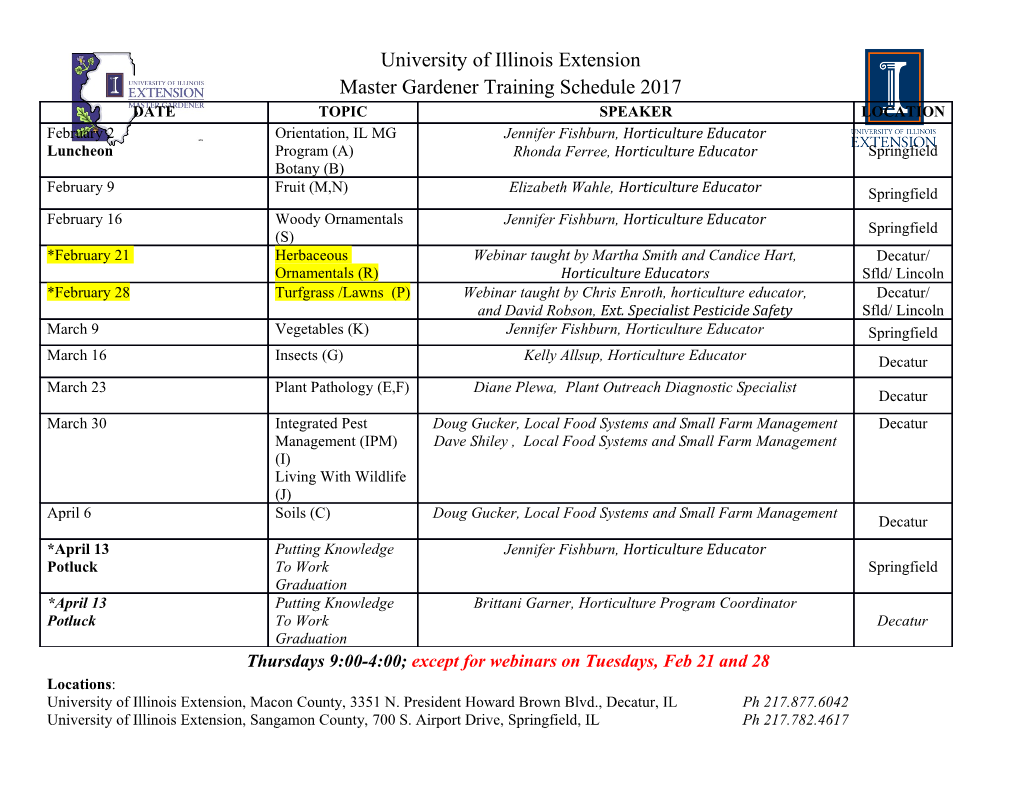
EyeLink Programmer’s Guide Version 3.0 Copyright ©2012, SR Research Ltd. Contents 1 Introduction 1 1.1 Organization of This Document ............................... 1 2 Getting Started 3 2.1 Upgrading to ELSDK with GDI graphics .......................... 3 2.2 Using ELSDK with SDL graphics .............................. 4 3 Overview of Experiments5 3.1 Outline of a Typical Windows Experiment.......................... 5 3.2 EyeLink Operation in Experiments.............................. 6 3.3 Features of the ELSDK Library................................ 7 3.4 Displays and Experiments .................................. 9 4 Programming Experiments 11 4.1 Important Programming Notes................................ 11 4.2 Programming Tools and Environment ............................ 11 4.3 Starting a New Project .................................... 12 4.4 Building a New Project.................................... 12 4.5 Planning the Experiment ................................... 21 4.6 Developing and Debugging New Experiments........................ 22 4.7 Converting Existing Experiments............................... 23 5 Developer’s Toolkit Files 25 5.1 Libraries and Files ...................................... 25 6 EyeLink Programming Conventions 29 6.1 Standard Messages ...................................... 29 7 EyeLink Data Types 31 7.1 Basic Portable Data Types .................................. 31 7.2 Link Data Types........................................ 31 8 Issues for Programming Experiments 37 8.1 Issues for DOS Programmers................................. 37 8.2 Issues for Windows Programmers .............................. 37 8.3 Windows Timing Issues.................................... 38 8.4 Hardware I/O under Windows ................................ 39 8.5 Message Pumps and Loops.................................. 40 8.6 Windows Key Support .................................... 40 8.7 Terminating the Program................................... 41 9 Graphics Programming using SDL 43 9.1 Resolutions and Colors.................................... 44 9.2 Drawing Speed ........................................ 44 ii CONTENTS 9.3 Display Mode Information.................................. 44 9.4 Adapting to Display Resolutions............................... 45 9.5 Synchronization with Display Refresh............................ 45 9.6 Full-Screen Window ..................................... 45 10 Graphics Programming using GDI 47 10.1 Displays and Experiments .................................. 47 10.2 Graphics Modes........................................ 49 10.3 Full-Screen Window ..................................... 52 10.4 Windows Graphics Fundamentals .............................. 53 10.5 Drawing With Bitmaps.................................... 55 11 Eyelink Graphics Programming Using External Graphics Library 59 11.1 Roles of EyeLink Graphics library.............................. 59 11.2 Writing Your Own Core Graphics............................... 60 11.3 Source code for cal_graphics.c................................ 61 11.4 Source code for cam_graphics.c ............................... 65 11.5 Source code for graphics_main.c............................... 69 12 Controlling Calibration 73 12.1 Calibration Colors....................................... 73 12.2 Calibration Target Appearance................................ 73 12.3 Calibration Sounds ...................................... 74 12.4 Recording Abort Blanking .................................. 74 13 Connections and Multiple Computer Configurations 75 13.1 Connection Types....................................... 76 13.2 Finding Trackers and Remotes................................ 77 13.3 Inter-Remote Communication ................................ 77 14 Experiment Templates Overview 79 14.1 Template Types........................................ 79 15 SIMPLE Template 81 15.1 Source Files for "Simple"................................... 82 15.2 Analysis of "main.c" ..................................... 83 15.3 Analysis of "trials.c" ..................................... 87 15.4 Control by Scripts....................................... 90 15.5 Analysis of "trial.c"...................................... 91 15.6 Drift Correction........................................ 91 15.7 Starting Recording ...................................... 92 15.8 Starting Realtime Mode.................................... 93 15.9 Drawing the Subject Display................................. 94 15.10Recording Loop........................................ 95 15.11Cleaning Up and Reporting Trial Results........................... 96 15.12Extending "trial.c" ...................................... 97 16 TEXT Template 99 16.1 Source Files for "Text" .................................... 