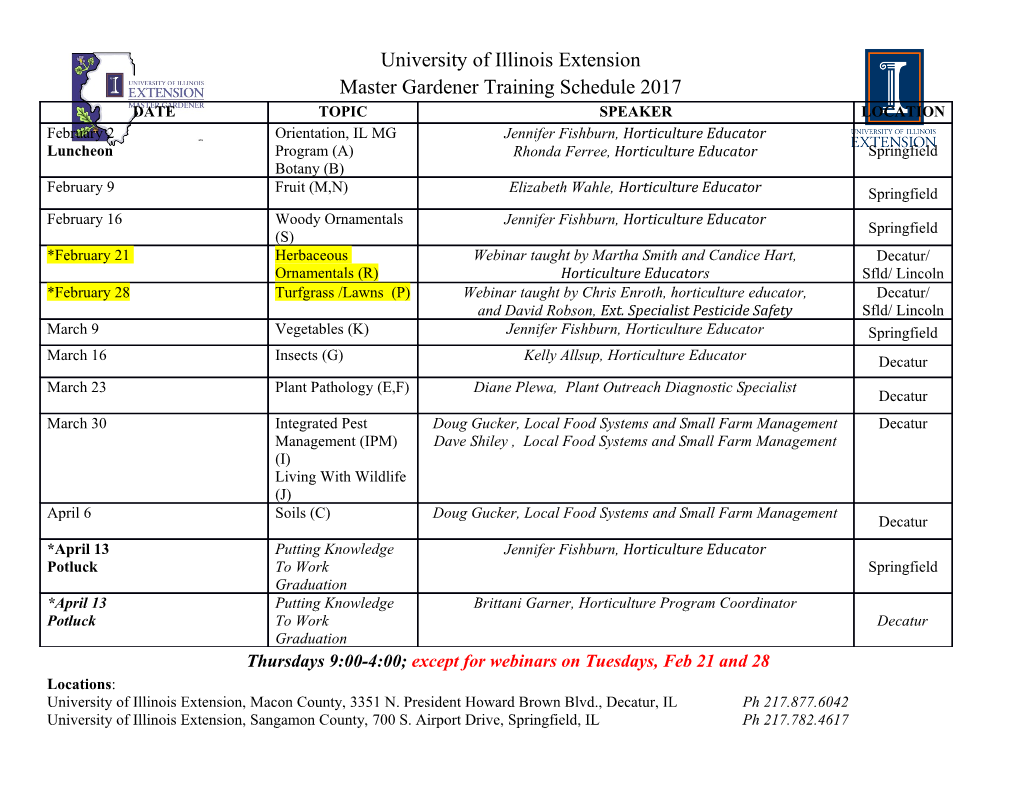
Lecture 8 • Template classes • Introduction to the Standard Template Library (STL) –Containers • vector • map –Iterators – Algorithms Lec 5 Programming in C++ 1 Class Templates • Class templates •Specify entire range of related classes •Generic programming – Class-template specializations •Example: Stack See tstack1.h •LIFO (last-in-first-out) structure •Describe notion of stack generically •Instantiate type-specific version template< class T > Class Stack { … } //end class stack Lec 5 Programming in C++ 2 1 Class Templates • Member functions (methods) template< class T > bool Stack< T >::push(const T &value) {…} Binds function definition to the class template • To create a stack of doubles Stack< double > doubleStack(5); doubleStack.push(5.6); See Fig11_03.cpp Lec 5 Programming in C++ 3 Class Templates and Nontype Parameters template< class T = String, int elements > class Stack { private: int size; Default type int top; T stackHolder[elements]; public: … } //end class stack Stack< double, 100 > myStack; Think: What is a advantage of this solution? Lec 5 Programming in C++ 4 2 Standard Template Library • Powerful, template-based components • Containers: template data structures – Objects that contain other objects • Iterators: like “pointers”, cycle through the contents of a container • Algorithms: manipulate containers, searching, sorting, etc. •Code reuse – Templates are used •Performance • STL specification requires that containers and algorithms be implemented in a way that ensures optimal run time performance. Lec 5 Programming in C++ 5 Containers • Several types of containers – Sequence containers •Linear data structures –Dynamic array <vector> – Linear list <list> – Associative containers •Store key/value pairs •Retrieve a value given its key – Container adapters • Transform the functionality of a container • A container automatically grows when elements are added and shrinks when elements are removed. Lec 5 Programming in C++ 6 3 Containers • Sequence containers – vector A dynamic array – deque A double-ended queue – list A linear list • Associative containers – set no duplicates – multiset duplicates allowed – map Associates one value for each key – multimap Associates multiple values for each key •Container adapters – stack – queue template <class T, class Container = dequee<T>> class queue – priority_queue Lec 5 Programming in C++ 7 vector Sequence Container • vector // vector class-template definition #include <vector> – Data structure with contiguous memory locations •Access elements with [] – Use when data is accessed randomly • When memory exhausted – Allocates larger, contiguous area of memory – Copies itself there –Deallocatesold memory • Has random access iterators Lec 5 Programming in C++ 8 4 vector Sequence Container • Declarations vector<int> v1; //create a zero-length int vector vector<Point> v2(20); vector<char> v3(5, ‘x’); vector<int> v4(v1); • Subscripting [] v3[6] = ‘b’; //Error!! – Access out of bounds (silent error) – Will not increase vector size • Expanding the vector v1.push_back(8); //memory is allocated cout << v1.size();// display number of elements stored v1.pop_back(); Lec 5 Programming in C++ 9 Vector Example