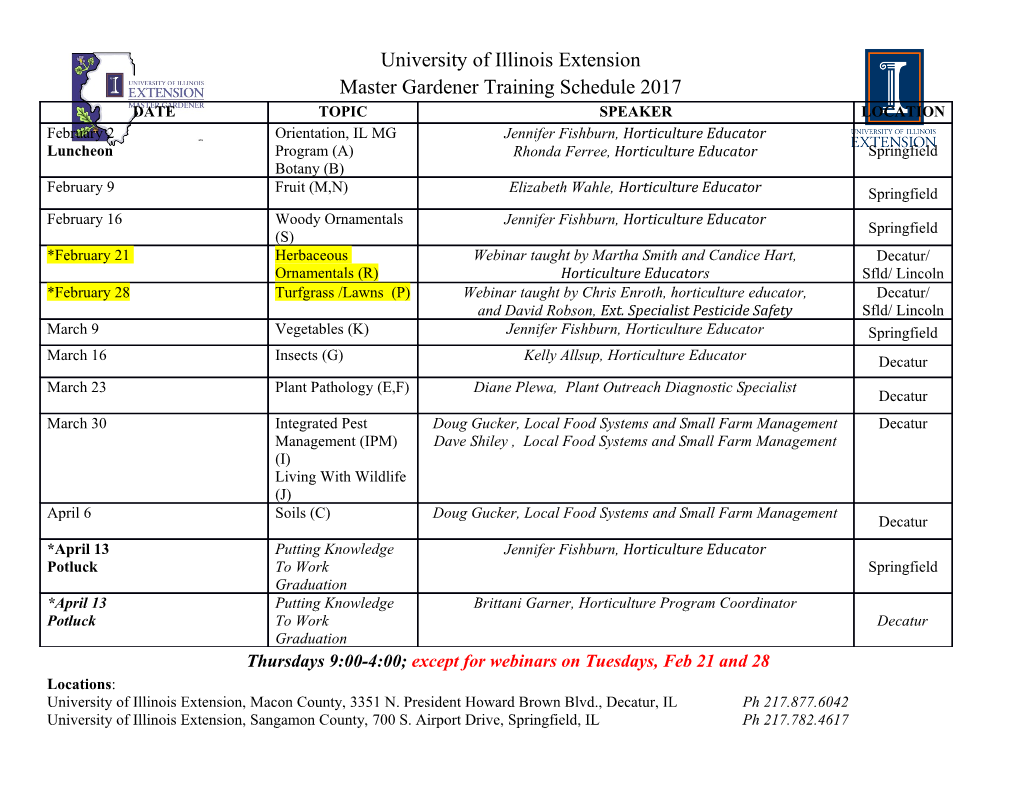
AN953 Data Encryption Routines for the PIC18 Author: David Flowers ENCRYPTION MODULE OVERVIEW Microchip Technology Inc. • Four algorithms to choose from, each with their own benefits INTRODUCTION • Advanced Encryption Standard (AES) This Application Note covers four encryption - Modules available in C, Assembly and algorithms: AES, XTEA, SKIPJACK® and a simple Assembly written for C encryption algorithm using a pseudo-random binary - Allows user to decide to include encoder, sequence generator. The science of cryptography decoder or both dates back to ancient Egypt. In today’s era of informa- - Allows user to pre-program a decryption key tion technology where data is widely accessible, into the code or use a function to calculate sensitive material, especially electronic data, needs to the decryption key be encrypted for the user’s protection. For example, a • Tiny Encryption Algorithm version 2 (XTEA) network-based card entry door system that logs the persons who have entered the building may be suscep- - Modules available in C and Assembly tible to an attack where the user information can be - Programmable number of iteration cycles stolen or manipulated by sniffing or spoofing the link • SKIPJACK between the processor and the memory storage - Module available in C device. If the information is encrypted first, it has a • Pseudo-random binary sequence generator XOR better chance of remaining secure. Many encryption encryption algorithms provide protection against someone reading - Modules available in C and Assembly the hidden data, as well as providing protection against tampering. In most algorithms, the decryption process - Allows user to change the feedback taps at will cause the entire block of information to be run-time destroyed if there is a single bit error in the block prior - KeyJump breaks the regular cycle of the to to decryption. increase the variability of the sequence • Out-of-the-box support for MPLAB® C 18 • Various compiling options to customize routines for a specific application - Available as a Microchip Application Maestro™ module to simplify customization 2005 Microchip Technology Inc. DS00953A-page 1 AN953 AES Overview/History/Background The Advanced Encryption Standard (AES) is a means of encrypting and decrypting data adopted by the National Institute of Standards and Technology (NIST) on October 2, 2000. In the late 1990s, NIST held a contest to initiate the development of encryption algorithms that would replace the Data Encryption Standard (DES). The contest tested the algorithms’ security and execution speed to determine which would be named the AES. The algorithm chosen is called the “Rijndael” algorithm after its two designers, Joan Daemen and Vincent Rijmen of Belgium. AES is a symmetric block