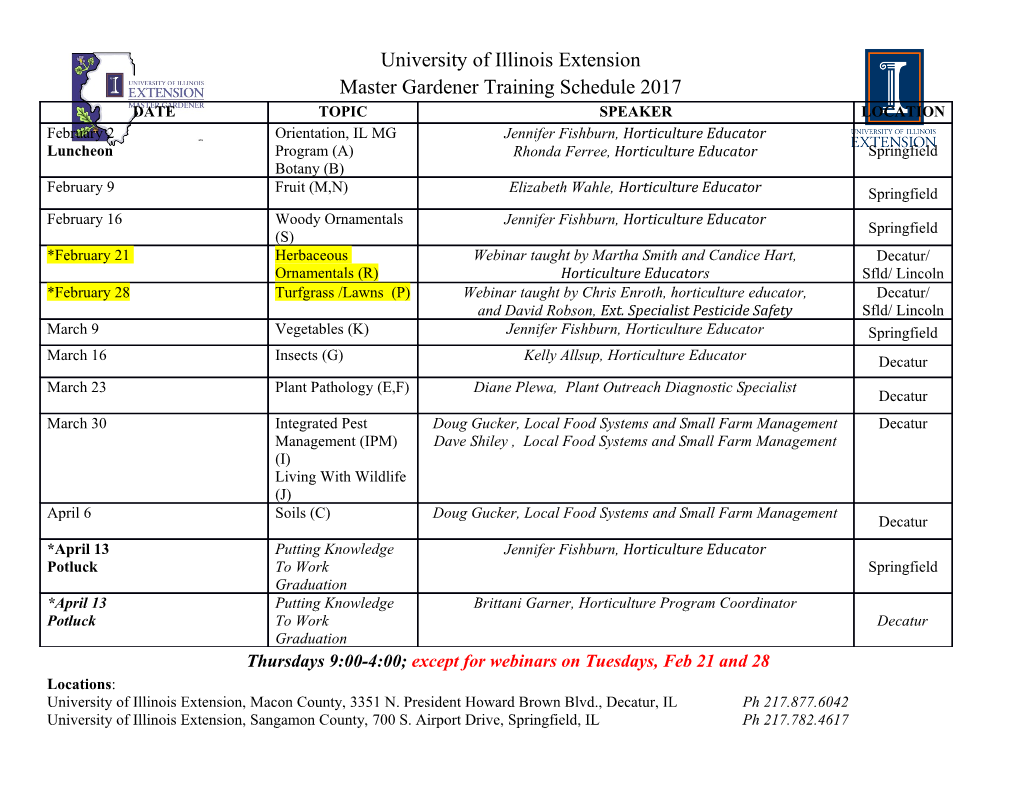
Introduction to Object-Oriented Design Patterns [email protected] – 2016 Sorting 1: String Sorting 2 jonkv@ida First: An illustrating problem Suppose you need to sort some strings Implement sorting algorithms public class StringSorter { public void quicksort(String[] strings) { … } public void mergesort(String[] strings) { … } } Use the algorithms String[] strings = new String[numberOfStrings]; … StringSorter mySorter = new StringSorter(); mySorter.quicksort(strings); Sorting 2: String Comparisons 3 jonkv@ida A sorting algorithm must be able to compare two elements Obvious: Comparison inside the sort method public class StringSorter { public void quicksort(String[] array) { … // Should element #i be before element #j? if (array[i].compareTo(array[j]) < 0) { … } } } String has a built-in compareTo method Returns -1, 0 or 1: str1 should be before, equal to, or after str2 Sorting 3: Different Sort Orders 4 jonkv@ida And sometimes you need an inverse sort order Obvious solution: Add a flag public class StringSorter { private boolean inverseOrder; public StringSorter(boolean inverse) { this.inverseOrder = inverse; } public void quicksort(String[] strings) { … // Should element #i be before element #j? if ( !inverseOrder && array[i].compareTo(array[j]) < 0 || inverseOrder && array[i].compareTo(array[j]) > 0) { … } } Different comparisons } depending on standard or inverse order Sorting 4: Additional Sort Orders 5 jonkv@ida You sometimes use different locales . Danish: …xyzäöå . Swedish: …xyzåäö . English: aåäbcde… . Add a locale specifier to the StringSorter Someone wants to sort strings representing dates . In day/month/year format . Add a new flag indicating date sorting should be used … Sorting 5: Lack of Flexibility 6 jonkv@ida So what’s the problem? Every time we want a new sorting criterion, the sort method must be changed can't be in a class library Having many sorting criteria leads to long and complex conditional tests A pure sorting algorithm should not have knowledge about the type of data being sorted Inflexible! Callbacks using Higher-Order Functions 8 jonkv@ida One general approach: Higher-order functions Python example from TDDD73 def combine(func,seq): Takes another function result = seq[0] as parameter for element in seq[1:]: result = func(result,element) return result String sorting example void quicksort(String[] strings, function compare) { Takes a comparison function … as a parameter // Should element #i be before element #j? if (compare(strings[i], strings[j]) < 0) { … Call this callback function } to determine element order! } Many OO languages do not support this! How do we find a pure OO solution? Callbacks using Objects 9 jonkv@ida OO approach: Let an object compare the elements for us public interface StringComparator { Returns -1, 0 or 1: str1 should be int compare(String str1, String str2); before, equal to, or after str2 } public class StringSorter { private final StringComparator comp; public StringSorter(StringComparator comp) { this.comp = comp; } Specify a comparator public void quicksort(String[] strings) { when the sorter is created … // Should element #i be before element #j? if (comp.compare(strings[i], strings[j]) < 0) { … } } } Call the specified comparator's method This is an object-oriented way of implementing callbacks! Implementing Comparators 10 jonkv@ida Now you can implement as many comparators as needed! . No need to change the StringSorter – reuse it for arbitrary sort orders public class LengthComparator implements StringComparator { public int compare(String str1, String str2) { int len1 = str1.length(); int len2 = str2.length(); if (len1 > len2) return 1; else if (len1 < len2) return -1; else return 0; } } Using Comparators 11 jonkv@ida StringComparator lengthcomp= new LengthComparator(); StringSorter sorter = new StringSorter(lengthcomp); sorter.quicksort(strings); sorter … comp.compare() … StringSorter class Object The sorter (data) sort() [code] is told which header: … [code] comparator comp … [code] to use … … LengthComparator class Object The comparator (data) compare() [code] may have no fields / data, header: … [code] but it has methods … [code] that the sorter can call Java Collections Framework: Comparator 12 jonkv@ida The Collections Framework has a generic comparator interface public interface Comparator<T> { int compare(T obj1, T obj2); } public class Sorter<T> { private final Comparator<T> comp; public Sorter(Comparator<T> comp) { this.comp = comp; } public void quicksort(List<T> list) { // ... // Should element #i be before element #j? if (comp.compare(list.get(i), list.get(j)) < 0) { /* ... */} } } Also, built-in sort method: Collections.sort(students, new NameLengthComparator()); Generalization 1: Similar Problems 14 jonkv@ida Similar problems and solutions