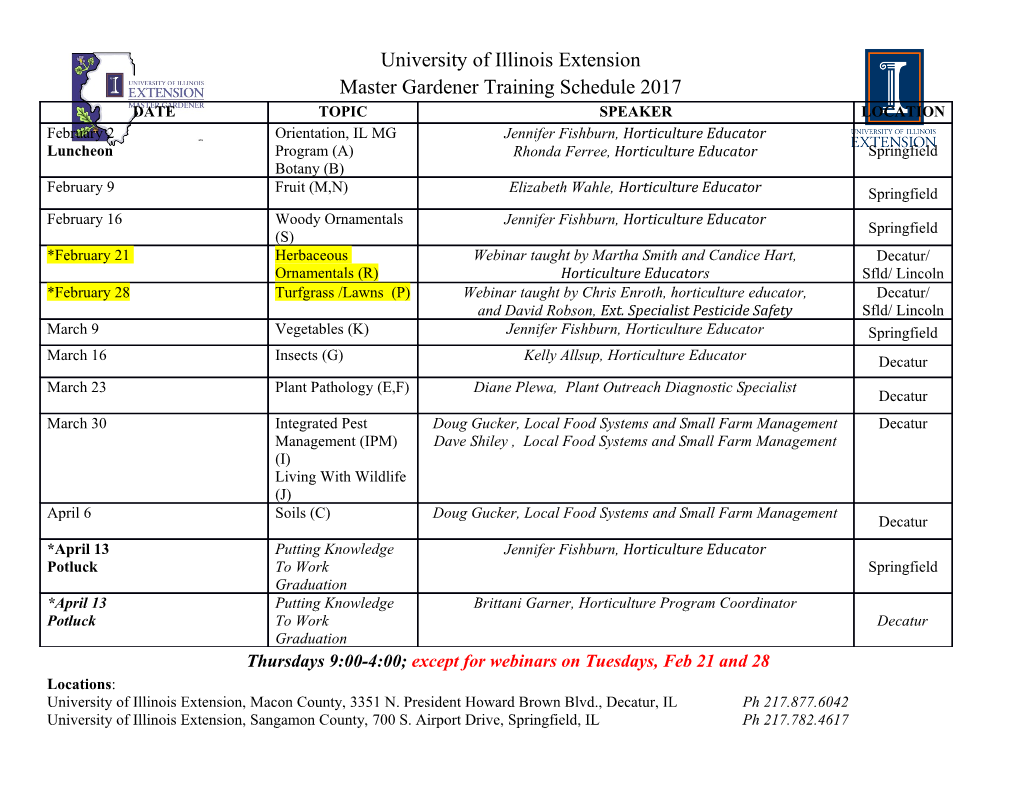
CS162: Operating Systems and Systems Programming Lecture 19: Networking: Sockets/TCP/ P 22 Ju"y 201$ Char"es &eiss %ttps://cs162.eecs.berkeley'edu/ Recal": Echo client-ser+er code void client(int sockfd) { int n; char sndbuf[MAXIN]; char rcvbuf[MAXOUT]; while (getreq(sndbuf, MAXIN)) { /* prompt + read */ write(sockfd, sndbuf, strlen(sndbuf)); /* send */ n=read(sockfd, rcvbuf, MAXOUT-1); /* receive */ if (n <= 0) return; /* handle error or EOF */ write(STDOUT_FILENO, rcvbuf, n); /* echo */ 3 3 void server(int consockfd) { int n; char reqbuf[MAXREQ]; while (1) { n = read(consockfd,reqbuf,MAXREQ-1); /* Recv */ if (n <= 0) return; /* handle error or EOF */ n = write(STDOUT_FILENO, reqbuf, strlen(reqbuf)); n = write(consockfd, reqbuf, strlen(reqbuf)); /* echo */ 3 2 3 Recal": Socket Setup o+er TCP/ P Server Socket new socket socket connection socket Client Server Specia" kind o- socket: server socket ― .as fi"e descriptor ― Can0t read or write Two operations: ― "isten1): start a""owing c"ients to connect ― accept(): create a new socket -or a particu"ar c"ient , Recal": Client Protocol char *hostname; char *portname; int sockfd; struct addrinfo *server; server = buildServerAddr(hostname, portname); /* Create a T P socket */ /* server-$ai%famil&: A(%)*+! ()Pv,) or A(%)*+!6 ()"v6) */ /* server-$ai%sockt&pe' S. /%ST0EA1 (byte-oriented) */ /* server-$ai%protocol' I""0.!.%! " */ sockfd = socket(server#>ai_family, server#$ai_socktype, server#$ai_protocol) /* Connect to server on port */ connect(sockfd, server#$ai_addr, server#>ai_addrlen); /* Carr& out lient#Server protocol */ client(sockfd); /* Clean up on termination */ close(sockfd); 3 freeaddrinfo(server); Recal": Server Protocol 1+12 /* Create Socket to receive requests*/ lstnsockfd = socket(server->ai_family, server->ai_socktype, server->ai_protocol); /* Bind socket to port */ bind(lstnsockfd, server->ai_addr, server->ai_addrlen); while (1) { /* Listen for incoming connections */ listen(lstnsockfd, MAXQUEUE); /* Accept incoming connection, obtaining a new socket for it */ consockfd = accept(lstnsockfd, NULL, NULL); server(consockfd); close(consockfd); : close(lstnsockfd); $ .andling mu"tiple connectoins One option – fork a process for each connection ― Strong iso"ation between each connection ― Can accept new connections while other connections are active Second option – spawn a t%read -or each connection T%ird option – event-sty"e (later2 6 Process Per Connection 1One at a Time) Client Server Create Server Socket Create C"ient Socket 7ind it to an Address (%ost:port2 Connect it to server (host:port2 Listen for Connection Child 8ccept connection Parent C"ose Listen Socket write re6uest read request C"ose Connection Socket read response write response C"ose C"ient Socket C"ose Conn Socket 5 Server Protocol (+,2 while (1) { listen(lstnsockfd, 1AX89+9E); consockfd = accept(lstnsockfd, *966, *966); cpid = fork(); /* ne3 process for connection */ if (cpid > 0) { /* parent process */ close(consockfd); //tcpid = wait(&cstatus); /* Ignore SIGCHLD instead? */ : else if (cpid == ;) 5 /* child process */ close(lstnsockfd); /* let go of listen socket */ server(consockfd); close(consockfd); exit(EXIT_SUCCESS); /* exit