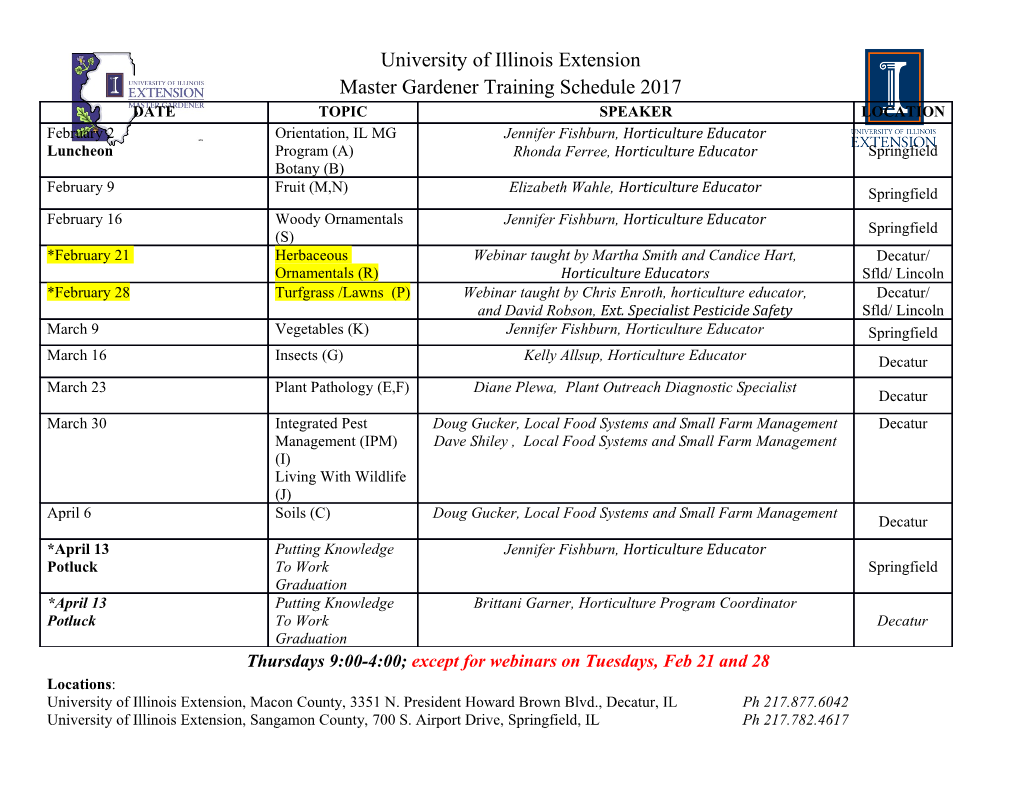
pyAtmosThermo Documentation Release 0.1 Ravi Shekhar February 06, 2016 Contents i ii pyAtmosThermo Documentation, Release 0.1 This library allows calculation of moist atmospheric thermodynamic quantities. For example, the following snippet will calculate moist static energy from mixing ratio of 0.001 kg/kg, 298 Kelvin, and 200 meters height. Thus, MSE has the value 304161.6 J/kg. import atmos_thermo h= atmos_thermo.h_from_r_T_z(0.001, 298, 200) This code calculates the saturation vapor pressure and produces 3533.7 Pa. e_saturation= atmos_thermo.esat_from_T(300) All functions are designed around using numpy arrays transparently, and using numpy arrays is vastly faster than passing in values one by one. Contents: Contents 1 pyAtmosThermo Documentation, Release 0.1 2 Contents CHAPTER 1 atmos_thermo package 1.1 Submodules 1.2 atmos_thermo.atmoslib module atmos_thermo.atmoslib.Lv_from_T(T) Calculate latent heat of vaporization for water, Lv. Args: T: temperature (Kelvin) Returns: Latent heat of vaporization [J / kg K] atmos_thermo.atmoslib.T_from_p_theta(p, theta, p0=100000.0) Calculate the temperature of air. Calculate the temperature (T) given pressure (p) and potential temperature (theta). Formula is : T = theta * (p / p0) ** (R_d / C_pd) Args: p: total pressure (Pascals) theta: potential temperature (Kelvin) p0: reference pressure used in calculating potential temperature Returns: temperature in Kelvin atmos_thermo.atmoslib.Tv_from_r_T(r, T) Calculate the virtual temperature. Calculate the virtual temperature (T_v) given temperature (T) and mixing ratio (r). Args: r: mixing ratio (dimensionless) T: temperature (Kelvin) Returns: Virtual Temperature in Kelvin atmos_thermo.atmoslib.e_from_r_p(r, p) Calculate partial pressure of water vapor. Calculate partial pressure of water vapor (e) from mixing ratio (r) and total pressure (p). Args: r: Mixing ratio in dimensionless ([kg H2O]/[kg Air]) units. p: Total pressure in Pascals. Returns: Vapor pressure (e) in Pascals. atmos_thermo.atmoslib.esat_from_T(T) Calculates saturation vapor pressure. Using the reference Emanuel 1994 (Atmospheric Convection) p.116, equation 4.4.13 , this function calculates the saturation vapor pressure (esat) at the given temperature. 3 pyAtmosThermo Documentation, Release 0.1 Args: T: Air temperature in Kelvin. Can be numpy array. Returns: Saturation vapor pressure (esat) in units of Pa. atmos_thermo.atmoslib.h_Em1994_from_r_T_z(r, T, z) Calculate the moist static energy (h). Using the definition of Emanuel 1994 (Atmospheric Convection). Equation 4.5.23 h = (C_pd + r_t C_pv) T + Lv r + g z Args: r: mixing ratio (dimensionless) T: temperature (Kelvin) z: height (meters) Returns: Moist static energy in J/kg (m^2 / s^2) atmos_thermo.atmoslib.h_KF_from_r_T_z_rliq_rfroz(r, T, z, rliq, rfroz) Calculate moist static energy according to the Kain-Fritsch definition. The standard frozen MSE formulation is: h = cp * T + Lv * r + g * z - Lf * r_froz Kain-Fritsch uses: cp_moist = cp_dry * (1 + 0.887 * r) # this is only the vapor r Lv = XLV0 - T * XLV1 Lf = XLS0 - T * XLS1 XLV0 = 3.15E6 J/kg XLV1 = 2370 J/(kg K) XLS0 = 2.905E6 J/kg XLS1 = 259.532 J/(kg K) atmos_thermo.atmoslib.h_from_r_T_z(r, T, z) Calculate