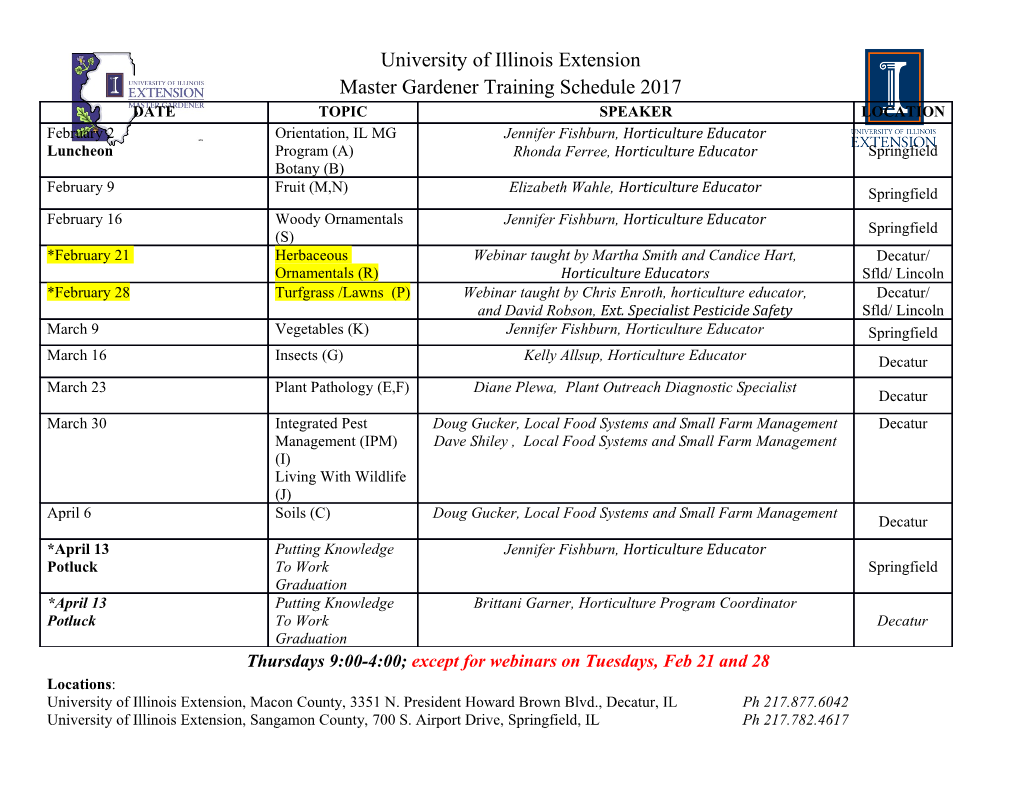
JavaServer Pages Hans Bergsten First Edition, December 2000 ISBN: 1-56592-746-X, 572 pages JavaServer Pages shows how to develop Java-based web applications without having to be a hardcore programmer. The author provides an overview of JSP concepts and illuminates how JSP fits into the larger picture of web applications. There are chapters for web authors on generating dynamic content, handling session information, and accessing databases, as well as material for Java programmers on creating Java components and custom JSP tags for web authors to use in JSP pages.JavaServer Pages shows how to develop Java-based web applications without having to be a hardcore programmer. The author provides an overview of JSP concepts and illuminates how JSP fits into the larger picture of web applications. There are chapters for web authors on generating dynamic content, handling session information, and accessing databases, as well as material for Java programmers on creating Java components and custom JSP tags for web authors to use in JSP pages. Release Team[oR] 2001 Preface 1 What's in This Book Audience Organization About the Examples Conventions Used in This Book How to Contact Us Acknowledgments i JSP Application Basics This part of the book describes the fundamentals of HTTP (the protocol used by all web applications), how servlets and JSP are related, and how to set up a JSP development environment and install the book examples. 1 Introducing JavaServer Pages 8 1.1 What Is JavaServer Pages? 1.2 Why Use JSP? 1.3 What You Need to Get Started 2 HTTP and Servlet Basics 13 2.1 The HTTP Request/Response Model 2.2 Servlets 2.3 Packaging Java Web Applications 3 JSP Overview 25 3.1 The Problem with Servlets 3.2 The Anatomy of a JSP Page 3.3 JSP Processing 3.4 JSP Application Design with MVC 4 Setting Up the JSP Environment 34 4.1 Installing the Java Software Development Kit 4.2 Installing the Tomcat Server 4.3 Testing Tomcat 4.4 Installing the Book Examples 4.5 Example Web Application Overview ii JSP Application Development The focus of this part of the book is on developing JSP-based web applications using both standard JSP elements and custom components. Through the use of practical examples, you will learn how to handle common tasks such as validating user input, accessing databases, authenticating users and protecting web pages, localizing your web site, and more. 5 Generating Dynamic Content 42 5.1 What Time Is It? 5.2 Input and Output 6 Using Scripting Elements 55 6.1 Java Primer 6.2 Implicit JSP Objects 6.3 Conditional Processing 6.4 Displaying Values 6.5 Using an Expression to Set an Attribute 6.6 Declaring Variables and Methods 7 Error Handling and Debugging 74 7.1 Dealing with Syntax Errors 7.2 Debugging a JSP-Based Application 7.3 Dealing with Runtime Errors 8 Sharing Data Between JSP Pages, Requests, and Users 87 8.1 Passing Control and Data Between Pages 8.2 Sharing Session and Application Data 8.3 Using Custom Actions 8.4 Online Shopping 8.5 Memory Usage Considerations 9 Database Access 109 9.1 Accessing a Database from a JSP Page 9.2 Input Validation Without a Bean 9.3 Using Transactions 9.4 Application-Specific Database Actions 10 Authentication and Personalization 130 10.1 Container-Provided Authentication 10.2 Application-Controlled Authentication 10.3 Other Security Concerns 11 Internationalization 148 11.1 How Java Supports Internationalization and Localization 11.2 Generating Localized Output 11.3 A Brief History of Bits 11.4 Handling Localized Input 12 Bits and Pieces 165 12.1 Buffering 12.2 Including Page Fragments 12.3 XML and JSP 12.4 Mixing Client-Side and Server-Side Code 12.5 Precompiling JSP Pages 12.6 Preventing Caching of JSP Pages 12.7 How URLs Are Interpreted III: JSP in J2EE and JSP Component Development If you're a programmer, this is the part of the book where the real action is . Here you will learn how to develop your own custom actions and JavaBeans, and how to combine JSP with other Java server-side technologies, such as servlets and Enterprise JavaBeans (EJB). 