cipher that utilizes a secret key to encrypt data. The implementation of AES in this application note is based on a 16-byte block of data and a 16-byte key size. DS00953A-page 2 2005 Microchip Technology Inc. AN953 ENCRYPTION FIGURE 1: AES ENCRYPT FLOWCHART ENCRYPTION Round Counter = 10 Rcon = 1 Key Addition S-Table Substitution Encode Row Shift True Round Counter = 1? False Encode Mix Column Encode Key Schedule Key Addition Round Counter - 1 Round Counter = 0? False True END 2005 Microchip Technology Inc. DS00953A-page 3 AN953 There are five basic subdivisions of the encryption flowchart. A detailed explanation of each will follow. The number of rounds needed in the transformation is taken from the following table. 16-Byte 24-Byte 32-Byte Block Block Block 16-byte key 10* 12 14 24-byte key 12 12 14 32-byte key 14 14 14 * Used in this implementation. This implementation of AES uses a 16-byte block and a 16-byte key and thus uses 10 rounds of encryption. On the last encryption round, the mix column subdivision is left out. The structures of the key and data blocks are shown below. TABLE 1: KEY MATRIX: Key [0] Key [4] Key [8] Key [12] Key [16] Key [20] Key [24] Key [28] Key [1] Key [5] Key [9] Key [13] Key [17] Key [21] Key [25] Key [29] Key [2] Key [6] Key [10] Key [14] Key [18] Key [22] Key [26] Key [30] Key [3] Key [7] Key [11] Key [15] Key [19] Key [23] Key [27] Key [31] TABLE 2: DATA MATRIX: Data [0] Data [4] Data [8] Data [12] Data [16] Data [20] Data [24] Data [28] Data [1] Data [5] Data [9] Data [13] Data [17] Data [21] Data [25] Data [29] Data [2] Data [6] Data [10] Data [14] Data [18] Data [22] Data [26] Data [30] Data [3] Data [7] Data [11] Data [15] Data [19] Data [23] Data [27] Data [31] To fit into the data matrix structure, the plain text to be encrypted needs to be broken into the appropriate size blocks, with any leftover space being padded. For example, take the following quote from “A Tale of Two Cities”, by Charles Dickens. “It was the best of times, it was the worst of times, …”. Broken into 16-byte blocks, the data would now look similar to this: EXAMPLE 1: PLAIN TEXT DIVIDED INTO 16-BYTE BLOCKS It was the best of times, it was the worst of ti mes, ... tuvwxyz(1) Note 1: If any of the blocks do not fit into the correct size matrix, the values must be padded at the end of the block. In this case, “tuvwxyz” is added to the end of the quote to complete the last block. Finally a key must be selected that is 128-bits long. For all of the examples in this document, the key will be [Charles Dickens]. With a key selected and the data sectioned off into appropriate size blocks, the encryption cycle may now begin. DS00953A-page 4 2005 Microchip Technology Inc. AN953 S-Table (Encryption Substitution Table): S-Table Substitution is a direct table lookup and