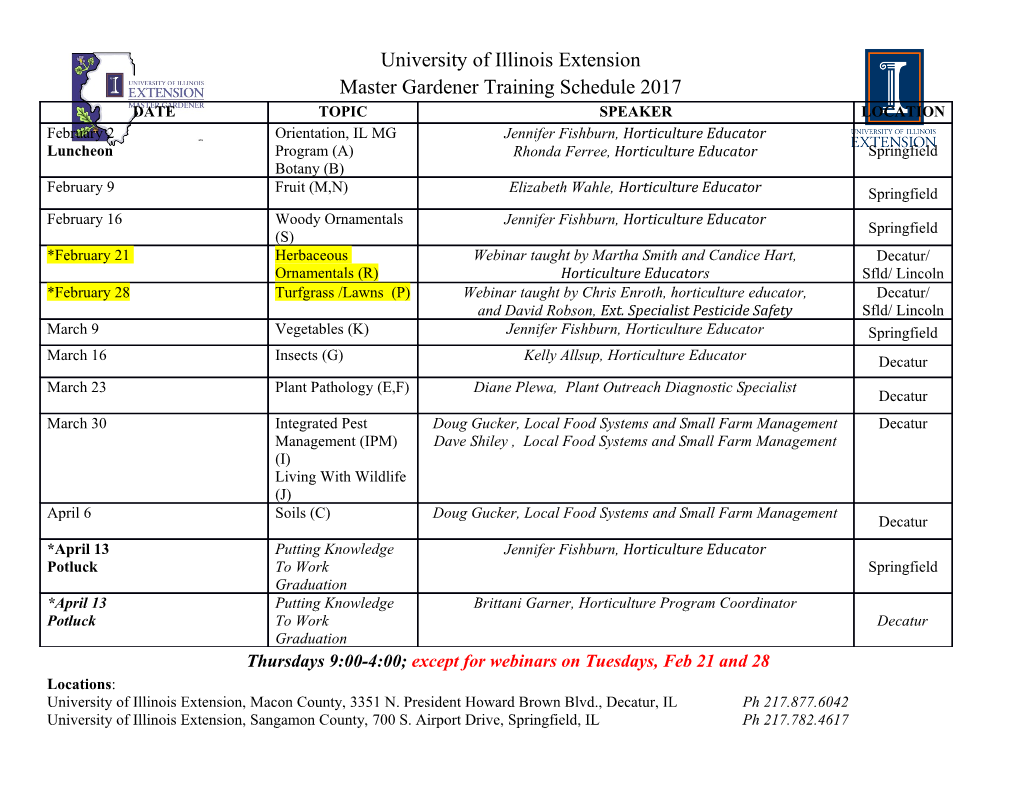
INTRODUCTION TO COMPUTER GRAPHICS DAVID J. ECK Introduction to Computer Graphics Version 1.2, January 2018 David J. Eck Hobart and William Smith Colleges This is a PDF version of a free, on-line book that is available at http://math.hws.edu/graphicsbook/. The web site includes source code for all example programs. ii c 2015–2018, David J. Eck David J. Eck ([email protected]) Department of Mathematics and Computer Science Hobart and William Smith Colleges Geneva, NY 14456 This book can be distributed in unmodified form for non-commercial purposes. Modified versions can be made and distributed for non-commercial purposes provided they are distributed under the same license as the original. More specifically: This work is licensed under the Creative Commons Attribution- NonCommercial-ShareAlike 4.0 License. To view a copy of this license, visit http://creativecommons.org/licenses/by-nc-sa/4.0/. Other uses require permission from the author. The web site for this book is: http://math.hws.edu/graphicsbook Contents Preface ix 1 Introduction 1 1.1 PaintingandDrawing .............................. ... 1 1.2 Elementsof3DGraphics. .... 4 1.3 HardwareandSoftware ............................. ... 6 2 Two-Dimensional Graphics 11 2.1 Pixels,Coordinates,andColors . ........ 11 2.1.1 PixelCoordinates .............................. 11 2.1.2 Real-numberCoordinateSystems. ..... 14 2.1.3 AspectRatio .................................. 16 2.1.4 ColorModels .................................. 17 2.2 Shapes.......................................... 19 2.2.1 BasicShapes .................................. 20 2.2.2 StrokeandFill ................................. 22 2.2.3 Polygons,Curves,andPaths . 24 2.3 Transforms ...................................... 26 2.3.1 ViewingandModeling . 26 2.3.2 Translation ................................... 29 2.3.3 Rotation..................................... 30 2.3.4 CombiningTransformations . 31 2.3.5 Scaling ..................................... 32 2.3.6 Shear ...................................... 33 2.3.7 Window-to-Viewport. 34 2.3.8 MatricesandVectors. 35 2.4 HierarchicalModeling . ..... 36 2.4.1 BuildingComplexObjects. 36 2.4.2 SceneGraphs.................................. 40 2.4.3 TheTransformStack. 43 2.5 JavaGraphics2D .................................. 44 2.5.1 Graphics2D................................... 45 2.5.2 Shapes...................................... 46 2.5.3 StrokeandFill ................................. 49 2.5.4 Transforms ................................... 51 2.5.5 BufferedImageandPixels . 53 2.6 HTMLCanvasGraphics .............................. 54 2.6.1 The2DGraphicsContext . 55 iii iv CONTENTS 2.6.2 Shapes...................................... 56 2.6.3 StrokeandFill ................................. 58 2.6.4 Transforms ................................... 60 2.6.5 AuxiliaryCanvases. 61 2.6.6 PixelManipulation. 62 2.6.7 Images...................................... 64 2.7 SVG:ASceneDescriptionLanguage . ...... 66 2.7.1 SVGDocumentStructure . 66 2.7.2 Shapes,Styles,andTransforms . ..... 69 2.7.3 PolygonsandPaths .............................. 71 2.7.4 HierarchicalModels . 72 2.7.5 Animation.................................... 