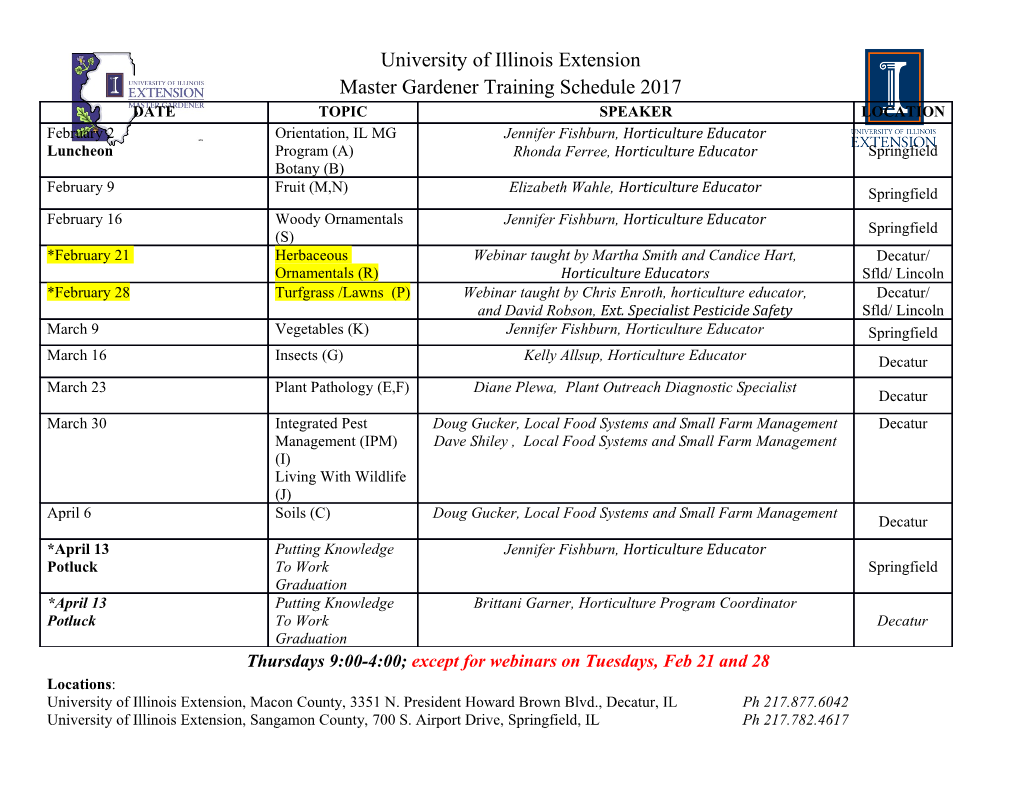
Introduction to Design Patterns !" 2003 - 2007 DevelopIntelligence What is a Pattern? ! " There are many definitions for a pattern: ! " A model or design used as a guide ! " A set of instructions to be followed when implementing something ! " An example for others to follow ! " A decorative design ! " Where do patterns exist? ! " In arts and crafts (think quilts) ! " In buildings and architecture ! " In manufacturing (think models and production) ! " In leadership (think of followers modeling a leader) ! " In software 5 History of Design Patterns ! " Concept of design patterns originated as an architectural concept by Christopher Alexander in 1977 ! " Introduced into the programming community in 1987 by Ward Cunningham and Kent Beck ! " Focused on apply design pattern concept to software ! " Applied the concept using the SmallTalk language ! " Released a paper at OOPSLA 87 called “Using pattern languages for object-oriented programmers” ! " Gained popularity in the 90s ! " Result of “Design Patterns: Elements of Reusable Object-Oriented Software” book written by Gamma, Helm, Johnson, and Vlissides ! " AKA: Gang of Four (GoF) book ! " Have grown in popularity over time !" 2003 - 2007 DevelopIntelligence What is a Design Pattern? ! " Term comes from work performed by architect Christopher Alexander ! " Used to describe architectural solutions to reoccurring problems ! " Specifically, "Each pattern describes a problem which occurs over and over again in our environment, and then describes the core of the solution to that problem, in such a way that you can use this solution a million times over, without ever doing it the same way twice” ! " Other definitions: ! " A identified and proven solution to a reoccurring problem in a specific context ! " A proven recipe describing how to solve a problem ! " A blue-print of how to solve a problem !" 2003 - 2007 DevelopIntelligence What is a Design Pattern? [Redux] Let’s think in OO terms ! " A class is a blueprint describing an object ! " An object is an instance of that blueprint ! " A design pattern is a blueprint describing a design ! " An implementation is an instance of that design within your software !" 2003 - 2007 DevelopIntelligence Purpose of Design Patterns ! " Think about motivations for OO ! " Reusable components ! " Modular software ! " Flexible and extensible solutions ! " It’s hard to get that right !" 2003 - 2007 DevelopIntelligence Purpose of Design Patterns [cont.] ! " Think about basic object oriented concepts ! " Abstraction – details are left ambiguous ! " Encapsulation – details are hidden ! " Inheritence – reusability through specialization; “is-a” ! " Polymorphism – many forms ! " Composition – collection of objects; “has-a” ! " High-cohesion – self-contained ! " Loose-coupling – flexible ! " It’s harder to get that right !" 2003 - 2007 DevelopIntelligence Purpose of Design Patterns [Redux] Specifically – design patterns solve design problems: ! " Find appropriate objects – help you identify less-obvious abstractions and objects that capture them ! " Determine object granularity – patterns can help simplify the process of composition ! " Specify object interfaces – help harden your interfaces, what’s included and what’s not included in the interface ! " Describe object implementations – help you understand the implications of certain implementations ! " Encourage reusability – don’t have to think about best approach ! " Support extensibility – change and flexibility built right in !" 2003 - 2007 DevelopIntelligence Types of Design Patterns ! " Architectural patterns ! " Specify schema / structure of a software solution ! " Example: 3-tier architecture ! " General object oriented patterns ! " Typically technology agnostic; can be applied in any OO system ! " Focus on good OO design ! " Example: Model-View-Controller ! " Technology specific patterns ! " Typically idioms or blueprints specific to a language, technology, or infrastructure ! " Focus on solving technology specific problems [possibly due to underlying technology design problems ! " Example: Java EE Service Locator Pattern !" 2003 - 2007 DevelopIntelligence Issues with Design Patterns ! " Patterns aren’t the panacea of good OO; they can be misused ! " Over-engineering ! " Slow and cumbersome ! " Confusing to maintain ! " Antipatterns – “it seemed like a good idea at the time” ! " You many end up nailing in a screw ! " First introduced by Andrew Koenigh in 1995 ! " Standard work is AntiPatterns by Brown, Malveau, McCormick, and Mowbray !" 