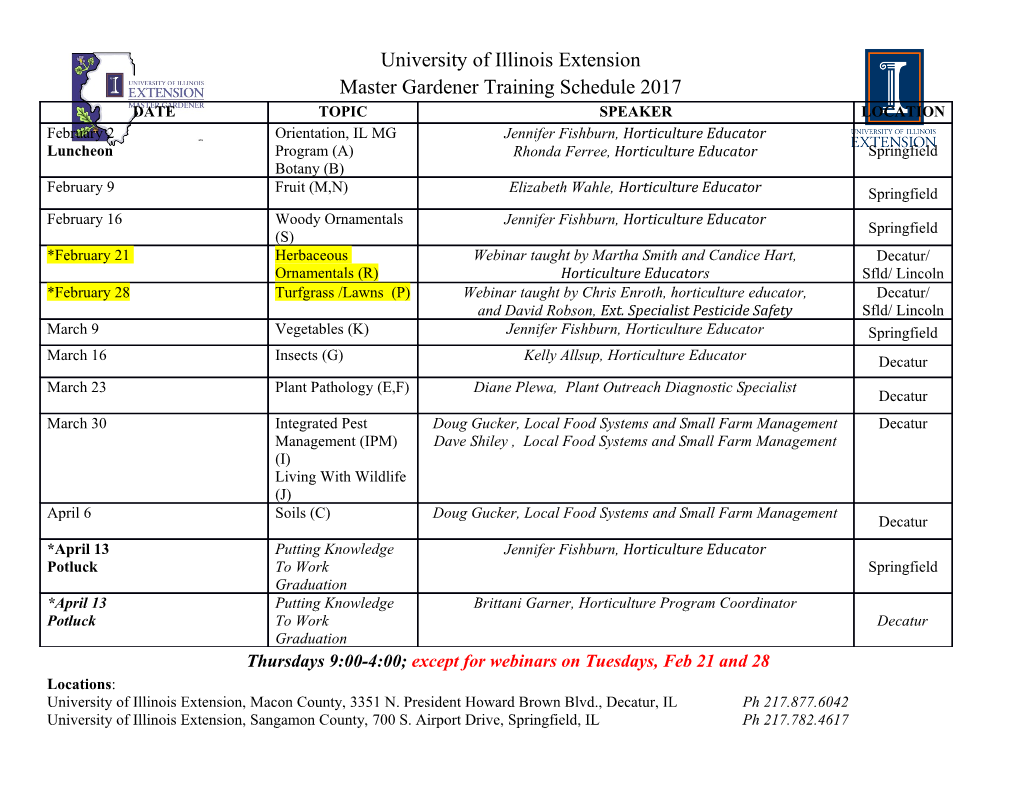
JSPP: Morphing C++ into JavaScript Christopher Chedeau, Didier Verna To cite this version: Christopher Chedeau, Didier Verna. JSPP: Morphing C++ into JavaScript. [Research Report] LRDE - Laboratoire de Recherche et de Développement de l’EPITA. 2012. hal-01543025 HAL Id: hal-01543025 https://hal.archives-ouvertes.fr/hal-01543025 Submitted on 20 Jun 2017 HAL is a multi-disciplinary open access L’archive ouverte pluridisciplinaire HAL, est archive for the deposit and dissemination of sci- destinée au dépôt et à la diffusion de documents entific research documents, whether they are pub- scientifiques de niveau recherche, publiés ou non, lished or not. The documents may come from émanant des établissements d’enseignement et de teaching and research institutions in France or recherche français ou étrangers, des laboratoires abroad, or from public or private research centers. publics ou privés. JSPP: Morphing C++ into JavaScript Christopher Chedeau, Didier Verna EPITA Research and Development Laboratory 14–16, rue Voltaire 94276 Le Kremlin-Bicêtre CEDEX, France {christopher.chedeau,didier.verna}@lrde.epita.fr ABSTRACT original JavaScript syntax as much as possible. Ultimately, In a time where the differences between static and dynamic we would like to be able to simply copy and paste JavaScript languages are starting to fade away, this paper brings one code in a C++ source file and compile it as-is. more element to the \convergence" picture by showing that A first prototypical implementation of a syntax-preserving thanks to the novelties from the recent C++0x standard, it JavaScript engine in C++ has been developed. The project is relatively easy to implement a JavaScript layer on top of is called JSPP. JSPP currently implements all the core fea- C++. By that, we not only mean to implement the language tures of JavaScript in about 600 lines of code. Being only features, but also to preserve as much of its original notation a prototype however, JSPP is not currently optimized and as possible. In doing so, we provide the programmer with a does not provide the JavaScript standard libraries. JSPP is an open-source project. The source code and some examples means to freely incorporate highly dynamic JavaScript-like 1 code into a regular C++ program. can be found at the project's Github Repository . In section 2, we describe the implementation of JavaScript's dynamic type system and object notation (a.k.a. JSON[4]). Categories and Subject Descriptors Section 3 on page 3 provides an explanation on how to use D.3.2 [Programming Languages]: Language Classifica- the C++0x lambda functions to emulate JavaScript func- tion|multi-paradigm languages; D.3.3 [Programming Lan- tions and object constructors. Section 4 on page 5 addresses guages]: Language Constructs and Features the support for JavaScript object properties (most notably prototypal inheritance and contents traversal). Finally sec- General Terms tion 5 on page 6 discusses some other aspects of the language along with their implementation. Design, Languages 2. DATA TYPES AND JSON Keywords In this section, we describe the implementation of JavaScript's C++, JavaScript, Multi-Paradigm Programming dynamic type system and object notation. 1. INTRODUCTION 2.1 Primitive Types The relations between static and dynamic language com- JavaScript has few primitive types: munities are notoriously tense. People from both camps of- Boolean: true or false, ten have difficulties communicating with each other and tend Number: double precision floating-point values, to become emotional when it comes to such concerns as ex- String: unicode-encoded character sequences, pressiveness, safety or performance. However, and perhaps undefined: special type with only one eponymous value. due (at least partially) to the constantly increasing popu- larity of modern dynamic languages such as Ruby, Python, The value undefined is the default value for (undeclared) PHP or JavaScript, those seemingly irreconcilable worlds are variables. JSPP values are implemented as instances of a starting to converge. For example, while C# recently pro- single Value class. This class emulates the usual boxing vided support for dynamic types, Racket[8], a descendant technique of dynamic languages, and notably stores a type from Scheme, allows you to freely mix static typing with an flag for each value. A JavaScript variable assignment such otherwise dynamically typed program. as: C++0x[13], the new standard for the C++ language, in- var foo = 1; corporates many concepts well known in the functional or dynamic worlds, such as \lambda (anonymous) functions", essentially translates into the following C++ code: \range-based iterators" (an extended iteration facility) and \initializer lists" (an extended object initialization facility). Value foo = Integer (1); To bring one more element to this general trend towards In JSPP, var is simply typedef'ed to Value, so as to pre- convergence, this article relates the surprising