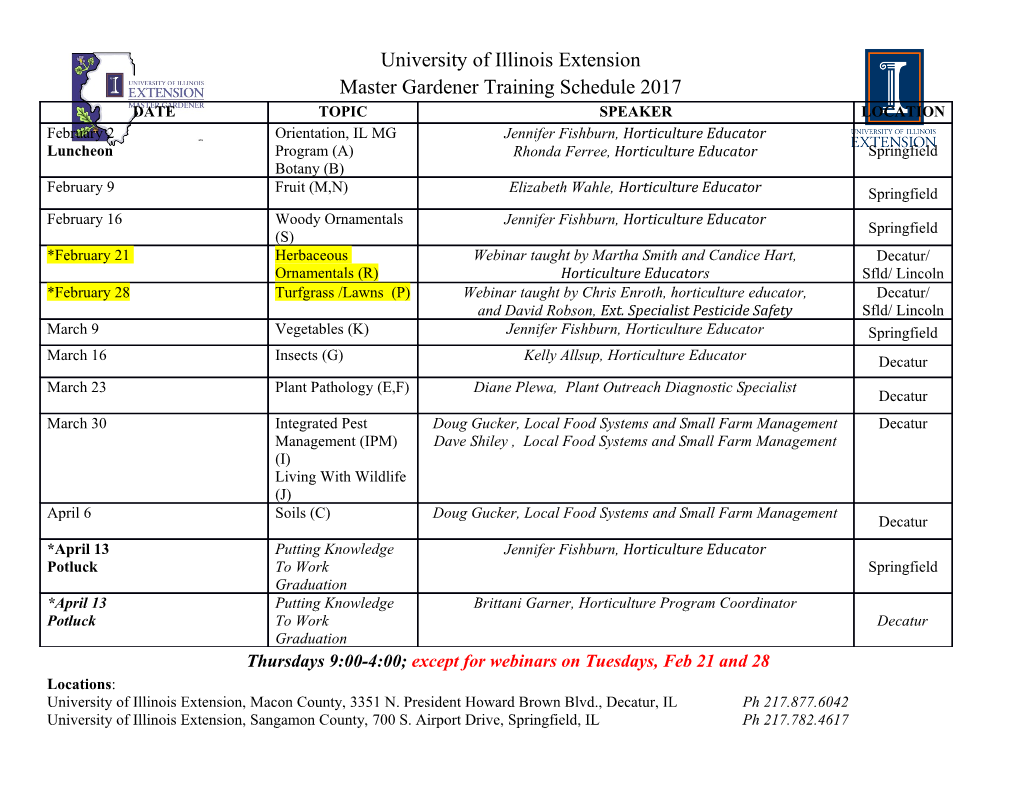
HTML5 Video Video Codecs and File Formats Video codec – compression/decompression program that transforms streams of data into video files that can be played back objective is to maintain high quality video while reducing file size VideoCodec File Formats theora ogg ◦ open source, www.xiph.org ◦ ogg is the container format ◦ theora the the name of the codec algorithm ◦ popular for gaming mp4 (h.264) ◦ Developed by Motion Picture Expert Group (www.mpeg.com) ◦ patented and proprietary ◦ used by YouTube webm ◦ project of www.webmproject.org ◦ Supported by Mozilla, Opera, Adobe, Google ◦ high quality, royalty-free, open video format ◦ compressed with VP8 coding ◦ Google acquired rights to VP8 ◦ VP8 used with WebM container Video Formats and Browser Support Check out https://www.w3schools.com/html/tryit.asp?filename=tryhtml5_video_autoplay Browser Support for Audio and Video Formats Encoding Your Video File Converter programs (some examples) ◦ Miro Video Converter www.mirovideoconverter.com desktop application converts to theora ogg, mp4, webm runs on Windows and Mac ◦ Media Converter www.mediaconverter.org online application converts to mp4, Flash flv and theora ogg ◦ Handbrake http://handbrake.fr open source converts to mp4 and theora ogg runs on Windows, Mac and Linux The Challenge of Video Before html5 video inclusion was complex The <video> element was introduced to simplify this Example: <!DOCTYPE html> <html lang="en"> <head> <title>Video Player</title> </head> <body> <section id="player"> <video src="trailer.mp4" controls> </video> </section> </body> </html> The <video> element width and height (the size of the video will be adjusted to fit) separate <source> tags are required for both types of video. the browser decides which one to play <!DOCTYPE html> <html lang="en"> <head> <title>Video Player</title> </head> <body> <section id="player"> <video id="media" width="720" height="400" controls> <source src="trailer.mp4"> <source src="trailer.ogg"> </video> </section> </body> </html> <video> attributes controls ◦ shows video controls provided by browsers autoplay ◦ the browser will automatically play the video loop ◦ when the video reaches an end it restarts poster ◦ provides the URL of an image that is displayed while waiting for the video to load preload ◦ none – do not cache the video ◦ metadata – browser fetches information before loading ◦ auto – prompts the browser to download the file as soon as possible Playing a Video File To play a webm file ◦ <video src="snow.webm" controls> </video> Starts playing automatically ◦ <video src="snow.webm" controls autoplay> </video> Start playing and repeat (bad idea?) <video src="snow.webm" controls autoplay loop> </video> ◦ forces modality on the user ◦ might not sync perfectly with audio on looping and play over audio that is inserted for accessibility reasons Playing a Video File (continued) Play video but mute the audio ◦ <video src="snow.webm" controls autoplay muted> </video> Do not load, let user run it when they want to ◦ <video src="snow.webm" controls preload="none"> </video> Hardcoding the size <video src="snow.webm" controls width=300 height=210> </video> Removing