2003 - 2007 DevelopIntelligence AntiPatterns ! " AntiPatterns are described in terms of the: ! " Cause – how did we get here ! " Symptoms – how did we recognized there’s a problem ! " Consequences – what’s the looming disaster ! " Solution – a plan to get out of the mess ! " Common AntiPatterns ! " Functional Decomposition ! " Programmer still stuck in procedural programming mindset ! " Creates classes that act like “functions” ! " Too much decomposition ! " The Blob ! " Programmer stuck in procedural mindset ! " Creates single class that contains everything ! " Too little decomposition !" 2003 - 2007 DevelopIntelligence Summary ! " Design Patterns conceptually grew from architectural works ! " Three main types of patterns: Architectural, Design, and Idioms ! " Watch out for AntiPatterns !" 2003 - 2007 DevelopIntelligence Applying Design Patterns 16 Adopting Design Patterns ! " Adopting patterns should be easy, but be aware of ! " Cultural barriers ! " Organizational barriers ! " Implementation barriers ! " Like most new things start small ! " Pick an easy pattern to implement ! " Experiment with the pattern and how it effects your system ! " Document outcomes and move forward ! " Familiarize yourself with pattern catalogues ! " Define a process !" 2003 - 2007 DevelopIntelligence Pattern Catalogues Each pattern in the catalogue should be defined by: ! " Pattern name – easy to convey a concept once its part of your vocabulary ! " Classification – where it “fits” into the overall design of things ! " Intent – answers the question “What does the pattern do?” ! " Also known as – different names of the pattern ! " Motivation – a scenario that helps illustrate the program ! " Applicability – describes situations when the pattern applies ! " Structure and Implementation – guidelines on how to implement ! " Participants and Collaborations – entities involved in the implementation ! " Consequences – side effects of applying the pattern ! " Related patterns – other patterns closely related !" 2003 - 2007 DevelopIntelligence Adopting Design Patterns Define a selection and adoption process ! " Determine need – what’s driving the adoption of a pattern ! " Determine the classification needed ! " Take an inventory of available patterns ! " Examine the intent of the pattern ! " Consider how the pattern solves the problem ! " Determine the patterns implementation constraints ! " Review any “like” patterns ! " Determine level of effort and weigh it against need ! " Implement !" 2003 - 2007 DevelopIntelligence Implementing Design Patterns ! " Two implementation strategies: ! " Design-time application ! " Refactoring application ! " Once you have identified a pattern, you’ll need to implement it: ! " Familiarize yourself with pattern ! " Examine the structure, participants, collaboration, etc. ! " Look at some sample code or example implementations ! " Choose implementation appropriate names ! " Define the classes ! " Define the application specific names for operations ! " Implement the operations !" 2003 - 2007 DevelopIntelligence Design-Time Application ! " Design-time application occurs during system design ! " Might be part of class design ! " Might be the result of a system review ! " Occurs before any code has been written ! " Design-time application gives you the most ! " Flexibility ! " Creativity ! " Choice ! " Choosing the wrong design pattern during design is easy to fix ! " But there are down-sides ! " May over-engineer ! " Design something that is hard to implement ! " Etc. !" 2003 - 2007 DevelopIntelligence What is Refactoring? ! " Purist definition: “modifying a piece of software without changing its external behavior or interface” ! " General definition: “modifying a piece of software to make the implementation more robust, flexible, maintainable, reusable, etc.” ! " As the number of artifacts in a software project grows, refactoring to design patterns is a common way to “clean up an implementation” !" 2003 - 2007 DevelopIntelligence The Refactoring Process ! " Refactoring is commonly attributed to the test-driven development and Agile process software engineering methodologies ! " The basic TDD refactoring process ! " Identify high-level considerations for the refactoring ! " Reconcile any issues that may exist prior to refactoring ! " Simplify the scope ! " Extract / inline a method ! " Extract / push-down an interface ! " Test ! " Repeat !" 2003 - 2007 DevelopIntelligence Refactoring Application ! " Refactoring application occurs during system evolution ! " Might be part of initial development ! " May occur late in the applications lifecycle ! " Changes made to existing software ! " Refactoring application can create ! " Create extensibility, reusability, maintainability, etc. ! " Limit over-engineering ! " But there are down-sides ! " May introduce a large number of bugs and potentially other design issues ! " Choosing the wrong design pattern during refactoring is hard to fix !" 2003 - 2007 DevelopIntelligence Refactoring Design Patterns ! " Refactored always refactoring…
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages26 Page
-
File Size-