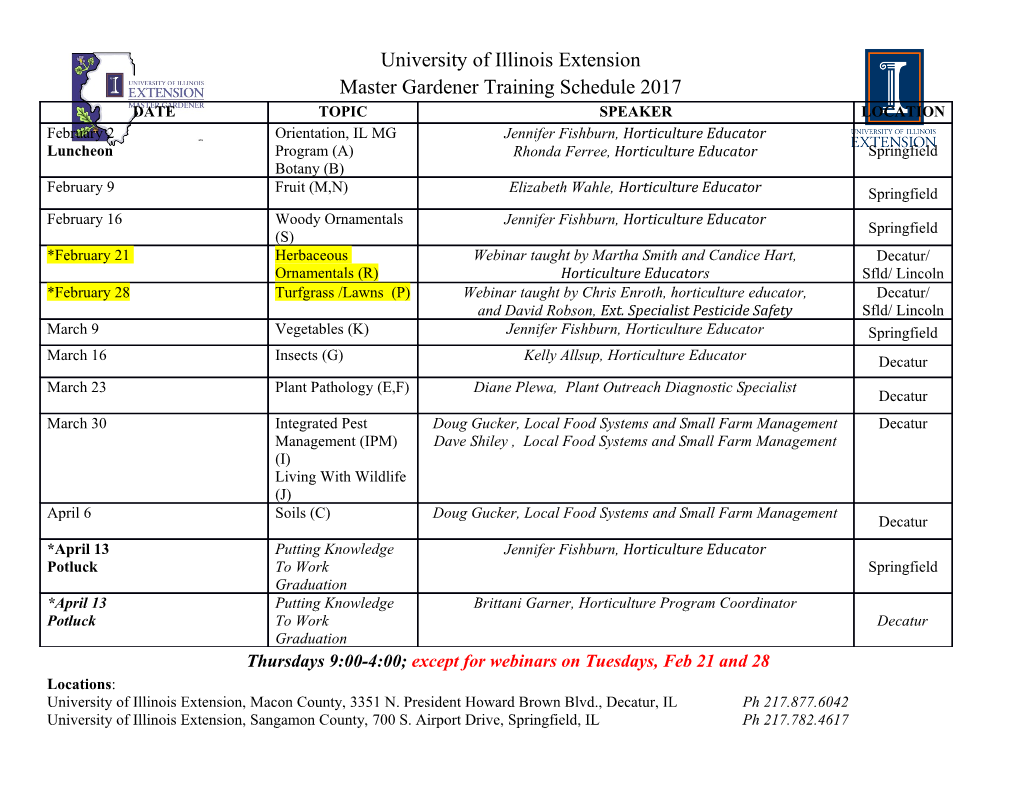
Single-Source Shortest Paths (Ch. 24) Single-Source Shortest Paths The single-source shortest path problem (SSSP) Weights in SSSP algorithms include distances, times, hops, input: a directed graph G = (V, E) with edge weights, and a cost, etc. Used in lots of routing applications. specific source node s. goal: find a minimum weight (shortest) path from s to every other Note: BFS finds the shortest paths for the special case when all node in V (sometimes we only want the cost of these paths) edge weights are 1. Running time = O(V + E) It turns out that, in the worst case, finding the shortest path between s and a single The result of a SSSP algorithm can be viewed as a tree node t is no easier than finding the shortest paths between s and all other nodes rooted at s, containing a shortest (wrt weight) path from s à g = ¥ s to all other nodes. s à b = 2 2 3 s à c = 5 s à h = 7 a b c 2 3 5 6 a b 6 c s à d = 6 S 5 3 d 1 e S 3 s à e = 5 2 d 1 e 2 s à f = 3 f 4 g 2 h f 4 g 2 h 2 Negative-weight cycles Single-Source Shortest Paths Some graphs may have negative-weight cycles and these are Question: Can a shortest path contain a cycle? a problem for SSSP algorithms. What is the shortest path from a to d? No. b 9 · path a-c-e-d = -12+3-2 = -11 2 8 6 Suppose we have a shortest path p = áv0, v1, ..., vkñ and S -12 3 c = áv , v , ..., v ñ is a positive-weight cycle on p so that v = v · path a-c-e-d-a-c-e-d = a c e i i+1 j i j -12+3-2+(10-12+3-2)= -13 6 4 and w(c) > 0. Then the path (obtained from splicing out c) 10 p' = áv , v , ..., v , v , v ,...v ñ If we keep going around the cycle d -2 0 1 i j+1 j+2 k (d-a-c-e-d), we keep shortening the has w(p') = w(p) – w(c) < w(p). So p can’t be a shortest path. weight of the path. So the shortest path has weight -¥ Therefore, we can assume, wlog, that shortest paths have no cycles. To avoid this problem, we require that the graph has no negative weight cycles, otherwise the solution does not exist. 3 4 Dijkstra’s SSSP Algorithm Dijkstra’s SSSP Algorithm Algorithm SSSP-Dijkstra (G, s) Algorithm SSSP-Dijkstra (G, s) 1. Q = Æ //** Q is a priority queue 1. Q = Æ //** Q is a priority queue Tree nodes are Trace execution 2. d[s] = 0 and insert s into Q 2. d[s] = 0 and insert s into Q of Dijkstra’s algorithm nodes extracted 3. for each v ¹ s 3. for each v ¹ s from Q (in S, on graph below. 