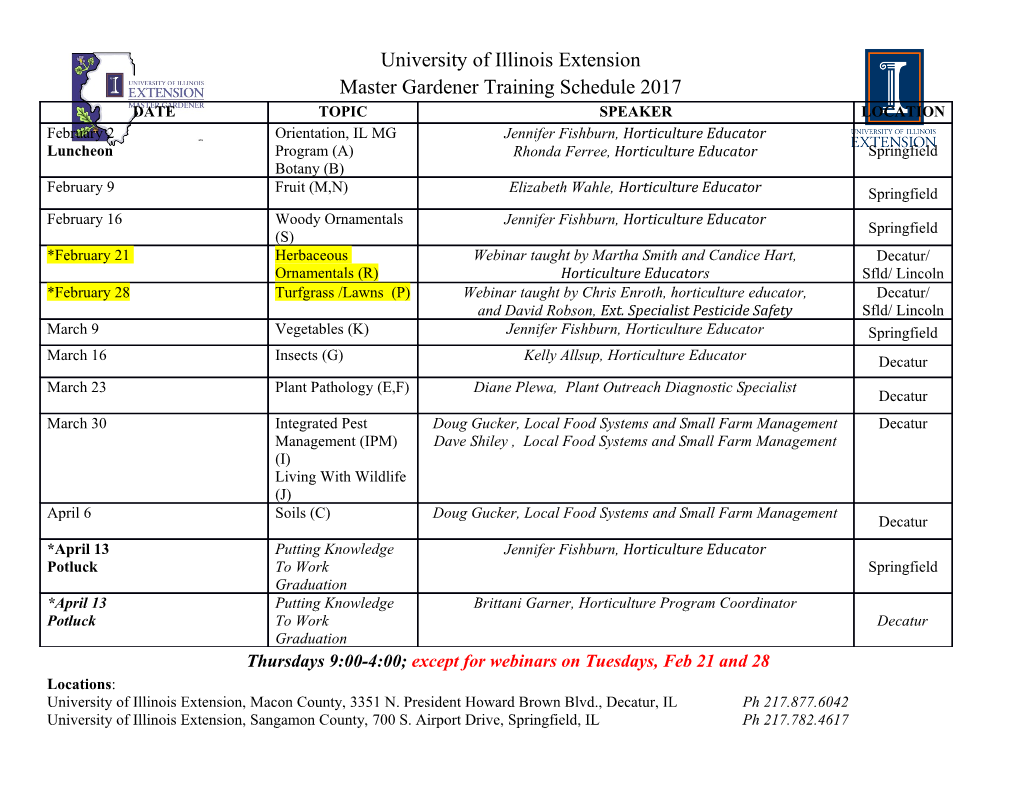
SUBMITTED TO IEEE TRANSACTIONS ON COMPUTERS 1 Moore: Interval Arithmetic in Modern C++ W. F. Mascarenhas I. INTRODUCTION libraries, and it is significantly faster than the current reference implementation of the IEEE Standards in NTERVAL arithmetic is a powerful tool, which C++ [9] (the other reference implementation, [5], can be used to solve practical and theoretical I is implemented in Octave and is not comparable to problems. However, implementing an interval arith- our library.) metic library for general use is difficult, and requires This article was written for people which are much attention to detail. These details involve the already familiar with interval arithmetic, who will specification of interfaces, the implementation of understand us when we say that, when used prop- efficient and accurate algorithms and dealing with erly, our library satisfies all the usual containment bugs, which can be our own fault or the compiler’s. requirements of interval arithmetic. Our purpose is Regarding interfaces, there are many design de- to describe the library, show that it is competitive cisions to be made and each author has his own with well known libraries, and expose its limitations opinions, as one can see by comparing the libraries (see the last section.) Even with these limitations, in [1]–[11]. Recently two documents [12], [13] were we would like to invite readers to experiment with elaborated in order to provide a common interface the library. In fact, by reading this article one will for interval arithmetic libraries. Formally, the first have only a glimpse of the library, and the only way document [12] is an active IEEE standard, while too fully understand it is to try it in practice. The the second [13] is still a project. For simplicity, Moore library is open source software, distributed throughout the article we will refer to these docu- under the Mozilla 2.0 license, and its source code ments as “The IEEE standards.” These standards are can be obtained by sending an e-mail message to a significant step forward, but there is controversy in the author. the interval arithmetic community regarding them. In the rest of this article we present the library, For instance, prof. U. Kulisch has made clear his starting from the basic arithmetic operations and disagreement with them. moving to more advanced issues. We also describe This article presents the Moore library, which in which points our library deviates from the IEEE implements part of the IEEE standards in the most standards and the parts of the standards that we do recent version of the C++ language, using new implement. features of this language. The library was written mostly to be used on our own research, and we arXiv:1611.09567v1 [cs.MS] 29 Nov 2016 focus on improving the code more likely to be used II. HELLO INTERVAL WORLD often. For this reason, we do not fully implement The Moore library can be used by people with the IEEE standards. However, we do hope that varying degrees of expertise. Non experts can sim- the library will be useful to others. People for ply follow what is outlined in the code below: which compliance with the standards is a priority would be better served by the libraries in [5] and #include "moore/minimal.h" [9]; people looking for better performance or more Moore::RaiiRounding r; precise types of endpoints for their intervals may Moore::Interval<> x(2.0, 3.0); consider using our library. For instance, in Section Moore::Interval<> y("[-1,2]"); VII we present experiments showing that our library is competitive in terms of speed with well known for(int i = 0; i < 10; ++i) { Walter Mascarenhas works at the Institute of Mathematics and y = (sin(x) - (y/x + 5.0) * y) * 0.05; Statistics, Universidade de São Paulo, Brazil. His e-mail address is cout << y << endl; [email protected]. } SUBMITTED TO IEEE TRANSACTIONS ON COMPUTERS 2 In English, with the Moore library one can construct • The interval x has endpoints of type double. intervals by providing their endpoints as numbers • y has endpoints of type float. or strings, and then use them in arithmetical ex- • The endpoints of z have quadruple precision. pressions as if they were numbers. The library also • w has endpoints of type Real<256>, which rep- provides the trigonometric and hyperbolic functions, resents floating point numbers with N = 256 their inverses, exponentials and logarithms, and con- bits of mantissa, and the user can choose other venient ways to read and write intervals to streams. values for N. The file minimal.h in the code above contains • The compiler deduces that i is an interval the required declarations for using the library with with endpoints of type double, which is the double endpoints. The line appropriate type for storing the convex hull of