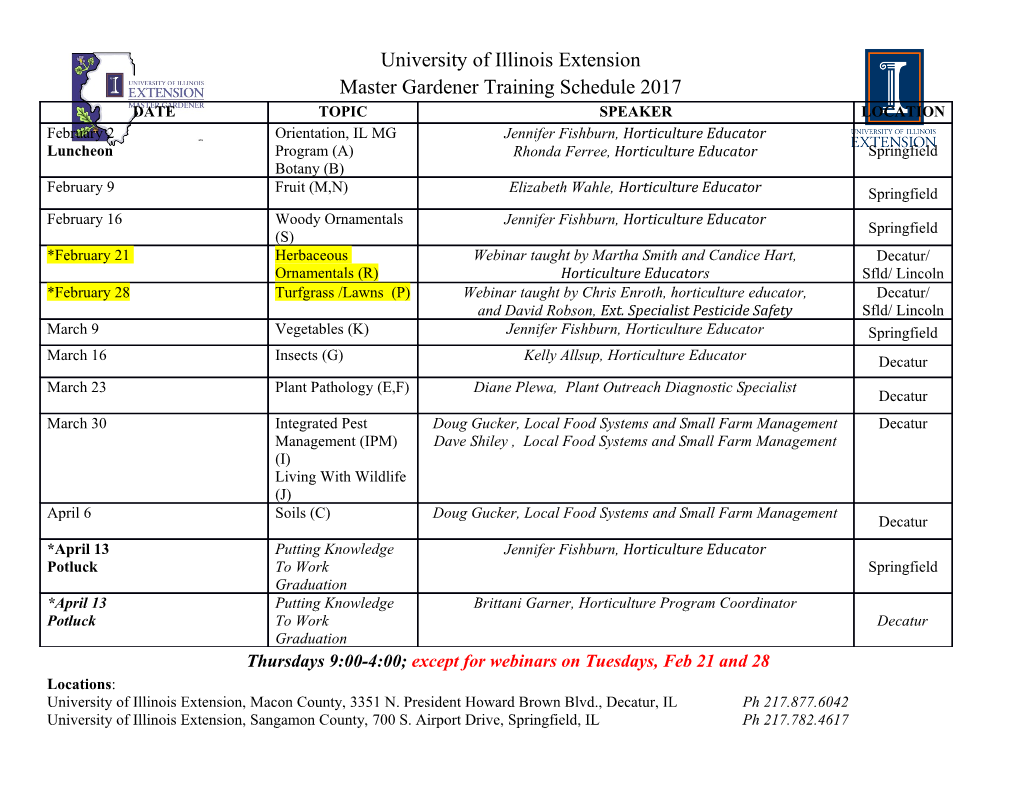
Linux Basics Shell script: • Shell script is series of commands wri7en in plain text file. We need to modify the permission to make the file executable. Why to create shell script: • To automate the task of day-to-day ac@vi@es. • Save lot of @me and manual work. • Useful to create our own commands How to write the shell scripts: 1. Open the file with vi editor 2. Type in the commands with some logic using loops and condi@onal statements. 3. Save the files and modify the permissions to make it executable. How to run the the script: • sh <script-name> • ./<script-name> • sh –x <script-name>---to debug. • sh <script-name> &---to run in background. • nohup sh <script-name> &-àto run in background and redirect o/p to nohup.out Linux Basics Components of shell script: • Hashbang(#!): Ø Hashbang tells the operang system what interpreter to use to run the script. Ø Example:#!/bin/sh,#!/bin/ksh,#!/bin/bash,#!/bin/csh • Commands: Ø Linux command like cat, ls, pwd, mkdir, cd • Variables: Ø A variable is a character string to which we assign a value. The value assigned could be a number, text, filename or any other type of data. Ø In shell script we don't need to declare a variable we can just define the variable as <variable- name>=<value> Ø To unset a variable value we use unset <variable-name> Ø There are two type of variables in unix Environmental variable: An environmental variable is a variable available to child process of a shell. Local variable: A local variable is a variable present local to the current shell ` Shell Scripng • QuoAng: Ø Quo@ng is used to groups words and limit subs@tu@ons. Ø Shell recognizes both single and double quote characters. Ø Single quote will suppress the subs@tu@on for variable where double quote performs the subs@tu@on. • RedirecAon: Ø When we execute a command by default it prints output to stdout if it succeeds and to stderr if it errors out. Stdout and stderror prints out to screen. Ø File descriptor for input is 0,stdout is 1 and stderror is 2. Ø To redirect the output of a command we use <command> > <file-name> Ø To catch user generated error messages we use <command> > &2 Ø To redirect stdout and stderr to blackhole we use <command> > /dev/null 2>&1 Ø To redirect command output to a file If it executes successfully and to redirect output to a different file it if errors out we use <command> 1 > <file1> 2> <file2> • Exit: Ø Whenever the command fails it will exit with non-zero value and whenever it is successful it exits with 0. Ø To check the exit status in the script we use $?. • Backslash: Ø Backslash is used to let the shell to ignore the special character test=10 Echo "\$test" ----print output as $test instead of 10. • Command Line Arguments: Ø We can pass arguments to shell scrip when we execute it Ø $0 is the script name,$1,$2..so on will be the inputs. • AND OR Lists: Ø And operator is && and or operator is || command1 && command2 ---command2 is executed if, and only if, comand1 returns an exit status of 0 command1 || command2 ---command2 is executed if, and only if, command1 returns a non zero exit status. • Expr: Ø expr evaluates arguments and expression Ø Expr prints the value of expression to standard output. a=5 b=5 expr text : tex c=`expr $a + $b` expr 10 \> 5 echo $c expr substr "Oracle Dba" 7 10 expr length "Oracle Dba" Linux Basics • Condional statements: Ø Unix shell supports condi@onal statements which are used to perform different ac@ons based on different condi@ons. Ø Two condi@onal statements are if…else and case...esac Ø If.....fi,if....else...fi,if...elif...else..fi are the variaons of if..else Examples: a=30 b=40 #!