4. d[v] = ¥ 4. d[v] = ¥ the set of 5. insert v into Q with key d[v] 5. insert v into Q with key d[v] shortest paths). 6. while Q ¹ Æ 6. while Q ¹ Æ 7. u = extract-min(Q) 7. u = extract-min(Q) 8. for each (outgoing) neighbor of u 8. for each outgoing neighbor of u 9. d[v] = min{d[v], d[u] + wt(u,v)} 9 9. d[v] = min{d[v], d[u] + wt(u,v)} 10. endfor b 11. endwhile 2 10. endfor Procedure relax (x, y) 8 6 d[y] = min{d[y], d[x] + wt(x, y)} S 12 3 11. endwhile a c e 4 Fringe nodes are nodes in Q with d[v] < ¥ 6 10 Unseen nodes are nodes in Q with d[v] = ¥ 5 d 2 1 Algorithm SSSP-Dijkstra (G, s) Algorithm SSSP-Dijkstra (G, s) iteration 0: 1. Q = Æ //** Q is a priority queue 1. Q = Æ //** Q is a priority queue Q a b c d e 2. d[s] = 0 and insert s into Q iteration 4: Q = Q – {e} 2. d[s] = 0 and insert s into Q 3. for each v ¹ s 3. for each v ¹ s d[v] 0 ¥ ¥ ¥ ¥ 4. d[v] = ¥ Q xa xb xc d xe 4. d[v] = ¥ iteration 1: Q = Q – {a} 5. insert v into Q with key d[v] d[v] 0 2 10 13 11 5. insert v into Q with key d[v] 6. while Q ¹ Æ 6. while Q ¹ Æ Q xa b c d e 7. u = extract-min(Q) 7. u = extract-min(Q) d[v] 0 2 12 ¥ ¥ 8. for each neighbor of u 8. for each neighbor of u 9. d[v] = min{d[v], d[u] + wt(u,v)} iteration 5: Q = Q – {d} 9. d[v] = min{d[v], d[u] + wt(u,v)} iteration 2: Q = Q – {b} 10. endfor 10. endfor 11. endwhile Q xa xb xc xd xe 11. endwhile Q xa xb c d e d[v] 0 2 10 13 11 d[v] 0 2 10 ¥ 11 b 9 b 9 2 2 8 6 8 6 iteration 3: Q = Q – {c} S 12 3 DONE S 12 3 a c e a c e Q xa xb xc d e 6 4 6 4 0 2 10 16 11 10 2 10 2 d[v] d d 8 Dijkstra’s SSSP Algorithm Dijkstra’s SSSP Algorithm iter u d[a] d[b] d[c] d[d] d[e] Algorithm SSSP-Dijkstra (G, s) Exercise: 0 - 0 ¥ ¥ ¥ ¥ 1. Q = Æ //** Q is a priority queue Example: 2. d[s] = 0 and insert s into Q 1 a x 2 12 ¥ ¥ 3. for each v ¹ s 1. Give a simple example of a directed graph with 2 b x x 10 ¥ 11 4. d[v] = ¥ 5. insert v into Q with key d[v] negative weight edges 3 c x x x 16 11 6. while Q ¹ Æ (but no neg wt cycles) 7. u = extract-min(Q) for which Dijkstra’s alg 4 e x x x 13 x 8. for each neighbor of u produces incorrect 5 d x x x x x 9. d[v] = min{d[v], d[u] + wt(u,v)} answers. 