arise in many places . Any JComponent in the Java GUI can have a border ▪ Not a built-in repertoire of borders ▪ Instead, callback objects implementing the Border interface ▪ Change border: component.setBorder(Border b) ▪ Can easily define a new Border, with arbitrary design Generalization 2: Similar Problems 15 jonkv@ida . Any Container in the Java GUI can lay out its subcomponents ▪ Set a layout callback object using component.setLayout() ▪ Uses a LayoutManager interface with concrete managers FlowLayout, BorderLayout, … ▪ Can easily define a new LayoutManager . A text component might need input validation ▪ JTextField supports an InputVerifier interface ▪ You can provide Numeric, AlphaNumeric, TelNumber verifier classes . … Generalization 3: A Pattern 16 jonkv@ida There is a certain pattern in the design of these classes! Class General interface that needs to be parameterized for parametrization StringSorter – which sort order? StringSorter – StringComparator JComponent – which border? JComponent – Border Container – which layout? Container – LayoutManager JTextField – which input pattern? JTextField – InputVerifier This parametrization requires Concrete Concrete implementation code, not just values! Concrete implementation Concrete implementation implementation Strategy pattern: A comparator object specifies which comparison strategy to use, a border object specifies which border painting strategy to use, … One Use of Strategy: String Sorting 17 jonkv@ida . StringSorter + Comparator shows one way of using a Strategy StringComparator StringSorter <<interface>> comp: Comparator ”Arrow with diamond” quicksort(String[] array) compare(String first, means aggregation: mergesort(String[] array) String second) Any StringSorter has a Comparator DefaultOrder InverseOrder DateOrder compare(String first, compare(String first, compare(String first, String second) String second) String second) Separation of concerns: StringSorter knows nothing about specific sort orders One Use of Strategy: Border Drawing 18 jonkv@ida . JComponent + Border shows another way of using a Strategy JComponent Border <<interface>> bord: Border paint() paintBorder(Graphics g) LineBorder EtchedBorder ThickBorder paintBorder(Graphics g) paintBorder(Graphics g) paintBorder(Graphics g) Separation of concerns: JComponent knows nothing about specific border types The General Strategy Pattern 19 jonkv@ida We can lift out a general design pattern that can be reused! Context Strategy<<interface>> Standardized terminology for this pattern type: ContextInterface() AlgorithmInterface() Context, Strategy, ConcreteStrategyX ConcreteStrategyA ConcreteStrategyB AlgorithmInterface() AlgorithmInterface() Patterns – in general 21 jonkv@ida "Pattern" has many meanings . Some relevant in this context: ▪ 5. a combination of qualities, acts, tendencies, etc., forming a consistent or characteristic arrangement – Dictionary.com ▪ 10: a discernible coherent system based on the intended interrelationship of component parts <foreign policy patterns> – Merriam-Webster Design Patterns 22 jonkv@ida Originates in architecture! . Christopher Alexander et al. (1977) state that a pattern: ▪ describes a problem which occurs over and over again in our environment, and then describes the core of the solution to that problem, in such a way that you can use this solution a million times over, without ever doing it the same way twice. Design Patterns and Classes 23 jonkv@ida Object-oriented design patterns: . How do we arrange our classes to solve a particular type of problem? ▪ Which interfaces and classes to create? ▪ Who knows about whom? ▪ Who inherits from whom? ▪ How do they call each other? ▪ … Creational Structural Behavioral Abstract factory Adapter Chain of responsibility Builder Bridge Command Factory method Composite Interpreter Prototype Decorator Iterator Singleton Facade Mediator Flyweight Memento Proxy Observer State Strategy Template Visitor Object-Oriented Design Patterns 24 jonkv@ida A typical object-oriented design pattern: . Describes a general way of organizing your classes, objects, methods to solve a kind of problem Context Strategy<<interface>> ContextInterface() AlgorithmInterface() ConcreteStrategyA ConcreteStrategyB AlgorithmInterface() AlgorithmInterface() . Can be quite different from how the problem would be solved in a non-OO language Object-Oriented Design Patterns (2) 25 jonkv@ida A typical object-oriented design pattern: . Can be quite different from void how the problem would be quicksort(String[] strings, function compare) { solved in a non-OO language … // Should element #i be before element #j? if (compare(strings[i], strings[j]) < 0) { … } } . Gives you a repertoire of techniques that you might not have thought
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages69 Page
-
File Size-