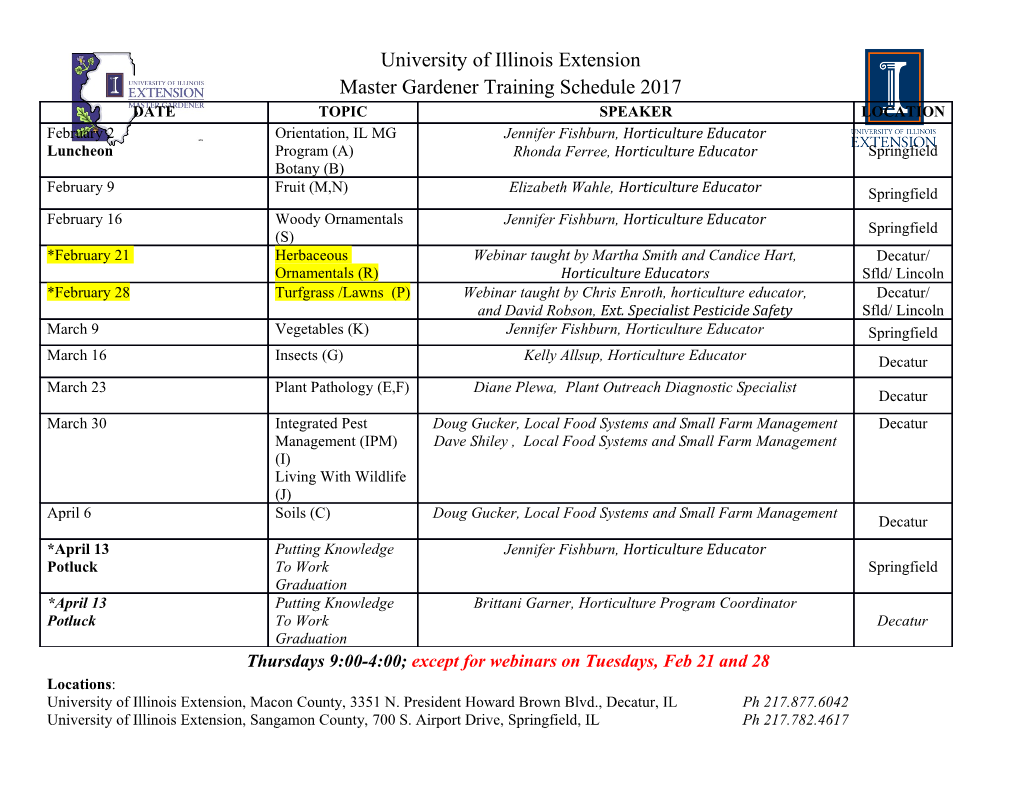
Institutionen för datavetenskap Department of Computer and Information Science Final thesis Designing and implementing an architecture for single-page applications in Javascript and HTML5 by Jesper Petersson LIU-IDA/LITH-EX-A--12/063--SE 2012-11-21 Linköpings universitet Linköpings universitet SE-581 83 Linköping, Sweden 581 83 Linköping Final thesis Designing and implementing an architecture for single-page applications in Javascript and HTML5 by Jesper Petersson LIU-IDA/LITH-EX-A--12/063--SE November 21, 2012 Supervisors: Johannes Edelstam (Valtech), Massimiliano Raciti (LiU) Examiner: Prof. Simin Nadjm-Tehrani Abstract A single-page application is a website that retrieves all needed components in one single page load. The intention is to get a user experience that reminds more of a native appli- cation rather than a website. Single-page applications written in Javascript are becoming more and more popular, but when the size of the applications grows the complexity is also increased. A good architecture or a suitable framework is therefore needed. The thesis begins by analyzing a number of design patterns suitable for applications containing a graphical user interface. Based on a composition of these design patterns, an architecture that targets single-page applications was designed. The architecture was designed to make applications easy to develop, test and maintain. Initial loading time, data synchronization and search engine optimizations were also important aspects that were considered. A framework based on the architecture was implemented, tested and compared against other frameworks available on the market. The framework that was implemented was designed to be modular, supports routing and templates as well as a number of different drivers for communicating with a server-side database. The modules were designed with a variant of the pattern Model-View-Controller (MVC), where a presentation model was introduced between the controller and the view. This allows unit tests to bypass the user interface and instead communicate directly with the core of the application. After minification and compression, the size of the framework is only 14.7 kB including all its dependencies. This results in a low initial loading time. Finally, a solution that allows a Javascript application to be indexed by a search engine is presented. It is based on PhantomJS in order to produce a static snapshot that can be served to the search engines. The solution is fast, scalable and easy to maintain. Acknowledgments First off I would like to thank my examiner Simin Nadjm-Tehrani and my supervisor Massimiliano Raciti for taking the time to examine and supervise me during my master thesis. Your feedback have been really helpful and encouraging. The master thesis was carried out at Valtech in Stockholm. It has been a real pleasure to be surrounded by professionals in diverse areas during the thesis, it has really helped me to look at the thesis from different angles. A special thanks goes to my supervisor and Javascript-guru Johannes Edelstam, I am really grateful for all the help and support! Glossary Term Description Asynchronous JavaScript and XML, a technique used to let clients AJAX asynchronously do API-requests after the page has been rendered. Application Programming Interface, an interface for a computer API program. Backbone.js A lightweight Javascript framework. Content Delivery Network, a network of servers that serves content CDN with high availability and performance. A program that browses the web, used by search engines to index Crawler websites. DNS Domain Name System, a naming system for computer services. Document Object Model, a tree representation of the objects on a DOM web page. DOM-element A node in the DOM-tree. DOM-selector A method of retrieving nodes from the DOM-tree. Ember.js A Javascript framework with two-way data-bindings. GET parameter A parameter sent as a GET request in HTTP. Hypertext Markup Language, a markup language used to describe HTML objects on a website Hypertext Transfer Protocol, an application protocol used to dis- HTTP tribute websites in a network. Hashbang A name for the character sequence #! Graphical User Interace, an interface that communicates with the GUI user using images rather than text. jQuery A popular Javascript library commonly used in web applications. JavaScript Object Notation, a text-based standard to format data JSON in a compact way. MySQL An open source relational database manager. A server-side scripting language commonly used for web develop- PHP ment. PhantomJS A headless Webkit browser. REpresentational State Transfer, a simple software architecture REST style for distributed systems. Commonly used for web services. Search-Engine Optimization, when optimizing a website with the SEO goal to get a higher page rank. Transmission Control Protocol, a reliable protocol for programs to TCP communicate with each other. Simple Object Access Protocol, a software architecture style for SOAP distributed systems. Single-Page Application, a web application that fits all content on SPA one single web page. SPI A synonym for SPA. A value that is evaluated as true in a logical context. In Javascript, Truthy any value except false, NaN, null, undefined, 0 and "". Uniform Resource Locator, a string that refers to an Internet re- URL source. Extensible Markup Language, a text-based standard to format XML 4 data. Contents 1Introduction 9 1.1 Background ................................ 