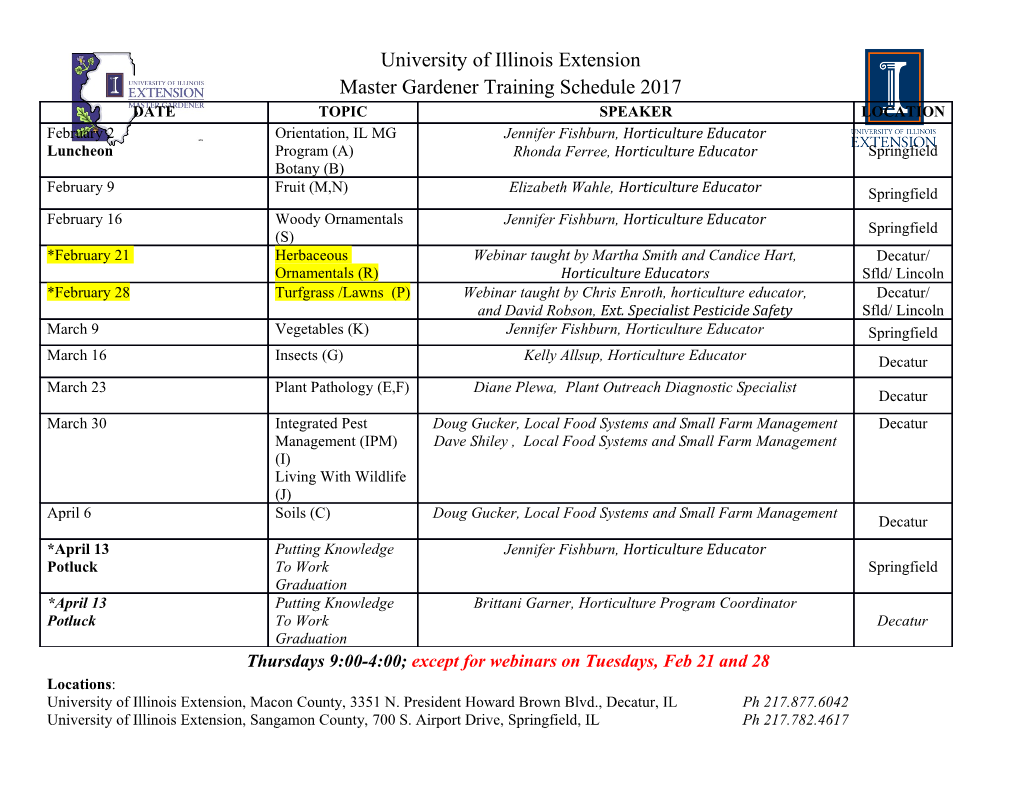
Week 2: Numpy+Matplotlib AST 325 tutorial week 2 TA: Siqi Liu, Alex Roman 2018-09-17/18 Installation Scipy Stack (ref from www.numpy.org) ● Installing the Scipy Stack: ○ Python (2.x >= 2.6 or 3.x >= 3.2) ○ NumPy (>= 1.6) ○ SciPy library (>= 0.10) ○ Matplotlib (>= 1.1) ■ dateutil ■ pytz ■ Support for at least one backend ○ IPython (>= 0.13) ■ pyzmq ■ tornado ○ pandas (>= 0.8) ○ Sympy (>= 0.7) ○ nose (>= 1.1) Installation (Linux) ● Installing through pip: ○ Upgrade pip: ■ python -m pip install --upgrade pip ○ Install the scipy package: ■ pip install --user numpy scipy matplotlib ipython jupyter pandas sympy nose ○ Make sure the dir is correct: ■ Linux: export PATH="$PATH:/home/your_user/.local/bin" ■ MacOS: export PATH="$PATH:/Users/your_user/Library/Python/3.5/bin" ● Install systemwide via a Linux package manager¶ ○ Ubuntu & Debian: ■ sudo apt-get install python-numpy python-scipy python-matplotlib ipython ipython-notebook python-pandas python-sympy python-nose ○ Fedora: ■ sudo dnf install numpy scipy python-matplotlib ipython python-pandas sympy python-nose atlas-devel Installation (MacOS) ● Pip ● Macports ● Homebrew ● Anaconda ● Spyder (what we are using for this lab) Installation (Windows) ● Compared to OSX and Linux, building NumPy and SciPy on Windows is difficult, largely due to the lack of compatible, open-source libraries like LAPACK or ATLAS that are necessary to build both libraries and have them perform relatively well. You can’t sudo apt-get install everything like you can on the other two platforms. ○ MinGW ○ CygWin Python basics (if statement) ● If statement a = 200 b = 33 if b > a: print("b is greater than a") elif a == b: print("a and b are equal") else: print("a is greater than b") Python basics: loop ● For loop for x in range(6): print(x) ● While loop: i = 1 while i < 6: print(i) i += 1 Functions ● Define a function: def my_abs(x): if x >= 0: return x else: return -x Python basics: Numpy Array (1) ● NumPy’s main object is the homogeneous multidimensional array: ○ The ndarray ○ The data-type object that describes the layout of a single fixed-size element of the array ○ The array-scalar Python object that is obtained when a single element of the array is assessed. Python basics: Numpy Array (2) ● Constructing ○ From list ■ np.array(my_list) ○ Zeros with a shape tuple ■ np.zeros(shape_tuple) e.g. ● np.zeros( (10, 5) ) gives a 10x5 array of value 0.0 ○ Random float distributed on (0,1) of length n: ■ np.random.rand(n) ○ Random float matrix of size m x n ■ ar = np.mat( np.random.rand( m * n ).reshape(m, n) ) ○ n samples drawn from a normal distribution with mean 0, variance 1: ■ np.random.normal(0.0, 1.0, n) ○ Range of integers from 0 to n with step size 1 ■ np.arange(n) ○ n equispaced floats from a to b ■ np.linspace(a, b, n) Python basics: Numpy Array (3) ● Indexing and slicing ○ Import numpy as np ○ >>> x = np.array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]) >>> x[1:7:2] array([1, 3, 5]) >>>x[0::2] array([0, 2, 4, 6, 8]) ○ ar = np.random.rand(100) ar[ar > 0.5] -> only the elements above 0.5 (note that ar > 0.5 actually is a mask of booleans!) ● Array methods: ○ Shape manipulate: x.reshape(2,5) ○ Conversion to list: x.tolist() ● Data type: int, bool, float, complex, bytes, str, unicode, buffer ○ In general, the dtype you need is np.