the controls <video src="snow.webm"> </video> The type Attribute The type attribute specifies the format of the src file type attributes can also contain the actual codec. This allows the browser to decide whether it can play it. examples ◦ <source src="snow.ogg" type='video/ogg; codecs="theora, vorbis"'> ◦ <source src="snow.webm" type='video/webm; codecs="vp8, vorbis"'> ◦ <source src="snow.mp4" type='video/mp4; codecs="mpa.40.2"'> poster images To display a poster image first, before the video plays, do the following: ◦ <video controls poster="snow-poster.gif" width="300" height="210"> ◦ <source src="snow.mp4" type=video/mp4> ◦ <source src="snow.webm" type=video/webm> ◦ </video> <video> attributes <!DOCTYPE html> <html lang="en"> <head> <title>Video Player</title> </head> <body> <section id="player"> <video id="media" width="720" height="400" preload controls loop poster="poster.jpg"> <source src="trailer.mp4"> <source src="trailer.ogg"> </video> </section> </body> </html> Problem Some <video> attributes work on some browsers but not others Some will work in a browser only under certain circumstances To have full control over these we must write the code to make our own video controls in javascript Chrome Firefox IE Programming a Video Player Notice that the graphic design for the video player controls differs in each browser. Making a control panel ◦ use the <nav> element to contain two <divs> one <div> contains the buttons one <div> for the progress bar <!DOCTYPE html> <html lang="en"> <head> <title>Video Player</title> <link rel="stylesheet" href="videodemo.css"> <script src="videodemo.js"></script> </head> <body> <section id="player"> <video id="media" width="720" height="400"> <source src="trailer.mp4"> <source src="trailer.ogg"> </video> <nav> <div id="buttons"> <button type="button" id="play">Play</button> </div> <div id="bar"> <div id="progress"></div> </div> <div style="clear: both"></div> </nav> </section> </body> </html> Playing a Video File with Different Sources and Legacy Fallback Legacy browsers need video formats that address formats they can play This embedded object element enables the Flash player It also enables the user to download the file if the want Example <video controls autoplay> <source src=" snow.mp4 " type="audio/mp4"> <source src=" snow.mp4 " type="audio/webm"> <object type="application/x-shockwave-flash" data="player.swf?audioUrl=snow.mp4&autoPlay=true" height="210" width="300" > <param name="movie" value="player.swf?audioUrl=snow.mp4&autoPlay=true"> </object> <a href=" snow.mp4 "> Download the video: snow.mp4</a> </video> CSS Analysis Notice that the width for the <div> element of the progress bar It is set to 0 This is because this element is used to simulate a progress bar that updates as the video is being played. The Stylesheet body{ text-align: center; } header, section, footer, aside, nav, article, figure, figcaption, hgroup{ display : block; } #player{ width: 720px; margin: 20px auto; padding: 5px; background: #999999; border: 1px solid #666666; -moz-border-radius: 5px; -webkit-border-radius: 5px; border-radius: 5px; } Stylesheet for <nav> bar nav{ margin: 5px 0px; } #buttons{ float: left; width: 85px; height: 