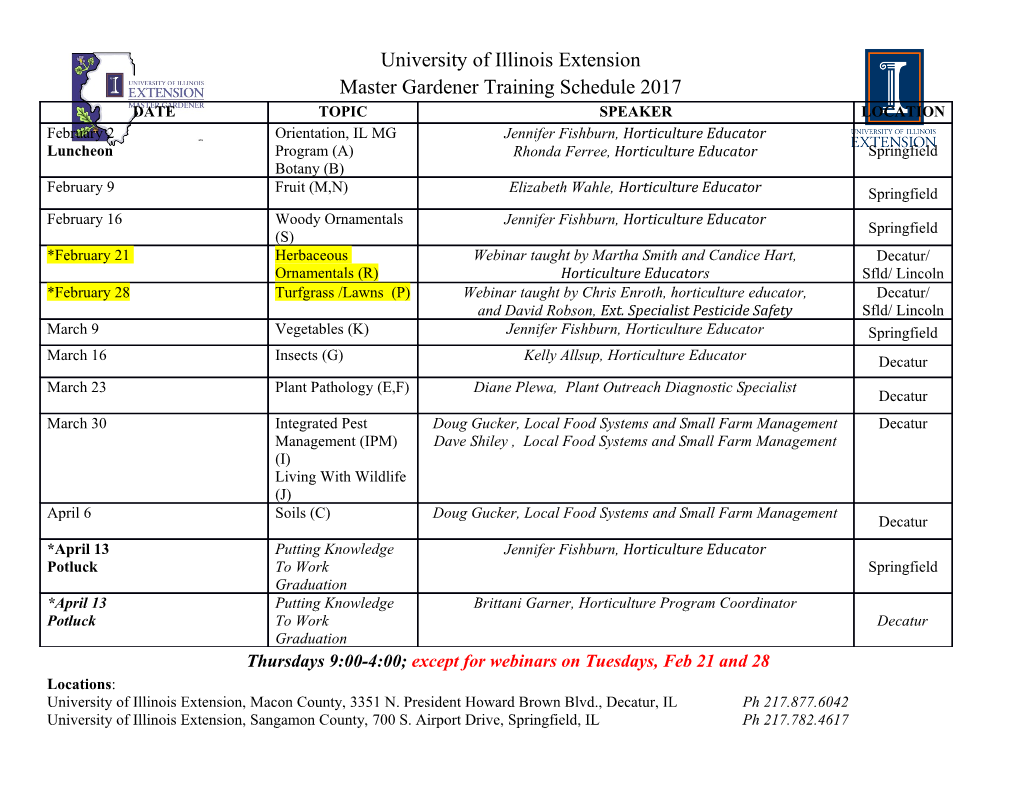
Advanced 3D Game Programming with DirectX® 10.0 Peter Walsh Wordware Publishing, Inc. Library of Congress Cataloging-in-Publication Data Walsh, Peter, 1980- Advanced 3D game programming with DirectX 10.0 / by Peter Walsh. p. cm. Includes index. ISBN 10: 1-59822-054-3 ISBN 13: 978-1-59822-054-4 1. Computer games--Programming. 2. DirectX. I. Title. QA76.76.C672W3823 2007 794.8'1526--dc22 2007041625 © 2008, Wordware Publishing, Inc. All Rights Reserved 1100 Summit Avenue, Suite 102 Plano, Texas 75074 No part of this book may be reproduced in any form or by any means without permission in writing from Wordware Publishing, Inc. Printed in the United States of America ISBN 10: 1-59822-054-3 ISBN 13: 978-1-59822-054-4 10987654321 0712 DirectX is a registered trademark of Microsoft Corporation in the United States and/or other counties. Other brand names and product names mentioned in this book are trademarks or service marks of their respective companies. Any omission or misuse (of any kind) of service marks or trademarks should not be regarded as intent to infringe on the property of others. The publisher recognizes and respects all marks used by companies, manufacturers, and developers as a means to distinguish their products. This book is sold as is, without warranty of any kind, either express or implied, respecting the contents of this book and any disks or programs that may accompany it, including but not limited to implied warranties for the book’s quality,performance, merchantability,or fitness for any particular purpose. Neither Wordware Publishing, Inc. nor its dealers or distributors shall be liable to the purchaser or any other person or entity with respect to any liability, loss, or damage caused or alleged to have been caused directly or indirectly by this book. All inquiries for volume purchases of this book should be addressed to Wordware Publishing, Inc., at the above address. Telephone inquiries may be made by calling: (972) 423-0090 To my wife, Lisa Peter This page intentionally left blank. Contents Acknowledgments..........................xiii Introduction..............................xv Chapter 1 Windows..........................1 AWordaboutWindows........................1 HungarianNotation..........................3 GeneralWindowsConcepts......................4 MessageHandlinginWindows.....................5 ProcessingMessages.........................6 HelloWorld—WindowsStyle.....................7 ExplainingtheCode........................11 RegisteringtheApplication...................13 InitializingtheWindow.....................13 WndProc—TheMessagePump.................17 ManipulatingWindowGeometry...................17 ImportantWindowMessages.....................20 ClassEncapsulation..........................23 COM:TheComponentObjectModel................30 Conclusion...............................33 Chapter 2 GettingStartedwithDirectX10.............35 WhatIsDirectX?...........................35 Installation...............................36 SettingUpVC++...........................36 WhatHappenedtoDirectDraw?...................39 Direct3D...............................40 2DGraphics101..........................41 Textures..............................44 ComplexTextures........................46 DescribingTextures.......................46 TheID3D10Texture2DInterface................50 TextureOperations.......................51 ModifyingtheContentsofTextures...............51 CreatingTextures........................52 ImplementingDirect3DwithcGraphicsLayer...........53 CreatingtheGraphicsLayer...................58 v Contents InitializingDirect3D........................58 Step1:CreatingaDeviceandSwapChain...........58 Step2:CreatingaRenderTargetView.............62 Step3:PuttingItAllTogether..................62 ShuttingDownDirect3D.....................64 SampleApplication:Direct3DSample................64 Conclusion...............................66 Chapter 3 InputandSound.....................67 DirectInput..............................67 Devices...............................68 ReceivingDeviceStates.....................70 CooperativeLevels.......................73 ApplicationFocusandDevices...................73 TheDirectInputObject......................74 ImplementingDirectInputwithcInputLayer..........74 AdditionstocApplication....................87 Sound.................................87 TheEssentialsofSound......................88 DirectSoundConcepts.......................89 DirectSoundBuffers.......................90 OperationsonSoundBuffers..................93 LoadingWAVFiles...........................96 ImplementingDirectSoundwithcSoundLayer..........103 CreatingtheDirectSoundObject...............103 SettingtheCooperativeLevel.................104 GrabbingthePrimaryBuffer..................105 ThecSoundClass.......................108 