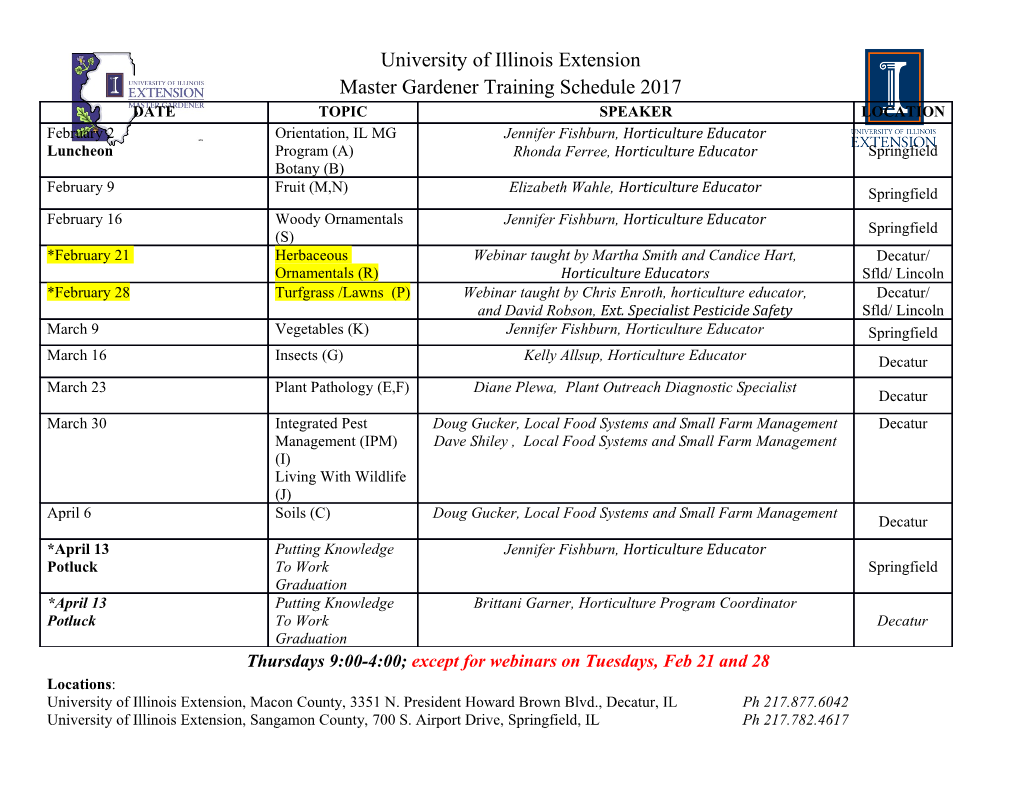
Beyond OOP Advanced Separation of Concerns Metaprogramming Reflection Aspect-Oriented Programming Eric Tanter DCC/CWR University of Chile [email protected] Éric Tanter – U.Chile [june | 2005] 1 Contents ● A brief history of programming ● Advanced modularization problems ● Reflection and metaprogramming ● Aspect-oriented programming Éric Tanter – U.Chile [june | 2005] 2 Part I A Quick History of Programming Adapted from Brian Hayes, “The Post-OOP Paradigm”, American Scientist, 91(2), March-April 2003, pp. 106-110 Éric Tanter – U.Chile [june | 2005] 3 Software? ● Real challenge: building the machine ● Software was not important as such ● First programs (40's) ● Written in pure binary: 0s and 1s ● Explicit memory address management Éric Tanter – U.Chile [june | 2005] 4 Then came the assembly... ● Assembly language ● No more raw binary codes ● Symbols: load, store, add, sub ● Converted to a binary program by an assembler ● Calculates memory addresses ● First program to help programming Éric Tanter – U.Chile [june | 2005] 5 ...and then, compilers ● Assembly required knowledge of the specific computer instruction set ● Higher-level languages (e.g. Fortran) ● Think in terms of variables/equations ● Not registers/addresses ● 60's: big projects = late/costly/buggy Éric Tanter – U.Chile [june | 2005] 6 Structured Programming ● Manifesto by Edsger W. Dijkstra: “Go to statement considered harmful” [1968] ● Build programs out of sub-units ● Single entrance point, single exit ● 3 constructs: ● Sequencing, alternation, and iteration ● Proof that these 3 idioms are sufficient to express all programs by Bohm & Jacopini [1966] Éric Tanter – U.Chile [june | 2005] 7 Structured Programming ● Early programming principles ● Top-down design and stepwise refinement ● Dijkstra [1968] ● Introduction of the notion of modularity and the principle of Separation of Concerns (SOC) ● Self-contained units with interfaces between them ● Parnas [1972] ● Notion of encapsulation (data hiding) ● Internal working of modules kept private Éric Tanter – U.Chile [june | 2005] 8 Variety of Paradigms ● Different ways of structuring programs ● Functional, declarative, logic, procedural, object- oriented... ● Ways to solve a problem ● through divide-and-conquer ● Decompose – solve – recompose Éric Tanter – U.Chile [june | 2005] 9 Object orientation ● Problem with procedural programs ● Procedures and data structures are separate but interdependent ● Changing implementation ● Changing representation ● Contribution of OOP ● Pack data and procedures together ● Objects communicate through messages Éric Tanter – U.Chile [june | 2005] 10 Object orientation ● Key mechanisms ● Instantiation (or cloning) ● Inheritance ● Polymorphism ● Aggregation and delegation Éric Tanter – U.Chile [june | 2005] 11 Object-oriented languages ● Origins ● SIMULA by Dahl and Nygaard (60s) ● SIMULA I (62-65), SIMULA 67 (inheritance) ● Parnas ideas on encapsulation ● Smalltalk (70s) ● Alan Kay & Co. at Xerox PARC ● Introduce GUI and interactive program execution ● Then ● C++, Smalltalk-80, Java, ... ● OO versions of older languages, e.g. CLOS Éric Tanter – U.Chile [june | 2005] 12 Part II Advanced Modularization Problems