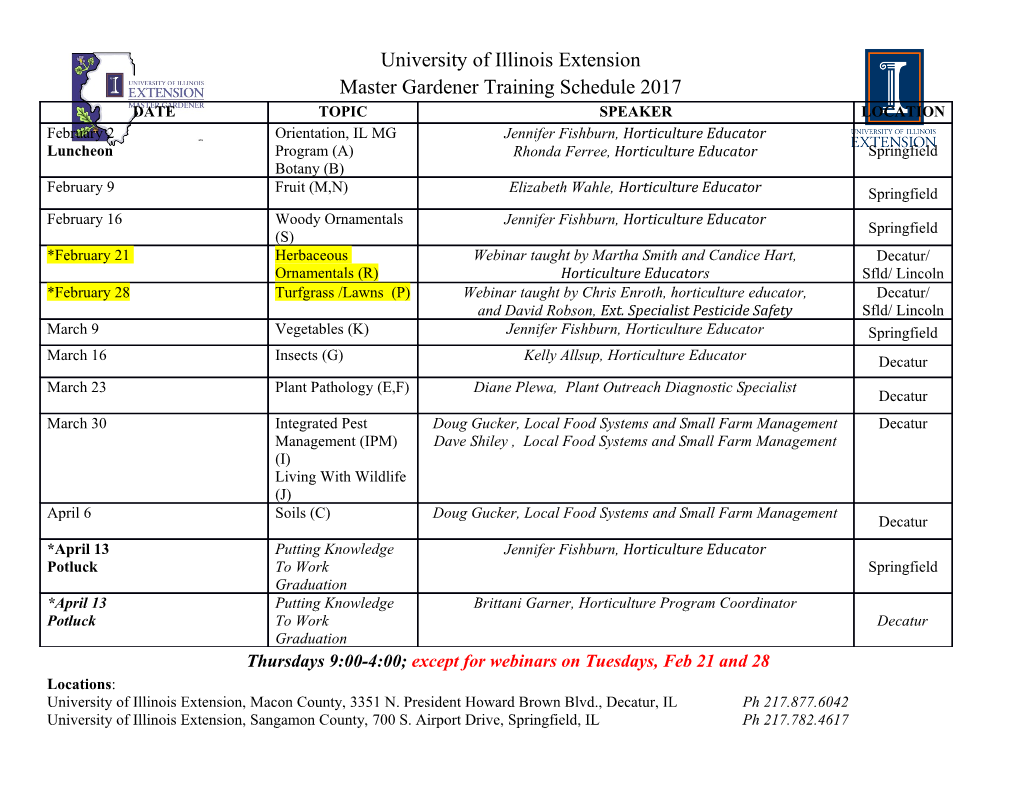
Efficiently Calculating the Determinant of a Matrix Felix Limanta 13515065 Program Studi Teknik Informatika Sekolah Teknik Elektro dan Informatika Institut Teknologi Bandung, Jl. Ganesha 10 Bandung 40132, Indonesia [email protected] [email protected] Abstract—There are three commonly-used algorithms to infotype is either real or integer, depending on calculate the determinant of a matrix: Laplace expansion, LU implementation, while matrix is an arbitrarily-sized decomposition, and the Bareiss algorithm. In this paper, we array of array of infotype. first discuss the underlying mathematical principles behind the algorithms. We then realize the algorithms in pseudocode Finally, we analyze the complexity and nature of the algorithms and compare them after one another. II. BASIC MATHEMATICAL CONCEPTS Keywords—asymptotic time complexity, Bareiss algorithm, A. Determinant determinant, Laplace expansion, LU decomposition. The determinant is a real number associated with every square matrix. The determinant of a square matrix A is commonly denoted as det A, det(A), or |A|. I. INTRODUCTION Singular matrices are matrices which determinant is 0. In this paper, we describe three algorithms to find the Unimodular matrices are matrices which determinant is 1. determinant of a square matrix: Laplace expansion (also The determinant of a 1×1 matrix is the element itself. known as determinant expansion by minors), LU 퐴 = [푎] ⇒ det(퐴) = 푎 decomposition, and the Bareiss algorithm. The determinant of a 2×2 matrix is defined as the product The paper is organized as follows. Section II reviews the of the elements on the main diagonal minus the product of basic mathematical concepts for this paper, which includes the elements off the main diagonal. 푎 푏 determinants, elementary matrix operations, algorithm | | = 푎푑 − 푏푐 푐 푑 complexities, time complexities, and asymptotic time Finding the determinant of larger matrices will be complexities. In Section III, the three methods are discussed in later sections. discussed in-depth mathematically, whereas in Section IV, Some basic properties of determinants are the three methods, as well as subroutines necessary to 1. det(퐼) = 1 implement them, are implemented in a language-agnostic 2. det(퐴푇) = det (퐴) pseudocode. In Section V, the methods implemented in 1 3. det(퐴−1) = Section V are analyzed regarding its asymptotic time det (퐴) complexity and other features before being compared to 4. For square matrices A and B of equal size, one another. Finally, Section VI concludes the paper while det(퐴퐵) = det(퐴) × det(퐵) suggesting further improvements. 5. The determinant of a triangular matrix is the product of the diagonal entries (pivots) d1, d2, …, dn. Terminology and Notation 푛 Unless noted otherwise, all mentions of matrices in this det(퐴) = 푎1,1푎2,2 … 푎푛,푛 = ∏ 푎푖,푖 paper are assumed to be square matrices. 푖=1 Determinants are useful for other mathematical subjects. To describe iterations mathematically, the notation 푖 ∈ For example, following Cramer’s rule, a matrix can be used [푎. 