discovery that serve the original JavaScript notation. The Value class con- given this new C++ standard, it is relatively easy to imple- structor is overloaded to handle all basics C++ types such ment JavaScript in C++. The idea is to make all the fea- tures of JavaScript available in C++ while preserving the 1http://github.com/vjeux/jspp EPITA / LRDE Technical Report #201201-TR 1 1 var undefined, 1 var undefined, 2 string = "SAC", 2 string = "SAC", 3 number = 4.2; 3 number = 4.2; 4 4 5 var json = f 5 var jsppon = f 6 "number": 42, 6 ["number"] = 42, 7 "string": "vjeux", 7 ["string"] = "vjeux", 8 "array": [1, 2, "three"], 8 ["array"] = f1, 2, "three"g, 9 9 10 "nested": f 10 ["nested"] = f 11 "first": 1, 11 ["first"] = 1, 12 "second": 2 12 ["second"] = 2 13 g 13 g 14 g; 14 g; Listing 1: Original JSON Listing 2: JSPP JSON as const char*, int, double etc. The argument-less ver- type are not class instances, and hence cannot be operator- sion of the constructor is overloaded to create an undefined overloaded (something required for property initialization, value. as explained below). One possible notation to transform a const char* into an instance of a class is the following: 2.2 Composite Types _["key"] In JavaScript, everything that is not of a primitive type is an object. All JavaScript objects are actually just asso- The choice of this notation is motivated by the fact that it is ciative arrays (or dictionaries) in which the keys are strings. reminiscent of the JavaScript object property access syntax, Keys are often referred to as the object's \properties". and hence rather easy to remember: All values of a primitive type may have an object coun- terpart. This allows them to have properties and specific obj["string"] \methods". JavaScript automatically boxes primitive values In order to implement this notation, we create a singleton when used in an object context[7]. In such a situation, the class named Underscore equipped with an eponymous in- objects in question are passed by reference instead of by stance. This class overloads both the bracket [] and equal value[12]. In the current implementation of JSPP, primitive = operators so as to create a KeyValue object, that is, an values are always boxed. object holding both a property name (the key), and its as- A JavaScript array is an object the keys of which are the sociated value. base 10 string representation of the elements positions. The Initializing an object contents can now be done in two first element has the key "0", the second has the key "1" and ways: either by providing KeyValue objects, or only Value so on. Additionally, arrays have a special length property objects. When a KeyValue object is encountered, the pair which is updated automatically when new elements (with a is inserted directly into the object's internal map. When a numerical key) are added. Value object is encountered, it is regarded as an array ele- As mentioned before, JavaScript introduces a purely syn- ment. As a consequence, the key is the string representation tactic notation for objects and arrays called JSON[4] (JavaScript of the object's current length property, which is automati- Object Notation). JSON introduces two distinct syntactic cally increased by one after the insertion. forms for creating regular objects and arrays. Implement- ing this notation (or something close), turns out to be the 2.3 Caveats biggest challenge for JSPP. The result is depicted in list- From a syntactical point of view, the notation provided ings 1 and 2. Eventhough JavaScript exhibits two different by JSPP is heavier than the original JavaScript one. The syntactic forms for variadic object and array initialization, key part, notably, is more verbose, and equal signs are wider we need a single C++0x feature, called \initializer lists"[14], than colons. On the other hand, a slight improvement over to implement both. JSON is that keys can in fact be any expression leading to As far as the array notation is concerned, the implemen- instantiating the Underscore class, whereas in JavaScript, tation is straightforward, although one drawback is that keys can only be literal strings. This allows for dynamic JavaScript's square brackets [] needs to be replaced with computation of keys, something not possible in JavaScript. C++'s curly braces fg. See line 8 on the corresponding Unfortunately, using initializer lists introduces some corner- listings. cases. For instance, constructing an object using an empty The object notation, on the other hand, requires more initialization list does not call the constructor with an empty trickery. JavaScript uses colons (:) to separate keys from initializer list but without any argument instead. This means their corresponding values. Our problem is that the colon that {} is equivalent to undefined instead of an empty ob- is not an overloadable operator in C++. Consequently, we ject. choose to use the equal sign (=) instead.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages10 Page
-
File Size-