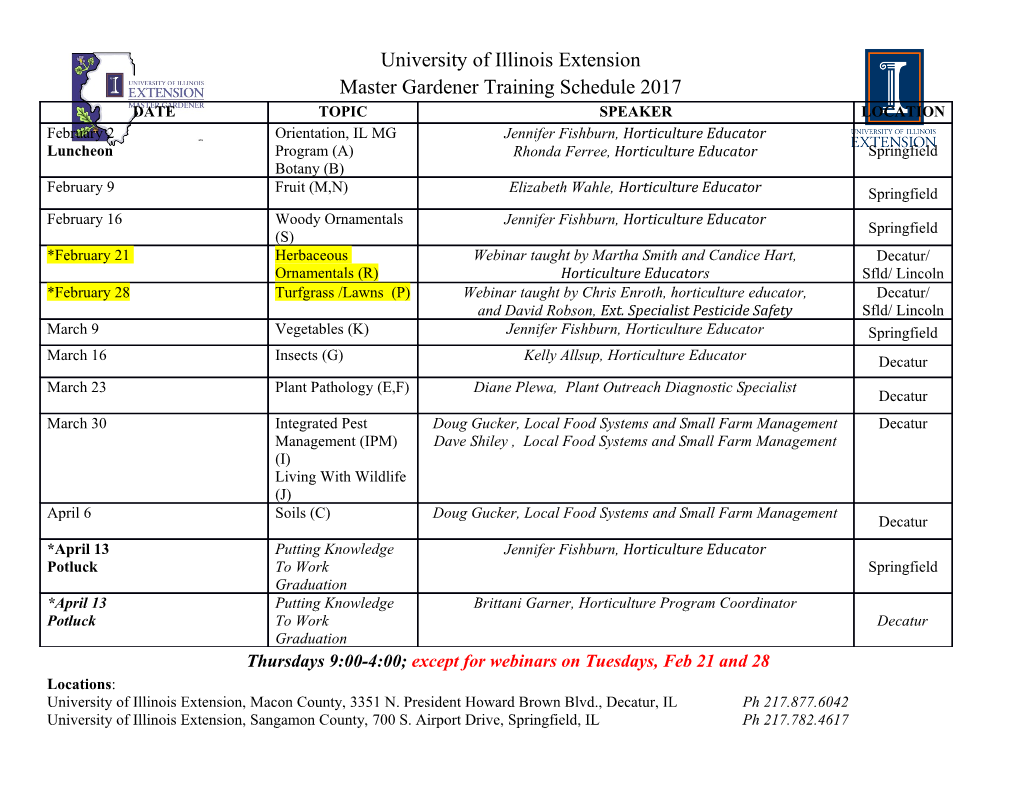
A Smart Book Web Development in Pascal with Smart Mobile Studio Primož Gabrijelčič ©2012 - 2013 Primož Gabrijelčič Tweet This Book! Please help Primož Gabrijelčič by spreading the word about this book on Twitter! The suggested hashtag for this book is #asmartbook. Find out what other people are saying about the book by clicking on this link to search for this hashtag on Twitter: https://twitter.com/search/#asmartbook Also By Primož Gabrijelčič Parallel Programming with OmniThreadLibrary Параллельное программирование с OmniThreadLibrary Programación Paralela con OmniThreadLibrary Contents Credits ............................................... i Introduction ............................................ ii Sample Code ........................................... iii Formatting Conventions .................................... iv Work in Progress ........................................ v Advertisement .......................................... vi Release Notes ........................................... vii 1 Prerequisites .......................................... 1 2 Getting Started ......................................... 2 2.1 Brief Introduction to Smart Mobile Studio ........................ 3 2.1.1 Project Types .................................... 3 2.1.2 Visual Project .................................... 3 2.1.3 Game Project .................................... 3 2.1.4 Console Project ................................... 3 2.2 Supported Browsers .................................... 4 3 Smart IDE ............................................ 5 3.1 Working with Designer .................................. 6 4 Smart Pascal .......................................... 7 4.1 Data Types ......................................... 8 4.1.1 Base Types ..................................... 8 4.1.1.1 Integer .................................. 8 4.1.1.2 Float ................................... 8 4.1.1.3 Boolean .................................. 8 4.1.1.4 String ................................... 8 4.1.1.5 Variant .................................. 9 4.1.1.6 Type Mappings .............................. 10 4.1.2 Enumerations .................................... 10 4.1.3 Sets ......................................... 11 4.1.4 Arrays ........................................ 11 CONTENTS 4.1.4.1 Static Arrays ............................... 11 4.1.4.2 Dynamic Arrays ............................. 12 4.1.5 Records ....................................... 14 4.1.5.1 Anonymous Records [¹.⁰.¹.¹²⁰] ...................... 14 4.1.5.2 Default Field Values [¹.⁰.¹.¹²⁰] ....................... 14 4.1.6 Classes ....................................... 15 4.1.6.1 Default Field Values [¹.⁰.¹.¹²⁰] ....................... 16 4.1.6.2 Partial Classes ............................... 16 4.1.6.3 External Classes .............................. 16 4.1.7 Interfaces ...................................... 17 4.1.8 Helpers ....................................... 18 4.1.9 Delegates ...................................... 19 4.1.10 Closures ....................................... 19 4.1.10.1 Lambdas [¹.¹.⁰.⁹¹¹] ............................. 20 4.1.10.1.1 Lambda Functions ........................ 20 4.1.10.1.2 Lambda Statements ....................... 21 4.2 Statements ......................................... 23 4.3 Operators ......................................... 24 4.3.1 Operator Overloading [¹.⁰.¹.¹²⁰] .......................... 26 4.3.2 Helper Methods [¹.⁰.¹.¹²⁰] .............................. 27 4.4 Comments ......................................... 28 4.5 Memory Model ...................................... 29 4.6 Code Structure ...................................... 30 4.6.1 Unit Namespaces [¹.¹.⁰.⁹¹¹] ............................. 31 4.7 Property Expressions [¹.¹.⁰.⁹¹¹] .............................. 32 4.8 Properties with Anonymous Storage [¹.¹.⁰.⁹¹¹] ...................... 35 4.9 Contracts ......................................... 36 4.10 Directives ......................................... 37 4.10.1 Conditional Compilation .............................. 37 4.10.2 Conditional Compilation Symbols ......................... 38 4.10.3 Code Inclusions ................................... 38 4.10.4 Errors and Messages ................................ 39 4.11 Predefined Constants ................................... 40 4.12 Built-in Functions ..................................... 41 4.12.1 Mathematical Functions .............................. 41 4.12.2 String Functions .................................. 45 4.12.3 Date/Time Functions [¹.⁰.¹.¹²⁰] ........................... 48 4.13 Including JavaScript Code ................................ 51 4.13.1 Name Conflicts and Obfuscation Support ..................... 51 4.13.2 You May Be Better Without It ........................... 