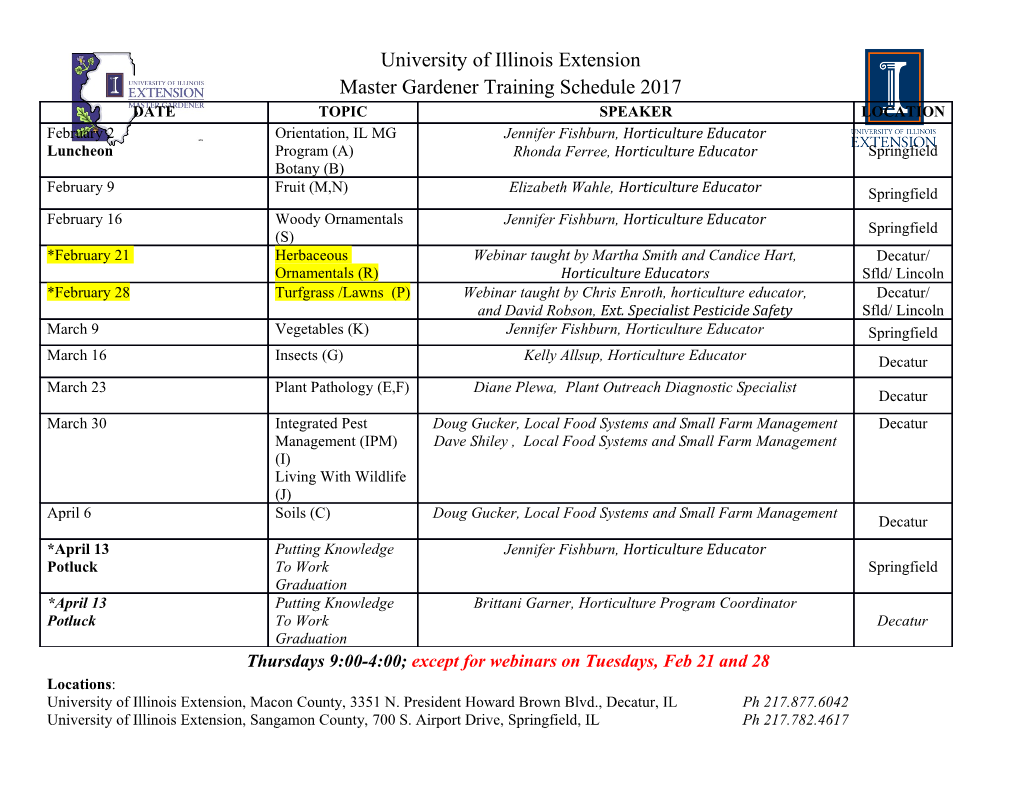
Synchronization Fall 2016 Reading • Chapter 2, Sec 2.3, “Modern Operating Systems, Fourth Ed.”, Andrew S. Tanenbaum • you may skip 2.3.9 and 2.3.10 • “POSIX Threads Programming”, available at: https:// computing.llnl.gov/tutorials/pthreads/ • Andrew D. Birrell, “An Introduction to Programming with Threads”, available at: https://birrell.org/andrew/papers/035- Threads.pdf Fall 2016 Operating Systems 2 Parallelism vs Concurrency • A parallel system can perform more than one task simultaneously • multicore, multiprocessor, GPU • A concurrent system supports more than one task by allowing all the tasks to make progress • one processor Fall 2016 Operating Systems 3 Can All Code be Parallelized? int params[10] int params[10] for(i=0;i<10;i++) for(i=0;i<10;i++) { { dosomething(params[i]); val = docomplicated(params[i],val); } } can be parallelized cannot be parallelized Fall 2016 Operating Systems 4 Outline • Interprocess Communication • Race Conditions • Critical Regions • Mutual Exclusion • Case Study • Hardware Solution • Semaphores • Mutexes and Condition Variables • More Mutual Exclusion Mechanisms Fall 2016 Operating Systems 5 Independent Threads • A thread that cannot affect or be affected by any other threads • Its state is not shared • The input state determines its result —> deterministic • Reproducible • Stop and continue without “side effects” Fall 2016 Operating Systems 6 Communication Between Processes • Sharing Storage • Main Memory • Files • Messages Fall 2016 Operating Systems 7 Cooperating Threads • These threads share state • Their behaviour is nondeterministic as it depends on relative execution of threads • Their behaviour might be irreproducible Fall 2016 Operating Systems 8 Cooperating Threads: Example 1 • x and y are shared variables between processes A and B • Their initial values are zeros Process A Process B x=1 y=2 x=y+1 y=y*2 which order is correct? Fall 2016 Operating Systems 9 Cooperating Threads: Example 2 • i is a shared variable between processes A and B Process A Process B i=0; i=0; while(i<10) while(i>-10) { { i++; i--; } } printf(“A wins”); printf(“B wins”); which order is correct? Fall 2016 Operating Systems 10 Case Study: Print Spooling Processes A and B want to access shared memory at the same time the next file to be printed processes A and B are queuing files for printing the next free slot in the directory which order is correct? Fall 2016 Operating Systems 11 Case Study:Too Much Milk Problem Person A Person B 3:00 Look in Fridge: no milk 3:05 Leave for store 3:10 Arrive at store Look in Fridge: no milk 3:15 Leave at store Leave for store 3:20 Arrive at Home, put milk away Arrive at store 3:25 Leave at store 3:30 Arrive at Home, too much milk! which order is correct? Fall 2016 Operating Systems 12 Atomic Operations • Atomic operation: can be performed entirely with no interruption • an operation that can be done entirely before the interrupts are checked • Mostly, memory load and store operations are atomic • Many of the other operations are not atomic:i++, i--,x=y+3 • Atomic operations are needed to make higher level constructs to simulate atomicity Fall 2016 Operating Systems 13 Outline • Interprocess