74 3 OpenGL 1.1: Geometry 77 3.1 ShapesandColorsinOpenGL1.1 . 77 3.1.1 OpenGLPrimitives .............................. 78 3.1.2 OpenGLColor ................................. 80 3.1.3 glColorandglVertexwithArrays . ..... 83 3.1.4 TheDepthTest................................. 85 3.2 3DCoordinatesandTransforms. ...... 87 3.2.1 3DCoordinates................................. 87 3.2.2 Basic3DTransforms............................. 88 3.2.3 HierarchicalModeling . 90 3.3 ProjectionandViewing ............................ 93 3.3.1 ManyCoordinateSystems. 93 3.3.2 TheViewportTransformation. 95 3.3.3 TheProjectionTransformation . ..... 96 3.3.4 TheModelviewTransformation . 100 3.3.5 ACameraAbstraction. 103 3.4 PolygonalMeshesandglDrawArrays . .......105 3.4.1 IndexedFaceSets ............................... 105 3.4.2 glDrawArraysandglDrawElements. 109 3.4.3 DataBuffersinJava .............................. 111 3.4.4 DisplayListsandVBOs . 113 3.5 SomeLinearAlgebra............................... 115 3.5.1 VectorsandVectorMath . 115 3.5.2 MatricesandTransformations. 117 3.5.3 HomogeneousCoordinates. 119 3.6 UsingGLUTandJOGL ................................ 120 3.6.1 UsingGLUT ..................................121 3.6.2 UsingJOGL ..................................127 3.6.3 Aboutglsim.js ................................. 132 4 OpenGL 1.1: Light and Material 133 4.1 IntroductiontoLighting . 133 4.1.1 LightandMaterial.............................. 134 4.1.2 LightProperties ............................... 136 4.1.3 NormalVectors................................. 137 CONTENTS v 4.1.4 TheOpenGLLightingEquation . 139 4.2 LightandMaterialinOpenGL1.1 . 142 4.2.1 WorkingwithMaterial. 142 4.2.2 DefiningNormalVectors. 144 4.2.3 WorkingwithLights ............................. 147 4.2.4 GlobalLightingProperties . 149 4.3 ImageTextures ................................... 151 4.3.1 TextureCoordinates . 152 4.3.2 MipMapsandFiltering . 154 4.3.3 Texture Target and Texture Parameters . 155 4.3.4 TextureTransformation . 157 4.3.5 LoadingaTexturefromMemory . 158 4.3.6 TexturefromColorBuffer. 158 4.3.7 TextureObjects ................................ 159 4.3.8 LoadingTexturesinC . .. .. .. .. .. .. 161 4.3.9 UsingTextureswithJOGL . 162 4.4 Lights,Camera,Action ............................ 164 4.4.1 AttributeStack................................ 164 4.4.2 MovingCamera.................................166 4.4.3 MovingLight ..................................168 5 Three.js: A 3D Scene Graph API 171 5.1 Three.jsBasics .................................. 171 5.1.1 Scene,Renderer,Camera . 172 5.1.2 THREE.Object3D ...............................174 5.1.3 Object,Geometry,Material . 176 5.1.4 Lights ......................................182 5.1.5 AModelingExample.............................. 185 5.2 BuildingObjects ................................. 187 5.2.1 IndexedFaceSets ............................... 187 5.2.2 CurvesandSurfaces ............................. 191 5.2.3 Textures.....................................194 5.2.4 Transforms ...................................197 5.2.5 LoadingJSONModels............................. 198 5.3 OtherFeatures ................................... 199 5.3.1 AnaglyphStereo ................................ 