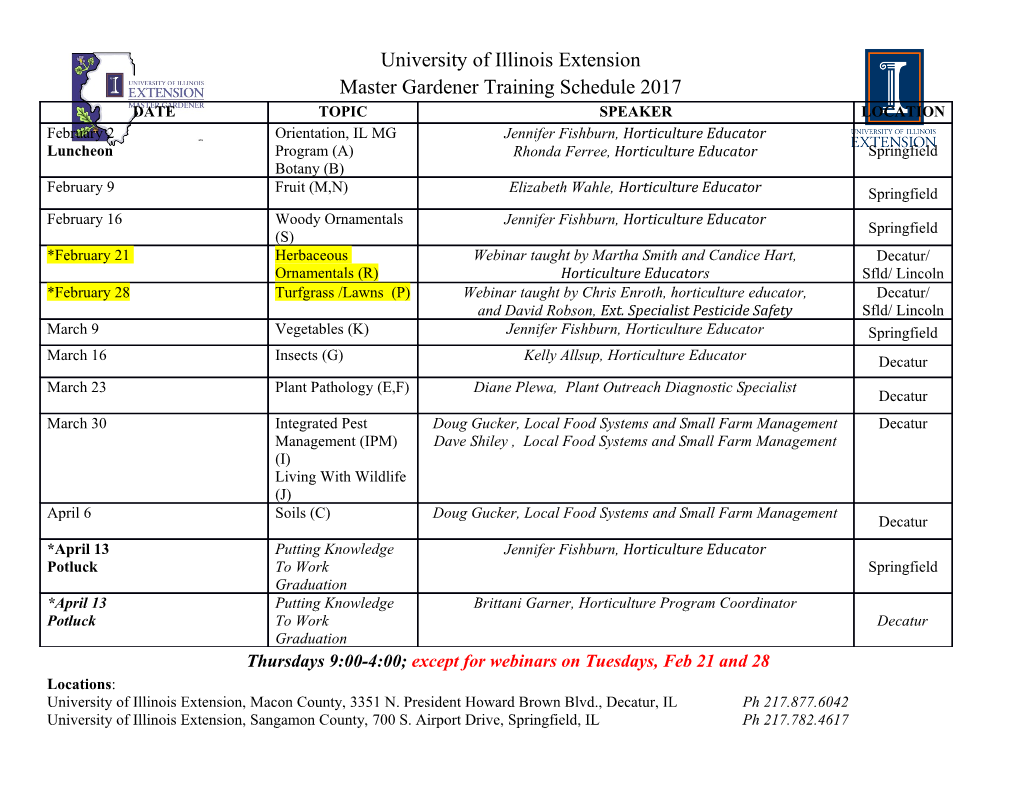
Daml SDK Documentation Digital Asset Version : 1.16.0 Copyright (c) 2021 Digital Asset (Switzerland) GmbH and/or its affiliates. All rights reserved. Any unauthorized use, duplication or distribution is strictly prohibited. Table of contents Table of contents i 1 Getting started 1 1.1 Installing the SDK ............................................. 1 1.1.1 1. Install the dependencies .................................. 1 1.1.2 2. Install the SDK ......................................... 1 1.1.3 Installing the Enterprise Edition ............................... 1 1.1.4 Next steps ............................................. 2 1.1.5 Alternative: manual download ................................ 2 1.2 Getting Started with Daml ........................................ 6 1.2.1 Prerequisites ........................................... 6 1.2.2 Running the app ......................................... 6 1.3 App Architecture .............................................. 10 1.3.1 The Daml Model .......................................... 11 1.3.2 TypeScript Code Generation .................................. 12 1.3.3 The UI ................................................ 12 1.4 Your First Feature .............................................. 13 1.4.1 Daml Changes .......................................... 13 1.4.2 Running the New Feature ................................... 14 1.4.3 Messaging UI ........................................... 14 1.4.4 Running the updated UI .................................... 15 1.4.5 Next Steps ............................................. 17 1.5 Testing Your App .............................................. 17 1.5.1 Setting up our tests ....................................... 18 1.5.2 Example: Logging in and out ................................. 18 1.5.3 Accessing UI elements ..................................... 19 1.5.4 Writing CSS Selectors ...................................... 19 1.5.5 The Full Test Suite ........................................ 20 2 Writing Daml 21 2.1 An introduction to Daml ......................................... 21 2.1.1 1 Basic contracts ......................................... 21 2.1.2 2 Testing templates using Daml Script .......................... 23 2.1.3 3 Data types ............................................ 29 2.1.4 4 Transforming data using choices ............................. 44 2.1.5 5 Adding constraints to a contract ............................. 51 2.1.6 6 Parties and authority ..................................... 60 2.1.7 7 Composing choices ...................................... 69 2.1.8 8 Exception Handling ...................................... 77 2.1.9 9 Working with Dependencies ................................ 81 i 2.1.10 10 Functional Programming 101 ................................ 84 2.1.11 11 Intro to the Daml Standard Library ............................ 95 2.1.12 12 Testing Daml Contracts ................................... 101 2.2 Language reference docs ......................................... 105 2.2.1 Overview: template structure ................................. 105 2.2.2 Reference: templates ...................................... 108 2.2.3 Reference: choices ........................................ 111 2.2.4 Reference: updates ....................................... 115 2.2.5 Reference: data types ...................................... 118 2.2.6 Reference: built-in functions ................................. 124 2.2.7 Reference: expressions ..................................... 126 2.2.8 Reference: functions ...................................... 128 2.2.9 Reference: scenarios ...................................... 131 2.2.10 Reference: Daml file structure ................................ 132 2.2.11 Reference: Daml packages .................................. 133 2.2.12 Contract keys ........................................... 139 2.2.13 Exceptions ............................................. 148 2.3 The standard library ............................................ 149 2.3.1 Module Prelude .......................................... 149 2.3.2 Module DA.Action ......................................... 179 2.3.3 Module DA.Action.State ..................................... 180 2.3.4 Module DA.Action.State.Class ................................. 181 2.3.5 Module DA.Assert ......................................... 182 2.3.6 Module DA.Bifunctor ....................................... 182 2.3.7 Module DA.BigNumeric ..................................... 184 2.3.8 Module DA.Date .......................................... 185 2.3.9 Module DA.Either ......................................... 187 2.3.10 Module DA.Exception ...................................... 188 2.3.11 Module DA.Foldable ....................................... 190 2.3.12 Module DA.Functor ........................................ 