/bin/sh echo $a RDBMS_DATABASE="oracle" echo $b case "$RDBMS_DATABASE" in c=`expr $a - 20` "oracle") echo "Oracle is founded by Larry Ellison." if [ ! -z "$c" ] ;; then "SQL SERVER") echo "SQL server is product from Microsop." echo $c ;; else "MY SQL") echo "MY SQL is free database." echo "c is not defined" ;; fi esac i#!/bin/sh RDBMS_DATABASE="oracle" i#!/bin/ sh if [ $RDBMS_DATABASE = "oracle" ] RDBMS_DATABASE="oracle" then if ["$RDBMS_TYPE" = "oracle"] echo "Oracle is founded by Larry Ellison. " then elif [ $RDBMS_DATABASE = "SQL SERVER" ] echo "Oracle is foundes by Larry Ellison." then else echo "SQL SERVER is a product from microsop" echo "Its not oracle database" else fi echo "its not oracle or sql server" fi Linux Basics • Condional expressions: expression Descripon -d file True if directory exists -e file True if file exists -r file True if file exists and readable -w file True if file exists and writable -x file True if file exists and executable -f file True if file exists and regular file string1 = string2 True if string1 equals string2 String1 != string2 True if string1 not equal string2 -z varname True if variable is defined Arg1 op arg2 Op will take –eq,-ge,-lt,-le,-ne. returns true based on the op. Shell Scripng • Loops: Ø Looping is repeatedly execu@ng a sec@on of your program based on a condi@on. Ø The shell provides three commands for looping:while,unl and for. 1. While loop: while command causes a block to be executed over and over again, as long as condi@on is true. #!/bin/bash ################################################################################ Script to demonstrate while loop ############################################################################### count=0 cat /etc/oratab|(while read line; do count=$((count+1)) done echo "There are $count databases on the server") Shell Scripng 2. Un@l loop: The un@l command works exactly the same way as while loop except block of code repeated as long as the condi@on is false #!/bin/bash i=0 unl [ $i -gt 6 ] do i=$(( $i+1 )) case $i in 1)aempt=st echo "$i$aempt aempt" ;; 2)aempt=nd echo "$i$aempt aempt" ;; 3)aempt=rd echo "$i$aempt aempt" ;; 4|5|6|7|8|9|10)aempt=th echo "$i$aempt aempt" ;; esac done echo "Loop ran for $i mes" Shell Scripng 3. For Loop: for assigns a word from the list of words to the specified variable, executes the statements, and repeats this over and over un@l all the words have been used up. #!/bin/bash ############################################################################### script to demonstrate for loop ############################################################################## for i in `cat /etc/oratab` do auto_restart=`echo $i | awk -F ":" {'print $3'}` database_name=`echo $i |awk -F ":" {'print $1'}` if ["$auto_restart" = 'Y' ] then echo "physical database $database_name exist on the system" else echo "virtual database $database_name exist on the system" fi done exit FuncAons: Ø As programs get longer and more complex, they become more difficult to debug and maintain, Shell also provides us to create func@on by which it becomes easier to maintain and debug the code. Ø If we want to have same code mul@ple @mes in the script we can create func@ons and refer that in the code whenever we require the func@onality. !#/bin/bash Func@on date { echo `date +”%m-%d-%y” } date .
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages9 Page
-
File Size-