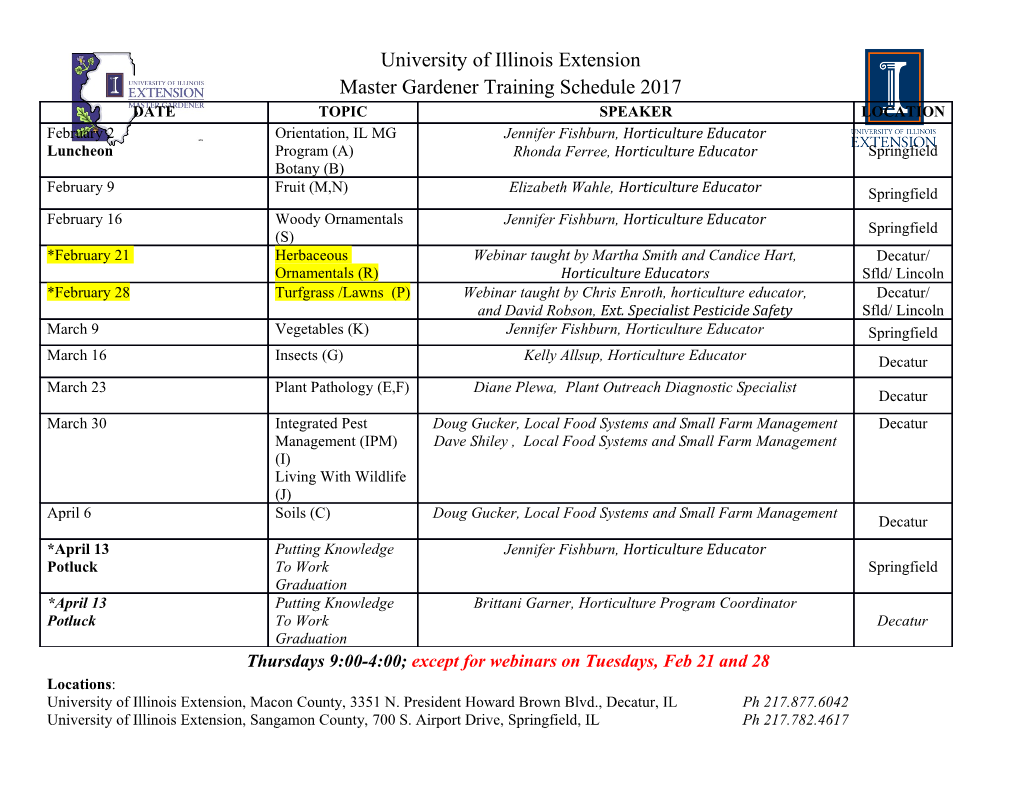
Journal of Technology Research Security vulnerabilities of the top ten programming languages: C, Java, C++, Objective-C, C#, PHP, Visual Basic, Python, Perl, and Ruby Stephen Turner Known-Quantity.com, part of Turner & Associates, Inc. ABSTRACT Programming languages are like genetics, in that there are a few ancestors with common traits that have proliferated. These can be traced back over time. This paper will explore the common traits between the top ten programming languages, which runs almost 80 percent of all of software used today. These common traits are both good and bad. In programming, the bad traits equate to security vulnerabilities that are often exploited by hackers. Many programmers are not aware of these flaws and do not take the time to take corrective action as they build software applications. They cannot simply fix the problems at the end; they must continually adjust how they program throughout the process. This paper will also provide guidance on what can be done to make computing environments more secure. Chief information officers (CIOs) have a responsibility to oversee all aspects of software development, and should consider assigning a project manager familiar with the security challenges inherent with a particular programming language. A CIO should also sign-off at every stage, starting with system conceptualization, system requirements of the system, analysis of benefits, and scope of project. The business and system analyses are critical and must include security parameters for programmers in software requirement specifications. Once the planning and design stages are approved, unit development, software and system integration, followed by testing and retesting, are essential. All of this precedes installation, site testing and acceptance, training/documentation, implementation and maintenance. Keywords: Software, Computer Programming, Applications, Security, System Analysis, CIO Copyright statement: Authors retain the copyright to the manuscripts published in AABRI journals. Please see the AABRI Copyright Policy at http://www.aabri.com/copyright.html. Security vulnerabilities of the top, page 1 Journal of Technology Research INTRODUCTION The National Vulnerability Database is a comprehensive website that allows risk managers and security professionals to track security problems, and rate the level of risk. There are 56,009 Common Vulnerabilities and Exposures (CVE) listed, and 2,708 US-CERT vulnerability notes, along with 245 US-CERT alerts (DHS National Cyber Security Division/US- CERT, 2013). Only a fraction of known problems are listed in CVE. According to Robert Seacord, 100,000 software vulnerabilities are identified in a given year, and 400,000 incidents occur during that same timeframe (Seacord, 2009). Seacord leads the secure coding initiative at the Software Engineering Institute. It is very difficult to keep up with so many threats, but there are some common themes that emerge again and again. Most problems are related to buffer overflows, un-validated input, race conditions, access-control problems, authentication or authorization weaknesses, or cryptographic practices (Mac Developer Library, 2013). This paper will analyze common errors that can be corrected with proper development processes. Over the past twenty-five years, some languages have been consistently popular among programmers, such as C, C++, Visual Basic, Perl, Lisp and Ada. According to the TIOBE Programming Community Index (TIOBE) (2013), other languages have grown in usage very rapidly, such as PHP and Ruby. Objective-C was ranked 45th most used in 2008, and it is now third. Java first appeared in the fourth position in 1998 and was number one by 2008. THE TOP TEN LANGUAGES Number One: Java Java remains most used in 2013; its praxis grew by 1.34 percent from the previous year, and 18.39 percent of lines of code are developed using Java (TIOBE Software, 2013). Naturally, the most popular language is subject to more scrutiny than others. Fred Long asserts that Java is secure if used properly, but engineers can misuse it or improperly implement it. Java is a multi- threaded programming language built on the type-safe model, which does not permit a buffer’s boundary to be superseded. Hoglund and McGraw (2004) assert that buffer overflows – in the classic sense – do not happen in Java because “falling off the end of an object and spilling elsewhere is not possible.” However, external code that interacts with Java – such as code written in C – is an exception (Hoglund & McGraw, 2004). Attackers understand that internal buffer overflow attacks are not easy, but have demonstrated that a type confusion attack could exploit a weakness by using a public field like a private field – as early as Java 5. This made Java’s Security Manager accessible to manipulation or foul play (Long, 2005). This is also known as a language-based attack. CERT has warned of log injection attacks in Java – often through web browsers. These attacks can be averted through validation, or authentication of submitted