characters operator ?. can be used to soak up null and matches both sides against each the scope, so assignment can be used · references in a chain of properties other, assigning the values on the right Double-quoted strings allow for inter- within expressions, even for variables polated values, using #{ ... } · Use ?. instead of the dot accessor . in to the variables on the left that have not been seen before · cases where the base value may be null · The simplest case is parallel assignment Multiline strings are allowed · Statements, when used as part of an ex- or undefined. If all of the properties ex- [a,b] = [b,a] · A heredoc ''' can be used for formatted pression, are converted into expressions ist then the expected result is returned, · It can be used with functions that return or indentation-sensitive text (or to avoid with a closure wrapper. This allows as- if the chain is broken, then undefined is escaping quotes and apostrophes) signment of the result of a comprehen- multiple values returned instead · sion to a variable · It can be used with any depth of array The indentation level that begins a here- · and object nesting to get deeply nested doc is maintained throughout, so the The following are not expressions: Classes, Inheritance, and Super text can be aligned with the body of the break, continue, and return properties and can be combined with splats code · Object orientation as in most other ob- · Double-quoted heredocs """ allow for ject oriented languages Operators and Aliases interpolation · The class structure allows to name the Function binding · Block comments ### are similar to · class, set the superclass with extends, CoffeeScript compiles == into ===, and heredocs, and are preserved in the gen- assign prototypal properties, and define · The fat arrow => can be used to define a != into !==. There is no equivalent to erated code the JavaScript == operator a constructor, in a single assignable function and bind it to the current value expression of this · The alias is is equivalent to ===, and · · isnt corresponds to !== Constructor functions are named as the This is helpful when using callback- Extended Regular Expressions class name, to support reflection based libraries, for creating iterator · Logical operator aliases: and is &&, or is · · Lower level operators: The extends functions to pass to each or event- Extended regular expressions are de- || and not is an alias for ! operator helps with proper prototype handler functions to use with bind limited by /// and are similar to here- · In while, if/else and switch/when docs and block comments. setup. :: gives access to an object’s · Functions created with => are able to ac- statements the then keyword can be prototype. super() calls the immediate cess properties of the this where they · They ignore internal whitespace and used to keep the body on the same line ancestor’s method of the same name are defined can contain comments · Alias for boolean true is on and yes (as · A class definition is a block of exe- in YAML) cutable code, which may be used for Aliases · Alias for boolean false is off and no meta programming. Switch/When/Else · · In the context of a class definition, For single-line statements, unless can · The switch statement do not need a and : && or : || not :! this is the class object itself (the be used as the inverse of if break after every case is : == isnt : != constructor function), so static · Use @property or @method instead of · properties can be assigned by using A switch is a returnable, assignable ex- yes : true no : false this.something @property: value, and functions de- pression on : true off : false · Use in to test for array presence fined in parent classes can be called · The format is: switch condition, when · Use of to test for object-key presence with: @inheritedMethodName() clauses, else the default case http://autotelicum.github.com/Smooth-CoffeeScript/ 2.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages2 Page
-
File Size-