main(){ vector<string> SS; SS.push_back("The number is 10"); SS.push_back("The number is 20"); SS.push_back("The number is 30"); cout << "Loop by index:" << endl; int ii; for(ii=0; ii < SS.size(); ii++) { cout << SS[ii] << endl; } return 0; } Lec 5 Programming in C++ 10 5 Iterators • Iterators similar to pointers – Iterator operators same for all containers • * dereferences • ++ points to next element – Create an iterator given a container c • c.begin() returns iterator to first element • c.end() returns iterator to after last element – Container adapters do not support iterators Lec 5 Programming in C++ 11 Iterator Categories • Input – Retrieve, but not store values. Forward moving only. • Output – Store, but not retrieve. Forward moving only. • Forward – Store and retrieve values. Forward moving only. • Bidirectional – Store and retrieve values. Forward and backward moving. • Random access – Store and retrieve values. Elements can be accessed randomly. Lec 5 Programming in C++ 12 6 Iterator Operations • All – ++p, p++ • Input, bidirectional, and random iterators – *p – p = p1 – p == p1, p != p1 • Output iterators – *p = … – p = p1 • Input iterators – x = *p – p = p1 – p-> – p == p1, p != p1 Lec 5 Programming in C++ 13 Iterator Operations • Bidirectional – --p, p-- • Random access – p + i, p += i – p - i, p -= i – p[i] – p < p1, p <= p1 – p > p1, p >= p1 Lec 5 Programming in C++ 14 7 Iterators Example main(){ vector<string> SS; //add elements to SS vector<string>::const_iterator cii; for(cii=SS.begin(); cii!=SS.end(); cii++) { cout << *cii << endl; } //Reverse Iterator vector<string>::reverse_iterator rii; for(rii=SS.rbegin() ; rii!=SS.rend() ; ++rii) { cout << *rii << endl; } return 0; } Lec 5 Programming in C++ 15 Const and reverse Iterators • Iterators std::vector<type>::const_iterator iterVar; • const_iterator cannot modify elements std::vector<type>::reverse_iterator iterVar; •Visits elements in reverse order (end to beginning) •Use iterVar.rbegin() to get starting point •Use iterVar.rend() to get ending point Lec 5 Programming in C++ 16 8 vector Sequence Container • vector functions – v.push_back(value) • Add element to end (found in all sequence containers). – v.size() • Current number of elements stored in the vector – v.capacity() • How much vector can hold before reallocating memory – vector<type> v(a, a + SIZE) •Creates vector v with elements from array a up to (not including) a + SIZE Lec 5 Programming in C++ 17 vector Sequence Container • vector functions – v.insert(iterator, value ) •Inserts value before location of iterator – v.insert(iterator, array , array + SIZE) • Inserts array elements (up to, but not including array + SIZE) into vector before iterator – v.erase( iterator ) • Remove element pointed by iterator from container – v.erase( iter1, iter2 ) • Remove elements starting from iter1 and up to (not including) iter2 – v.clear() • Erases entire container Lec 5 Programming in C++ 18 9 vector Sequence Container • vector functions – v.front(), v.back() • Return a reference to the first and last element – v[index] = value; • Assign value to an element – v.at(index) = value; •As above, with range checking • out_of_bounds exception See Fig21_14.cpp Lec 5 Programming