Moore::RaiiRounding r; x, y and 0.3. • It also deduces that Real<256> is the ap- is required in order to use the library. It sets the propriate type of endpoints for the intervals rounding mode to upwards, and the rounding mode representing the intersection of x,y, z and w and is restored when r is destroyed, following the Re- the result of the evaluation of the expression source Acquisition is Initialization (Raii) pattern in assigned to j. C++. Our approach is similar to one option provided We ask the reader not to underestimate the code in by the boost library [1]. However, the boost library the previous paragraph. It is difficult to develop the is more flexible than ours: we only provide one infrastructure required for users to handle intervals rounding policy. In fact, providing fewer options with endpoints of different types in expressions as instead of more is the usual choice made by the natural as the ones in that code. In fact, there are Moore library. We only care about concrete use numerous issues involved in dealing with intervals cases motivated by our own research, instead of with generic endpoints, and simply writing generic all possible uses of interval arithmetic. This is the code with this purpose is not enough. The code main difference between the spirit of our library must be tested, and our experience shows that it and the purpose of the IEEE standards for interval may compile for some types of endpoints and may arithmetic. We prefer to provide a better library for not compile for others. a few users rather than trying to please a larger There are two main points in which the Moore audience which we will never reach. library does not follow the IEEE standards: decora- The intervals in the Moore library are parame- tions and exceptions. As we explain in Section V, terized by a single type. It does not contain class hierarchies, virtual methods or policy classes. On we do not provide decorated intervals, because in the one hand, users can only choose the type of the our opinion decorations are a bad idea. We agree endpoints defining the intervals of the form [a; b] that it is quite useful to have standards, and we are with −∞ ≤ a ≤ b ≤ +1, or the empty interval. glad to acknowledge the positive influence of the On the other hand, we do believe that our library IEEE standards in our library. However, we also goes beyond what is offered by other libraries in believe that standards should cover only the minimal its support of generic endpoints and operations. As we explain in Section IV, the library can work with number of features required to achieve the goals of several types of endpoints “out of the box,” that is, the majority of the users, and decorations do not it provides tested code in which several types of satisfy this basic criterium. We should also mention endpoints can be combined, as in this example: that we did implement a library which supported decorations, and that when we analyzed the result RaiiRounding r; Interval<> x("[-1,2]"); it was evident that this implementation should be Interval<float> y("[-1/3,2/3]"); thrown away. Interval<__float128> z("[-inf,4"]); We also treat exceptions differently. In the Moore Interval<Real<256>> w("5?"); library, the term exception applies to an error from which no recovery is possible (or desirable), and auto h = hull(x, y, 0.3); such that its occurrence leads to the termination of auto i = intersection(x, y, z, w); the program. For instance, as the excellent GMP auto j = sin(z * x/cos(y * z)) - exp(w); library, we find it appropriate to terminate the pro- The code above handles four kinds of endpoints: gram when we are not able to allocate memory. In SUBMITTED TO IEEE TRANSACTIONS ON COMPUTERS 3 fact, any library relying on GMP would be subject fied standard [13], including the ones which would to program termination in case of errors in memory qualify our library as a flavor of the full standard allocation, unless precautions are taken. The Moore [12] in the absence of the points mentioned in the library uses GMP and did not take any precautions. previous paragraph. We also implement the reverse In our library, warnings are indicated by return and overlapping functions mandated by the full values instead of raising flags. For instance, instead standard [12]. In the end, people not interested of raising an inexact flag when constructing an inter- in decorations and exceptions will be able to use val from the string "[1/3,2/3]" we provide a con- structor with a second argument of type Accuracy our library as they would use any other library which indicates the accuracy with which the string conforming with the standards, with the additional is converted to an interval, as in functionality described in the next sections.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages8 Page
-
File Size-