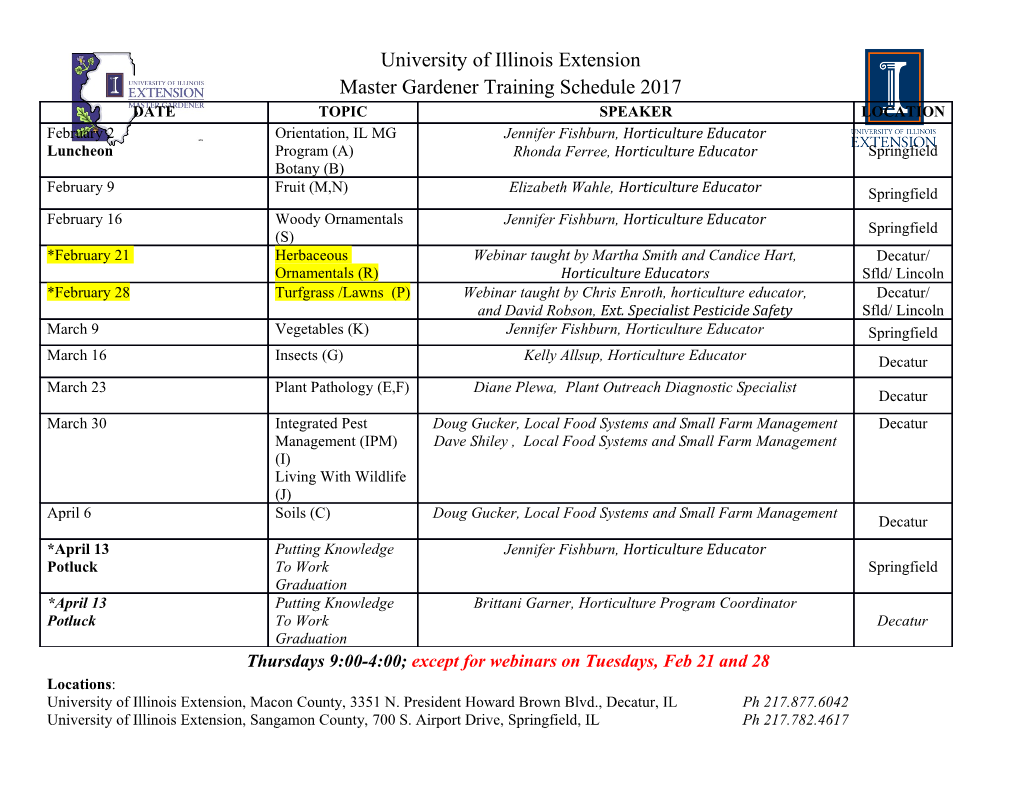
70 TWO COMPUTATIONAL GEOMETRY LIBRARIES: LEDA AND CGAL Michael Hoffmann, Lutz Kettner and Stefan N¨aher INTRODUCTION Over the past decades, two major software libraries that support a wide range of ge- ometric computing have been developed: Leda, the Library of Efficient Data Types and Algorithms, and Cgal, the Computational Geometry Algorithms Library. We start with an introduction of common aspects of both libraries and major differ- ences. We continue with sections that describe each library in detail. Both libraries are written in C++. Leda is based on the object-oriented par- adigm and Cgal is based on the generic programming paradigm. They provide a collection of flexible, efficient, and correct software components for computational geometry. Users should be able to easily include existing functionality into their programs. Additionally, both libraries have been designed to serve as platforms for the implementation of new algorithms. Correctness is of crucial importance for a library, even more so in the case of geometric algorithms where correctness is harder to achieve than in other areas of software construction. Two well-known reasons are the exact arithmetic assumption and the nondegeneracy assumption that are often used in geometric algorithms. However, both assumptions usually do not hold: floating point arithmetic is not exact and inputs are frequently degenerate. See Chapter 46 for details. EXACT ARITHMETIC There are basically two scientific approaches to the exact arithmetic problem. One can either design new algorithms that can cope with inexact arithmetic or one can use exact arithmetic. Instead of requiring the arithmetic itself to be exact, one can guarantee correct computations if the so-called geometric primitives are exact. So, for instance, the predicate for testing whether three points are collinear must always give the right answer. Such an exact primitive can be efficiently implemented using floating-point filters or lazy evaluation techniques. This approach is known as exact geometric computing paradigm and both libraries, Leda and Cgal, adhere to this paradigm. However, they also offer straight floating point implementations. DEGENERACY HANDLING An elegant (theoretical) approach to the degeneracy problem is symbolic per- turbation. But this method of forcing input data into general position can cause serious problems in practice. In many cases, it increases the complexity of (interme- 1 2 M. Hoffmann, L. Kettner and S. N¨aher diate) results considerably; and furthermore, the final limit process turns out to be difficult in particular in the presence of combinatorial structures. For this reason, both libraries follow a different approach. They cope with degeneracies directly by treating the degenerate case as the \normal" case. This approach proved to be effective for many geometric problems. However, symbolic perturbation is used in some places. For example, in Cgal the 3D Delaunay triangulation uses it to realize consistent point insert and removal functions in the degenerate case of more than four points on a sphere [DT11]. GEOMETRIC PROGRAMMING Both Cgal and Leda advocate geometric programming. This is a style of higher level programming that deals with geometric objects and their corresponding prim- itives rather than working directly on coordinates or numerical representations. In this way, for instance, the machinery for the exact arithmetic can be encapsulated in the implementation of the geometric primitives. COMMON ROOTS AND DIFFERENCES Leda is a general-purpose library of algorithms and data structures, whereas Cgal is focused on geometry. They have a different look and feel and different design principles, but they are compatible with each other and can be used together. A Leda user can benefit from more geometry algorithms in Cgal, and a Cgal user can benefit from the exact number types and graph algorithms in Leda, as will be detailed in the individual sections on Leda and Cgal. Cgal started six years after Leda. Cgal learned from the successful decisions and know-how in Leda (also supported by the fact that Leda's founding institute is a partner in developing Cgal). The later start allowed Cgal to rely on a better C++ language support, e.g., with templates and traits classes, which led the developers to adopt the generic programming paradigm and shift the design focus more toward flexibility. Successful spin-off companies have been created around both Leda1 and Cgal2. After an initial free licensing for academic institutions, all Leda licenses are now fee-based. In contrast, Cgal is freely available under the GPL/LGPL [GPL07] since release 4.0 (March 2012). Users who consider the open source license to be too restrictive can also obtain a commercial license. GLOSSARY Exact arithmetic: The foundation layer of the exact computation paradigm in computational geometry software that builds correct software layer by layer. Exact arithmetic can be as simple as a built-in integer type as long as its pre- cision is not exceeded or can involve more complex number types represent- ing expression DAGs, such as, leda::real from Leda [BFMS00] or Expr from Core [KLPY99]. 