the moist static energy (h). Using the classical definiton of moist static energy h = C_pd T + Lv r + g z Args: r: mixing ratio (dimensionless, or kg/kg) T: temperature (Kelvin) z: height (meters) Returns: Moist static energy in J/kg (m^2 / s^2) atmos_thermo.atmoslib.hd_Em1994_from_r_T_z(r, T, z) Calculate the dry static energy (hd). Using the definition of Emanuel 1994 (Atmospheric Convection). Equation 4.5.23. Notice that the mixing ratio is required to calculate the heat capacity correctly. hd = (C_pd + r_t C_pv) T + g z Args: r: mixing ratio (dimensionless) p: total pressure (Pascals) T: temperature (Kelvin) z: height (meters). Returns: Dry static energy in J/kg (m^2 / s^2) atmos_thermo.atmoslib.hd_from_r_T_z(r, T, z) Calculate the classical dry static energy (hd). Args: p: total pressure (Pascals) T: temperature (Kelvin) z: height (meters). Returns: Dry static energy in J/kg (m^2 / s^2) atmos_thermo.atmoslib.hstar_Em1994_from_p_T_z(p, T, z) Calculate the saturation moist static energy (h). Using the definition of Emanuel 1994 (Atmospheric Convection). Equation 4.5.23 h = (C_pd + r_sat C_pv) T + Lv (r_sat) + g z Args: p: total pressure (Pascals) T: temperature (Kelvin) z: height (meters) Returns: Saturation moist static energy in J/kg (m^2 / s^2) atmos_thermo.atmoslib.hstar_classical_from_p_T_z(p, T, z) Calculate the saturation moist static energy (h). Using the definition of Emanuel 1994 (Atmospheric Convection). Equation 4.5.23 4 Chapter 1. atmos_thermo package pyAtmosThermo Documentation, Release 0.1 h = (C_pd + r_sat C_pv) T + Lv (r_sat) + g z Args: p: total pressure (Pascals) T: temperature (Kelvin) z: height (meters) Returns: Saturation moist static energy in J/kg (m^2 / s^2) atmos_thermo.atmoslib.moist_specific_entropy_Emanuel_from_r_p_T(r, p, T, r_rain=0, r_cloud=0, r_ice=0) Calculate the moist specific entropy. Using the definition of Emanuel 1994 (Atmospheric Convection). Equation 4.5.9 s = (C_pd + C_pv * r_t) * ln(T) • R_d ln (pd) • Lv r / T • r R_v ln (H) Args: r: mixing ratio (dimensionless) p: total pressure (Pascals) T: temperature (Kelvin) Returns: Equivalent potential temperature in Kelvin atmos_thermo.atmoslib.moist_specific_entropy_Emanuel_sat_from_p_T(p, T, r_rain=0, r_cloud=0, r_ice=0) Calculate the moist specific entropy. Using the definition of Emanuel 1994 (Atmospheric Convection). Equation 4.5.9 s = (C_pd + C_pv * r_t) * ln(T) • R_d ln (pd) • Lv r / T • r R_v ln (H) Args: p: total pressure (Pascals) T: temperature (Kelvin) Returns: Equivalent potential temperature in Kelvin atmos_thermo.atmoslib.moist_specific_entropy_from_r_p_T(r, p, T) Calculate the moist specific entropy. Calculate moist specific entropy for the mixing ratio (r), total pressure (p), and temperature (T). Uses the definition given in Benedict et. al 2014 - Gross Moist Stability and MJO Simulation Skill ... Equation 1 Args: r: mixing ratio (dimensionless) p: total pressure (Pascals) T: temperature (Kelvin) Returns: Moist specific entropy in J / (kg K) atmos_thermo.atmoslib.pd_from_r_p(r, p) Calculate dry pressure without water vapor. Calculate dry pressure (pd) from mixing ratio (r) and total pressure (p) Args: r: Mixing ratio in dimensionless ([kg H2O]/[kg Air]) units. p: Total pressure in Pascals. 