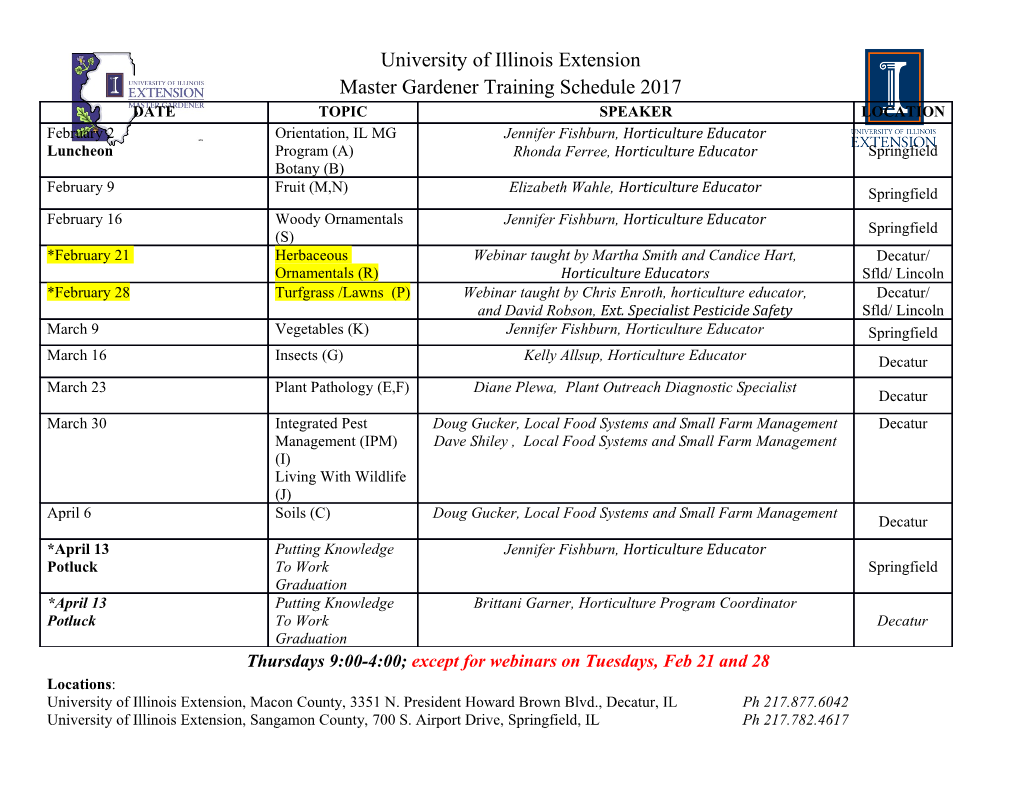
Solving Combinatorial Search Problems Using B-Prolog Neng-Fa Zhou 周 能法 The City University of New York [email protected] by Neng-Fa Zhou at Kyutech 1 B-Prolog: Prolog + Tabling + CLP(FD) Prolog – Rule-based relational language • SQL + Recursion + Unification + Backtracking Tabling – Memorize and reuse intermediate results • Suitable for dynamic programming problems CLP(FD) – Constraint Logic Programming over Finite Domains • Suitable for constraint satisfaction problems (NP-complete) by Neng-Fa Zhou at Kyutech 2 Prolog A program consists of relations defined by facts and rules Unification Recursion Nondeterminism realized through backtracking by Neng-Fa Zhou at Kyutech 3 Prolog – An example app([],Ys,Ys). app([X|Xs],Ys,[X|Zs]):- app(Xs,Ys,Zs). by Neng-Fa Zhou at Kyutech 4 Syntax of Prolog Term • Atom – string of letters, digits, and '_' starting with a low-case letter – string of characters enclosed in quotes • Number – integer & real • Variable – string of letters, digits and '_' starting with a capital letter or '_' by Neng-Fa Zhou at Kyutech 5 Syntax of Prolog (Cont) • Structure – f(t1,t2,...,tn) » f is an atom, called the functor of the structure » t1,t2,...,tn are terms • List – '.'(H,T) => [H|T] – '.'(1,'.'(2,'.'(3,[]))) => [1,2,3] by Neng-Fa Zhou at Kyutech 6 Syntax of Prolog (Cont) Clause • Fact – p(t1,t2,...,tn) Head • Rule Body – H :- B1,B2,...,Bm. Predicate • a sequence of clauses Program • a set of predicates Query by Neng-Fa Zhou at Kyutech 7 Unification t1 = t2 succeeds if – t1 and t2 are identical – there exists a substitution q for the variables in t1 and t2 such that t1q = t2q. f(X,b)=f(a,Y). X=a Y=b q = {X/a, Y/b} by Neng-Fa Zhou at Kyutech 8 Unification: Examples ?-X=1. assignment X=1 ?- f(a,b)=f(a,b). test yes ?- a=b. test no ?- f(X,Y)=f(a,b) matching X=a Y=b ?-f(X,b)=f(a,Y). unification X=a Y=b ?-X = f(X). without occur checking X=f(f(...... by Neng-Fa Zhou at Kyutech 9 Operational Semantics of Prolog (Resolution) G0: initial query Gi: (A1,A2,...,An) H:-B1,...,Bm A1q=Hq Gi+1: (B1,...,Bm,A2,...,An)q Succeed if Gk is empty for some k. Backtrack if Gk is a dead end (no clause can be used). by Neng-Fa Zhou at Kyutech 10 Deductive Database parent(Parent,Child):-father(Parent,Child). parent(Parent,Child):-mother(Parent,Child). uncle(Uncle,Person) :- brother(Uncle,Parent), parent(Parent,Person). sibling(Sib1,Sib2) :- parent(Parent,Sib1), parent(Parent,Sib2), Sib1 ¥= Sib2. cousin(Cousin1,Cousin2) :- parent(Parent1,Cousin1), parent(Parent2,Cousin2), sibling(Parent1,Parent2). by Neng-Fa Zhou at Kyutech 11 Exercises Define the following relations – son(X,Y) -- X is a son of Y – daughter(X,Y) -- X is a daughter of Y – grandfather(X,Y) -- X is the grandfather of Y – grandparent(X,Y) -- X is a grandparent of Y – ancestor(X,Y) – X is an ancestor of Y by Neng-Fa Zhou at Kyutech 12 Recursive Programming on Lists A list is a special structure whose functor is '.'