52 4.13.3 Implicit Parameters Structure ........................... 52 4.13.4 Variables ...................................... 52 CONTENTS 4.13.5 Handling Callbacks with Variant Methods .................... 53 4.13.6 Handling Callbacks in an asm section ....................... 54 4.13.7 Limitations ..................................... 54 5 Programming with Smart ................................... 55 5.1 My First Smart Program ................................. 57 5.2 File Management ..................................... 60 5.3 Deploying Smart Programs ................................ 63 5.4 Application Architecture ................................. 64 5.5 Forms and Navigation .................................. 65 5.5.1 Creating Secondary Forms ............................. 65 5.5.2 Navigating Between Forms ............................ 66 5.6 Message Dialogs ...................................... 68 5.6.1 ShowMessage .................................... 68 5.6.2 ShowDialog ..................................... 69 5.7 Modal Dialogs [¹.¹.⁰.⁹¹¹] .................................. 73 5.7.1 ShowModal ..................................... 73 5.7.2 HideModal ..................................... 75 5.8 Running Code on Application Shutdown ........................ 76 5.9 Themes and Styles .................................... 78 5.10 Command-line Applications ............................... 80 5.10.1 Example ....................................... 81 5.11 Writing Games ...................................... 83 5.11.1 Anatomy of a Game Project ............................ 83 5.11.2 Galaxy Clock .................................... 84 5.11.3 Alternatives to the Game Project ......................... 87 6 Smart Controls ......................................... 88 6.1 Overview ......................................... 89 6.2 TW3TagObj ........................................ 92 6.3 TW3Component ...................................... 93 6.3.1 Public Methods [¹.¹.⁰.⁹¹¹] .............................. 93 6.4 TW3MovableControl ................................... 94 6.5 TW3CustomControl .................................... 97 6.5.1 Public Methods ................................... 97 6.5.2 Public Properties .................................. 97 6.5.3 Events ........................................ 98 6.5.4 Published Properties ................................103 6.6 TW3GraphicControl ...................................105 6.7 Styling with CSS .....................................106 6.8 Button ...........................................107 6.8.1 Button with Image .................................107 6.8.2 Sample Application .................................108 CONTENTS 6.9 Checkbox .........................................110 6.9.1 Sample Application .................................111 6.10 ComboBox .........................................113 6.10.1 Sample Application .................................114 6.11 EditBox ..........................................116 6.11.1 Sample Application .................................117 6.12 Header ...........................................120 6.12.1 Sample Application .................................120 6.13 HTML Elements [¹.¹.⁰.⁹¹¹] .................................122 6.13.1 Sample Aplication .................................122 6.14 Image ...........................................125 6.14.1 Sample Application .................................126 6.15 Label ............................................128 6.15.1 Sample Application .................................129 6.16 Listbox [¹.¹.⁰.⁹¹¹] ......................................131 6.16.1 Listbox Styles ....................................133 6.16.2 Sample Application .................................134 6.17 ListMenu ..........................................137 6.17.1 Sample Application .................................138 6.18 Memo ...........................................140 6.18.1 Sample Application .................................140 6.19 PaintBox ..........................................142 6.19.1 Sample Application .................................142 6.20 Panel ............................................144 6.20.1 Sample Application .................................144 6.21 Progress bar [¹.¹.⁰.⁹¹¹] ...................................147 6.21.1 Sample Application .................................147 6.22 Scroll bar [¹.¹.⁰.⁹¹¹] .....................................150 6.22.1 Sample Application .................................151 6.23 Scrollbox [¹.¹.⁰.⁹¹¹] .....................................154 6.23.1 Sample Application .................................154 6.24 Scroll Control .......................................157 6.24.1 Sample Application .................................158 6.25 TeeChart [¹.¹.⁰.⁹¹¹]
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages274 Page
-
File Size-