20px; } #bar{ position: relative; float: left; width: 600px; height: 16px; padding: 2px; border: 1px solid #CCCCCC; background: #EEEEEE; } #progress{ position: absolute; width: 0px; height: 16px; background: rgba(0,0,150,.2); } Javascript Code We need to create the necessary controls, events, methods and properties We will add a few simple controls ◦ play ◦ pause ◦ progress bar clickable to rewind or forward Video Editing on-the-fly Not supported evenly across browsers ◦ Security risks ◦ IE – yes ◦ FF – no ◦ chrome - no function initiate(){ var elem=document.getElementById('canvas'); canvas=elem.getContext('2d'); video=document.getElementById('media'); video.addEventListener('click', push, false); } function push(){ if(!video.paused && !video.ended){ video.pause(); window.clearInterval(loop); inverts the colors of the }else{ video while it is running video.play(); IE only loop=setInterval(processFrames, 33); } } function processFrames(){ canvas.drawImage(video,0,0); var info=canvas.getImageData(0,0,483,272); var pos; for(x=0;x<=483;x++){ for(y=0;y<=272;y++){ pos=(info.width*4*y)+(x*4); info.data[pos]= 255 - info.data[pos]; info.data[pos+1]= 255 - info.data[pos+1]; info.data[pos+2]= 255 - info.data[pos+2]; } } canvas.putImageData(info,0,0); } window.addEventListener("load", initiate, false); Targeting Devices with Different Video Files Using Media Types and Queries You may want to serve up different video files depending on the device ◦ if the device is a mobile phone then use the smaller version ◦ to do this you want to use a media query Media queries were introduced with CSS2 (www.w3.org/TR/CSS2/media.html) Media Types all – all devices braille – for braille tactile feedback devices embossed – for braille page printers handheld – mobile phones print – for paged material and print preview projection – for projected presentations screen – for color computer screens speech – for speech synthesisers tty – for terminal devices with fixed pitch character grid tv – television devices Media Query Device Features Feature Definition width Width of the display area height Height of the display area device-width Width of the rendering area device-height Height of the rendering area orientation Portrait or landscape aspect-ratio Ratio of target width to height device-aspect-ratio Ratio of device-width to device-height resolution Density of pixels in this device color Number of bits per color component color-index Number of entries in color lookup table grid Tests if the device is grid-based monochrome Number of bits per pixel in monochrome device scan For tv browsing: progressive or scan Media Queries created by the w3c ◦ www.w3.org/TR/css3-mediaqueries ◦ check for conditions of media features ◦ useful when detecting mobile devices you can combine media types and devices <source src="snow-small.mp4" type="video/mp4" media="all and (max-width:600px)"> Example 1 Serving video to all media types with a maximum of 600 pixels <video controls> <source src="snow-small.mp4" type="video/mp4" media="all and (max-width:600px)"> <source src="snow-small.webm" type="video/webm" media="all and (max-width:600px)">
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages40 Page
-
File Size-