Communication • Race Conditions • Critical Regions • Mutual Exclusion • Case Study • Hardware Solution • Semaphores • Mutexes and Condition Variables • More Mutual Exclusion Mechanisms Fall 2016 Operating Systems 14 Race Condition • Race condition: Two or more threads (processes) are reading and writing shared data and the final result depends on the relative order of executing these threads (processes) • Debugging is hard! • Some final answers are not correct Fall 2016 Operating Systems 15 Synchronization • Order of interleaving between threads: • Irrelevant: operations are independent • Relevant: operations are dependent • Any possible interleaving should result in a correct answer • Synchronization: using atomic operations to ensure correct operations of cooperating threads • Correct interleaving between threads • Controls order of execution of threads Fall 2016 Operating Systems 16 Outline • Interprocess Communication • Race Conditions • Critical Regions • Mutual Exclusion • Case Study • Hardware Solution • Semaphores • Mutexes and Condition Variables • More Mutual Exclusion Mechanisms Fall 2016 Operating Systems 17 Critical Region (Critical Section) • Critical Region: a section of code (several operations) in which only one thread may be executing at a given time • shared memory is (or other shared resources are) accessed in this section of the code • the objective is that this section be executed atomically with no interruptions —> no two threads are executing in this section at the same time • Mutual exclusion: a mechanism used to ensure that only one thread is executing in a critical section • typically done through locking mechanism, for example, leave a note on the refrigerator before shopping for milk Fall 2016 Operating Systems 18 Example: Critical Region Solution 1 Solution 2 Solution 3 1 Enter_Critical_Section if (milk == 0) { Enter_Critical_Section 2 if (milk == 0) { Enter_Critical_Section if (milk == 0) { 3 buyMilk() buyMilk() Exit_Critical_Section 4 } Exit_Critical_Section …………… 5 Exit_Critical_Section } Enter_Critical_Section 6 buyMilk() Exit_Critical_Section } Fall 2016 Operating Systems 19 Example: Critical Region Solution 1 Solution 2 Solution 3 1 Enter_Critical_Section if (milk == 0) { Enter_Critical_Section 2 if (milk == 0) { Enter_Critical_Section if (milk == 0) { 3 buyMilk() buyMilk() Exit_Critical_Section 4 } Exit_Critical_Section …………… 5 Exit_Critical_Section } Enter_Critical_Section 6 buyMilk() Exit_Critical_Section } Fall 2016 Operating Systems 20 Conditions for a Good Mutual Exclusion Solution • No two processes may be simultaneously inside their critical regions • No assumptions may be made about speeds or the number of CPUs • No process running outside its critical region may block any process • No process should have to wait forever to enter its critical region Fall 2016 Operating Systems 21 Summary • Atomic operation: can be performed entirely with no interruption • Race condition: Final result depend on the relative order of executing threads • Critical Section: a section of code in which only one thread may be executing at a given time • Mutual exclusion: a mechanism used to create critical section • Synchronization: using atomic operations to ensure