/2 – [] – '.'(H,T) => [H|T] – '.'(1,'.'(2,'.'(3,[]))) => [1,2,3] Unification of lists – [X|Xs]=[1,2,3] X= 1 Xs=[2,3] – [1,2,3] = [1|[2|X]] X=[3] – [1,2|3] = [1|X] X=[2|3] by Neng-Fa Zhou at Kyutech 13 Relations on Lists isList(Xs) isList([]). isList([X|Xs]):-isList(Xs). member(X,Xs) member(X,[X|Xs]). member(X,[_|Xs]):-member(X,Xs). append(Xs,Ys,Zs) append([],Ys,Ys). append([X|Xs],Ys,[X|Zs]):-append(Xs,Ys,Zs). length(Xs,N) length([],0). length([X|Xs],N):by Neng--Falength(Xs,N1),N Zhou at Kyutech is N1+1. 14 Exercise Implement the – reverse(Xs,Ys) following predicates. • Ys is the reverse of Xs – sum(Xs,N) – length(Xs,N) • N is the sum of the • the length of Xs is N integers in the list Xs – last(X,Xs) – sum1(Xs,Ys) • assume Xs is • X is the last element of [x1,x2,...,xn], then Ys will Xs. be [y1,y2,...,yn] where yi – prefix(Pre,Xs) is xi+1. • Pre is a prefix of Xs. – sort(L,SortedL) • use the exchange sort – suffix(Pos,Xs) algorithm • suffix is a postfix of Xs by Neng-Fa Zhou at Kyutech 15 Recursive Programming on Binary Trees Representation of binary trees void -- empty tree t(N, L,R) -- N : node L : Left child R : Right child Example a t(a, t(b, void,void), t(c,void,void)) b c by Neng-Fa Zhou at Kyutech 16 Relations on Binary Trees isBinaryTree(T)-- T is a binary tree isBinaryTree(void). isBinaryTree(t(N,L,R)):- isBinaryTree(L), isBinaryTree(R). count(T,C) -- C is the number of nodes in T. count(void,0). count(t(N,L,R),N):- count(L,N1), count(R,N2), N is N1+N2+1. by Neng-Fa Zhou at Kyutech 17 Relations on Binary Trees (Cont.) preorder(T,L) • L is a pre-order traversal of the binary tree T. preorder(void,[]). preorder(t(N,Left,Right),L):- preorder(Left,L1), preorder(Right,L2), append([N|L1],L2,L). by Neng-Fa Zhou at Kyutech 18 Exercise Write the following predicates on binary trees. – leaves(T,L): L is the list of leaves in T. The order is preserved. – equal(T1,T2): T1 and T2 are the same tree. – postorder(T,L): L is the post-order traversal of T. by Neng-Fa Zhou at Kyutech 19 Tabling (Why?) Eliminate infinite loops :-table path/2. path(X,Y):-edge(X,Y). path(X,Y):-edge(X,Z),path(Z,Y). Reduce redundant computations :-table fib/2. fib(0,1). fib(1,1). fib(N,F):- N>1, N1 is N-1,fib(N1,F1), N2 is N-2,fib(N2,F2), F is F1+F2. by Neng-Fa Zhou at Kyutech 20 Mode-Directed Tabling Table mode declaration :-table p(M1,...,Mn):C. – C: Cardinality limit – Modes • + : input • - : output • min: minimized • max: maximized by Neng-Fa Zhou at Kyutech 21 Shortest Path Problem :-table sp(+,+,-,min). sp(X,Y,[(X,Y)],W) :- edge(X,Y,W). sp(X,Y,[(X,Z)|Path],W) :- edge(X,Z,W1), sp(Z,Y,Path,W2), W is W1+W2. sp(X,Y,P,W) – P is a shortest path between X and Y with weight W. by Neng-Fa Zhou at