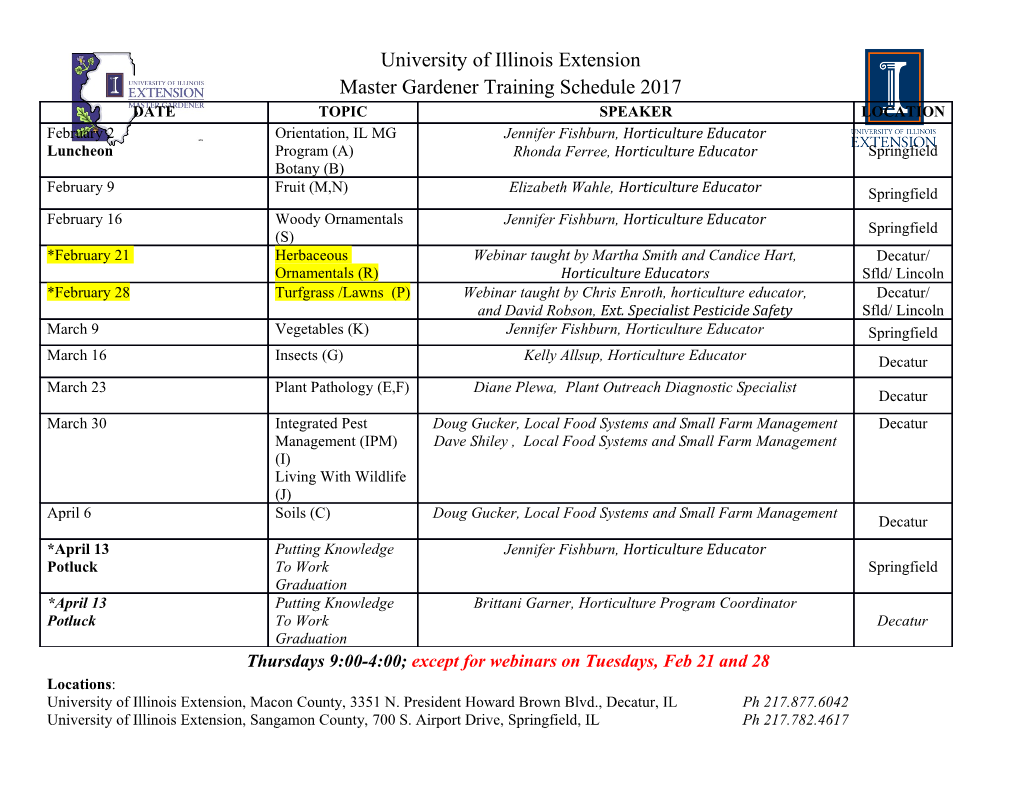
Expressions & I/O 2.14 Arithmetic Operators l An operator is a symbol that tells the computer Unit 2 to perform specific mathematical or logical manipulations (called operations). Sections 2.14, 3.1-10, 5.1, 5.11 l An operand is a value used with an operator to CS 1428 perform an operation. Fall 2017 l C++ has unary and binary operators: Jill Seaman ‣ unary (1 operand) -5 ‣ binary (2 operands) 13 - 7 1 2 Arithmetic Operators Integer Division l Unary operators: • If both operands are integers, / (division) operator always performs integer division. SYMBOL OPERATION EXAMPLES The fractional part is lost!! + unary plus +10, +y - negation -5, -x cout << 13 / 5; // displays 2 cout << 91 / 7; // displays 13 l Binary operators: SYMBOL OPERATION EXAMPLE • If either operand is floating point, the result is + addition x + y floating point. - subtraction index - 1 * multiplication hours * rate cout << 13 / 5.0; // displays 2.6 / division total / count cout << 91.0 / 7; // displays 13 % modulus count % 3 3 4 Modulus 3.1 The cin Object • % (modulus) operator computes the l cin: short for “console input” remainder resulting from integer division ‣ a stream object: represents the contents of the screen that are entered/typed by the user using the keyboard. cout << 13 % 5; // displays 3 ‣ requires iostream library to be included cout << 91 % 7; // displays 0 l >>: the stream extraction operator ‣ use it to read data from cin (entered via the keyboard) • % requires integers for both operands cin >> height; cout << 13 % 5.0; // error ‣ when this instruction is executed, it waits for the user to type, it reads the characters until space or enter (newline) is typed, cout << 91.0 % 7; // error then it stores the value in the variable. ‣ right-hand operand MUST be a variable. 5 6 Console Input Example program using cin #include <iostream> • Output a prompt (using cout) to tell the user using namespace std; what type of data to enter BEFORE using cin: int main() { float diameter; int length, width, area; cout << "This program calculates the area of a "; cout << “What is the diameter of the circle? ”; cout << "rectangle.\n"; cin >> diameter; cout << "Enter the length and width of the rectangle "; cout << "separated by a space.\n"; cin >> length >> width; • You can input multiple values in one statement: area = length * width; cout << "The area of the rectangle is " << area << endl; int x, y; return 0; cout << “Enter two integers: “ << endl; } cin >> x >> y; This program calculates the area of a rectangle. Enter the length and width of the rectangle ‣ the user may enter them on one line (separated by a space) output screen: separated by a space. or on separate lines. 7 10 20 8 The area of the rectangle is 200 3.2 Mathematical Expressions Where can expressions occur? • An expression is a program component that • The rhs (right-hand-side) of an assignment evaluates to a value. statement: • An expression can be x = y * 10 / 3; y = 8; – a literal, x = y; – a variable, or aLetter = 'W'; – a combination of these using operators and parentheses. num = num + 1; • Examples: • The rhs of a stream insertion operator (<<) (cout): 4 x * y / z num 'A' cout << “The pay is “ << hours * rate << endl; x + 5 -15e10 8 * x * x – 16 * x + 3 2 * (l + w) cout << num; cout << 25 / y; • Each expression has a type, which is the data type of the result value. • More places we don’t know about yet . 