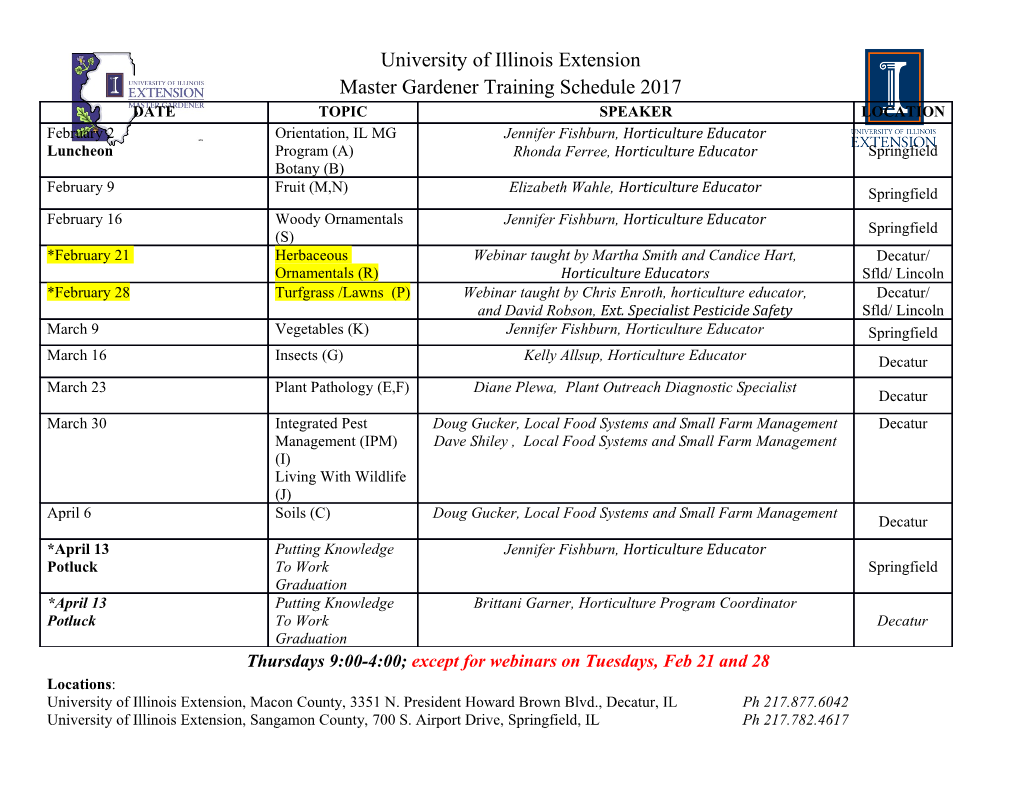
python edition 2D Game Development: From Zero to Hero A compendium of the community knowledge on game design and development 2D Game Development: From Zero To Hero (python edition, version v0.5.6) is licensed under the CC-BY-NC 4.0 license. This book can be found in the following official repositories: • https://github.com/Penaz91/2DGD_F0TH/ • https://gitlab.com/Penaz/2dgd_f0th Perseverance is the backbone of success. Anonymous To my family To my friends, both international and not To whom never gives up Daniele Penazzo 2D Game Development: From Zero To Hero Contents 1 Foreword 1 2 Introduction 2 2.1 Why another game development book?...............................2 2.2 Conventions used in this book....................................2 2.2.1 Logic Conventions......................................2 2.2.2 Code Listings.........................................3 2.2.3 Block Quotes.........................................3 2.3 Structure of this Book........................................3 3 The Maths Behind Game Development5 3.1 The modulo operator.........................................5 3.2 Vectors................................................5 3.2.1 Adding and Subtracting Vectors..............................5 3.2.2 Scaling Vectors........................................6 3.2.3 Dot Product.........................................7 3.2.4 Vector Length and Normalization..............................7 3.3 Matrices................................................8 3.3.1 What is a matrix.......................................8 3.3.2 Matrix sum and subtraction.................................8 3.3.3 Multiplication by a scalar..................................8 3.3.4 Transposition.........................................8 3.3.5 Multiplication between matrices..............................9 3.3.6 Other uses for matrices................................... 10 3.4 Trigonometry............................................. 10 3.4.1 Radians vs Degrees...................................... 11 3.4.2 Sine, Cosine and Tangent.................................. 11 3.4.3 Pythagorean Trigonometric Identity............................ 12 3.4.4 Reflections.......................................... 12 3.4.5 Shifts............................................. 12 3.4.6 Trigonometric Addition and subtraction.......................... 13 3.4.7 Double-Angle Formulae................................... 13 3.4.8 Inverse Formulas....................................... 13 3.5 Coordinate Systems on computers................................. 14 3.6 Transformation Matrices....................................... 15 3.6.1 Stretching........................................... 15 3.6.2 Rotation............................................ 16 3.6.2.1 Choosing the direction of the rotation...................... 16 3.6.2.2 Rotating referred to an arbitrary point..................... 16 3.6.3 Shearing............................................ 16 4 Some Computer Science Fundamentals 18 4.1 De Morgan’s Laws and Conditional Expressions.......................... 18 4.2 Estimating the order of algorithms................................. 18 4.2.1 O(1).............................................. 19 4.2.2 O(log(n))........................................... 19 4.2.3 O(n).............................................. 19 4.2.4 O(n·log(n))......................................... 20 4.2.5 O(n2)............................................. 20 4.2.6 O(2n)............................................. 20 4.3 A primer on calculating the order of your algorithms....................... 21 CONTENTS III 2D Game Development: From Zero To Hero 4.3.1 Some basics.......................................... 21 4.3.2 What happens when we have more than one big-O?................... 22 4.3.3 What do we do with recursive algorithms?......................... 22 4.3.4 How do big-O estimates compare to each other?..................... 23 4.4 Simplifying your conditionals with Karnaugh Maps........................ 24 4.4.1 “Don’t care”s......................................... 24 4.4.2 A more complex map.................................... 26 4.4.3 Guided Exercise....................................... 27 4.5 Object Oriented Programming................................... 28 4.5.1 Introduction......................................... 28 4.5.2 Objects............................................ 28 4.5.3 Abstraction and Interfaces.................................. 29 4.5.4 Inheritance and Polymorphism............................... 29 4.5.5 The Diamond Problem.................................... 29 4.5.6 Composition......................................... 30 4.5.7 Coupling........................................... 30 4.5.8 The DRY Principle...................................... 30 4.5.9 SOLID Principles....................................... 