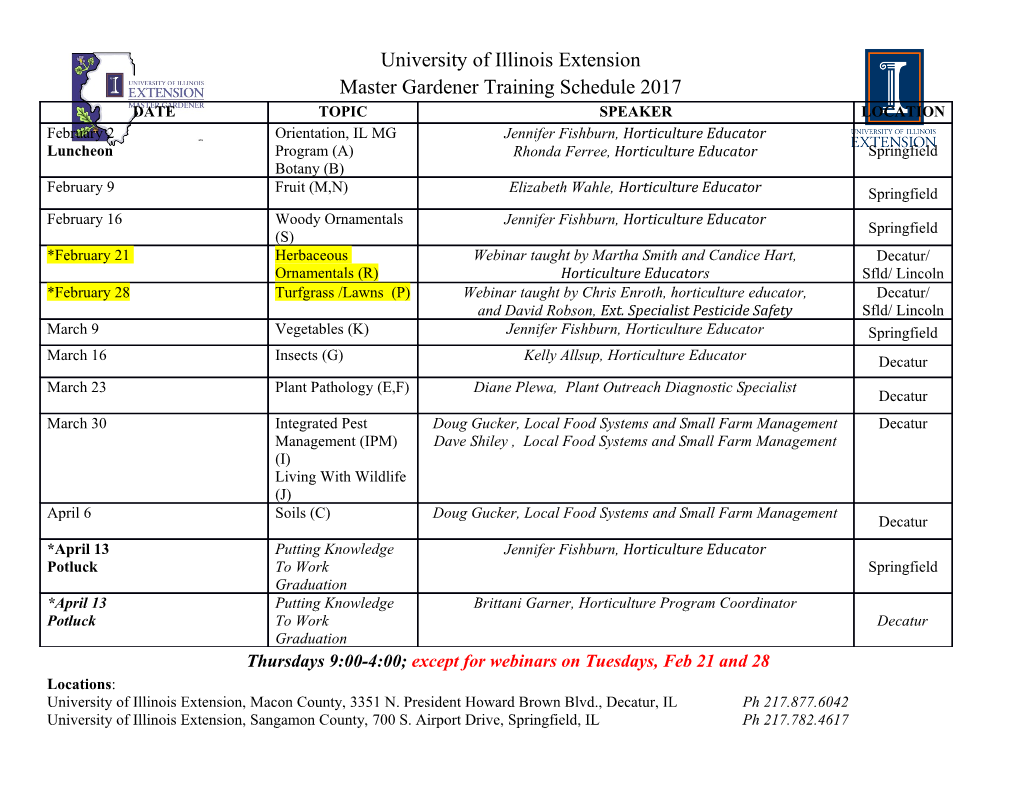
USENIX Association Proceedings of the 12th USENIX Security Symposium Washington, D.C., USA August 4–8, 2003 THE ADVANCED COMPUTING SYSTEMS ASSOCIATION © 2003 by The USENIX Association All Rights Reserved For more information about the USENIX Association: Phone: 1 510 528 8649 FAX: 1 510 548 5738 Email: [email protected] WWW: http://www.usenix.org Rights to individual papers remain with the author or the author's employer. Permission is granted for noncommercial reproduction of the work for educational or research purposes. This copyright notice must be included in the reproduced paper. USENIX acknowledges all trademarks herein. Dynamic Detection and Prevention of Race Conditions in File Accesses Eugene Tsyrklevich Bennet Yee [email protected] [email protected] Department of Computer Science and Engineering University of California, San Diego Abstract Some security vulnerabilities, such as buffer overflows, receive a lot of attention and have a variety of solutions to prevent them. Other vulnerabilities, such as race con- Race conditions in filesystem accesses occur when se- ditions, receive far less attention and have no definite quences of filesystem operations are not carried out in solutions to stop them. In addition, race conditions can an isolated manner. Incorrect assumptions of filesystem be very hard to spot and eliminate at compile time, it namespace access isolation allow attackers to elevate is thus beneficial to develop a dynamic and automated their privileges without authorization by changing the protection against this type of flaws. namespace bindings. To address this security issue, we propose a mechanism for keeping track of all filesystem This paper presents an analysis of filesystem race con- operations and possible interferences that might arise. If dition vulnerabilities and ways to prevent them. It also a filesystem operation is found to be interfering with an- describes a prototype system that detects and stops all other operation, it is temporarily suspended allowing the realistic filesystem race condition attacks known to us. first process to access a file object to proceed, thereby The proposed solution stops the attacks with minimum reducing the size of the time window when a race condi- performance overhead, while retaining application com- tion exists. The above mechanism is shown to be effec- patibility. tive at stopping all realistic filesystem race condition at- tacks known to us with minimal performance overhead. The rest of this paper is organized as follows. Sec- tion 2 describes race conditions in filesystem accesses and presents several sample vulnerabilities. The design of the prototype system and the rationale behind it is 1 Introduction covered in Section 3. Section 4 details the implementa- tion of the prototype system, as well as some of the de- cisions made during the design phase incorporating the security of the system and code portability. Section 5 Dating back to the 1970’s, race conditions, otherwise reviews the attack scenarios used for the security testing known as the Time Of Check To Time Of Use (TOCT- of the prototype system. Section 6 describes the com- TOU) bug, are one of the oldest known security flaws. patibility testing and Section 7 covers the system perfor- They are a class of local vulnerabilities that can be used mance. Section 8 reviews related work, Section 9 pro- to elevate one’s privileges without authorization and oc- poses future work and Section 10 provides the conclu- cur when operations are not carried out atomically as sions. expected. Race conditions have been found in a variety of sce- narios ranging from tracing processes [9] to filesystem accesses [13]. This paper focuses on a subset of race 2 Race Conditions in File Accesses conditions dealing with filesystem accesses. The threat model is that of an adversary with unprivileged access to a system who is trying to elevate his or her privileges by Race conditions in filesystem accesses occur when ap- exploiting a filesystem race condition vulnerability. plications incorrectly assume that a sequence of filesys- USENIX Association 12th USENIX Security Symposium 243 if (access(fn, R_OK | W_OK) == 0) { chdir("/tmp/a") fd = open(fn, O_RDWR, 0644); chdir("b") if (fd < 0) chdir("c") { unlink("*") err(1, fn); [attacker entrypoint: "mv /tmp/a/b/c /tmp"] } chdir("..") rmdir("c") /* use the file */ unlink("*") chdir("..") Figure 1: Race Condition in Access Operation rmdir("b") unlink("*") tem operations is atomic and the filesystem namespace rmdir("/tmp/a") is otherwise static. In reality, attackers can modify the filesystem namespace at any time by replacing files with Figure 2: Race Condition in Directory Operations symbolic links or by renaming files and directories. of a recursive removal of the /tmp/a directory tree that 2.1 Access Operation contains two nested subdirectories. Consider what hap- pens when an attacker moves /tmp/a/b/c directory to /tmp before the first chdir("..") call is invoked. After The