99 16.2 Differences from "Simple".................................. 99 16.3 Analysis of "trials.c" .....................................100 16.4 Analysis of "trial.c"......................................101 17 PICTURE Template 103 17.1 Source Files for "Picture"................................... 103 Generated on Fri Sep 14 2012 13:12:44 for EyeLink Programmer’s Guide by Doxygen CONTENTS iii 17.2 Differences from "Text".................................... 103 17.3 Analysis of "trials.c" .....................................104 18 EYEDATA Template 107 18.1 Source Files for "Eyedata" ..................................107 18.2 Differences from "Text" and "Picture"............................108 18.3 Analysis of "data_trials.c" ..................................108 18.4 Analysis of "data_trial.c"...................................109 18.5 Analysis of "playback_trial.c" ................................111 19 GCWINDOW Template 115 19.1 Gaze-Contingent Window Issues...............................115 19.2 Source Files for "GCWindow" ................................118 19.3 Analysis of "trials.c" .....................................118 19.4 Other Gaze-contingent Paradigms ..............................122 20 CONTROL Template 123 20.1 Source Files for "Control" ..................................123 20.2 Analysis of "trial.c"......................................123 21 DYNAMIC Template 129 21.1 Source Files for "Dynamic".................................. 130 21.2 Analysis of "targets.c" ....................................130 21.3 Analysis of "trial.c"......................................132 21.4 Analysis of "trials.c" .....................................133 21.5 Adapting the "Dynamic" template ..............................140 22 COMM SIMPLE and COMM LISTENER Templates 141 22.1 Source Files for "Comm_simple"............................... 142 22.2 Analysis of "comm_simple_main.c".............................142 22.3 Analysis of "comm_simple_trial.c".............................. 144 22.4 Source Files for "Comm_listener" ..............................145 22.5 Analysis of "comm_listener_main.c"............................. 145 22.6 Analysis of "comm_listener_loop.c".............................147 22.7 Analysis of "comm_listener_record.c"............................149 22.8 Extending the "comm_simple" and "comm_listener" Templates . 151 23 BROADCAST Template 153 23.1 Source Files for "broadcast" .................................154 23.2 Analysis of "broadcast_main.c"................................ 154 23.3 Analysis of "broadcast_record.c"...............................159 23.4 Extending "broadcast" ....................................161 24 ASC File Analysis 163 24.1 Creating ASC files with EDF2ASC.............................. 163 24.2 Analyzing ASC Files.....................................163 24.3 Functions defined by "read_asc.c" ..............................164 24.4 A Sample ASC Analysis Application............................. 169 25 Useful EyeLink Commands 175 25.1 Calibration Setup.......................................175 25.2 Configuring Key and Buttons................................. 176 25.3 Display Setup.........................................178 25.4 File Open and Close...................................... 179 25.5 Tracker Configuration..................................... 179 Generated on Fri Sep 14 2012 13:12:44 for EyeLink Programmer’s Guide by Doxygen iv CONTENTS 25.6 Drawing Commands .....................................182 25.7 File Data Control.......................................184 25.8 Link Data Control....................................... 185 25.9 Parser Configuration .....................................187 26 Function List 191 26.1 Initialize EyeLink Library ..................................191 26.2 Access Local Time ......................................205 26.3 Access Tracker Time .....................................209 26.4 Setup EyeLink tracker ....................................213 26.5 Keyboard Input Functions ..................................224 26.6 Data file utilities........................................ 231 26.7 Application/Thread priority control.............................. 236 26.8 Graphics display options ...................................238 26.9 Extract extended data from samples and events .......................245 26.10Time stamped messages to log file..............................247 26.11Online velocity and acceleration calculation.........................248 26.12Utility function to save bitmaps................................ 251 26.13Record control and data collection..............................255 26.14Accessing and reporting error messages ...........................262 26.15Playback and data acquisition. ................................263 26.16Message and
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages422 Page
-
File Size-