9 10 Operator Precedence Parentheses (order of operations) • Which operation does the computer perform first? l You can use parentheses to override the answer = 1 + x + z; precedence or associativity rules: result = x + 5 * y; a + b / 4 • Precedence Rules specify which happens first, in (a + b) / 4 this order: (4 * 17) + (3 – 1) - (negation) a – (b - c) * / % + - • If the expression has multiple operators from the l Some examples: same level, they associate left to right or right to 5 + 2 * 4 left: 10 / 2 - 3 - (negation) Right to left 8 + 12 * 2 - 4 * / % Left to right 4 + 17 % 2 -1 + - Left to right 6 - 3 * 5 / 2 - 1 11 12 Exponents 3.3 Type Conversion • There is no operator for exponentiation in C++ l The computer (ALU) cannot perform operations • There is a library function called “pow” between operands of different data types. y = pow(x, 3.0); // x to the third power l If the operands of an expression have different types, the compiler will convert one to be the • The expression pow(x,3.0) is a “call to the pow type of the other function with arguments x and 3.0”. • Arguments can have type double or int and the l This is called an implicit type conversion, or a result is a double. type coercion. Order of types: double • If x is 2.0, then 8.0 will be stored in y. l Usually, the operand with the The value stored in x is not changed. lower ranking type is converted to float the type of the higher one. long • #include <cmath> is required to use pow. int 13 14 char Type Conversion Rules 3.5 Type Casting • Binary ops: convert the operand with the lower • Type casting is an explicit (or manual) type ranking type to the type of the other operand. conversion. int years; Always safe y = static_cast<int>(x); // converts x to int float interestRate, result; . result = years * interestRate; • mainly used to force floating-point division // years is converted to float before being multiplied int hits, atBats; float battingAvg; Result: • Assignment ops: rhs is converted to the type of . 0.375 cin >> hits >> atBats; // assume: 3 8 the variable on the lhs. battingAvg = static_cast<float>(hits)/atBats; int x, y = 4; Not always safe, OUTPUT: float z = 2.7; information loss x = 4 * z; 10 • why not: //4 is converted to float, ? battingAvg = static_cast<float>(hits/atBats); //then 10.8 is converted to int (10) cout << x << endl; 15 16 3.4 Overflow/Underflow 3.6 Multiple Assignment • Happens when the value assigned to a variable • You can assign the same value to several is too large or small for its type (out of range). variables in one statement: • integers tend to wrap around, without warning: a = b = c = 12; short testVar = 32767; cout << testVar << endl; // 32767, max value testVar = testVar + 1; cout << testVar << endl; //-32768, min value • is equivalent to: • floating point value overflow/underflow: a = 12; b = 12; ‣ may or may not get a warning c = 12; ‣ result may be 0 or random value 17 18 3.6 Combined Assignment 5.1 Increment and Decrement • Assignment statements often have this