192 2.3.13 Module DA.List .......................................... 192 2.3.14 Module DA.List.BuiltinOrder .................................. 196 2.3.15 Module DA.List.Total ....................................... 197 2.3.16 Module DA.Logic ......................................... 198 2.3.17 Module DA.Map .......................................... 199 2.3.18 Module DA.Math ......................................... 201 2.3.19 Module DA.Monoid ........................................ 202 2.3.20 Module DA.Next.Map ....................................... 203 2.3.21 Module DA.Next.Set ....................................... 205 2.3.22 Module DA.NonEmpty ...................................... 207 2.3.23 Module DA.NonEmpty.Types .................................. 208 2.3.24 Module DA.Numeric ....................................... 208 2.3.25 Module DA.Optional ....................................... 209 2.3.26 Module DA.Optional.Total .................................... 210 2.3.27 Module DA.Record ........................................ 210 2.3.28 Module DA.Semigroup ..................................... 211 2.3.29 Module DA.Set ........................................... 212 2.3.30 Module DA.Stack ......................................... 213 2.3.31 Module DA.Text .......................................... 214 2.3.32 Module DA.TextMap ....................................... 217 2.3.33 Module DA.Time .......................................... 218 2.3.34 Module DA.Traversable ..................................... 219 2.3.35 Module DA.Tuple ......................................... 220 2.3.36 Module DA.Validation ...................................... 221 2.4 Troubleshooting ............................................... 222 2.4.1 Error: “<X> is not authorized to commit an update” .................. 222 2.4.2 Error “Argument is not of serializable type” ....................... 222 2.4.3 Modeling questions ....................................... 222 2.4.4 Testing questions ........................................ 224 2.5 Good design patterns ........................................... 225 2.5.1 Initiate and Accept ........................................ 225 2.5.2 Multiple party agreement ................................... 227 2.5.3 Delegation ............................................. 230 2.5.4 Authorization ........................................... 232 2.5.5 Locking ............................................... 235 2.5.6 Diagram legends ......................................... 243 3 Building applications 244 3.1 Application architecture ......................................... 244 3.1.1 Backend .............................................. 246 3.1.2 Frontend .............................................. 246 3.1.3 Authorization ........................................... 246 3.1.4 Developer workflow ....................................... 247 3.2 JavaScript Client Libraries ........................................ 250 3.2.1 JavaScript Code Generator ................................... 250 3.2.2 @daml/react ........................................... 255 3.2.3 @daml/ledger ........................................... 255 3.2.4 @daml/types ........................................... 255 3.3 HTTP JSON API Service ........................................... 255 3.3.1 Daml-LF JSON Encoding .................................... 255 3.3.2 Query language .......................................... 262 3.3.3 Metrics ............................................... 264 3.3.4 Running the JSON API ...................................... 266 3.3.5 HTTP Status Codes ........................................ 270 3.3.6 Create a new Contract ...................................... 272 3.3.7 Creating a Contract with a Command ID .......................... 274 3.3.8 Exercise by Contract ID ..................................... 274 3.3.9 Exercise by Contract Key .................................... 276 3.3.10 Create and Exercise in the Same Transaction ...................... 277 3.3.11 Fetch Contract by Contract ID ................................. 279 3.3.12 Fetch Contract by Key ...................................... 280 3.3.13 Get all Active Contracts ..................................... 281 3.3.14 Get all Active Contracts Matching a Given Query .................... 282 3.3.15 Fetch Parties by Identifiers .................................. 283 3.3.16 Fetch All Known Parties ..................................... 285 3.3.17 Allocate a New Party ....................................... 285 3.3.18 List All DALF Packages ..................................... 286 3.3.19 Download a DALF Package ................................... 286 3.3.20 Upload a DAR File ........................................
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages593 Page
-
File Size-