푏] is introduced. The notation is taken from the to represent the coefficients of a system of linear equations, mathematical concept of number intervals, but instead of and the determinant can be used to solve the system of describing whether i is between a and b or not, it denotes linear equations if and only if the matrix is nonsingular. that the iterator i starts from a and ends in b, incrementing Determinants are also used in determining the inverse of a i by 1 after each iteration. It is equivalent to the for syntax matrix, where any matrix has a unique inverse if and only in programming languages, in which 푖 ∈ [푎. 푏] is if the matrix is nonsingular. equivalent to for i = a to b. The pseudocode described in section III follows the B. Elementary Matrix Operations syntax detailed in reference [5]. For convenience, two data types are defined here: Elementary matrix operations play important roles in Makalah IF2120 Matematika Diskrit – Sem. I Tahun 2016/2017 various matrix algebra applications, such as solving a D. Time Complexity system of linear equations using and finding the inverse of The time complexity of an algorithm is measured from a matrix. the number of instructions executed. The time complexity There are three kinds of elementary matrix operations: of an algorithm is not measured from the running time of 1. Switch two rows (or columns). said algorithm because of the differences between every 2. Multiply each element in a row (or column) by a computer’s architecture, every programming language and non-zero number. its compiler, and so on. Because of that, the same algorithm 3. Add or subtract a row (or column) by multiples of implemented in different languages or the same executable another row (or column). run in different machines would yield different running When these operations are performed on rows, they are times. called elementary row operations and, likewise, when they In calculating the time complexity of an algorithm, are performed on columns, they are called elementary ideally, all operations are accounted for. For decently column operations. complex algorithms, this is all but impossible, so Elementary matrix operations have the following effect practically, only basic operations are accounted for. For on determinants. example, the basic operation of a searching algorithm 1. Exchanging two rows or columns from a matrix would be the comparison of the searched element with reverse the sign of its determinant from positive to elements in the array. By counting how many comparisons negative and vice-versa. are made, we can obtain the relative efficiency of the 푎 푏 푐 푑 | | = − | | algorithm. 푐 푑 푎 푏 The time complexity of an algorithm can be divided into 2. Multiplying one row or column of a matrix by t three cases: multiplies the determinant by t. 푡푎 푡푏 푡푎 푏 푎 푏 Tmin(푛): Best case | | = | | = 푡 | | 푐 푑 푡푐 푑 푐 푑 Tmax(푛): Worst case 3. If two rows or two columns of a matrix are equal, or Tavg(푛): Average case a row or column is the multiply of another row or column, its determinant is zero. Example 1. Find the best case, worst case, and average 푎 푏 | | = 0 case time complexity of the following sequential search 푡푎 푡푏 algorithm. 