in (OO) Programs Éric Tanter – U.Chile [june | 2005] 13 OOP Promises ● Major objectives ● Evolution, extension ● Maintenance ● Reuse ● Additional mechanisms ● Frameworks ● Design Patterns [GoF95] ● “the quality without a name” [Alexander,1979] Éric Tanter – U.Chile [june | 2005] 14 Apache Tomcat case study ● Realized by AspectJ folks ● eclipse.org/aspectj ● Illustrates modularization problems in the Apache Tomcat engine Éric Tanter – U.Chile [june | 2005] 15 XML Parsing in Tomcat ● Cleanly modularized in one class Éric Tanter – U.Chile [june | 2005] 16 URL Pattern matching in Tomcat ● Cleanly modularized ● one abstract class/interface and one class Éric Tanter – U.Chile [june | 2005] 17 Logging in Tomcat ● Is this modularized??? Éric Tanter – U.Chile [june | 2005] 18 Session Expiration in Tomcat ● Is this modularized??? Éric Tanter – U.Chile [june | 2005] 19 Problem: decomposition ● Challenge in OOP ● The right decomposition into classes/objects ● e.g., modeling polygons, animals, ... ● Tyranny of the dominant decomposition ● Some issues are not easily arranged in a tree-like hierarchy ● Multiple inheritance (C++) ● Complex semantics due to merging conflicts Éric Tanter – U.Chile [june | 2005] 20 Problem: modularization ● Fact: code scattering ● Code replication = same code repeated in different places ● Code tangling = code for different concerns in the same place ● Why? ● Nature of concerns Éric Tanter – U.Chile [june | 2005] 21 Nature of concerns ● Some concerns are not related to application logic ● exception handling, security, persistence, distri- bution, concurrency, etc. ● Such non-functional concerns are often mixed with the application logic Éric Tanter – U.Chile [june | 2005] 22 Nature of concerns ● Some concerns do not fit in the global decomposi- tion scheme ● They crosscut the application's structure ● Usually handle interactions between modules ● e.g., display update in graphic editor Éric Tanter – U.Chile [june | 2005] 23 Example public void withdraw (double amount) throws NotAllowedException { if(!authorized(Thread.currentThread()) throw new NotAllowedException(); try { checkAmount(amount); doWithdraw(amount); notifyListeners(); } catch(LimitAmountExceeded e){ doWithdraw(e.getMaxAmount()); } } This is code tangling Éric Tanter – U.Chile [june | 2005] 24 Example boolean search(Object e){ boolean found = false; Enumeration elems = this.elements(); while(!found && (elems.hasMoreElements())) found = e.equals(elems.nextElement()) return found; } ● Should be located in many classes (that under- stand the elements() message) ● Such classes are not located in the same class hierarchy: this is a crosscutting concern Éric Tanter – U.Chile [june | 2005] 25 Example ● Design patterns ● Provide design reuse, but no code reuse ● Some patterns lead to tangled code register(this) Observer 1 Subject stateChanged(this) notifyObservers() Observer 2 Éric Tanter – U.Chile [june | 2005] 26 Summary of Issues ● Tangled code ● Readability is decreased ● Detecting/resolving errors ● Reusability is killed ● Replicated code ● Hard to evolve, extend, maintain ● Crosscutting concerns ● No explicit structure ● Hard to reason about, have a global vision Éric Tanter – U.Chile [june | 2005] 27 “Post-OOP” ● Address modularization issues further ● Extend OOP rather than replace it ● Program generation ● Metaprogramming and reflection ● Aspect-oriented programming ● Etc... ● Adaptive programming, composition filters, hyper- spaces, subject-oriented programming, ... Éric Tanter – U.Chile [june | 2005] 28 Generative Programming ● Transform high-level specs into a ready-to-run program ● Old dream ● Area of success: compiler compilers ● Take grammar as input and generate compilers ● Generative Programming ● Idem for other domains ● Manufacturing, product-line engineering ● Create families of tailored programs Éric Tanter – U.Chile [june | 2005] 29 Modularity Requirements ● Concerns in their own modules ● “Connect” them at appropriate places ● Issues ● Intrusiveness, obliviousness ● Symmetry of the approach ● Genericity, degree of coupling ● Expressive power given to modules Éric Tanter – U.Chile [june | 2005] 30 Part III Reflection and Metaprogramming Éric Tanter – U.Chile [june | 2005] 31 Introduction History and concepts Éric Tanter – U.Chile [june | 2005] 32 Programs and Data ● Distinction data/program ● appears in 1830s, by Charles Babbage ● Difference Engine No.2 ● The store with data and the mill with programs ● 1958, von Neumann ● Architecture of numerical computer ● Idea: store programs in memory, that same where data is stored ● Perspective: a program could alter its own in- structions! Éric Tanter – U.Chile [june | 2005] 33 Programs and Data ● From computing theory ● Lambda calculus (Church) ● Universal Turing Machine ● Metaprogram ● A program that affects a program ● Metaprogramming ● Developing metaprograms Éric Tanter – U.Chile [june | 2005] 34 Reflection ● Philosophy (Brian Cantwell Smith) ● Study on psychology and human experiences ● Foundations of consciousness ● Varieties of self-references ● Varietes of self-descriptions ● Definition of reflection: “A process' integral ability to represent, operate on, and otherwise deal with itself in the same way that it represents, operates on and deals with its primary subject matter” Éric Tanter – U.Chile [june | 2005] 35 Reflection in Computer Science ● Artificial intelligence: self-reasoning systems ● Brian Smith [1982] ● “reflection and semantics in a procedural language” ● 3LISP with Jim Des Rivieres ● Pattie Maes [1987] ● “computational reflection” ● Merging OOP languages and reflective architectures ● Smalltalk, Scheme, Loops, ObjVLisp,... Éric Tanter – U.Chile [june | 2005] 36 Concepts [Maes87] ● Computational System (CS) ● Act and reason about a domain ● Causal connection ● Changes in the domain are reflected in the CS, and vice- versa ● Metasystem ● CS whose domain is another CS ● Reflective system ● Metasystem causally connected to itself Éric Tanter – U.Chile [june | 2005] 37 A Computational System causal connection data reason and act upon world program executer Éric Tanter – U.Chile [june | 2005] 38 A Metasystem data data world program program executer executer metasystem base system Éric Tanter – U.Chile [june | 2005] 39 A Reflective System data world program executer Éric Tanter – U.Chile [june | 2005] 40 Metalevels and towers ●
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages112 Page
-
File Size-