form: • C++ provides unary operators to increment and number = number + 1; //add 1 to number decrement. total = total + x; //add x to total ‣ Increment operator: ++ y = y / 2; //divide y by 2 ‣ Decrement operator: -- int number = 10; • can be used before (prefix) or after (postfix) a number = number + 1; cout << number << endl; variable • C/C++ offers shorthand for these: • Examples: int num = 10; number += 1; // short for number = number+1; num++; //equivalent to: num = num + 1; total -= x; // short for total = total-x; num--; //equivalent to: num = num - 1; y /= 2; // short for y = y / 2; ++num; //equivalent to: num = num + 1; --num; //equivalent to: num = num - 1; 19 20 Prefix vs Postfix 3.9 More Math Library Functions • ++ and -- operators can be used in expressions • These require cmath header file • In prefix mode (++val, --val) the operator increments or decrements, then returns the new • These take double argument, return a double value of the variable • Commonly used functions: • In postfix mode (val++, val--) the operator returns the original value of the variable, then increments pow y = pow(x,d); returns x raised to the power d or decrements abs y = abs(x); returns absolute value of x sqrt y = sqrt(x); returns square root of x int num, val = 12; ceil y = ceil(x); returns the smallest integer >= x cout << val++; // cout << val; val = val+1; cout << ++val; // val = val + 1; cout << val; sin y = sin(x); returns the sine of x (in radians) num = --val; // val = val - 1; num = val; num = val--; // num = val; val = val -1; etc. It’s confusing, don’t do this! 21 22 3.10 Hand Tracing a Program 3.7 Formatting Output • You be the computer. Track the values of the variables as the program executes. l Formatting: the way a value is printed: ‣ step through and ‘execute’ each statement, one-by-one ‣ spacing ‣ record the contents of variables after each statement ‣ decimal points, fractional values, number of digits execution, using a hand trace chart (table) or boxes. ‣ scientific notation or decimal format int main() { num1 num2 double num1, num2; l cout << “Enter first number”; ? ? cout has a standard way of formatting values cin >> num1; ? ? cout << “Enter second number”; 10 ? of each data type cin >> num2; 10 ? l num1 = (num1 + num2) / 2; 10 20 use “stream manipulators” to override this num2++; 15 20 l 15 21 they require #include <iomanip> cout << “num1 is ” << num1 << endl; 15 21 cout << “num2 is “ << num2 << endl; } 15 21 23 24 Formatting Output: setw setw: examples • setw is a “stream manipulator”, like endl • Example with no formatting: • cout << 2897 << “ “ << 5 << “ “ << 837 << endl; setw(n)specifies the minimum width for the cout << 34 << “ “ << 7 << “ “ << 1623 << endl; next item to be output 2897 5 837 Prog 3-12 output in 34 7 1623 the book is WRONG ‣ cout << setw(6) << age << endl; ‣ print in a field at least 6 spaces wide. • Example using setw: ‣ value is right justified (padded with spaces on left). cout << setw(6) << 2897 << setw(6) << 5 << setw(6) << 837 << endl; ‣ if the value is too big to fit in 6 spaces, it is printed in full, cout << setw(6) << 34 << setw(6) << 7 using more positions.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages11 Page
-
File Size-