child normally */ : : 9 Client: getting the ser+er address struct addrinfo *buildServerAddr(char *hostname, char *portname) 5 struct addrinfo *result; struct addrinfo hints; int rv; memset(&hints, 0, sizeof(hints)); /* Clean unused hints */ hints.ai_family = AF_UNSPEC; /* IPv4 or IPv6 */ hints.ai_socktype = SOCK_STREAM; /* Stream socket – TCP / byte-oriented */ rv = getaddrinfo(hostname, portname, &hints, &result); if (rv != 0) { /* handle error */ : return result; : /* Later freeaddrinfo(result) */ 9 Server address: with getaddrin-o struct addrinfo *server; struct addrinfo hints; int rv; memset(&hints, 0, sizeof(hints)); /* Clean unused hints */ hints.ai_family = AF_UNSPEC; /* IPv4 or IPv6 */ hints.ai_socktype = SOCK_STREAM; /* Stream socket – TCP / byte-oriented */ hints.ai_flags = AI_PASSIVE; /* for listening */ rv = getaddrinfo(NULL /* hostname */, portname, &hints, &result); if (rv != 0) { /* handle error */ : /* Later freeaddrinfo(result) */ 10 Server address: manually 1 Pv32 int port_number = …; struct addrinfo server; struct sockaddr_in server_ip_port; server_ip_port.sin_family = AF_INET; /* IPv4 */ server_ip_port.sin_addr.s_addr = INADDR_ANY; /* 0.0.0.0 – means all addresses available on the machine */ server_ip_port.sin_port = htons(port_number); /* htons → host to "network" (Big Endian) order short */ server.ai_addr = (struct sockaddr*) &server_ip_port; server.ai_addrlen = sizeof(struct sockaddr_in); server.ai_family = AF_INET; server.ai_socktype = SOCK_STREAM; /* byte-oriented */ server.ai_protocol = IPPROTO_TCP; /* or 0 – "choose any" */ 11 Recal": Peer-to-Peer Communication No always-on ser+er at the center of it a"" ― .osts can come and go, and change addresses ― .osts may have a di;erent address each time )<amp"e: peer-to-peer /"e s%aring (e'g.: 7itTorrent) ― 8ny host can re6uest files, send files, 6uery to find where a file is located, respond to 6ueries: and forward 6ueries ― Scalability by harnessing millions o- peers ― )ach peer acting as both a client and server 12 Networking Definitions Network: physical connection that al"ows two computers to communicate Frame/Packet/Segment: unit of transfer, sequence of bits carried o+er the network ― Network carries packets from one CPU to another ― =estination gets interrupt when frame arrives ― Name depends on what layer 1later2 Protocol: agreement between two parties as to %ow information is to be transmitted 13 The Problem (1) Many different applications ― email, we(, P2P, etc' Many different network sty"es and techno"ogies ― @ireless vs. wired vs. optical, etc' .ow do we organize this mess? 14 The Problem (2) Application Skype SSH NFS HTTP Transmission Coaxial Fiber Packet Media cable optic Radio Re-implement e+ery application for e+ery technologyB NoC 15 The Problem (,2 Application Skype SSH NFS HTTP Sockets UDP/IPv4 UDP/IPv6 TCP/IPv4 TCP/IPv6 Transmission Coaxial Fiber Packet Media cable optic Radio Re-implement e+ery type of sockets for every technologyB No' 16 Layering Comple< ser+ices from simpler ones TCP/IP networking layers: ― Physical + Link (@ireless, Ethernet, ..'2 ● Unrelia("e, loca" e<c%ange o- limited-siAed frames ― Network 1IP2 4 routing (etween networks ● Unrelia("e, glo(a" e<c%ange o- limited*siAed packets ― Transport (TCP, >=P) – routing ● &elia("ity, streams o- bytes instead o- packets, … ― 8pplication – everything on top o- sockets 17 Network Protocols Protoco": Agreement between two parties as to %ow information is to be transmitted Protoco"s on today’s Internet: e%mail NFS $$$ ssh RPC Transport !"P TCP Network P Physical/'ink Ethernet ATM Packet radio 18 Layering Comple< ser+ices from simpler ones TCP/IP networking layers: ― Physical + Link ($ireless, Ethernet, .