code fragment in Figure 1 is taken from an inse- removing the contents of the /tmp/a/b/c subdirectory cure setuid application that needs to check whether a (now /tmp/c) and executing two chdir("..") calls, in- user running the program has the right to write to a file stead of changing the directory to /tmp/a, the rm utility (since the application is setuid root, it can write to any would find itself in the root directory that it would con- file). tinue to delete. The source code incorrectly assumes that a file object The race condition was fixed by adding additional referenced by the filename “fn” does not change in be- checks to verify that the inode of the directories did not tween the access and open invocations. The assump- change before and after the chdir calls; in other words, tion is invalid since an attacker can replace the file ob- the directory tree namespace did not change during the ject “fn” with another file object (such as a symbolic link execution of the tool. to another file), tricking the program into accessing ar- bitrary files. To avoid this race condition, an application should change its effective id to that of a desired user and then make the open system call directly or use fstat af- 2.3 Setuid Shell Scripts ter the open instead of invoking access. The secure execution of setuid shell scripts is a well- 2.2 Directory Operations known problem in the earlier Unix systems. To deter- mine whether a file is a script and should be processed by an interpreter, a Unix kernel checks the first two bytes of Another subtle race condition was recently discovered in a file for a special “#!” character sequence. If the char- the GNU file utilities package [13]. During the recursive acter sequence is found, the rest of the line is treated as removal of a directory tree, an insecure chdir("..") the interpreter pathname (and its parameters) that should operation is performed, after removing the content of a be executed to process the script. Unfortunately, in some subdirectory, to get back to the upper directory. An at- kernels, the operation of opening and processing the first tacker, who is able to exploit this race condition, can line of a file and the subsequent execution of the inter- trick users into deleting arbitrary files and directories. preter that reopens the script is not atomic. This allows The bug is triggered when an attacker moves a subdi- attackers to trick the operating system into processing rectory, that the rm utility is processing, to a different one file while executing the interpreter with root privi- directory. leges on another file. Figure 2 contains a slightly abbreviated system call trace Various Unix systems deal with this problem in differ- 244 12th USENIX Security Symposium USENIX Association if (stat(fn, &sb) == 0) { gram is the only program accessing the filesystem. In- fd = open(fn, O_CREAT | O_RDWR, 0); stead, an attacker can change the filesystem namespace if (fd < 0) in the midst of a sequence of filesystem system calls and { exploit the race condition in the code. We call the se- quence of filesystem system calls that programmers as- err(1, fn); } sumed to be free from interference a pseudo-transaction. To address the problem of race conditions, it would be /* use the file */ sufficient to provide the illusion of a filesystem names- pace that behaves as if these pseudo-transactions were Figure 3: Race Condition in Temporary File Accesses executed in isolation and free from interference. To achieve this, the system needs to be able to detect race condition attacks and stop them. ent ways. Earlier Linux systems simply disregarded the setuid bits when executing the shell scripts. Some SysV systems as well as BSD 4.4 kernels pass to the in- 3.1 Transactions terpreter a filename in a private, per-process filesystem namespace representing the already opened descriptor to the script (e.g. /dev/fd/3) in lieu of the original path- The goal of detecting and preventing race conditions name. Passing such a pathname closes down the race would be trivially achieved if executions of processes condition vulnerability window since it is impossible for (or process groups) were transactions that enjoyed the an attacker to change the private per-process filesystem ACID properties (Atomicity, Consistency, Isolation and namespace bindings. Durability). ACID transactions were designed to permit concurrent database accesses while maintaining correct- ness. These ideas can be readily translated to filesystem 2.4 Temporary File Accesses accesses. The atomicity property requires that a transaction com- Another type of race condition occurs in the handling of mits all the involved data or none at all. temporary files. An unsafe way to create a temporary file is to use the stat system call to check whether a The consistency property guarantees that a transaction file exists and if the file does not exist, invoke open to produces only consistent results; otherwise it aborts.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages14 Page
-
File Size-