in C++ 19 Common STL typedefs • typedefs for first-class containers – const_reference – reference – pointer vector<int> v(10); – iterator vector<int>::reference ref = v.begin(); – const_iterator – reverse_iterator – const_reverse_iterator – value_type – … Lec 5 Programming in C++ 20 10 Algorithms • STL has algorithms used generically across containers – Operate on elements indirectly via iterators • #include <algorithm> – Often operate on a range of elements •Defined by pairs of iterators – Algorithms often return iterators • find() •Returns iterator to element, or end() if not found – Pre-made algorithms save programmers time and effort See Fig21_15.cpp Lec 5 Programming in C++ 21 Algorithms Example bool even(int n) { return (n % 2? false: true); } int main(){ int array[6] = { 1, 2, 3, 4, 5, 6 }; vector<int> b(array, array+6); int c = count_if(b.begin(), b.end(), even); cout << "Number of evens: " << c << endl; vector<int> x(5, 0); // It only copies values 1,2,3,4 copy(b.begin(), b.end(), ++x.begin()); reverse(x.begin(), x.end()); vector<int>::iterator ptr; for(ptr = x.begin(); ptr != x.end(); ++ptr ) cout << *ptr << ' '; // Output looks like: 4 3 2 1 0 } Lec 5 Programming in C++ 22 11 Types of Algorithms •Copying • Searching unsorted sequences • Replacing and removing elements • Reordering a sequence •Sorting • Sorted Sequence Searching • Merging sorted sequences • Set operations • Heap operations • Minimum and maximum •Permutations • Miscellaneous Lec 5 Programming in C++ 23 Stream Iterators • Possible to view I/O streams as another type of container • #include <iterator> • Input streams: istream_iterator • To read values into the sequence in a type safe manner • Operators: ->, *, ++ • Output streams: ostream_iterator • To write values into the sequence in a type safe manner • Operators: =, *, ++ Lec 5 Programming in C++ 24 12 istream_iterator std::istream_iterator<int> inputInt(cin) •Can read input from cin • *inputInt – Dereference to read first int from cin • ++inputInt –Go to next int in stream Lec 5 Programming in C++ 25 istream_iterator and Algorithms int main() { vector<int> v(5); cout << "Enter 5 integers." << endl; istream_iterator<int> int_itr(cin); copy(int_itr, istream_iterator<int>(), v.begin()); for(int i = 0; i < v.size(); i++) cout << v[i] << " "; } End-of-stream: – istream_iterator<int>() –End-of-file (ctrl-Z) for cin Lec 5 Programming in C++ 26 13 ostream_iterator std::ostream_iterator<int> outputInt(cout) •Can output intsto cout • *outputInt = 7 –Outputs 7 to cout • ++outputInt – Advances iterator so we can output next int Lec 5 Programming in C++ 27 ostream_iterator and Algorithms std::vector<int> v; std::vector<int>::iterator it; std::ostream_iterator<int> output(cout, " " ); for(it = v.begin(); it != v.end(); ++it) cout << *it << endl; std::copy(v.begin(), v.end(), output ); Lec 5 Programming in C++ 28 14 Usage of ostream_iterator • ostream_iterator – std::ostream_iterator< type > Name( outputStream, separator ); • type: outputs values of a certain type • outputStream: iterator output location • separator: character separating outputs •Example std::ostream_iterator<int> output(cout,
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages16 Page
-
File Size-