AdditionstocApplication...................114 Application:DirectSoundSample..................114 Conclusion..............................119 Chapter 4 3DMathFoundations..................121 Points.................................121 Thepoint3Structure.......................124 Basicpoint3Functions......................125 Assign..............................125 MagandMagSquared.....................126 Normalize...........................126 Dist...............................126 point3Operators.........................127 Addition/Subtraction......................127 Vector-ScalarMultiplication/Division..............129 VectorEquality.........................130 DotProduct..........................131 vi Contents CrossProduct.........................134 Polygons...............................135 Triangles...............................138 StripsandFans..........................139 Planes.................................141 DefiningLocalitywithRelationtoaPlane............144 Back-face Culling .........................147 ClippingLines...........................148 ClippingPolygons.........................149 ObjectRepresentations.......................153 Transformations...........................156 Matrices..............................156 Thematrix4Structure.....................166 Translation...........................168 BasicRotations.........................169 Axis-AngleRotation......................170 TheLookAtMatrix.......................172 PerspectiveProjectionMatrix.................174 InverseofaMatrix.......................174 Collision Detection with Bounding Spheres ...........175 Lighting................................178 RepresentingColor........................178 LightingModels..........................180 SpecularReflection........................182 LightTypes............................183 ParallelLights(orDirectionalLights).............183 PointLights...........................184 Spotlights............................185 ShadingModels..........................186 Lambert............................186 Gouraud............................186 Phong..............................187 BSPTrees...............................187 BSPTreeTheory.........................188 BSPTreeConstruction......................189 BSPTreeAlgorithms.......................194 SortedPolygonOrdering...................194 TestingLocalityofaPoint...................196 TestingLineSegments.....................196 BSPTreeCode..........................197 WrappingItUp............................207 Chapter 5 Artificial Intelligence ..................209 StartingPoint.............................210 Locomotion............................210 vii Contents Steering—BasicAlgorithms.....................211 Chasing..............................211 Evading..............................211 Pattern-basedAI.........................212 Steering—AdvancedAlgorithms...................213 PotentialFunctions........................214 TheGood...........................215 TheBad............................215 Application:potentialFunc...................216 PathFollowing..........................218 Groundwork..........................220 GraphTheory.........................221 UsingGraphstoFindShortestPaths..............225 Application:PathPlanner....................227 Motivation..............................230 NondeterministicFiniteAutomata(NFAs)............230 GeneticAlgorithms........................233 Rule-BasedAI...........................234 NeuralNetworks.........................235 ABasicNeuron.........................236 SimpleNeuralNetworks....................238 TrainingNeuralNetworks...................240 UsingNeuralNetworksinGames...............241 Application:NeuralNet......................241 ExtendingtheSystem........................253 Chapter 6 Multiplayer Internet Networking with UDP ......255 Terminology.............................255 Endianness............................255 NetworkModels.........................257 Protocols.............................258 Packets..............................259 Implementation1:MTUDP.....................260 DesignConsiderations......................260 ThingstoWatchOutFor.....................260 Mutexes..............................263 Threads, Monitor, and the Problem of the try/throw/catch Construction..........................264 MTUDP:TheEarlyYears.....................265 MTUDP::Startup()andMTUDP::Cleanup()..........266 MTUDP::MTUDP()andMTUDP::~MTUDP()........267 MTUDP::StartListening()....................267 MTUDP::StartSending()....................268 MTUDP::ThreadProc().....................269 MTUDP::ProcessIncomingData()...............270 viii Contents MTUDP::GetReliableData()...................271 ReliableCommunications.....................271
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages552 Page
-
File Size-