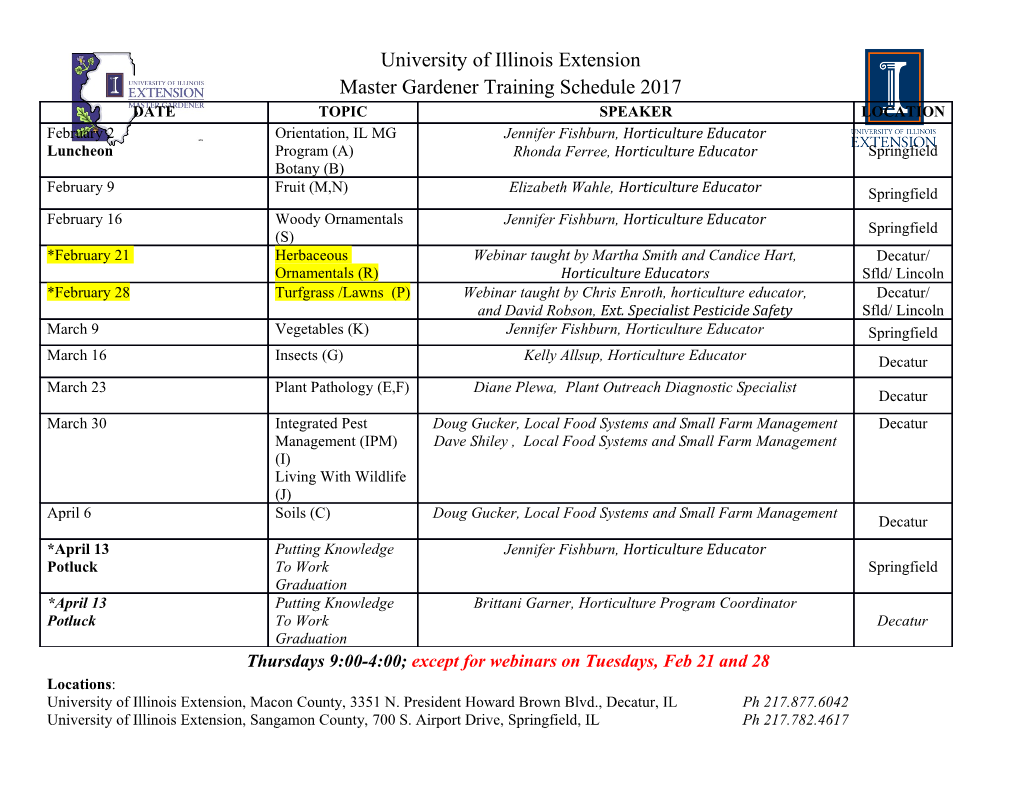
Mastering C# and .NET Framework Deep dive into C# and .NET architecture to build efficient, powerful applications Marino Posadas BIRMINGHAM - MUMBAI Mastering C# and .NET Framework Copyright © 2016 Packt Publishing All rights reserved. No part of this book may be reproduced, stored in a retrieval system, or transmitted in any form or by any means, without the prior written permission of the publisher, except in the case of brief quotations embedded in critical articles or reviews. Every effort has been made in the preparation of this book to ensure the accuracy of the information presented. However, the information contained in this book is sold without warranty, either express or implied. Neither the author, nor Packt Publishing, and its dealers and distributors will be held liable for any damages caused or alleged to be caused directly or indirectly by this book. Packt Publishing has endeavored to provide trademark information about all of the companies and products mentioned in this book by the appropriate use of capitals. However, Packt Publishing cannot guarantee the accuracy of this information. First published: December 2016 Production reference: 1091216 Published by Packt Publishing Ltd. Livery Place 35 Livery Street Birmingham B3 2PB, UK. ISBN 978-1-78588-437-5 www.packtpub.com Credits Author Project Coordinator Marino Posadas Izzat Contractor Reviewers Proofreader Fabio Claudio Ferracchiati Safis Editing Commissioning Editor Indexer Edward Gordon Rekha Nair Acquisition Editor Graphics Denim Pinto Disha Haria Content Development Editor Production Coordinator Priyanka Mehta Aparna Bhagat Technical Editor Cover Work Dhiraj Chandanshive Aparna Bhagat Copy Editor Stuti Srivastava About the Author Marino Posadas is an independent senior trainer, writer, and consultant in Microsoft Technologies and Web Standards. He is a Microsoft MVP in C#, Visual Studio, and Development Technologies; an MCT, MAP (2013), MCPD, MCTS, MCAD, MCSD, and MCP. Additionally, he was the former director for development in Spain and Portugal for Solid Quality Mentors. He's published 14 books and more than 500 articles on development technologies in several magazines. Topics covered in his books range from Clipper and Visual Basic 5.0/6.0 to C #, .NET-safe programming, Silverlight 2.0 and 4.0, and Web Standards. The Guide to Programming in HTML5, CSS3 and JavaScript with Visual Studio is his latest book. He is also a speaker at Microsoft events, having lectured in Spain, Portugal, England, the USA, Costa Rica, and Mexico. You can find him on LinkedIn at https://es.linkedin.com/in/mposadas. His website, http//elavefenix.net, also contains developer's resources and videos, in English and Spanish, interviewing representatives of the Microsoft and Web Standards development world. Acknowledgements I'd like to thank Denim Pinto, Priyanka Mehta, and Fabio Claudio Ferracchiati from Packt Publishing for their continuous support and confidence while writing this book. Special thanks to some professionals and technology evangelists whose work inspired different parts of this book, in particular, Mark Russinowich, Scott Hanselman, Scott Hunter (the "lesser" Scotts), Daniel