replacement of the data. Below is the C code for this procedure: for(i=0;i<BLOCKSIZE;i++) { block[i]=STable[block[i]]; } The values of the table are defined in the following chart. TABLE 3: S-BOX OR ENCRYPTION SUBSTITUTION TABLE (VALUES IN HEXADECIMAL) y 00 10 20 30 40 50 60 70 80 90 A0 B0 C0 D0 E0 F0 00 63 7C 77 7B F2 6B 6F C5 30 01 67 2B FE D7 AB 76 01 CA 82 C9 7D FA 59 47 F0 AD D4 A2 AF 9C A4 72 C0 02 B7 FD 93 26 36 3F F7 CC 34 A5 E5 F1 71 D8 31 15 03 04 C7 23 C3 18 96 05 9A 07 12 80 E2 EB 27 B2 75 04 09 83 2C 1A 1B 6E 5A A0 52 3B D6 B3 29 E3 2F 84 05 53 D1 00 ED 20 FC B1 5B 6A CB BE 39 4A 4C 58 CF x 06 D0 EF AA FB 43 4D 33 85 45 F9 02 7F 50 3C 9F A8 07 51 A3 40 8F 92 9D 38 F5 BC B6 DA 21 10 FF F3 D2 08 CD 0C 13 EC 5F 97 44 17 C4 A7 7E 3D 64 5D 19 73 09 60 81 4F DC 22 2A 90 88 46 EE B8 14 DE 5E 0B DB 0A E0 32 3A 0A 49 06 24 5C C2 D3 AC 62 91 95 E4 79 0B E7 C8 37 6D 8D D5 4E A9 6C 56 F4 EA 65 7A AE 08 0C BA 78 25 2E 1C A6 B4 C6 E8 DD 74 1F 4B BD 8B 8A 0D 70 3E B5 66 48 03 F6 0E 61 35 57 B9 86 C1 1D 9E 0E E1 F8 98 11 69 D9 8E 94 9B 1E 87 E9 CE 55 28 DF 0F 8C A1 89 0D BF E6 42 68 41 99 2D 0F B0 54 BB 16 2005 Microchip Technology Inc. DS00953A-page 5 AN953 Xtime xtime(a) is a predefined linear feedback shift register 6. Column 3 is XORed with column 2: that repeats every 51 cycles. The operation is defined K12 ^= K8 as: K13 ^= K9 if(a<0x80) { K14 ^= K10 a<<=1; K15 ^= K11 } else Key Addition: { a=a<<1)^0x1b; Key addition is defined as each byte of the key XORed } with each of the corresponding data bytes. The key addition process is the same for both the encryption Key Schedule and decryption processes. The following is a C-code example: Each round of AES uses a different encryption key based on the previous encryption key. An example for(i=0;i<16;i++) follows that takes a given key and calculates the next { data[i] ^= key[i]; round’s key. } EXAMPLE 2: KEY GENERATION Row Shift Given the generic key: Row shift is a cyclical shift to the left of the rows in the data block according to the table below: K1 K4 K8 K12 K2 K5 K9 K13 TABLE 4: ENCRYPTION CYCLICAL K3 K6 K10 K14 SHIFT TABLE K4 K7 K11 K15 # shifts # shifts # shifts # shifts The key scheduling goes as follows: of row 0 of row 1 of row 2 of row 3 1. Column 0 is XORed with the S_Table lookup of 16-byte block 0 1 2 3 column 3: 24-byte block 0 1 2 3 K0 ^= S_Table[K13] 32-byte block 0 1 3 4 K1 ^= S_Table[K14] Note that this transformation is different for encryption K2 ^= S_Table[K15] and decryption. K3 ^= S_Table[K12] EXAMPLE 3: TRANSFORMATION 2. K0 is XORed with Rcon Given the original data: K0 ^= Rcon; 04812 3. Rcon is updated with the xtime of Rcon 15913 Rcon = xtime(Rcon); 2 6 10 14 (the starting value of Rcon is 0x01 for encoding) 371115 4.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages34 Page
-
File Size-