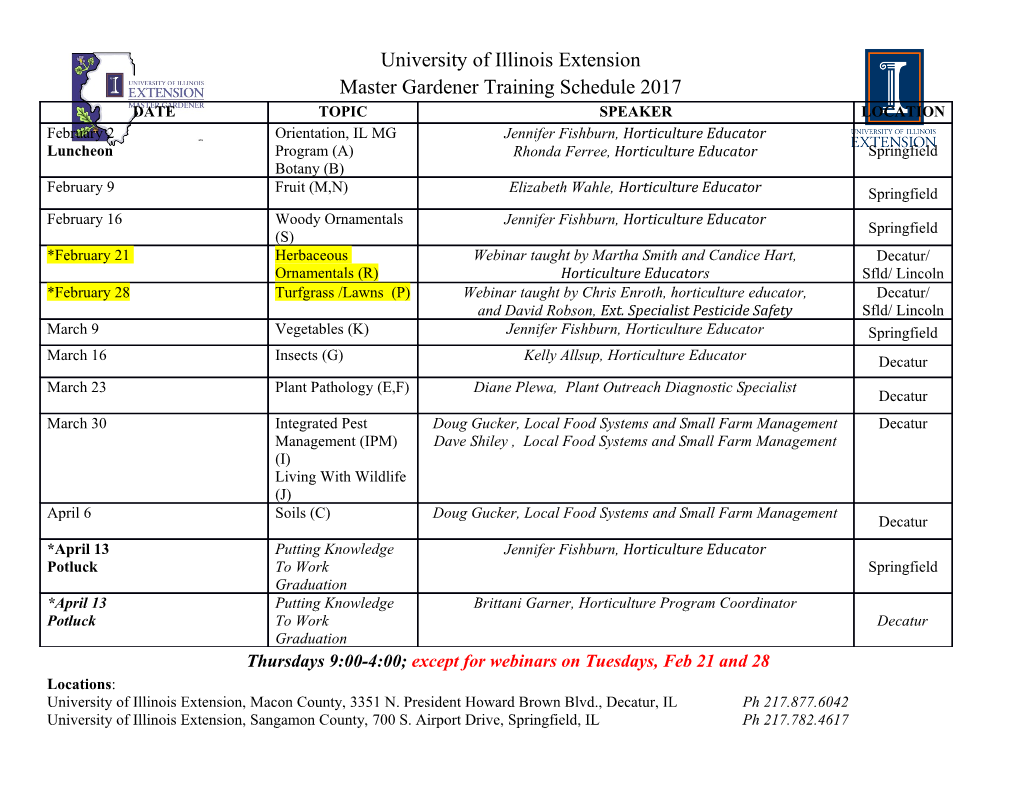
Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Agenda 1. Data Types 2. Unsigned & Signed Integers 3. Arithmetic Operations Chapter 2 4. Logical Operations 5. Shifting Bits, Data Types, 6. Hexadecimal & Octal Notation and Operations 7. Other Data Types COMPSCI210 S1C 2009 2 Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. 1. Data Types Computer is a binary digital system. How do we represent data in a computer? Digital system: Binary (base two) system: At the lowest level, a computer is an electronic machine. • finite number of symbols • has two states: 0 and 1 • works by controlling the flow of electrons Easy to recognize two conditions: 1. presence of a voltage – we’ll call this state “1” 2. absence of a voltage – we’ll call this state “0” Basic unit of information is the binary digit, or bit. Values with more than two states require multiple bits. • A collection of two bits has four possible states: Could base state on value of voltage, 00, 01, 10, 11 but control and detection circuits more complex. • A collection of three bits has eight possible states: • compare turning on a light switch to 000, 001, 010, 011, 100, 101, 110, 111 measuring or regulating voltage • A collection of n bits has 2n possible states. COMPSCI210 S1C 2009 3 COMPSCI210 S1C 2009 4 1 Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. What kinds of data do we need to represent? 2. Unsigned & Signed Integers Decimal Numbers • Numbers – signed, unsigned, integers, floating point, complex, rational, irrational, … “decimal” means that we have ten digits to use in our • Text – characters, strings, … representation (the symbols 0 through 9) • Images – pixels, colors, shapes, … • Sound What is 3,546? • Logical – true, false • it is three thousands plus five hundreds plus four tens plus six ones. • Instructions • i.e. 3,546 = 3.103 + 5.102 + 4.101 + 6.100 • … How about negative numbers? • we use two more symbols to distinguish positive and negative: Data type: + and – • representation and operations within the computer Note that not everyone uses base 10 We’ll start with numbers… • Vigesimal (base 20)http://en.wikipedia.org/wiki/Vigesimal COMPSCI210 S1C 2009 5 COMPSCI210 S1C 2009 6 Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Unsigned Integers Unsigned Binary Integers Non-positional notation Y = “abc” = a.22 + b.21 + c.20 • could represent a number (“5”) with a string of ones (“11111”) • problems? (where the digits a, b, c can each take on the values of 0 or 1 only) N = number of bits 3-bits 5-bits 8-bits Weighted positional notation 0 000 00000 00000000 • like decimal numbers: “329” Range is: • “3” is worth 300, because of its position, while “9” is only worth 9 0 i < 2N - 1 1 001 00001 00000001 2 010 00010 00000010 most least significant significant 3 011 00011 00000011 329 101 Problem: 4 100 00100 00000100 102 101 100 22 21 20 • How do we represent 3x100 + 2x10 + 9x1 = 329 1x4 + 0x2 + 1x1 = 5 negative numbers? COMPSCI210 S1C 2009 7 COMPSCI210 S1C 2009 8 2 Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Unsigned Binary Arithmetic Signed Integers Base-2 addition – just like base-10! With n bits, we have 2n distinct values. • add from right to left, propagating carry • assign about half to positive integers (1 through 2n-1) and about half to negative (- 2n-1 through -1) carry • that leaves two values: one for 0, and one extra Positive integers • just like unsigned – zero in most significant (MS) bit 00101 = 5 Negative integers • sign-magnitude – set MS bit to show negative, other bits are the same as unsigned 10101 = -5 -1 * (0×23 + 1×22 + 0×21 + 1×20) • one’s complement – flip every bit to represent negative 11010 = -5 (-00101) Subtraction, multiplication, division,… • in either case, MS bit indicates sign: 0=positive, 1=negative COMPSCI210 S1C 2009 9 COMPSCI210 S1C 2009 10 Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Signed Magnitude One’s Complement Leading bit is the sign