Roth, Lluis Franco, Luis Ruiz Pavón, Dino Esposito, Miguel Katrib, James Gray, Paul Cotton, Stephen Toub, and Troy Hunt. Also, I would like to remember my MVP lead, Cristina González Herrero, for her continuous encouragement and help, and other people at Microsoft who have always supported my activities. My memories go here to Alfonso Rodríguez, David Carmona, David Salgado, José Bonnín, César de la Torre, Andy Gonzalez, and Leon Welicki. My appreciation also goes to my mates at Netmind, Alex and Bernat Palau, Miquel Rodriguez, Israel Zorrilla, and Angel Rayo, for their support in this initiative from the beginning. About the Reviewer Fabio Claudio Ferracchiati is a senior consultant and a senior analyst/ developer using Microsoft technologies. He works for React Consulting (www. reactconsulting.it) as Microsoft Dynamics 365 Solution Architect. He is a Microsoft Certified Solution Developer for .NET, a Microsoft Certified Application Developer for .NET, a Microsoft Certified Professional, and a prolific author and technical reviewer. Over the past 10 years, he's written articles for Italian and international magazines and coauthored more than 10 books on a variety of computer topics. www.PacktPub.com eBooks, discount offers, and more Did you know that Packt offers eBook versions of every book published, with PDF and ePub files available? You can upgrade to the eBook version at www.PacktPub. com and as a print book customer, you are entitled to a discount on the eBook copy. Get in touch with us at [email protected] for more details. At www.PacktPub.com, you can also read a collection of free technical articles, sign up for a range of free newsletters and receive exclusive discounts and offers on Packt books and eBooks. https://www.packtpub.com/mapt Get the most in-demand software skills with Mapt. Mapt gives you full access to all Packt books and video courses, as well as industry-leading tools to help you plan your personal development and advance your career. Why subscribe? • Fully searchable across every book published by Packt • Copy and paste, print, and bookmark content • On demand and accessible via a web browser I dedicate this book to my wife, Milagros, and my family, with a special mention to my nephews and nieces: Fernando, Sarah, Ana, Paula, Pablo, Javier, Adrian, Irene, Luis, and Juan. Table of Contents Preface xi Chapter 1: Inside the CLR 1 An annotated reminder of some important computing terms 2 Context 2 The OS multitask execution model 2 Context types 3 Thread safety 3 State 4 Program state 4 Serialization 4 Process 5 Thread 6 SysInternals 8 Static versus dynamic memory 9 Garbage collector 10 Concurrent computing 11 Parallel computing 11 Imperative programming 11 Declarative programming 12 The evolution of .NET 12 .NET as a reaction to the Java World 13 The open source movement and .NET Core 13 Common Language Runtime 15 Common Intermediate Language 16 Managed execution 16 Components and languages 17 Structure of an assembly file 18 [ i ] Table of Contents Metadata 21 Introducing metadata with a basic Hello World 22 PreJIT, JIT, EconoJIT, and RyuJIT 27 Common Type System 29 A quick tip on the execution and memory analysis of an assembly in Visual Studio 2015 32 The stack and the heap 33 Garbage collection 37 Implementing algorithms with the CLR 44 Data structures, algorithms, and complexity 44 Big O Notation 45 Relevant features appearing in versions 4.5x, 4.6, and .NET Core 1.0 and 1.1 49 .NET 4.5.x 49 .NET 4.6 (aligned with Visual Studio 2015) 50 .NET Core 1.0 50 .NET Core 1.1 51 Summary 52 Chapter 2: Core Concepts of C# and .NET 53 C# – what's different in the language? 