4. If 푖 ≠ 푗, adding or subtracting t times row i from row j or vice-versa does not change the determinant. function SeqSearch (a: array of infotype, 푎 푏 푎 푏 | | = | | n: integer, x: infotype) → integer 푐 − 푡푎 푑 − 푡푏 푐 푑 DICTIONARY 5. If A has a row that is all zeros, then det(퐴) = 0. i: integer 푎 푏 found: boolean | | = 0 0 0 ALGORITHM i ← 1 C. Algorithm Complexity found ← false while ((i ≤ n) and not(found)) do Algorithms are defined as the series of steps necessary found ← (a[i] = x) to solve a problem systematically. An algorithm, first and if not(found) then foremost, is not only required to be effective and actually i ← i + 1 solve the problem, but also expected to be efficient. An if (found) then → i algorithm might be able to effectively solve a problem, but else if the algorithm demands an unreasonable amount of → 0 processing time or takes up disproportionately large amount of memory, the algorithm is next to useless. Best case: 푎1 = 푥 ⇒ Tmin(푛) = 1 In application, every algorithm uses up two resources Worst case: 푎푛 = 푛 or 푛 not found ⇒ Tmax(푛) = 푛 which can be used as measurement: the number of steps 1+2+⋯+푛 푛+1 Average case: T (푛) = = taken (time) and the amount of memory reserved to execute avg 푛 2 those steps (space). The number of steps taken is defined as the time complexity, denoted as T(n), whereas the E. Asymptotic Time Complexity amount of memory reserved is defined as the space Usually, the exact time complexity of an algorithm is complexity, denoted as S(n). unnecessary. Instead, what is needed is a rough estimate of Matrices are a type of data structure which takes up a the time required by the algorithm and how fast that time relatively large amount of memory, compared to more requirement grow with the size of the input. This is because compact data structures such as linked lists. Therefore, the the efficiency of an algorithm would only be apparent at a space complexity of the algorithms discussed later will not large number of inputs. Running the determinant- be examined in favor of focusing on their time complexity. calculating algorithms on a 3×3 matrix would not yield any notable differences between the algorithm running Makalah IF2120 Matematika Diskrit – Sem. I Tahun 2016/2017 times. However, if the algorithms are run on a large matrix More informally, expansion by minors can be written as (say, 5000×5000), differences between running times 푎11 푎12 푎13 ⋯ 푎1푛 푎 푎 푎 ⋯ 푎 would be much more apparent. | 21 22 23 2푛| The asymptotic time complexity is a rough estimate of ⋮ ⋮ ⋮ ⋱ ⋮ the time complexity as the number of inputs approaches 푎푛1 푎푛2 푎푛3 ⋯ 푎푛푛 푎 푎 ⋯ 푎 infinite. The asymptotic time complexity is commonly 22 23 2푛 = 푎 | ⋮ ⋮ ⋱ ⋮ | denoted with the Big-O notation, though other notations 11 푎푛1 푎푛2 ⋯ 푎푛푛 exist. Generally, the asymptotic time complexity of an 푎21 푎23 ⋯ 푎2푛 algorithm can be taken from the most significant term in − 푎12 | ⋮ ⋮ ⋱ ⋮ | + ⋯ the time complexity function. For example, 푎푛2 푎푛2 ⋯ 푎푛푛 5 푛 푛 T(푛) = 192푛 + 2 + log 푛! = O(2 ) 푎21 푎22 ⋯ 푎2(푛−1) 푛 because for non-negative integer values of 푛, 2 ± 푎1푛 | ⋮ ⋮ ⋱ ⋮ | contributes the most to the function T(푛). 푎푛1 푎푛2 ⋯ 푎푛(푛−1) Table I shows categories of algorithm based on its Big- O notation.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages8 Page
-
File Size-