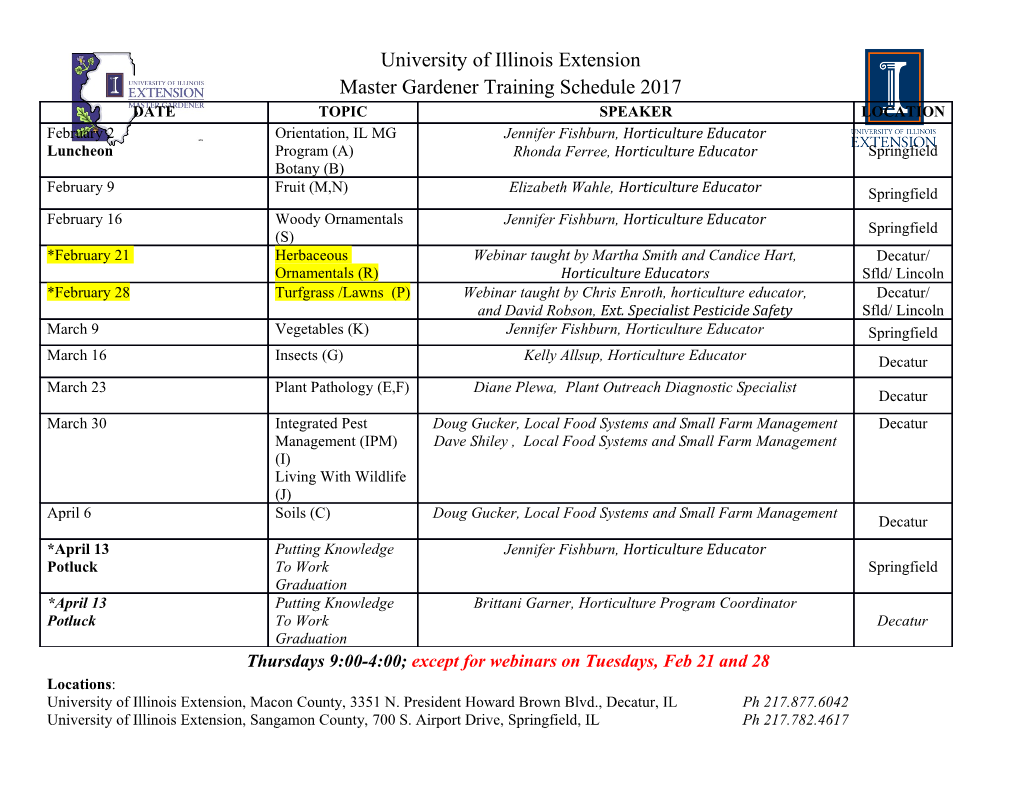
OpenGL ES 2.0 API Quick Reference Card OpenGL® ES is a software interface to graphics hardware. The interface Errors [2.5] consists of a set of procedures and functions that allow a programmer to specify enum GetError( void ); //Returns one of the following: the objects and operations involved in producing high-quality graphical images, INVALID_ENUM Enum argument out of range specifically color images of three-dimensional objects. INVALID_FRAMEBUFFER_OPERATION Framebuffer is incomplete • [n.n.n] refers to sections and tables in the OpenGL ES 2.0 specification. INVALID_VALUE Numeric argument out of range • [n.n.n] refers to sections in the OpenGL ES Shading Language 1.0 INVALID_OPERATION Operation illegal in current state specification. OUT_OF_MEMORY Not enough memory left to execute command Specifications are available at www.opengl.org/registry/gles NO_ERROR No error encountered OpenGL ES Command Syntax [2.3] GL Data Types [2.3] Open GL ES commands are formed from a return type, a name, and optionally a type letter i for 32-bit int, or f for 32-bit float, GL types are not C types. as shown by the prototype below: Minimum return-type Name{1234}{if}{v} ([args ,] T arg1 , . , T argN [, args]); GL Type Bit Width Description boolean 1 Boolean The arguments enclosed in brackets ([args ,] and [, args]) may or may not be present. byte 8 Signed binary integer The argument type T and the number N of arguments may be indicated by the command name suffixes. N is 1, 2, 3, or 4 if present, or else corresponds to the type letters. If “v” is present, an array of N items is passed by a pointer. ubyte 8 Unsigned binary integer char 8 Characters making up strings For brevity, the OpenGL documentation and this reference may omit the standard prefixes. The actual names are of the forms: glFunctionName(), GL_CONSTANT, GLtype short 16 Signed 2’s complement binary integer ushort 16 Unsigned binary integer int 32 Signed 2’s complement binary integer Updating Buffer Object Data Stores Buffer Objects [2.9] uint 32 Unsigned binary integer Buffer objects hold vertex array data or indices in void BufferSubData(enum target, intptr offset, high-performance server memory. sizeiptr size, const void *data); fixed 32 Signed 2’s complement 16.16 scaled integer target: ARRAY_BUFFER, ELEMENT_ARRAY_BUFFER void GenBuffers(sizei n, uint *buffers); sizei 32 Non-negative binary integer size void DeleteBuffers(sizei n, const uint *buffers); Buffer Object Queries [6.1.6, 6.1.3] enum 32 Enumerated binary integer value boolean IsBuffer(uint buffer); Creating and Binding Buffer Objects void GetBufferParameteriv(enum target, enum value, intptr ptrbits Signed 2’s complement binary integer void BindBuffer(enum target, uint buffer); T data); sizeiptr ptrbits Non-negative binary integer size target: ARRAY_BUFFER, ELEMENT_ARRAY_BUFFER target: ARRAY_BUFFER, ELEMENT_ ARRAY_BUFFER bitfield 32 Bit field Creating Buffer Object Data Stores value: BUFFER_SIZE, BUFFER_USAGE void BufferData(enum target, sizeiptr size, float 32 Floating-point value const void *data, enum usage); clampf 32 Floating-point value clamped to [0; 1] usage: STATIC_DRAW, STREAM_DRAW, DYNAMIC_DRAW Vertices Viewport and Clipping [4.3.1] Reading Pixels Current Vertex State [2.7] Controlling the Viewport [2.12.1] void ReadPixels(int x, int y, sizei width, sizei height, void DepthRangef(clampf n, clampf f); enum format, enum type, void *data); void VertexAttrib{1234}{f}(uint index, T values); void Viewport(int x, int y, sizei w, sizei h); format: RGBA type: UNSIGNED_BYTE void VertexAttrib{1234}{f}v(uint index, T values); Note: ReadPixels() also accepts a queriable implementation- definedformat/type combination, see [4.3.1]. Vertex Arrays [2.8] Vertex data may be sourced from arrays that are stored in application memory (via a pointer) or faster GPU memory (in a buffer object). Texturing [3.7] void TexSubImage2D(enum target, int level, int xoffset, int yoffset, sizei width, sizei height, enum format, void VertexAttribPointer(uint index, int size, enum type, Shaders support texturing using at least enum type, void *data); boolean normalized, sizei stride, const void *pointer); MAX_VERTEX_TEXTURE_IMAGE_UNITS images for vertex target: TEXTURE_CUBE_MAP_POSITIVE_{X, Y, Z}, type: BYTE, UNSIGNED_BYTE, SHORT, UNSIGNED_SHORT, FIXED, FLOAT shaders and at least MAX_TEXTURE_IMAGE_UNITS images TEXTURE_CUBE_MAP_NEGATIVE_{X, Y, Z} index: [0, MAX_VERTEX_ATTRIBS - 1] for fragment shaders. format and type: See TexImage2D If an ARRAY_BUFFER is bound, the attribute will be read from the void ActiveTexture(enum texture); void CopyTexSubImage2D(enum target, int level, int xoffset, bound buffer, and pointer is treated as an offset within the buffer. texture: [TEXTURE0..TEXTUREi] where i = int yoffset, int x, int y, sizei width, sizei height); void EnableVertexAttribArray(uint index); MAX_COMBINED_TEXTURE_IMAGE_UNITS-1 target: TEXTURE_2D, TEXTURE_CUBE_MAP_POSITIVE_{X, Y, Z}, void DisableVertexAttribArray(uint index); TEXTURE_CUBE_MAP_NEGATIVE_{X, Y, Z} index: [0, MAX_VERTEX_ATTRIBS - 1] Texture Image Specification [3.7.1] format and type: See TexImage2D void DrawArrays(enum mode, int first, sizei count); void TexImage2D(enum target, int level, int internalformat, Compressed Texture Images [3.7.3] sizei width, sizei height, int border, enum format, void DrawElements(enum mode, sizei count, enum type, enum type, void *data); void CompressedTexImage2D(enum target, int level, void *indices); target: TEXTURE_2D, TEXTURE_CUBE_MAP_POSITIVE_{X,Y,Z} enum internalformat, sizei width, sizei height, mode: POINTS, LINE_STRIP, LINE_LOOP, LINES, TRIANGLE_STRIP, TEXTURE_CUBE_MAP_NEGATIVE_{X,Y,Z} int border, sizei imageSize, void *data); TRIANGLE_FAN, TRIANGLES internalformat: ALPHA, LUMINANCE, LUMINANCE_ALPHA, RGB, target and internalformat: See TexImage2D type: UNSIGNED_BYTE, UNSIGNED_SHORT RGBA If an ELEMENT_ARRAY_BUFFER is bound, the indices will be read format: ALPHA, RGB, RGBA, LUMINANCE, LUMINANCE_ALPHA void CompressedTexSubImage2D(enum target, int level, int xoffset, int yoffset, sizei width, sizei height, from the bound buffer, and indices is treated as an offset within type: UNSIGNED_BYTE, UNSIGNED_SHORT_5_6_5, the buffer. UNSIGNED_SHORT_4_4_4_4, UNSIGNED_SHORT_5_5_5_1 enum format, sizei imageSize, void *data); target and internalformat: See TexImage2D Conversion from RGBA pixel components to internal texture components: Texture Parameters [3.7.4] void TexParameter{if}(enum target, enum pname, Base Internal Format RGBA Internal Components T param); Rasterization [3] ALPHA A A void TexParameter{if}v(enum target, enum pname, Points [3.3] T params); Point size is taken from the shader builtin gl_PointSize and LUMINANCE R L target: TEXTURE_2D, TEXTURE_CUBE_MAP clamped to the implementation-dependent point size range. pname: TEXTURE_WRAP_{S, T}, TEXTURE_{MIN, MAG}_FILTER LUMINANCE _ALPHA R, A L, A Line Segments [3.4] Manual Mipmap Generation [3.7.11] void LineWidth(float width); RGB R, G, B R, G, B void GenerateMipmap(enum target); target: TEXTURE_2D, TEXTURE_CUBE_MAP Polygons [3.5] RGBA R, G, B, A R, G, B, A Texture Objects [3.7.13] void FrontFace(enum dir); void BindTexture(enum target, uint texture); dir: CCW, CW Alt. Texture Image Specification Commands[3.7.2] void DeleteTextures(sizei n, uint *textures); void CullFace(enum mode); Texture images may also be specified using image data taken mode: FRONT, BACK, FRONT_AND_BACK directly from the framebuffer, and rectangular subregions of void GenTextures(sizei n, uint *textures); Enable/Disable(CULL_FACE) existing texture images may be respecified. Enumerated Queries [6.1.3] void PolygonOffset(float factor, float units); void CopyTexImage2D(enum target, int level, void GetTexParameter{if}v(enum target, enum value, Enable/Disable(POLYGON_OFFSET_FILL) enum internalformat, int x, int y, sizei width, T data); sizei height, int border); target: TEXTURE_2D, TEXTURE_CUBE_MAP target: TEXTURE_2D, TEXTURE_CUBE_MAP_POSITIVE_{X, Y, Z}, value: TEXTURE_WRAP_{S, T}, TEXTURE_{MIN, MAG}_FILTER TEXTURE_CUBE_MAP_NEGATIVE_{X, Y, Z} Texture Queries [6.1.4] Pixel Rectangles [3.6, 4.3] internalformat: See TexImage2D void PixelStorei(enum pname, int param); boolean IsTexture(uint texture); pname: UNPACK_ALIGNMENT, PACK_ALIGNMENT ©2010 Khronos Group - Rev. 0210 www.khronos.org/opengles OpenGL ES 2.0 API Quick Reference Card Shaders and Programs void BindAttribLocation(uint program, uint index, sizei *length, char *source); const char *name); Shader Objects [2.10.1] void GetShaderPrecisionFormat(enum shadertype, uint CreateShader(enum type); Uniform Variables enum precisiontype, int *range, int *precision); type: VERTEX_SHADER, FRAGMENT_SHADER int GetUniformLocation(uint program, const char *name); shadertype: VERTEX_SHADER, FRAGMENT_SHADER precision: LOW_FLOAT, MEDIUM_FLOAT, HIGH_FLOAT, LOW_INT, void ShaderSource(uint shader, sizei count, void GetActiveUniform(uint program, uint index, MEDIUM_INT, HIGH_INT const char **string, const int *length); sizei bufSize, sizei *length, int *size, enum *type, void GetVertexAttribfv(uint index, enum pname, void CompileShader(uint shader); char *name); *type: FLOAT, FLOAT_VEC{2,3,4}, INT, INT_VEC{2,3,4}, BOOL, float *params); void ReleaseShaderCompiler(void); BOOL_VEC{2,3,4}, FLOAT_MAT{2,3,4}, SAMPLER_2D, pname: CURRENT_VERTEX_ATTRIB , VERTEX_ATTRIB_ARRAY_x SAMPLER_CUBE (where x may be BUFFER_BINDING, ENABLED, SIZE, STRIDE, TYPE, void DeleteShader(uint shader); NORMALIZED) void Uniform{1234}{if}(int location, T value); Loading Shader Binaries [2.10.2] void GetVertexAttribiv(uint index,
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages4 Page
-
File Size-