10. endfor b 9 11. endwhile 2 8 6 S 12 3 2. Suppose we change line 6 to “while |Q| > 1” a c e This change causes the while loop to execute |V| - 1 times 6 4 instead of |V| times. Is this proposed algorithm correct? Why or 10 d 2 why not? 10 Running Time of Dijkstra’s SSSP Alg Running Time of Dijkstra’s SSSP Alg Algorithm SSSP-Dijkstra (G, s) Assume adjacency list 1. Q = Æ //** Q is a priority queue representation. The time If G is dense (i.e., q(V2) edges): 2. d[s] = 0 and insert s into Q will depend on what? 3. for each v ¹ s no point in being clever about extract-min. Store each 4. d[v] = ¥ Implementation of the d[v] in the vth entry of an array. Each insert and decrease- 5. insert v into Q with key d[v] priority queue. key takes O(1) time. Extract-min takes O(V) time (why?) 6. while Q ¹ Æ Total time: O(V2 + E) = O(V2) 7. u = extract-min(Q) In general, 8. for each neighbor of u 2 Steps 1-5: O(V) time If G is sparse (i.e., o(V ) edges: 9. d[v] = min{d[v], d[u] + wt(u,v)} Steps 6-11: try to minimize extract-min, use binary heap (heapsort) 10. endfor time for extract-min & decrease-key = O(lgV) 11. endwhile V iterations Suppose extract-min Total time: O(VlgV + ElgV) = O((V + E) lgV) Steps 8-10: E iterations overall takes O(X ) time (looks at each edge once) Q Suppose decrease-key takes O(YQ) time. Total: O(VXQ + EYQ) 11 12 2 Correctness of Dijkstra’s SSSP Alg Correctness of Dijkstra’s SSSP Alg Let S = V – Q be the nodes that have been removed from Q. Lemma: For all nodes x Î V: Lemma: For all nodes x Î V: (a) if x Î S (x Ï Q), then the shortest s to x path only uses nodes in S and (a) if x Î S (x Ï Q), then the shortest s to x path only uses nodes in S and d[x] is its weight. d[x] is its weight. (b) if x Ï S (x Î Q), then d[x] is weight of the shortest s to x path, all of (b) if x Ï S (x Î Q), then d[x] is weight of the shortest s to x path, all of whose intermediate nodes are in S. whose intermediate nodes are in S. Induction Step: Show Lemma is true for iteration i. Proof: By induction on i, the number of iterations of the while loop. Let u be the node selected in the ith iteration (the node in Q with minimum d[u] value). Basis: i = 1, S = {s}, and d[x] = ¥ if x is not a neighbor of s, and Proof of (a): (Assume x is in S) otherwise d[x] = wt(s,x). So both (a) and (b) hold. case 1: x ¹ u. Then x was in S before iteration i, and by the IHOP, we already had the best s to x path.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages5 Page
-
File Size-