correct operations of cooperating threads Fall 2016 Operating Systems 22 Outline • Interprocess Communication • Race Conditions • Critical Regions • Mutual Exclusion • Case Study • Hardware Solution • Semaphores • Mutexes and Condition Variables • More Mutual Exclusion Mechanisms Fall 2016 Operating Systems 23 Mutual Exclusion Mechanisms • Hardware: • Disable interrupts • Special instructions busy waiting • Software: • Peterson’s solution • Semaphores • Mutex • Monitors • Message passing Fall 2016 Operating Systems 24 Revisiting the Too Much Milk Problem Person A Person B 3:00 Look in Fridge: no milk 3:05 Leave for store 3:10 Arrive at store Look in Fridge: no milk 3:15 Leave at store Leave for store 3:20 Arrive at Home, put milk away Arrive at store 3:25 Leave at store 3:30 Arrive at Home, too much milk! Fall 2016 Operating Systems 25 Too Much Milk Problem — Attempt 1 Person A & B 1 if (milk == 0) { 2 if (note == 0) { 3 note = 1 4 buyMilk() 5 note = 0 6 } 7 } Fall 2016 Operating Systems 26 Lock Variables • Use a shared (lock) variable, initially 0 • When a process wants to enter its critical region • it tests the lock • if the lock is 0, set the lock to 1 and enters the critical region • if the lock is 1, wait until it becomes 0 • Therefore, 0 means that no process is in its critical region, and 1 means that a process is in its critical region • Does this work? Fall 2016 Operating Systems 27 Too Much Milk Problem — Attempt 2 Person A Person B 1 while (note == 0) { while (note == 1) { 2 if (milk == 0) { if (milk == 0) { 3 buyMilk() buyMilk() 4 } } 5 note = 1 note = 0 6 } } Note meaning: A buys if no note, B buys if there is a note. Fall 2016 Operating Systems 28 Strict Alternation • Use an integer variable turn, initially 0, keeps track of whose turn it is to enter the critical region • Procesdure: • process 0 inspects turn, finds it to be 0, and enters its critical region • process 1 finds turn to be 0, it continues to wait until it changes to 1 • A process changes the value of turn before leaving the critical section • Advantage: avoids races • Disadvantage: violates condition 3 • Note: spin lock is a lock that uses busy waiting Fall 2016 Operating Systems 29 Too Much Milk Problem — Attempt 3 Person A Person B 1 noteA = 1 noteB = 1 2 if (noteB == 0) { if (noteA == 0) { 3 if (milk == 0) { if (milk == 0) { 4 buyMilk() buyMilk() 5 } } 6 } } 7 noteA = 0 noteB = 0 Use separate notes for A and B Fall 2016 Operating Systems 30 Too Much Milk Problem — Attempt 4 Person A Person B 1 noteA = 1 noteB = 1 2 if (noteB == 0) { while (noteA == 1) { 3 if (milk == 0) { //do nothing 4 buyMilk() } 5 } if (milk == 0) { 6 } buyMilk() 7 noteA = 0 } noteB = 0 Fall 2016 Operating Systems 31 Too Much Milk Problem — Attempt 4 — Comments • Works — hurray ! • Disadvantages: • Asymmetric solution • Involves busy-waiting (Continuously testing a variable until some value appears) Fall 2016 Operating Systems 32 Peterson’s Algorithm Credit: Andrew Tanenbaum, Modern Operating Systems: 4th ED Fall 2016 Operating Systems 33 Outline • Interprocess Communication • Race Conditions • Critical Regions • Mutual Exclusion • Hardware Solution • Case
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages83 Page
-
File Size-