1.2. atmos_thermo.atmoslib module 5 pyAtmosThermo Documentation, Release 0.1 Returns: Dry pressure (pd) in Pascals. atmos_thermo.atmoslib.q_from_e_p(e, p) Calculates specific humidity. Calculates specific humidity given vapor pressure (e) and total pressure (p). Typically vapor pressure is e and specific humidity is q in the literature. q = [mass of H20 vapor] / [mass of total air] relationship is : q = epsilon * e / (p - e*(1-epsilon)) Args: e: Vapor pressure in Pascals p: Total pressure in Pascals Returns: Specific humidity in dimensionless ([kg H2O]/[kg Air]) units. atmos_thermo.atmoslib.q_from_r(r) Calculates specific humidity. Calculates specific humidity given mixing ratio. Typically mixing ratio is r and specific humidity is q in the literature. r = [mass of H20 vapor] / [mass of dry air] q = [mass of H20 vapor] / [mass of total air] relationship is : q = r / (1+r) Args: r: Mixing ratio in dimensionless ([kg H2O]/[kg Air]) units. Returns: Specific humidity in dimensionless ([kg H2O]/[kg Air]) units. atmos_thermo.atmoslib.r_from_e_p(e, p) Calculate mixing ratio of water vapor. Calculate mixing ratio of water vapor(r) from vapor pressure (e) and total pressure (p). Args: r: Mixing ratio in dimensionless ([kg H2O]/[kg Air]) units. p: Total pressure in Pascals. Returns: Vapor pressure (e) in Pascals. atmos_thermo.atmoslib.relhum_from_e_esat(e, esat) Calculate the relative humidity of air. Calculate the relative humidity of air (H) given the vapor pressure (e) and saturation vapor pressure (esat). Args: e: Vapor pressure in Pascals. esat: Saturation vapor pressure in Pascals. Returns: relative humidity in dimensionless units. atmos_thermo.atmoslib.relhum_from_r_p_T(r, p, T) Calculate the relative humidity of air. Calculate the relative humidity of air (H) given the mixing ratio (r), pressure (p) and temperature (T). Args: r: mixing ratio (dimensionless) p: total pressure (Pascals) T: temperature (Kelvin) Returns: relative humidity in dimensionless units. atmos_thermo.atmoslib.streamfunction_from_v_p(v, p) Calculate streamfunction from v, p atmos_thermo.atmoslib.theta_from_p_T(p, T, p0=100000.0) Calculate the potential temperature of air. Calculate the potential temperature (theta) given pressure (p) and temperature (T). Formula is : theta = T * (p0 / p) ** (R_d / C_pd) 6 Chapter 1. atmos_thermo package pyAtmosThermo Documentation, Release 0.1 Args: p: total pressure (Pascals) T: temperature (Kelvin) p0: reference pressure used in calculating potential temperature Returns: potential temperature in Kelvin atmos_thermo.atmoslib.thetae_Bolton_from_r_p_T(r, p, T, p0=100000, r_rain=0.0, r_cloud=0.0) Calculate the pseudoadiabatic equivalent potential temperature. Using the Bolton 1980 definition. Args: r: mixing ratio (dimensionless) p: total pressure (Pascals) T: temperature (Kelvin) Returns: Pseudoadibatic equivalent potential temperature in Kelvin atmos_thermo.atmoslib.thetae_from_r_p_T(r, p, T, p0=100000, r_cloud=0.0) Calculate the reversible equivalent potential temperature. Using the definition of Emanuel 1994 (Atmospheric Convection). Equation 4.5.11 theta_e = T * [(p0 / pd) ** (R_d / (C_pd
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages17 Page
-
File Size-