Kyutech 22 Knapsack Problem http://probp.com/examples/tabling/knapsack.pl :- table knapsack(+,+,-,max). knapsack(_,0,[],0). knapsack([_|L],K,Selected,V) :- knapsack(L,K,Selected,V). knapsack([F|L],K,[F|Selected],V) :- K1 is K - F, K1 >= 0, knapsack(L,K1,Selected,V1), V is V1 + 1. knapsack(L,K,Selected,V) – L: the list of items – K: the total capacity – Selected: the list of selected items – V: the length of Selected by Neng-Fa Zhou at Kyutech 23 Exercises (Dynamic Programming) 1. Maximum Value Contiguous Subsequence. Given a sequence of n real numbers a1, ... an, determine a contiguous subsequence Ai ... Aj for which the sum of elements in the subsequence is maximized. 2. Given two text strings A of length n and B of length m, you want to transform A into B with a minimum number of operations of the following types: delete a character from A, insert a character into A, or change some character in A into a new character. The minimal number of such operations required to transform A into B is called the edit distance between A and B. by Neng-Fa Zhou at Kyutech 24 CLP(FD) by Example (I) The rabbit and chicken problem The Kakuro puzzle The knapsack problem Exercises by Neng-Fa Zhou at Kyutech 25 The Rabbit and Chicken Problem In a farmyard, there are only chickens and rabbits. Its is known that there are 18 heads and 58 feet. How many chickens and rabbits are there? go:- [X,Y] :: 1..18, X+Y #= 18, 2*X+4*Y #= 58, labeling([X,Y]), writeln([X,Y]). by Neng-Fa Zhou at Kyutech 26 Break the Code Down go -- a predicate X,Y -- variables go:- 1..58 -- a domain [X,Y] :: 1..58, X :: D -- a domain declaration X+Y #= 18, E1 #= E2 -- equation (or 2*X+4*Y #= 58, equality constraint) labeling([X,Y]), writeln([X,Y]). labeling(Vars)-- find a valuation for variables that satisfies the constraints writeln(T) -- a Prolog built- in by Neng-Fa Zhou at Kyutech 27 Running the Program | ?- cl(rabbit) Compiling::rabbit.pl compiled in 0 milliseconds loading::rabbit.out yes | ?- go [7,11] by Neng-Fa Zhou at Kyutech 28 The Kakuro Puzzle Kakuro, another puzzle originated in Japan after Sudoku, is a mathematical version of a crossword puzzle that uses sums of digits instead of words. The objective of Kakuro is to fill in the white squares with digits such that each down and across “word” has the given sum. No digit can be used more than once in each “word”. by Neng-Fa Zhou at Kyutech 29 An Example go:- Vars=[X1,X2,…,X16], X1 X2 Vars :: 1..9, word([X1,X2],5), X3 X4 X5 X6 word([X3,X4,X5,X6],17), … X7 X8 X9 X10 word([X10,X14],3), labeling(Vars), X11 X12 X13 X14 writeln(Vars). X15 X16 word(L,Sum):- A Kakuro puzzle sum(L) #= Sum, all_different(L). by Neng-Fa Zhou at Kyutech 30 Break the Code Down sum(L) #= Sum The sum of the elements in L makes Sum.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages72 Page
-
File Size-