13 Web Application Models 182 13.1 The Java 2 Enterprise Edition Model 13.2 The MVC Model 13.3 Scalability 14 Combining Servlets and JSP 190 14.1 Using a Servlet as the Controller 14.2 A More Modular Design Using Action Objects 14.3 Sharing Data Between Servlets and JSP Pages 14.4 Using a JSP Error Page for All Runtime Errors 15 Developing JavaBeans for JSP 200 15.1 JavaBeans as JSP Components 15.2 JSP Bean Examples 15.3 Unexpected <jsp:setProperty> Behavior 16 Developing JSP Custom Actions 213 16.1 Tag Extension Basics 16.2 Developing a Simple Action 16.3 Processing the Action Body 16.4 Letting Actions Cooperate 16.5 Creating New Variables Through Actions 16.6 Developing an Iterating Action 16.7 Creating the Tag Library Descriptor 16.8 Validating Syntax 16.9 How Tag Handlers May Be Reused 16.10 Packaging and Installing a Tag Library 17 Developing Database Access Components 235 17.1 Using Connections and Connection Pools 17.2 Using a Generic Database Bean 17.3 Developing Generic Database Custom Actions 17.4 Developing Application-Specific Database Components iv Appendixes In this part of the book, you find reference material, such as descriptions of JSP elements and classes, all book example components, the web application deployment descriptor, and more. A JSP Elements Syntax Reference 260 A.1 Directive Elements A.2 Scripting Elements A.3 Action Elements A.4 Comments A.5 Escape Characters B JSP API Reference 270 B.1 Implicit Variables B.2 Servlet Classes Accessible Through Implicit Variables B.3 Tag Extension Classes B.4 Other JSP Classes C Book Example Custom Actions and Classes Reference 312 C.1 Generic Custom Actions C.2 Internationalization Custom Actions C.3 Database Custom Actions C.4 Utility Classes C.5 Database Access Classes D Web-Application Structure and Deployment Descriptor Reference 337 D.1 Web Application File Structure D.2 Web Application Deployment Descriptor D.3 Creating a WAR File E JSP Resource Reference 346 E.1 JSP-Related Products E.2 Web Hosting E.3 Information and Specifications Colophon 350 JavaServer Pages (JSP) technology provides an easy way to create dynamic web pages. JSP uses a component- based approach that allows web developers to easily combine static HTML for look-and-feel with Java components for dynamic features. The simplicity of this component-based model, combined with the cross-platform power of Java, allows a web development environment with enormous potential. JavaServer Pages shows how to develop Java-based web applications without having to be a hardcore programmer. The author provides an overview of JSP concepts and discusses how JSP fits into the larger picture of web applications. Web page authors will benefit from the chapters on generating dynamic content, handling session information, accessing databases, authenticating users, and personalizing content. In the programming- oriented chapters, Java programmers learn how to create Java components and custom JSP tags for web authors to use in JSP pages. JavaSercer Pages Preface JavaServer Pages™ (JSP) is a new technology for web application development that has received a great deal of attention since it was first announced. Why is JSP so exciting? One reason is that JSP is Java-based, and Java is well-suited for enterprise computing. In fact, JSP is a key part of the Java™ 2 Enterprise Edition (J2EE) platform and can take advantage of the many Java Enterprise libraries, such as JDBC, JNDI, and Enterprise JavaBeans™. Another reason is that JSP supports a powerful model for developing web applications that separates presentation from processing. Understanding why this is so important requires a bit of a history lesson. In the early days of the Web, the only tool for developing dynamic web content was the Common Gateway Interface (CGI). CGI outlined how a web server made user input available to a program, as well as how the program provided the web server with dynamically generated content to send back. CGI scripts were typically written in Perl. (In fact, CGI Perl scripts still drive numerous dynamic web sites.) However, CGI is not an efficient solution. For every request, the web server has to create a new operating-system process, load a Perl interpreter and the Perl script, execute the script, and then dispose of the entire process when it's done. To provide a more efficient solution, various alternatives to CGI have been added to programmers' toolboxes over the last few years: FastCGI, for example, runs each CGI program in an external permanent process (or a pool of processes). In addition, mod_perl for Apache, NSAPI for Netscape, and ISAPI for Microsoft's IIS all run server-side programs in the same process as the web server itself. While these solutions offer better performance and scalability, each one is supported by only a subset of the popular web servers. The Java Servlet API, introduced in early 1997, provides a solution to the portability issue. However, all these technologies suffer from a common problem: HTML code embedded inside programs. If you've ever looked at the code for a servlet, you've probably seen endless calls to out.println( ) that contain scores of HTML tags. For the individual developer working on a simple web site this approach may work fine, but it makes it very difficult for people with different skills to work together to develop a web application. This is becoming a significant problem.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages356 Page
-
File Size-