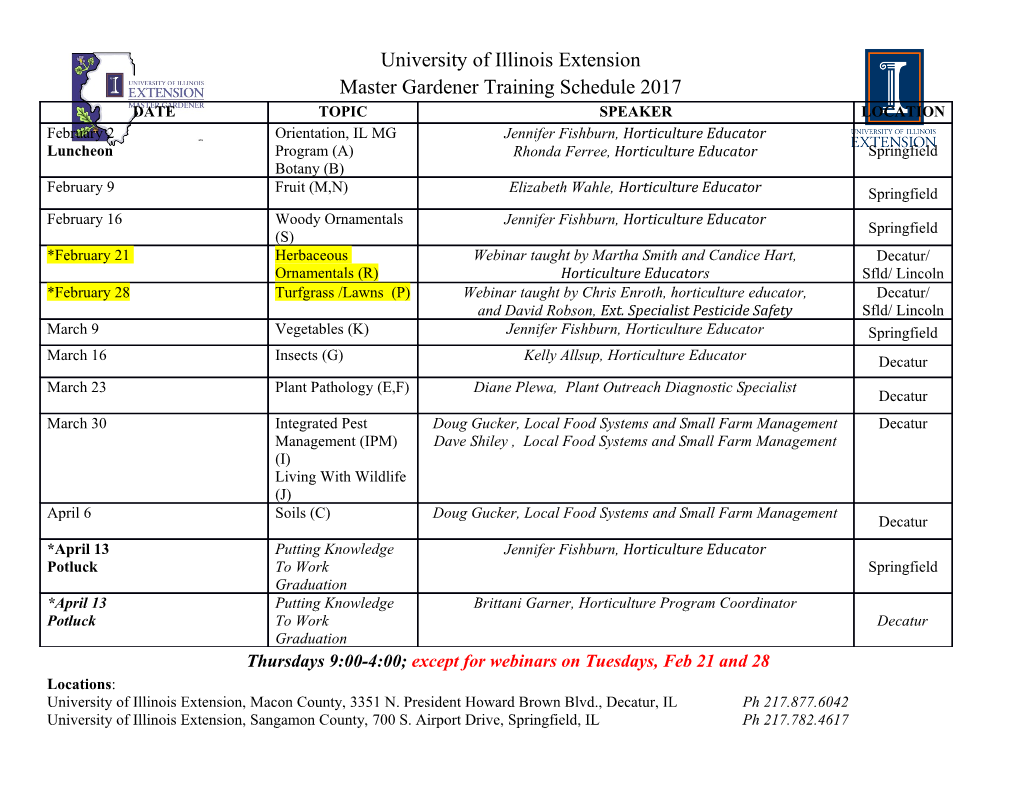
Implementing Cryptography Using Python® Shannon W. Bray Published by John Wiley & Sons, Inc. 10475 Crosspoint Boulevard Indianapolis, IN 46256 www.wiley.com Copyright © 2020 by John Wiley & Sons, Inc., Indianapolis, Indiana Published simultaneously in Canada ISBN: 978-1-119-61220-9 ISBN: 978-1-119-61222-3 (ebk) ISBN: 978-1-119-61545-3 (ebk) Manufactured in the United States of America No part of this publication may be reproduced, stored in a retrieval system or transmitted in any form or by any means, electronic, mechanical, photocopying, recording, scanning or otherwise, except as permitted under Sections 107 or 108 of the 1976 United States Copyright Act, without either the prior written permission of the Publisher, or authorization through payment of the appropriate per-copy fee to the Copyright Clear- ance Center, 222 Rosewood Drive, Danvers, MA 01923, (978) 750-8400, fax (978) 646-8600. Requests to the Publisher for permission should be addressed to the Permissions Department, John Wiley & Sons, Inc., 111 River Street, Hoboken, NJ 07030, (201) 748-6011, fax (201) 748-6008, or online at http://www.wiley.com/ go/permissions. Limit of Liability/Disclaimer of Warranty: The publisher and the author make no representations or war- ranties with respect to the accuracy or completeness of the contents of this work and specifically disclaim all warranties, including without limitation warranties of fitness for a particular purpose. No warranty may be created or extended by sales or promotional materials. The advice and strategies contained herein may not be suitable for every situation. This work is sold with the understanding that the publisher is not engaged in rendering legal, accounting, or other professional services. If professional assistance is required, the services of a competent professional person should be sought. Neither the publisher nor the author shall be liable for damages arising herefrom. The fact that an organization or Web site is referred to in this work as a citation and/or a potential source of further information does not mean that the author or the publisher endorses the information the organization or website may provide or recommendations it may make. Further, readers should be aware that Internet websites listed in this work may have changed or disappeared between when this work was written and when it is read. For general information on our other products and services please contact our Customer Care Department within the United States at (877) 762-2974, outside the United States at (317) 572-3993 or fax (317) 572-4002. Wiley publishes in a variety of print and electronic formats and by print-on-demand. Some material included with standard print versions of this book may not be included in e-books or in print-on-demand. If this book refers to media such as a CD or DVD that is not included in the version you purchased, you may down- load this material at http://booksupport.wiley.com. For more information about Wiley products, visit www.wiley.com. Library of Congress Control Number: 2020940306 Trademarks: Wiley and the Wiley logo are trademarks or registered trademarks of John Wiley & Sons, Inc. and/or its affiliates, in the United States and other countries, and may not be used without written permis- sion. Python is a registered trademark of Python Software Foundation. All other trademarks are the property of their respective owners. John Wiley & Sons, Inc. is not associated with any product or vendor mentioned in this book. To Stephanie, Eden, Hayden, and Kenna, with all my love, for making each and every day special. About the Author Shannon W. Bray started his career in information technology in 1997 after being honorably discharged from the United States Navy. He started his IT career working on programmable logic controllers out in the Gulf of Mexico and then started writing business applications using various technologies until 2000 when .NET was first released; since then, the languages of choice have been C++, C#, Windows PowerShell, and Python. From there, Shannon worked on writing software until he started working with Microsoft SharePoint. The Microsoft stack of technologies forced Shannon out of just writing code into building large secure solutions. Shannon’s career has taken him to engagements all over the world where he has worked with a number of U.S. government agencies. Shannon became interested in cryptography while pursuing a master’s degree in cybersecurity, and he has continued to research cryptography and cybersecurity as he pursues a PhD in computer science. Shannon has earned the following certifications: Microsoft Certified Master, Microsoft Certified Solutions Master, Certified Information Security Manager (CISM), Security+ (Plus), the CompTIA Advanced Security Practitioner (CASP+), and a number of other industry certifications. In addition to writing, Shannon speaks nationally at technology conventions and works as a mentor teaching technology to youth programs. Shannon lives in the Raleigh, North Carolina, area with his wife, Stephanie, two daughters, Eden and Kenna, and son, Hayden. During the dive season, Shannon enjoys diving off the coast of North Carolina. During the rest of the year, Shannon works on IT projects around the house that utilize single-board computers and, most often, propellers. He is currently building a home security system that uses Python, cryptography, and drones. As of the release of this v vi About the Author book, Shannon is currently running for the U.S. Senate for the state of North Carolina to help bring cybersecurity issues to the mainstream and to help people understand the importance of end-to-end encryption. Shannon can be contacted through LinkedIn at w w w.linkedin.com/in/ shannonbray. Acknowledgments While this is my third book, every book comes with its own set of challenges. Completing a book is not always a fun project but does become a labor of love. As soon as you start, life has a way of throwing changes at you, and it seems that you never have the time that you thought you would have. Shortly after starting this book, I started a PhD program with the Missouri University of Science and Technology and transitioned through a number of government contracts, ran for political office, and then became a work-from-home teacher as the world battled a global pandemic. I knew that the editing team would be earning their pay with whatever I put together. That team has been wonderful with their feedback and responsiveness. Specifically, I’d like to thank the follow- ing people on the Wiley team: Barath Kumar Rajasekaran, production editor; Jim Minatel, acquisitions editor; Pete Gaughan, content enablement manager; Brent Cook and James Langbridge, technical editors, and, most importantly, Kim Wimpsett, the project editor. Kim did her best to keep me on schedule, but I found new ways to miss a few deadlines. vii Contents at a Glance Introduction xvii Chapter 1 Introduction to Cryptography and Python 1 Chapter 2 Cryptographic Protocols and Perfect Secrecy 31 Chapter 3 Classical Cryptography 65 Chapter 4 Cryptographic Math and Frequency Analysis 95 Chapter 5 Stream Ciphers and Block Ciphers 139 Chapter 6 Using Cryptography with Images 171 Chapter 7 Message Integrity 199 Chapter 8 Cryptographic Applications and PKI 223 Chapter 9 Mastering Cryptography Using Python 247 Index 277 ix Contents Introduction xvii Chapter 1 Introduction to Cryptography and Python 1 Exploring Algorithms 2 Why Use Python? 2 Downloading and Installing Python 3 Installing on Ubuntu 4 Installing on macOS 4 Installing on Windows 4 Installing on a Chromebook 4 Installing Additional Packages 5 Installing Pip, NumPy, and Matplotlib 6 Installing the Cryptography Package 7 Installing Additional Packages 8 Testing Your Install 9 Diving into Python Basics 9 Using Variables 10 Using Strings 11 Introducing Operators 11 Understanding Arithmetic Operators 11 Understanding Comparison Operators 13 Understanding Logical Operators 13 Understanding Assignment Operators 14 Understanding Bitwise Operators 15 Understanding Membership Operators 15 Understanding Identity Operators 16 xi xii Contents Using Conditionals 16 Using Loops 17 for 17 while 18 continue 18 break 18 else 18 Using Files 19 Understanding Python Semantics 20 Sequence Types 20 Introducing Custom Functions 26 Downloading Files Using Python 27 Introducing Python Modules 28 Creating a Reverse Cipher 29 Summary 30 Chapter 2 Cryptographic Protocols and Perfect Secrecy 31 The Study of Cryptology 32 Understanding Cryptography 32 Cryptography’s Famous Family: Alice and Bob 33 Diffie-Hellman 34 Data Origin Authentication 34 Entity Authentication 35 Symmetric Algorithms 36 Asymmetric Algorithms 36 The Needham-Schroeder Protocols 36 The Otway-Rees Protocol 38 Kerberos 39 Multiple-Domain Kerberos 40 X.509 41 Formal Validation of Cryptographic Protocols 46 Configuring Your First Cryptographic Library 47 Understanding Cryptanalysis 47 Brute-Force Attacks 47 Side-Channel Attacks 48 Social Engineering 48 Analytical Attacks 48 Frequency Analysis 48 Attack Models 49 Shannon’s Theorem 50 One-Time Pad 51 XOR, AND, and OR 51 One-Time Pad Function 56 One-Way Hashes 58 Contents xiii Cryptographic One-Way Hashes 59 Message Authentication Codes 60 Perfect Forward Secrecy 60 Published and Proprietary Encryption Algorithms 61 Summary 62 References 62 Chapter 3 Classical Cryptography 65 Password Best Practices 66 Password Storage 66 Hashing Passwords 67 Salting Passwords 67 Stretching Passwords 68 Password Tools 68 Obfuscating Data 69 ASCII Encoding
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages297 Page
-
File Size-