<dtype_name> e.g. a 32-bit float is np.float32 ○ Follows C conventions (just google it!) ○ Generally safe to use np.float (same as float); this is a “double” in C parlance Python basics: Numpy Array (4) ● Arithmetic and matrix multiplication, comparison operations ○ Defined as element-wise operations, and generally yield ndarray objects as results. ○ Each of the arithmetic operations (+, -, *, /, //, %, divmod(), ** or pow(), <<, >>, &, ^, |, ~) and the comparisons (==, <, >, <=, >=, !=) is equivalent to the corresponding universal function (or ufunc for short) in NumPy ○ A*B # elementwise product EXCEPT if A and B are matrices, then it’s the matrix product! Read: matrix objects A.dot(B) # matrix product np.dot(A, B) # another matrix product ○ Note: use my_matrix.A to access an ndarray copy of your np.matrix object my_matrix, e.g. when you want to do elementwise operations Exercise 1 ● Constructing a 20x5 array, normally distributed (mean=0, variance=1) ● Calculate the sum of the array ● Calculate the mean, standard deviation (std), min and max value along the first axis (axis 0). Hint: new_array = np.random.normal(mean, variance, shape) statistic = np.stat_name(my_array, axis=my_axis) Matplotlib: Sample Plots Line plot Multiple Image Line subplots in plot a figure Histogram Contour plots and pseudo-color 3D plot Matplotlib: customize plots with style sheets and rcParameters import numpy as np ● Use style sheets: import matplotlib.pyplot as plt import matplotlib as mpl plt.style.use('ggplot') data = np.random.randn(50) ● Configure your own: mpl.rcParams['lines.linewidth'] = 2 mpl.rcParams['lines.color'] = 'r' plt.plot(data) Exercise 2: Plotting 2D Arrays ● We’re going to graphically ‘solve’ the equation x^2 - y^2 = -½ ● Make a 2D array of x^2 of size 100 x 100 on the range (0,1): ○ Mega hint: x = np.tile( np.linspace( 0.0, 1.0, 100 ), 100 ).reshape( 100, 100 ) ● Now, form y by taking the transpose of x (hint: x.T is the transpose of x) ● Now, form your result z = x^2 - y^2 ● Plot the result using matplotlib.pyplot.imshow() ○ Tip - use the import statement “import matplotlib.pyplot as plt” (lots of refs use plt to mean this) ● Save the first plot using plt.savefig(plotname.png) ● Make a ‘mask’ of elements between -0.51 and -0.49 ● Plot the mask in a separate figure and save ● You solved the equation! References: Ask Google! First Python Tutorial (REQUIRED) - The first tutorial on getting familiar with Python. (PDF) Pyplot Tutorial (REQUIRED) - The basic Python plotting tutorial. Numpy Tutorial - The basic Python numerical tutorial. How to think like a Computer Scientist - If you are completely new to computer programming, this is the place to start. Specifically, going through Chapters 1-9 to get started. Introduction to Scientific Computing with Python (PDF) - The slides of a brief tutorial on how to program for science in python with basic examples. Numpy: Lock'n Load - A followable tutorial on how to get using Python/Numpy right away. (External Site) Thesaurus of Mathematical Languages - Useful if you are familiar with Matlab/IDL and want to find the corresponding function in Python/Numpy. (External Site).
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages18 Page
-
File Size-