9 1.2 Problem description ........................... 9 1.3 Goal .................................... 10 1.4 Approach ................................. 11 1.5 Scope and limitations .......................... 11 1.6 Thesis structure ............................. 12 2Technicalbackground 13 2.1 Single-page applications ......................... 13 2.2 URLs, routes and states ......................... 13 2.3 API communication ........................... 14 2.4 Templates ................................. 15 2.5 Data bindings ............................... 15 2.6 Testing a Javascript application ..................... 15 2.7 Existing frameworks ........................... 16 2.8 Search engine optimization ....................... 16 3Designpatterns 18 3.1 Modularity ................................ 18 3.2 Communication between modules .................... 20 3.3 MVC - Model-View-Controller ..................... 21 3.4 MVP - Model-View-Presenter ...................... 23 3.5 MVVM - Model-View-ViewModel .................... 23 4Architecture 24 4.1 System-level architecture ......................... 24 4.2 Application-level architecture ...................... 25 4.3 Module-level architecture ........................ 26 4.4 API communication ........................... 27 4.5 Initial loading time ............................ 28 4.6 Search engine optimization ....................... 28 5MinimaJSimplementation 31 5.1 Classes and inheritance ......................... 31 5.2 Scopes in Javascript ........................... 32 5.3 API drivers and push synchronization ................. 32 5.4 Dependencies ............................... 33 5.5 Javascript render API for SEO ..................... 33 5.6 Deploying a Javascript application ................... 34 6Evaluation 36 6.1 The test application ........................... 36 6.2 Measuring the initial loading time ................... 36 6.3 Measuring the testability ........................ 38 6.4 Multiple clients .............................. 40 6.5 Test of a SEO-friendly Javascript site ................. 40 5 7Discussion 42 7.1 Test-driven development in Javascript ................. 42 7.2 The importance of delays ........................ 42 7.3 When to use SPAs ............................ 42 7.4 Future work ................................ 43 List of Figures 2.1 The flow of communication between a browser and the web server. ..... 14 2.2 The flow of how a typical crawler communicates with an AJAX-based website. 17 3.1 Communication between the different parts of the MVC pattern. ...... 22 4.1 The architecture seen from a system-level point of view. ........... 25 4.2 The architecture seen from an application-level. ................ 25 4.3 An abstract view of a module/service in the application. ........... 27 4.4 The flow of communication when using an API for HTML snapshot generation. 30 5.1 An illustration of a client sending two changes to the server. ......... 33 6.1 The test application. ............................... 36 6.2 A graph showing the accumulated loading time in milliseconds of the test application comparing different implementations of it. ............ 37 6.3 The result after submitting the test-site to Google’s Crawler. ........ 41 List of Tables 6.1 The loading time of the test application in different implementations. .... 37 6.2 File size of a few Javascript frameworks after minification and compression. 38 6.3 A comparison between different frameworks when measuring the line cover- age after a unit test was run. .......................... 39 Chapter 1 Introduction The thesis was carried out as a final project at master level for a 5 year degree in Computer science and engineering at Linköping University. 1.1 Background For many years traditional web services have been built upon complex backend systems that serve static HTML files to the users. When the Web 2.0-era came around, techniques of dynamic content loading such as Asynchronous JavaScript and XML (AJAX) became popular. These techniques allow the application to fetch data from the server without reloading
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages48 Page
-
File Size-