input. For example, attackers have manipulated Java by entering a Carriage Return and Line Feed (CRLF) sequence, which often deceives system administrators. Sanitizing the data assures that it matches the requirements of each field and keeps administrators alert to un-validated input (Mohrindra & Jumde, 2012). Unchecked input can include Structured Query Language (SQL) injection in Java (Livshits & Lam, 2013). Because Java is a multi-threaded programming language, it does enable deadlock and race conditions (Java Revisited, 2012). Deadlock occurs when two or more threads are waiting Security vulnerabilities of the top, page 2 Journal of Technology Research for each other, which can permanently obstruct progress (Java Tutorials, 2013). This is a bug, but not as much of a liability as are race conditions, which occur when synchronization has not been properly programmed. For example, check and act should start with a check thread and finish with an act thread. It is not uncommon that a null value is recorded when there is a delay that was not anticipated by the programmer (Java Revisited, 2012). Two or more threads can share physical memory as directed by Java. If the result of a thread is recorded before another thread, out of sequence, an attacker can manipulate the system in a way not anticipated by programmers (IBM Developer Works, 2004). One method is for a malicious user to claim to be a trusted user, and the system can be tricked into authentication. As a result, information submitted to logs could be accepted without being validated (OWASP, 2009). Trust exploits follow access-control vulnerabilities. Code-signing errors occur when there is no valid certificate that matches a user profile (Web Builders, 2013). Developer Richard Dallaway experienced many problems that were only resolved by obtaining digital certificates: “I needed to hand over money to a certificate authority so they could run some background checks on me to prove that I am who I say I am” (Dallaway, 2013). Essentially, it is possible to get a certificate from authorities that are less stringent, leaving Oracle Java 7 wide open for exploitation on Windows, OS X, and Linux platforms. CERT found that “a remote attacker may be able to execute arbitrary code on a vulnerable system” (CERT/Software Engineering Institute/Carnegie Mellon, 2013). Java patches have been frequently introduced to fix bugs after exploits expose vulnerabilities. H.D. Moore, CSO of Rapid7, indicated that Update 11 for Java 7 was not sufficient, and users should not keep Java on their desktop, nor rely on it to surf the web. He suggests that it will probably take two years to correct these vulnerabilities (Smith, 2013). Despite these challenges, Java is used on the Android platform, so it is likely to have staying power (Taft, 2012). Number Two: C Java and C are the only two programming languages in which more than 10 percent of code is used to write new software. In February 2013, TIOBE found that the usage of C grew by only 0.56 percent from the year before, and 17.080 percent of code is written in C (TIOBE Software, 2013). Vulnerabilities with the C programming language have been known for some time, so they are less shocking than recent warnings about Java. C is very influential, so you will see references back to C in other languages, such as Perl (number nine) and Ruby (number ten). The relationship with C++, C#, and Objective-C are more obvious. C’s vulnerabilities have rippled throughout software programming for decades. C traces back to Bell Labs in the early 1970s before multithreading was feasible on hardware. It’s a procedural language that has evolved over the decades as hardware has become more sophisticated (University of Michigan, 2013). This evolution has triggered race conditions, which has caused significant functionality problems and enabled some exploits. Java is object-oriented, while C is function-oriented. C’s basic programming unit is a function (Princeton University, 2013). C is vulnerable to code injection attacks. It allocates memory with local variables in a function (automatic), global variables (static) and malloc (dynamic). Some programmers do not know that they are responsible for allocating and de- allocating memory, including bounds and type checks. Compilers – which transform code into an executable program – do not detect common errors in C at run-time. Errors include pointers to de-allocated memory, reserving memory indefinitely after it is needed, and writing past bounds Security vulnerabilities of the top, page 3 Journal of Technology Research of allocated memory (Younan, 2013). C is much more vulnerable to buffer overflows than Java is. According to James Joyce, “C includes no way of telling when the end of an array or allocated block of memory is overrun. The only way of telling is to run, test, and wait for a segfault. A spectacular crash is also a telltale sign. Or a slow, steady leakage of memory from a program is a symptom of a buffer overflow in C” (2012).
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages16 Page
-
File Size-