++, ● Unrelia("e, loca" e<c%ange o- limited-siAed frames ― Network 1IP2 4 routing (etween networks ● Unrelia("e, glo(a" e<c%ange o- limited*siAed packets ― Transport (TCP, >=P) – glue ● &elia(i"ity (retry), ordering, streams o- bytes, … ― 8pplication – everything on top o- sockets 19 7roadcast Networks Shared Communication ?edium /- /O /- Processor "evice "evice "evice Memor& I n t e r n e t Shared Medium can be a set of wires Inside a computer, this is called a bus All devices simultaneously connected to devices 20 7roadcast Networks S%ared Communication Mechanism )<amp"es: ― Original )thernet ― 8ll types o- wireless 1@iFi, cellular, .''2 ― Coaxial ca(le 1e'g. cable internet2 21 7roadcast Networks Details 0od& Header )"ata) )"est:/, ID:1 Message (ignore) ":3 )sender, ":4 )ignore, ":/ )receive, 7uilding unicast (message to one) from (roadcast (message to a""): ― Put header on front o- packet: [ =estination | Packet ] ― =iscards i- not the target (o-ten in hardware2 22 7roadcast Network Arbitration Ar3itration: @%o can use shared medium whenB Girst examp"e: 8"o%a network 15#Fs): packet radio within Hawaii ― 7"ind (roadcast, with checks4m at end of frame' ― f two senders try to send at same time: (oth get gar("ed: (oth simp"y re*send "ater' Pro("ems: How many frames are lost? ― f network is too (usy, no one gets throug% ― Need to not re*send in sync 23 Carrier Sense, ?u"tiple Access/Col"ision Detection )thernet 1early 9#Fs2: ― Originally – shared wire, clip on to it (literally2 Carrier Sense: donFt send un"ess medium id"e ― Less good for wireless (propagation delays, colliding station mig%t be out o- range2 Co""ision =etect: sender c%ecks i- packet trampled. ― - so, abort, wait and retry 8dapti+e randomiAed backo; ― @ait a random amount of time before retransmitting ― 8+oids retransmitting at t%e same time as colliding mac%ine ― ncreasing wait times to adKust to how (usy t%e medium is 24 Point-to*point networks I n t e Switch Router r n e t No shared medium ― Strict"y simpler 4 no /ltering Switches: pro+ide shared-(us view wit% point*to*point links ― )<amines Ldestination" %eader, resends on appropriate link #o4ters: connects two networks ― =istinction (etween switc%es/routers is actua""y -uzzy.'' 25 Layering Comple< ser+ices from simpler ones TCP/IP networking layers: ― Physical + Link (@ireless, Ethernet, ..'2 ● Unrelia("e, loca" e<c%ange o- limited-siAed frames ― Network ( P, – routing between networks ● Unrelia("e, glo(a" e<c%ange o- limited*siAed packets ― Transport (TCP, >=P) – routing ● &elia("ity, streams o- bytes instead o- packets, … ― 8pplication – everything on top o- sockets 26 M"ue: Adding Gunctionality Physical Reality:
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages112 Page
-
File Size-