1Algorithmic Solutions Software GmbH <www.algorithmic-solutions.com>. 2GeometryFactory Sarl <www.geometryfactory.com>. Chapter 70: Two computational geometry libraries 3 Floating point filter: A technique that speeds up exact computations for com- mon easy cases; a fast floating-point interval arithmetic is used unless the error intervals overlap, in which case the computation is repeated with exact arith- metic. Coordinate representation: Cartesian and homogeneous coordinates are sup- ported by both libraries. Homogeneous coordinates are used to optimize exact rational arithmetic with a common denominator, not for projective geometry. Geometric object: The atomic part of a geometric kernel. Examples are points, segments, lines, and circles in 2D, planes, tetrahedra, and spheres in 3D, and hyperplanes in dD . The corresponding data types have value semantics; variants with and without reference-counted representations exist. Predicate: Geometric primitive returning a value from a finite domain that ex- presses a geometric property of the arguments (geometric objects), for example, CGAL::do intersect(p,q) returning a Boolean or leda::orientation(p,q,r) returning the sign of the area of the triangle (p; q; r). A filtered predicate uses a floating-point filter to speed up computations. Construction: Geometric primitive constructing a new object, such as the point of intersection of two non-parallel straight lines. Geometric kernel: The collection of geometric objects together with the related predicates and constructions. A filtered kernel uses filtered predicates. Program checkers: Technique for writing programs that check the correctness of their output. A checker for a program computing a function f takes an instance x and an output y. It returns true if y = f(x), and false otherwise. 70.1 LEDA Leda aims at being a comprehensive software platform for the entire area of com- binatorial and geometric computing. It provides a sizable collection of data types and algorithms. This collection includes most of the data types and algorithms described in the textbooks of the area ([AHU74, CLR90, Kin90, Meh84, NH93, O'R94, Tar83, Sed91, van88, Woo93]). A large number of academic and industrial projects from almost every area of combinatorial and geometric computing have been enabled by Leda. Examples are graph drawing, algorithm visualization, geographic information systems, loca- tion problems, visibility algorithms, DNA sequencing, dynamic graph algorithms, map labeling, covering problems, railway optimization, route planning, compu- tational biology and many more. See <www.algorithmic-solutions.com/leda/ projects/index.html> for a list of industrial projects based on Leda. The Leda project was started in the fall of 1988 by Kurt Mehlhorn and Stefan N¨aher.The first six months was devoted to the specification of different data types and selecting the implementation language. At that time the item concept arose as an abstraction of the notion \pointer into a data structure." Items provide direct and efficient access to data and are similar to iterators in the standard template library. The item concept worked successfully for all test cases and is now used for most data types in Leda. Concurrently with searching for the correct specifica- tions, existing programming languages were investigated for their suitability as an 4 M. Hoffmann, L. Kettner and S. N¨aher implementation platform. The language had to support abstract data types and type parameters (genericity) and should be widely available. Based on the experi- ences with different example programs, C++ was selected because of its flexibility, expressive power, and availability. We next discuss some of the general aspects of the Leda system. EASE OF USE The Leda library is easy to use. In fact, only a small fraction of the users are algorithms experts and many users are not even computer scientists. For these users the broad scope of the library, its ease of use, and the correctness and effi- ciency of the algorithms in the library are crucial. The Leda manual [MNSU] gives precise and readable specifications for the data types and algorithms mentioned above. The specifications are short (typically not more than a page), general (so as to allow several implementations) and abstract (so as to hide all details of the implementation). EXTENSIBILITY Combinatorial and geometric computing is a diverse area and hence it is impossible
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages33 Page
-
File Size-