bit -3 111 Invert all bits -3 100 -2 110 -2 101 Y = “abc” = (-1)a (b.21 + c.20) -1 101 If msb (most significant bit) is 1 then the -1 110 -0 100 number is negative (same as signed -0 111 Range is: magnitude) -2N-1 + 1 <= i <= 2N-1 - 1 +0 000 +0 000 +1 001 Range is: +1 001 E.g. 3 bits: -3 <= i <= 3 +2 010 -2N-1 + 1 <= i <= 2N-1 - 1 +2 010 Problems: +3 011 +3 011 E.g. 3 bits: -3 <= i <= 3 • How do we do addition/subtraction? Problems: • We have two numbers for zero (+/-)! •Same as for signed magnitude COMPSCI210 S1C 2009 11 COMPSCI210 S1C 2009 12 3 Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Two’s Complement Two’s Complement Representation Problems with sign-magnitude and 1’s complement If number is positive or zero, • two representations of zero (+0 and –0) • normal binary representation, zeroes in upper bit(s) • arithmetic circuits are complex If number is negative, How to add two sign-magnitude numbers? – e.g., try 2 + (-3) • start with positive number How to add to one’s complement numbers? • flip every bit (i.e., take the one’s complement) – e.g., try 4 + (-3) • then add one Two’s complement representation developed to make circuits easy for arithmetic. (5) (9) • for each positive number (X), assign value to its negative (-X), such that X + (-X) = 0 with “normal” addition, ignoring carry out (1’s comp) (1’s comp) (5) (9) (-5) (-9) (-5) (-9) (0) (0) COMPSCI210 S1C 2009 13 COMPSCI210 S1C 2009 14 Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Two’s Complement Shortcut Two’s Complement Signed Integers MS bit is sign bit – it has weight –2n-1. To take the two’s complement of a number: n-1 n-1 • copy bits from right to left until (and including) the first “1” Range of an n-bit number: -2 through 2 – 1. • flip remaining bits to the left • The most negative number (-2n-1) has no positive counterpart. -23 22 21 20 -23 22 21 20 0 0 0 0 0 1 0 0 0 -8 0 0 0 1 1 1 0 0 1 -7 (1’s comp) (flip) (copy) 0 0 1 0 2 1 0 1 0 -6 0 0 1 1 3 1 0 1 1 -5 0 1 0 0 4 1 1 0 0 -4 0 1 0 1 5 1 1 0 1 -3 0 1 1 0 6 1 1 1 0 -2 0 1 1 1 7 1 1 1 1 -1 COMPSCI210 S1C 2009 15 COMPSCI210 S1C 2009To go from n to –n: Two’s Complement conversion 16 4 Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Applet: Bin2Dec.html Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Converting Binary (2’s C) to Decimal More Examples 1. If leading bit is one, take two’s complement to get a positive number. X = 00100111two 5 2 1 0 2. Add powers of 2 that have “1” in the n 2n = 2 +2 +2 +2 = 32+4+2+1 n 2n corresponding bit positions. 0 1 = 39 0 1 1 2 ten 1 2 3. If original number was negative, 2 4 2 4 add a minus sign. 3 8 X = 11100110 3 8 4 16 two 4 16 5 32 -X = 00011010 5 32 4 3 1 X = 01101000two 6 64 = 2 +2 +2 = 16+8+2 6 64 6 5 3 7 128 7 128 = 2 +2 +2 = 64+32+8 8 256 = 26ten 8 256 = 104ten 9 512 X = -26 9 512 10 1024 ten 10 1024 Assuming 8-bit 2’s complement numbers. Assuming 8-bit 2’s complement numbers. COMPSCI210 S1C 2009 17 COMPSCI210 S1C 2009 18 Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Applet: Dec2Bin.html Copyright © The McGraw-Hill Companies, Inc. Permission required for reproduction or display. Converting Decimal to Binary (2’s C) Converting Decimal to Binary (2’s C) n 2n First Method: Division Second Method: Subtract Powers of Two 0 1 1. Find magnitude of decimal number. (Always positive.) 1 2 1. Find magnitude of decimal number. 2 4 2. Divide by two – remainder is least significant bit. 2. Subtract largest power of two 3 8 3. Keep dividing by two until answer is zero, less than or equal to number. 4 16 writing remainders from right to left. 5 32 4. Append a zero as the MS bit; 3. Put a one in the corresponding bit position. 6 64 if original number was negative, take two’s complement. 4. Keep subtracting until result is zero. 7 128 8 256 5. Append a zero as MS bit; 9 512 X = 104ten 104/2 = 52 r0 bit 0 52/2 = 26 r0 bit 1 if original was negative, take two’s complement.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages14 Page
-
File Size-