31 4.5.10 “Composition over Inheritance” design........................... 31 4.6 Designing entities as data...................................... 31 4.7 Reading UML diagrams....................................... 32 4.7.1 Use Case Diagrams...................................... 32 4.7.1.1 Actors........................................ 33 4.7.1.2 Use Cases...................................... 33 4.7.1.3 Notes........................................ 35 4.7.1.4 Sub-Use Cases................................... 35 4.7.2 Class Diagrams........................................ 35 4.7.2.1 Classes....................................... 35 4.7.2.2 Relationships between classes........................... 36 4.7.2.3 Notes........................................ 38 4.7.2.4 Interfaces...................................... 38 4.7.3 Activity Diagrams...................................... 39 4.7.3.1 Start and End Nodes............................... 39 4.7.3.2 Actions....................................... 39 4.7.3.3 Decisions (Conditionals) and loops........................ 40 4.7.3.4 Synchronization.................................. 41 4.7.3.5 Signals....................................... 41 4.7.3.6 Swimlanes..................................... 42 4.7.3.7 Notes........................................ 42 4.7.3.8 A note on activity diagrams........................... 43 4.8 Generic Programming........................................ 43 4.9 Advanced Containers......................................... 43 4.9.1 Dynamic Arrays....................................... 44 4.9.1.1 Performance Analysis............................... 44 4.9.2 Linked Lists.......................................... 46 4.9.2.1 Performance Analysis............................... 46 4.9.3 Doubly-Linked Lists..................................... 48 4.9.4 Hash Tables.......................................... 48 4.9.5 Binary Search Trees (BST)................................. 50 4.9.6 Heaps............................................. 51 4.9.7 Stacks............................................. 51 4.9.8 Queues............................................ 52 CONTENTS IV 2D Game Development: From Zero To Hero 4.9.9 Circular Queues....................................... 53 4.10 Introduction to MultiTasking.................................... 54 4.10.1 Co-Routines.......................................... 54 4.11 Introduction to MultiThreading................................... 54 4.11.1 What is MultiThreading................................... 54 4.11.2 Why MultiThreading?.................................... 55 4.11.3 Thread Safety......................................... 55 4.11.3.1 Race conditions.................................. 55 4.11.3.2 Critical Regions.................................. 57 4.11.4 Ensuring determinism.................................... 57 4.11.4.1 Immutable Objects................................ 57 4.11.4.2 Mutex........................................ 58 4.11.4.3 Atomic Operations................................. 58 5 Project Management Basics and tips 59 5.1 The figures of game design and development............................ 59 5.1.1 Producer/Project Manager................................. 59 5.1.2 Game Designer........................................ 59 5.1.3 Writer............................................. 59 5.1.4 Developer........................................... 59 5.1.5 Visual Artist......................................... 60 5.1.6 Sound Artist......................................... 60 5.1.7 Tester............................................. 60 5.2 Some generic tips........................................... 60 5.2.1 Be careful of feature creep.................................. 60 5.2.2 On project duration..................................... 60 5.2.3 Brainstorming: the good, the bad and the ugly...................... 61 5.2.4 On Sequels.......................................... 61 5.3 Common Errors and Pitfalls..................................... 61 5.3.1 Losing motivation...................................... 61 5.3.2 The “Side Project” pitfall.................................. 61 5.3.3 Making a game “in isolation”................................ 62 5.3.4 Mishandling Criticism.................................... 62 5.3.5 Not letting others test your game.............................. 62 5.3.6 Being perfectionist...................................... 62 5.3.7 Using the wrong engine................................... 63 5.4 Software Life Cycle Models..................................... 63 5.4.1 Iteration versus Increment.................................. 63 5.4.2 Waterfall Model....................................... 63 5.4.3 Incremental Model.....................................
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages262 Page
-
File Size-