53 Languages: strongly typed, weakly typed, dynamic, and static 54 The main differences 56 The true reason for delegates 59 The evolution in versions 2.0 and 3.0 64 Generics 64 Creating custom generic types and methods 66 Lambda expressions and anonymous types 71 Lambda expressions 72 The LINQ syntax 75 LINQ syntax is based on the SQL language 76 Deferred execution 77 Joining and grouping collections 78 Type projections 80 Extension methods 81 Summary 82 Chapter 3: Advanced Concepts of C# and .NET 83 C# 4 and .NET framework 4.0 84 Covariance and contravariance 84 Covariance in interfaces 86 Covariance in generic types 89 Covariance in LINQ 89 Contravariance 90 Tuples: a remembrance 92 [ ii ] Table of Contents Tuples: implementation in C# 93 Tuples: support for structural equality 94 Tuples versus anonymous types 94 Lazy initialization and instantiation 97 Dynamic programming 100 Dynamic typing 100 The ExpandoObject object 103 Optional and named parameters 105 The Task object and asynchronous calls 106 C# 5.0: async/await declarations 109 What's new in C# 6.0 110 String interpolation 110 Exception filters 111 The nameof operator 112 The null-conditional operator 113 Auto-property initializers 115 Static using declarations 115 Expression bodied methods 117 Index initializers 118 What's new in C# 7.0 119 Binary literals and digit separators 119 Pattern matching and switch statements 120 Tuples 122 Decomposition 124 Local functions 125 Ref return values 126 Summary 127 Chapter 4: Comparing Approaches for Programming 129 Functional languages 130 F# 4 and .NET Framework 132 The inevitable Hello World demo 133 Identifiers and scope 136 Lists 137 The TypeScript language 144 The new JavaScript 145 TypeScript: a superset of JavaScript 147 So, what exactly is TypeScript? 148 Main features and coalitions 148 Installing the tools 149 Transpiling to different versions 152 Advantages in the IDE 153 [ iii ] Table of Contents A note on TypeScript's object-oriented syntax 155 More details and functionality 155 Summary 156 Chapter 5: Reflection and Dynamic Programming 157 Reflection in the .NET Framework 158 Calling external assemblies 164 Generic Reflection 166 Emitting code at runtime 168 The System.CodeDOM namespace 168 The Reflection.Emit namespace 171 Interoperability 173 Primary Interop Assemblies 174 Formatting cells 179 Inserting multimedia in a sheet 180 Interop with Microsoft Word 185 Office apps 190 The Office app default project 191 Architectural differences 194 Summary 197 Chapter 6: SQL Database Programming 199 The relational model 200 Properties of relational tables 200 The tools – SQL Server 2014 203 The SQL language 206 SQL Server from Visual Studio 207 Data access in Visual Studio 213 .NET data
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages560 Page
-
File Size-