199 5.3.2 UserInput ...................................200 5.3.3 Shadows.....................................204 5.3.4 CubemapTexturesandSkyboxes. 206 5.3.5 ReflectionandRefraction . 208 6 Introduction to WebGL 213 6.1 TheProgrammablePipeline. 213 6.1.1 TheWebGLGraphicsContext . 214 6.1.2 TheShaderProgram.............................. 215 6.1.3 DataFlowinthePipeline . 217 6.1.4 ValuesforUniformVariables . 220 6.1.5 ValuesforAttributes. 220 vi CONTENTS 6.1.6 DrawingaPrimitive ............................. 223 6.2 FirstExamples ................................... 224 6.2.1 WebGLContextOptions . 224 6.2.2 ABitofGLSL .................................225 6.2.3 TheRGBTriangleinWebGL. 226 6.2.4 ShapeStamper .................................229 6.2.5 ThePOINTSPrimitive ............................ 233 6.2.6 WebGLErrorHandling . 234 6.3 GLSL ..........................................235 6.3.1 BasicTypes...................................235 6.3.2 DataStructures................................ 237 6.3.3 Qualifiers ....................................238 6.3.4 Expressions ................................... 241 6.3.5 FunctionDefinitions . 242 6.3.6 ControlStructures ............................. 243 6.3.7 Limits......................................244 6.4 ImageTextures ................................... 245 6.4.1 TextureUnitsandTextureObjects. 245 6.4.2 WorkingwithImages ............................. 249 6.4.3 MoreWaystoMakeTextures . 252 6.4.4 CubemapTextures............................... 254 6.5 Implementing2DTransforms . 257 6.5.1 TransformsinGLSL .............................. 257 6.5.2 TransformsinJavaScript . 258 7 3D Graphics with WebGL 263 7.1 Transformationsin3D ............................. 263 7.1.1 AboutShaderScripts ............................ 263 7.1.2 IntroducingglMatrix. 264 7.1.3 TransformingCoordinates . 267 7.1.4 TransformingNormals . 268 7.1.5 RotationbyMouse............................... 270 7.2 LightingandMaterial ............................. 272 7.2.1 MinimalLighting............................... 273 7.2.2 SpecularReflectionandPhongShading . 275 7.2.3 AddingComplexity .............................. 278 7.2.4 Two-sidedLighting. 279 7.2.5 MovingLights .................................281 7.2.6 Spotlights.................................... 282 7.2.7 LightAttenuation .............................. 284 7.2.8 Diskworld2...................................285 7.3 Textures........................................ 286 7.3.1 TextureTransformswithglMatrix . 286 7.3.2 GeneratedTextureCoordinates . 287 7.3.3 ProceduralTextures . 289 7.3.4 Bumpmaps ...................................292 7.3.5 EnvironmentMapping . 295 CONTENTS vii 7.4 Framebuffers ..................................... 299 7.4.1 FramebufferOperations . 299 7.4.2 RenderToTexture............................... 302 7.4.3 Renderbuffers.................................. 304 7.4.4 DynamicCubemapTextures . 304 7.5 WebGLExtensions................................. 307 7.5.1 AnisotropicFiltering. 308 7.5.2 Floating-PointColors . 309 7.5.3 DeferredShading............................... 312 8 Beyond Realtime Graphics 315 8.1 RayTracing.....................................
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages441 Page
-
File Size-